Microservices Meltdown: Managing Complexity in Scaling
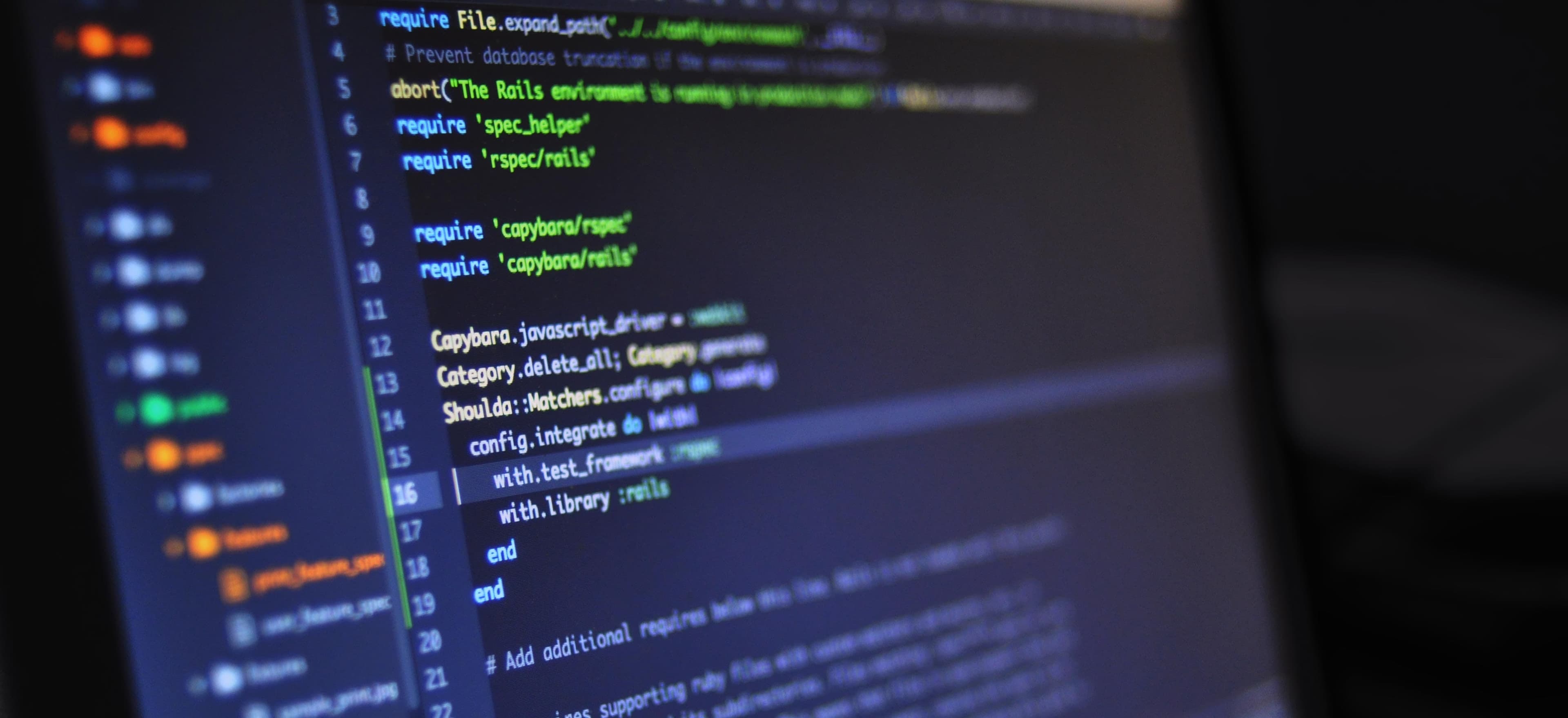
- Published on
Microservices Meltdown: Managing Complexity in Scaling
Microservices architecture has gained significant traction in recent years, heralded for its ability to enhance scalability, agility, and resilience in software engineering. However, implementing a microservices architecture can lead to complexities when scaling. This blog post delves into the strategies, best practices, and code examples that can help manage the intricacies associated with scaling microservices.
Understanding Microservices
Before diving into the scaling challenges, let's clarify what microservices are. Microservices is an architectural style that structures an application as a collection of small, loosely coupled services. Each service is independently deployable, focused on a specific business function, and can be developed using different programming languages and technology stacks.
Advantages of Microservices
- Scalability: Each microservice can be scaled independently according to its load and requirements.
- Resilience: Failure in one microservice doesn't necessarily bring down the entire application.
- Agility: Teams can work on different services simultaneously, leading to faster development cycles.
Disadvantages of Microservices
Despite the advantages, scalability often introduces complexity, leading to what we call a "microservices meltdown." Here are key challenges:
- Service Coordination: Managing communication between multiple services can become cumbersome.
- Data Management: Each service often operates on its own database, complicating data consistency.
- Deployment Complexity: Deploying and maintaining numerous services increases operational overhead.
Strategies for Managing Complexity
1. API Gateway
An API Gateway is a critical component in a microservices architecture that acts as a single entry point for all client requests. The gateway handles request routing, composition, and protocol translation.
@RestController
@RequestMapping("/api")
public class ApiGatewayController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/user/{id}")
public User getUser(@PathVariable String id) {
ResponseEntity<User> userResponse = restTemplate.getForEntity("http://user-service/users/" + id, User.class);
return userResponse.getBody();
}
}
Why Use an API Gateway?
- Concurrency Management: It can handle multiple simultaneous requests, reducing the load on individual services.
- Security: Centralized authentication can be enforced.
- Simplified Client Interaction: Clients can interact with a single service endpoint instead of multiple services.
2. Service Discovery
Service discovery is crucial in microservices where instances may need to scale up or down dynamically. Using a service registry like Eureka helps manage service instances.
@EnableEurekaClient
@SpringBootApplication
public class UserServiceApplication {
public static void main(String[] args) {
SpringApplication.run(UserServiceApplication.class, args);
}
}
Why Implement Service Discovery?
- Dynamic Instance Management: Automatically detects instance changes and adjusts accordingly.
- Load Balancing and Failover: Allows requests to be routed to healthy service instances.
3. Containerization
Using containers, primarily through Docker, allows microservices to be packaged with their dependencies.
FROM openjdk:11
COPY target/user-service.jar user-service.jar
ENTRYPOINT ["java", "-jar", "/user-service.jar"]
Why Containerize?
- Environment Consistency: Ensures that services run in the same way across different environments.
- Scalability: Containers can be quickly spun up or down based on load.
4. Monitoring and Logging
To manage complexity, having effective monitoring and logging in place is essential. Tools like Prometheus and ELK Stack can help in tracking service performance and health.
@RestController
@RequestMapping("/api/v1")
public class LoggingController {
private static final Logger logger = LoggerFactory.getLogger(LoggingController.class);
@GetMapping("/items")
public List<Item> getAllItems() {
logger.info("Fetching all items");
// Fetch items logic
}
}
Why Focus on Monitoring and Logging?
- Diagnosing Issues: Early fault detection helps prevent outages.
- Performance Metrics: Understanding usage patterns can inform scaling decisions.
5. Circuit Breaker Pattern
To manage failures in a microservices environment, the circuit breaker pattern can be incredibly valuable. It prevents an application from continually trying to execute an operation that's likely to fail.
@RestController
public class UserService {
@Autowired
private Resilience4jService resilience4jService;
@CircuitBreaker
public User getUser(String id) {
return resilience4jService.fetchUser(id);
}
}
Why Use the Circuit Breaker Pattern?
- Fail-fast Strategy: Prevents cascading failures across services.
- Graceful Degradation: The system can still provide partial functionality.
6. Event-Driven Architecture
An event-driven architecture allows services to communicate asynchronously and react to events, rather than tightly coupling them through direct calls. Using a message broker like Apache Kafka can facilitate this communication.
@Component
public class UserEventProducer {
@Autowired
private KafkaTemplate<String, User> kafkaTemplate;
public void sendUserEvent(User user) {
kafkaTemplate.send("user-events", user);
}
}
Why Implement Event-Driven Architecture?
- Decoupling Services: Each service can operate independently and process events at its own pace.
- Scalability: It’s easier to scale service components based on demand.
Final Thoughts
Scaling microservices can indeed lead to complexities, but using the approaches outlined in this blog post can help manage that complexity effectively. From implementing an API Gateway and service discovery to adopting monitoring strategies and using event-driven architectures, your microservices can remain resilient and scalable.
The journey to mastering microservices is ongoing. Stay informed about the most recent trends and best practices in this evolving field. For further reading, you might check resources such as the Spring Microservices or Microservices.io websites.
Implementing these strategies will not only bolster your application but also enhance your team's ability to adapt in a rapidly changing environment. Embrace complexity with the right tools and practices, and you'll harness the true power of microservices architecture.