Common Maven Mistakes New Java EE Developers Make
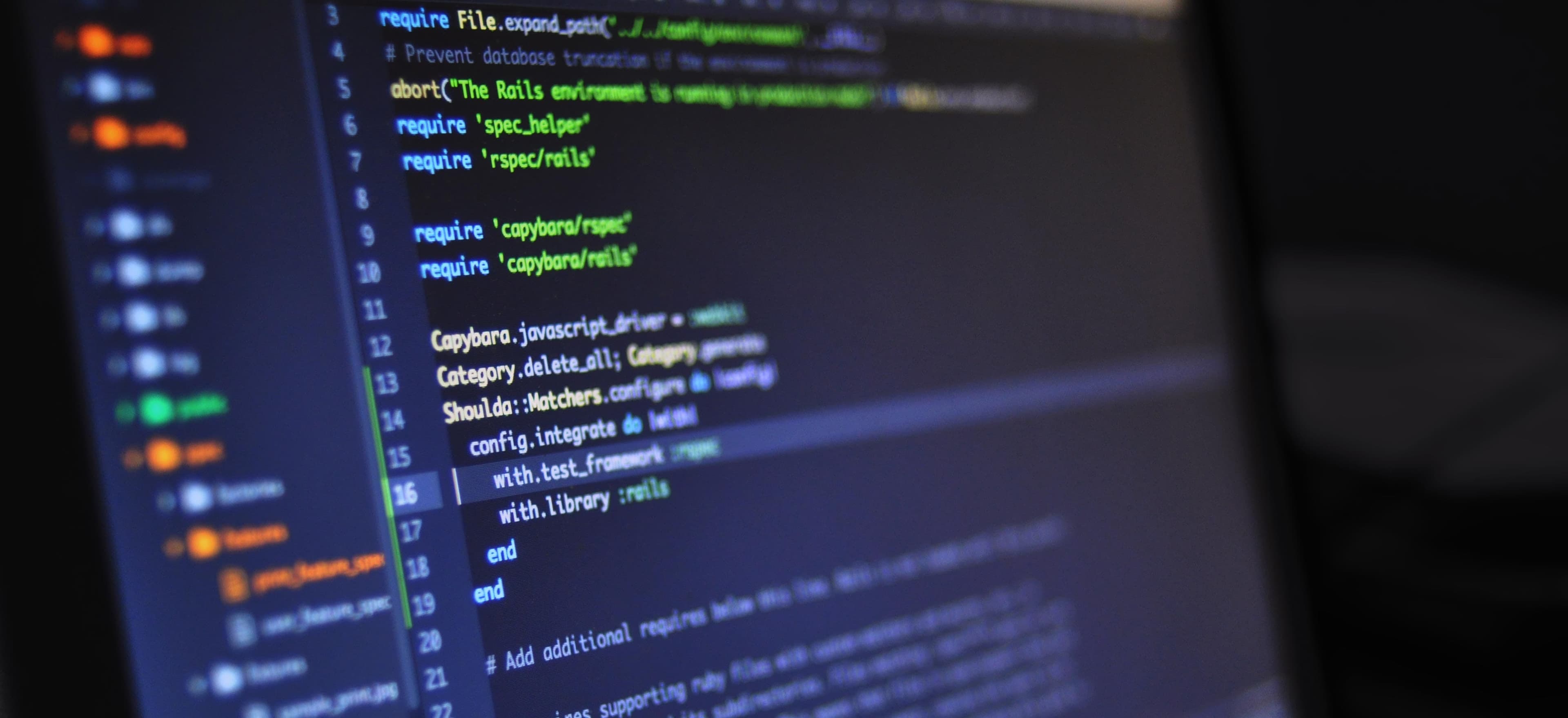
- Published on
Common Maven Mistakes New Java EE Developers Make
A Brief Overview
Maven is a powerful tool that streamlines project management and builds automation for Java projects. However, as with any tool, its utilization can lead to pitfalls, especially for new Java EE developers. In this blog post, we will explore common mistakes made with Maven and provide solutions to help you navigate these challenges.
What is Maven?
Maven is a build automation tool designed primarily for Java projects. It addresses two critical aspects of project management: project descriptions and dependency management. With Maven, developers can efficiently manage project lifecycles, including compilation, testing, packaging, and deployment, all with minimal configuration.
Mistake #1: Ignoring the POM File
The Project Object Model (POM) file is the heart of any Maven project, encapsulating configurations, dependencies, and plugins.
Explanation
New developers often overlook the importance of the pom.xml
file. This is a critical mistake as it hampers proper management of dependencies and project settings.
Solution
Always familiarize yourself with the structure and significance of the POM file. Here is a basic example:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Commentary
In this snippet, while creating a simple Java application artifact, we are also defining a test dependency on JUnit. This tells Maven to fetch JUnit whenever we run our tests, thus simplifying our build process.
Mistake #2: Forgetting to Update Dependencies
Maven simplifies dependency management, but it can lead to developers sticking with outdated libraries unintentionally. New developers might neglect to regularly update dependencies, which can increase security risks and compatibility issues.
Solution
Utilize the command mvn versions:use-latest-releases
and mvn versions:display-dependency-updates
to check for and implement updates. Regular maintenance of dependencies keeps your project secure and up-to-date.
Mistake #3: Not Understanding Scope
Maven dependencies come with specific scopes, such as compile, provided, runtime, test, and system. Unfortunately, many new developers don't fully understand these distinctions.
Explanation
Each scope affects how your dependencies are treated at different stages of the build process. For instance, a dependency marked with the "test" scope will not be included in the final packaged artifact.
Solution
Learn the implication of each scope. Here’s a more detailed example:
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
<scope>runtime</scope>
</dependency>
Commentary
In this example, slf4j-api
with a "runtime" scope gets included in the runtime classpath but is not included at compile time. This saves bandwidth and keeps your build clean, allowing for optimized deployment.
Mistake #4: Overly Complex POM Files
New developers sometimes make the mistake of over-architecting their POM files, embedding unnecessary complexity.
Explanation
While it can be tempting to include many plugins and configurations, over-complicated POMs can lead to maintenance headaches.
Solution
Start simple. Only include what you need and refactor as necessary. A clean, readable POM file will facilitate easier updates and debugging.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
Commentary
This snippet configures the Maven Compiler Plugin to compile to Java 1.8. It is straightforward and serves its purpose without unnecessary frills.
Mistake #5: Not Using a Local Repository
One of the best features of Maven is its capability to manage local and remote repositories. New developers often fail to understand how to effectively use a local repository, which can negatively impact build performance.
Explanation
Every time a build is executed, Maven checks for dependencies in specific repositories. If these dependencies are not tracked locally, Maven accesses the internet to download them, slowing down the build process.
Solution
Leverage the local repository and implement caching strategies. To utilize a local repository, adjust your settings.xml
file located in the Maven conf
directory or your user home .m2
directory.
Mistake #6: Failing to Leverage Profiles
Maven allows for distinct configurations using profiles, which enable developers to create multiple build configurations for different environments (development, testing, production).
Explanation
Developers may not be aware of this feature and might end up running the same configurations in different environments, which is inefficient and error-prone.
Solution
Utilize profiles effectively by defining environment-specific configurations.
<profiles>
<profile>
<id>development</id>
<properties>
<!-- Define dev-specific properties -->
</properties>
</profile>
<profile>
<id>production</id>
<properties>
<!-- Define prod-specific properties -->
</properties>
</profile>
</profiles>
Commentary
Profiles allow you to run specific configurations with a single command, such as mvn clean install -Pdevelopment
.
Lessons Learned
As a new Java EE developer, understanding Maven is essential for effective project management. By avoiding the common mistakes outlined here, you can leverage Maven's capabilities to streamline your Java projects, promote better practices, and enhance your development experience.
Further Reading
For an in-depth understanding of Maven, consider checking out Maven: The Complete Reference or the official Maven documentation.
By recognizing these pitfalls and equipping yourself with the right knowledge, you will undoubtedly become more proficient in Maven, enhancing your Java development skills. Happy coding!