Common Hibernate Pitfalls in Spring Boot Development
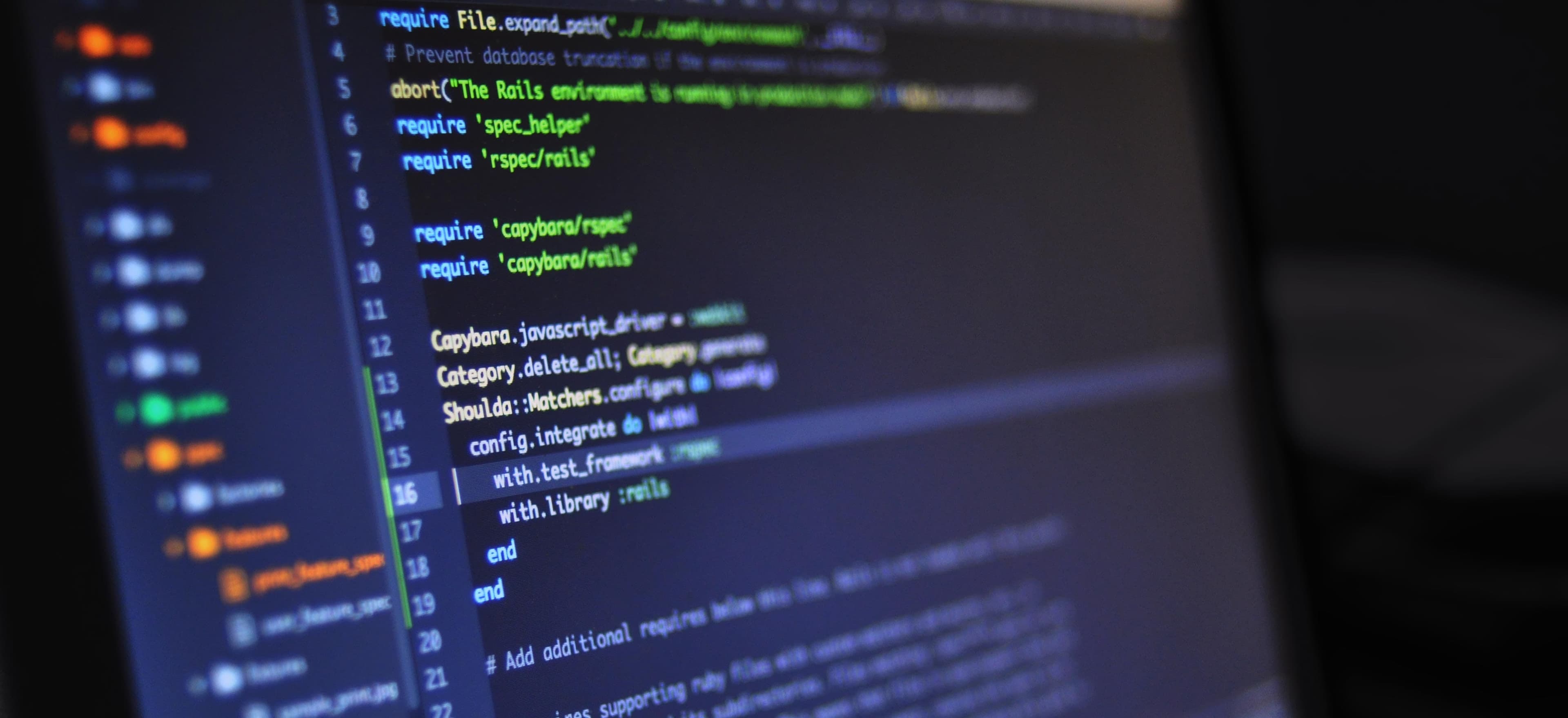
- Published on
Common Hibernate Pitfalls in Spring Boot Development
Hibernate, as an Object-Relational Mapping (ORM) tool, has gained exceptional popularity and adoption among Java developers, especially those using Spring Boot. While it can significantly simplify database interactions, builders often encounter various pitfalls owing to misconfigurations or misunderstanding its operational dynamics. In this post, we will explore common Hibernate pitfalls in Spring Boot development, providing insights and code examples to help you navigate these challenges effectively.
1. Ignoring Lazy Loading
One of Hibernate's powerful features is its ability to manage associations between entities with lazy loading. However, a common pitfall occurs when developers misinterpret how lazy loading operates, leading to LazyInitializationException
.
Understanding Lazy Loading
When an entity is fetched, its associated entities might not be loaded immediately. Instead, Hibernate waits until they are accessed (lazy loading). If the session is closed before accessing these associated collections, it results in an exception.
Code Example
@Entity
public class User {
@OneToMany(mappedBy = "user", fetch = FetchType.LAZY)
private List<Post> posts;
}
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public User getUser(Long id) {
return userRepository.findById(id).orElse(null);
// posts are not loaded here yet
}
}
Solution
To avoid LazyInitializationException
, ensure that you access the related objects while the session is still open. One approach is to use JOIN FETCH in your queries:
@Entity
public class User {
@OneToMany(mappedBy = "user", fetch = FetchType.LAZY)
private List<Post> posts;
//...
}
// Repository
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u JOIN FETCH u.posts WHERE u.id = :id")
User findUserWithPosts(@Param("id") Long id);
}
By using JOIN FETCH
, we ensure related entities are loaded within the same query execution, maintaining session integrity.
2. Not Leveraging Transaction Management
Another common mistake in Hibernate is neglecting transaction management. If you do not properly manage transactions, your database may become inconsistent, leading to various concurrency issues.
Understanding Transactions
Hibernate requires that all operations that manipulate the database are wrapped in transactions. In Spring Boot, you can use the @Transactional
annotation to manage this automatically.
Code Example
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
@Transactional // Ensures this method runs within a transaction
public User createUser(User user) {
return userRepository.save(user);
// transaction is committed after method execution
}
}
Solution
Always annotate service methods that perform data modifications with @Transactional
. This provides a reliable mechanism for handling commit and rollback operations, ensuring data consistency.
3. Unoptimized Queries
Hibernate can sometimes lead to performance bottlenecks if not configured correctly. Using complex relationships and large datasets can result in unoptimized queries, loading unnecessary data.
Diagnostic Tools
Using tools like Hibernate's SQL logging can provide insights into the actual queries being generated. You can enable SQL logging in your application.properties
with:
spring.jpa.show-sql=true
spring.jpa.properties.hibernate.format_sql=true
Code Example
Consider the following poorly optimized query:
public List<User> getAllUsers() {
return userRepository.findAll(); // Might load additional data
}
Solution
To improve optimization, consider using Pagination or Projections to reduce the volume of data loaded:
public Page<User> getUsers(Pageable pageable) {
return userRepository.findAll(pageable);
}
4. Misusing Entity Relationships
Hibernate supports several types of relationships, including One-to-One, One-to-Many, Many-to-One, and Many-to-Many. A common pitfall is misusing these relationships, which can lead to performance problems and incorrect data representation.
Example of Misunderstanding Relationships
If you declare a ManyToMany
relationship without managing the join table, you may face unintended consequences.
Code Example
@Entity
public class Course {
@ManyToMany(cascade = CascadeType.ALL)
private List<Student> students; // May not manage join table properly
}
Solution
Explicitly control join tables using the @JoinTable
annotation, ensuring that entities behave as expected:
@Entity
public class Course {
@ManyToMany
@JoinTable(
name = "course_student",
joinColumns = @JoinColumn(name = "course_id"),
inverseJoinColumns = @JoinColumn(name = "student_id")
)
private List<Student> students;
}
5. Versioning and Optimistic Locking
Another pitfall arises when concurrent modifications are made to the same entity instance. To manage this, Hibernate provides optimistic locking through versioning.
Code Example
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Version // This field will maintain the versioning
private Integer version;
// other properties...
}
Explanation
By adding a @Version
field, Hibernate can automatically handle concurrent updates, throwing an OptimisticLockException
if a conflict is detected. This pattern is essential for maintaining data integrity in concurrent environments.
In Conclusion, Here is What Matters
Hibernate is a powerful tool integrated seamlessly into Spring Boot applications. However, understanding its complexities and avoiding common pitfalls is essential for building robust applications.
To recap, always ensure proper transaction management, watch out for lazy loading pitfalls, optimize your queries, carefully manage entity relationships, and implement versioning for concurrent updates.
For further reading, consider exploring the official Spring Data JPA documentation and Hibernate documentation. These resources can provide you with deeper insights into making the most of Hibernate within your Spring Boot projects.
By actively addressing these common pitfalls, you can unlock the full power of Hibernate and Spring Boot, ensuring efficient, maintainable, and performant applications. Happy coding!
Checkout our other articles