Overcoming Application Scalability Challenges in 2023
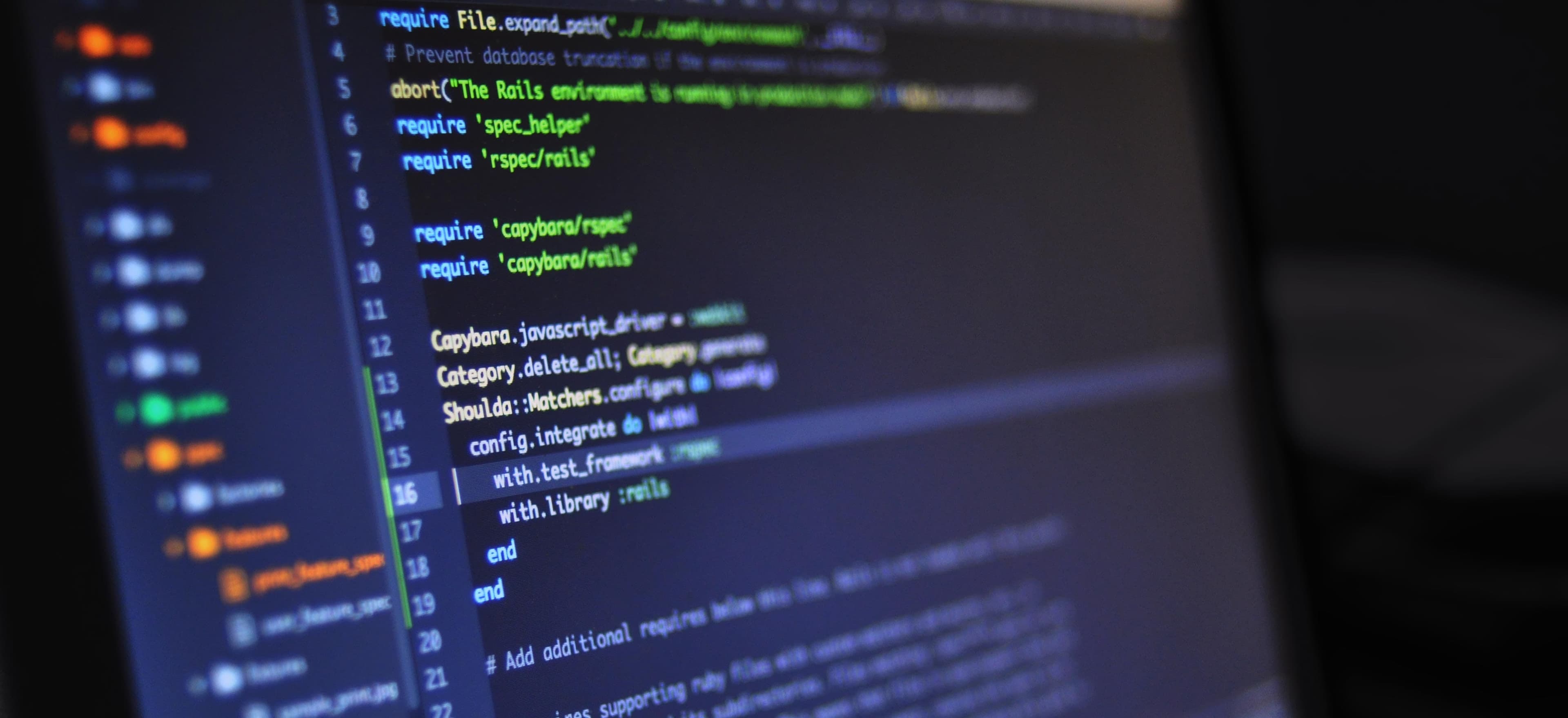
- Published on
Overcoming Application Scalability Challenges in 2023
In today's fast-paced digital landscape, application scalability has become a primary concern for developers and businesses alike. As user demographics shift and application usage surges, understanding the challenges of scaling applications—and knowing how to overcome them—can significantly impact performance and user satisfaction. This blog will delve into the common scalability challenges, emerging trends, and practical solutions to ensure that your applications remain robust and user-friendly in 2023.
What is Application Scalability?
Application scalability refers to the capability of a system to handle a growing amount of work or its potential to accommodate growth. There are two primary types of scalability:
- Vertical Scalability (Scaling Up): Adding more resources (CPU, RAM) to a single server.
- Horizontal Scalability (Scaling Out): Adding more servers to distribute the load of work.
Understanding these types is crucial, as they influence design decisions from the get-go.
The Landscape of Scalability Challenges in 2023
As we navigate through 2023, several challenges may arise when attempting to scale applications:
1. Increased User Load
The rise in remote work and online services has led to spikes in user load, pushing the limits of existing architectures. Consumers expect fast, responsive applications across different devices.
2. Data Management
Managing large datasets efficiently becomes crucial, especially when applications grow. Techniques such as caching, sharding, and replication are essential.
3. Cloud Costs and Resource Allocation
While the cloud provides remarkable flexibility, it can also lead to spiraling costs if resource usage isn’t continually monitored and optimized.
4. Microservices Complexity
While microservices provide modularity, they introduce complexity in communication and deployment.
5. Latency Issues
As an application increases in user base, latency can hurt user experience. Network-related delays become a big concern that developers must tackle effectively.
Strategies for Overcoming Scalability Challenges
Embrace Cloud Native Infrastructure
Using cloud-native architectures can greatly enhance scalability. Cloud platforms like AWS, Google Cloud, and Azure offer tools that allow dynamic scaling.
Example: AWS Auto Scaling
// Simple Java class to retrieve current instance count using AWS SDK
public class AwsAutoScaling {
public int getInstanceCount(String autoScalingGroupName) {
AmazonAutoScaling autoScaling = AmazonAutoScalingClientBuilder.defaultClient();
DescribeAutoScalingGroupsRequest request =
new DescribeAutoScalingGroupsRequest().withAutoScalingGroupNames(autoScalingGroupName);
DescribeAutoScalingGroupsResult response = autoScaling.describeAutoScalingGroups(request);
return response.getAutoScalingGroups().size();
}
}
Here, we use the AWS SDK for Java to retrieve the number of instances in an auto-scaling group. This provides a straightforward insight into whether additional resources are necessary.
Implement Load Balancing
Load balancing ensures no single server bears too much load. It can be achieved at various levels, including DNS level and application level.
Example: Setting Up a Load Balancer in Nginx
http {
upstream backend {
server backend1.example.com;
server backend2.example.com;
}
server {
location / {
proxy_pass http://backend;
}
}
}
This Nginx configuration enables load balancing. By using upstream blocks, we can route traffic effectively between multiple backend servers, ensuring that no single server is overwhelmed.
Optimize Database Performance
As your application scales, ensure your database can handle the increased query load. Techniques like indexing, query optimization, and using a distributed database can help.
Example: Using Indexing in SQL
CREATE INDEX idx_user_email ON users(email);
Creating an index on certain columns, such as email in this case, dramatically improves query performance, especially for larger datasets.
Utilize Caching Mechanisms
Caching is a powerful technique to reduce database load and decrease latency. Redis and Memcached are popular choices that can store frequently accessed data.
Example: Implementing Caching with Redis
import redis.clients.jedis.Jedis;
public class CacheManager {
private Jedis jedis;
public CacheManager() {
this.jedis = new Jedis("localhost");
}
public void cacheData(String key, String value) {
jedis.set(key, value);
}
public String getData(String key) {
return jedis.get(key);
}
}
In the above example, we create a simple CacheManager class using Redis. By caching data that is frequently requested, we can minimize the load on the main database and reduce latency.
Adopt Event-Driven Architecture
By embracing an event-driven architecture, you decouple services and allow for asynchronous processing. This pattern can significantly improve system responsiveness under load.
Example: Using Spring Boot for Event-Driven Programming
import org.springframework.context.event.EventListener;
import org.springframework.stereotype.Component;
@Component
public class UserRegistrationListener {
@EventListener
public void handleUserRegistration(UserRegistrationEvent event) {
// Process registration in a separate thread
CompletableFuture.runAsync(() -> processRegistration(event));
}
private void processRegistration(UserRegistrationEvent event) {
// Logic to handle user registration
}
}
In this example, the UserRegistrationListener
listens for user registration events and processes them asynchronously, which improves performance during peak loads.
Continuous Monitoring and Optimization
One of the most crucial components of overcoming scalability challenges is continuous monitoring of your application performance. Tools such as Prometheus, Grafana, and Datadog can provide insights into usage patterns and potential bottlenecks.
Additionally, implementing Continuous Integration/Continuous Deployment (CI/CD) pipelines can facilitate the rapid deployment of updates and optimizations when bottlenecks are detected.
Lessons Learned
The challenges of scalability are ever-evolving, and as applications continue to grow, developers must be proactive in addressing these challenges. By leveraging modern architecture and optimization techniques, you can ensure your application is well-equipped for the demands of 2023 and beyond.
As you consider your scalability strategy, take note of the resources mentioned in this post. For deeper insights on building scalable applications, check out AWS’s scalability documentation and Google Cloud’s guide on scaling applications.
Remember, the key to scalable applications is continuous learning and adaptation to new challenges. Equip yourself with the right tools, techniques, and mindset to stay ahead in this dynamic environment. Happy coding!
Checkout our other articles