Troubleshooting Common Issues in Spring Cloud Rest Client Calls
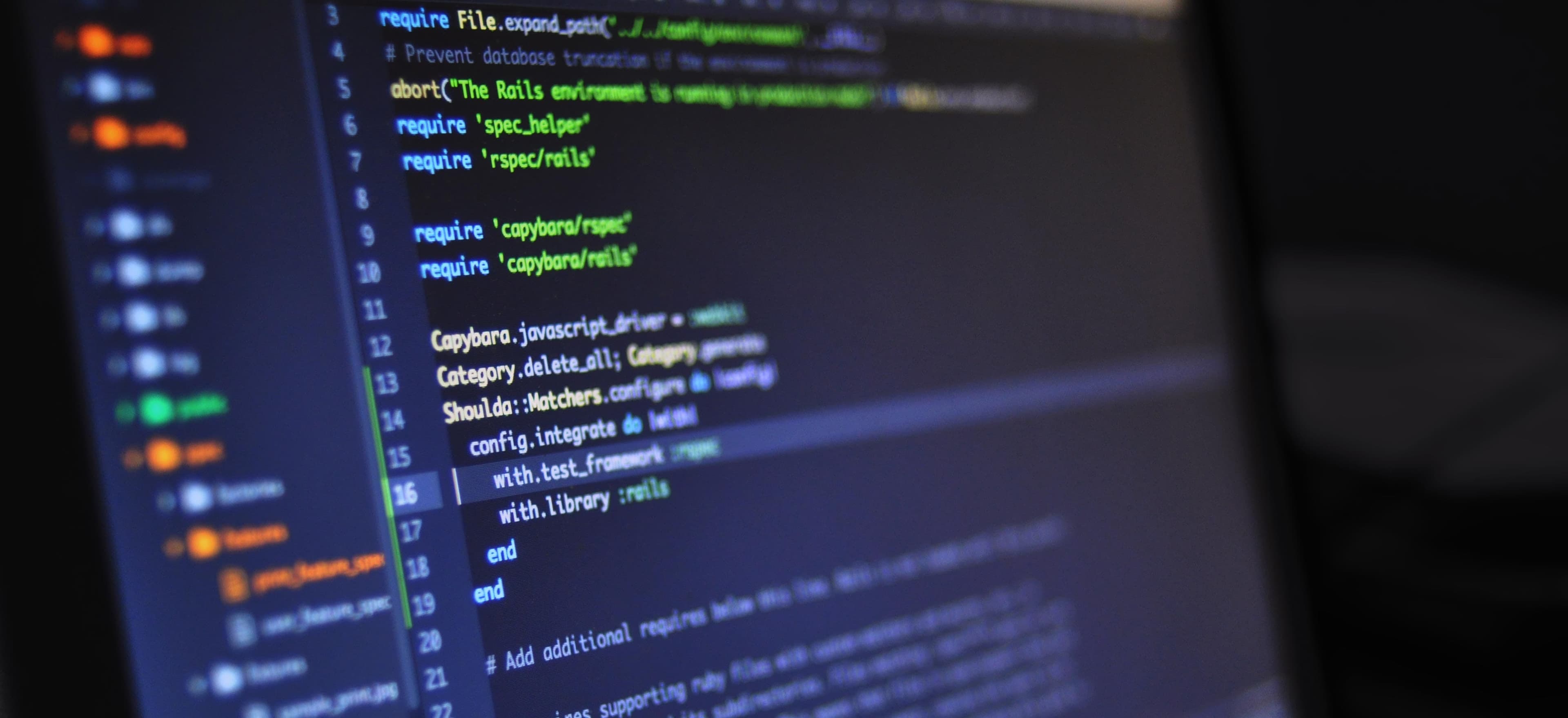
- Published on
Troubleshooting Common Issues in Spring Cloud Rest Client Calls
In the microservices architecture, building robust and efficient service-to-service calls is critical. Spring Cloud provides powerful tools to handle these scenarios with ease. However, when working with Spring Cloud Rest Client calls, you may encounter some common pitfalls that can lead to unexpected behavior. This blog post will help you identify, troubleshoot, and solve issues effectively in your Spring Cloud Rest Client calls.
Essentials at a Glance to Spring Cloud Rest Client
Spring Cloud Rest Client simplifies the process of making REST API calls to other microservices. It wraps the basic RestTemplate and allows you to implement service discovery, load balancing, and circuit breaker patterns.
To get started, ensure you have the necessary dependencies in your pom.xml
:
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
These dependencies set up your project with OpenFeign (for creating REST clients) and Eureka (for service discovery).
Common Issues Encountered
Understanding the common issues is crucial for effective troubleshooting. Below are typical problems developers face, along with their solutions.
1. Service Registration Issues
Symptoms
Your service might not be able to locate other services, leading to 404 Not Found
errors.
Troubleshooting Steps
- Check Eureka Configuration: Ensure that Eureka server is configured properly and your client is registering.
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka/
- Verify Instances: Check if your service is visible in the Eureka dashboard (usually at
http://localhost:8761
).
2. Load Balancing Problems
Symptoms
You are receiving the same instance despite having multiple service instances running.
Troubleshooting Steps
- Load Balancer Configuration: Make sure you are using the
@LoadBalanced
annotation.
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
return new RestTemplate();
}
- Client Configuration: Use
@FeignClient
correctly by assigning a correct name corresponding to the services registered in Eureka.
@FeignClient(name = "user-service")
public interface UserServiceClient {
@GetMapping("/users/{id}")
User getUserById(@PathVariable("id") String id);
}
3. Timeout Issues
Symptoms
API calls are taking too long, eventually resulting in a timeout exception.
Troubleshooting Steps
- Increase Timeout Settings: Modify the timeout parameters in your application properties.
feign:
client:
config:
default:
connectTimeout: 5000
readTimeout: 10000
- Network Monitoring: Monitor your network and service metrics to identify bottlenecks.
4. Handling Errors Gracefully
Symptoms
API calls may fail due to various reasons, but your application lacks error handling.
Troubleshooting Steps
- Implement Fallbacks: Use
@FeignClient
fallback attribute to provide an alternate method during failures.
@FeignClient(name = "user-service", fallback = UserServiceFallback.class)
public interface UserServiceClient {
@GetMapping("/users/{id}")
User getUserById(@PathVariable("id") String id);
}
@Component
class UserServiceFallback implements UserServiceClient {
@Override
public User getUserById(String id) {
return new User(); // returning an empty User as fallback
}
}
5. Serialization/Deserialization Issues
Symptoms
You are getting HttpMessageNotReadableException
or HttpMessageNotWritableException
.
Troubleshooting Steps
-
Check JSON Structure: Ensure the structure of the incoming/outgoing JSON matches the model classes.
-
Consider Annotations: Use Jackson annotations like
@JsonProperty
to map fields appropriately.
@JsonProperty("user_id")
private String id;
6. Dependency Issues
Symptoms
You are experiencing class loading issues or missing beans.
Troubleshooting Steps
-
Check Dependency Versions: Confirm that your Spring Boot and Spring Cloud versions are compatible. Use the Spring Initializr to find suitable versions.
-
Dependency Exclusions: Avoid version conflicts by excluding certain transitive dependencies if needed.
Wrapping Up
In a distributed system, issues are bound to arise. Understanding common problems with Spring Cloud Rest Client calls allows you to react quickly and maintain service reliability. By applying these troubleshooting steps, you will enhance your application’s resilience and responsiveness.
To delve deeper into Spring Cloud and microservices architecture, consider visiting Spring Cloud Documentation for extensive resources and examples.
This post aimed to provide a structured approach to troubleshooting Spring Cloud Rest Client calls. By leveraging the solutions offered, you can develop a more robust application while minimizing downtime and improving user experience. Keep experimenting and learning, and happy coding!