Streamline Your Code: Ditch Global Vars for Cleaner Calls
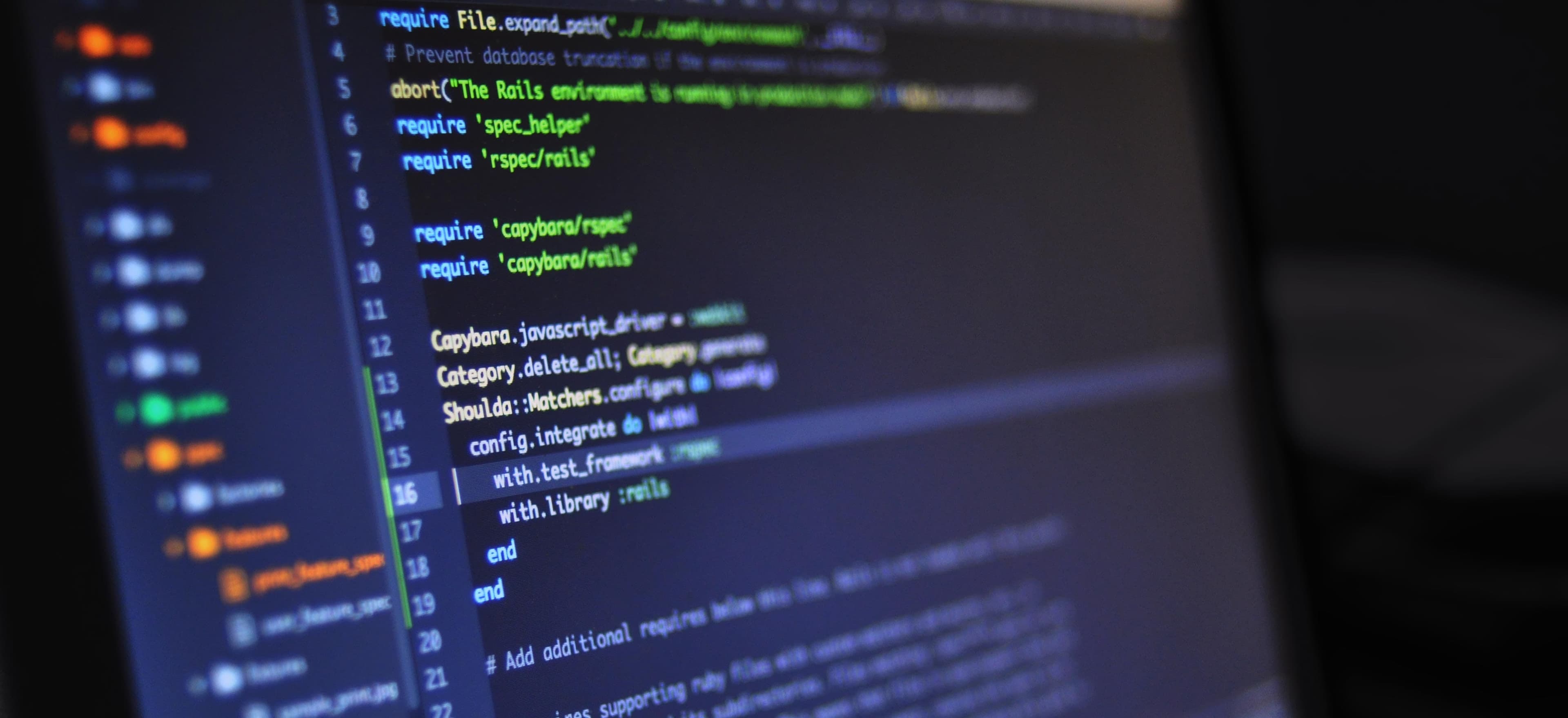
- Published on
Streamline Your Code: Ditch Global Vars for Cleaner Calls
In software development, especially in languages like Java, using global variables can lead to complicated and unmanageable code. While global variables might seem convenient at first glance, they can introduce a host of issues that can complicate your projects in the long run. In this blog post, we will explore the downsides of global variables and how you can streamline your code for better readability and maintainability through cleaner calls.
Understanding Global Variables
Global variables are variables that are accessible from any part of the code. This can lead to several problems:
- Namespace Pollution: Global variables occupy the global namespace, which can lead to name clashes and make debugging a nightmare.
- Hidden Dependencies: Functions that rely on global variables can be hard to understand or modify, since the context in which they are used changes based on the variable state.
- Difficult Testing: Functions that use global variables are harder to test in isolation, since the state of your application can impact test results unpredictably.
As a rule of thumb, it's best to restrict the scope of your variables as much as possible. So, how can we refactor our code to avoid global variables? Let’s dive into some practical examples.
Example 1: Using Function Parameters Instead of Global Variables
Let's say you are working on a simple banking application that processes transactions. Initially, you might rely on global variables to store your account balance.
Initial Code Using Global Variable
public class Bank {
private static double accountBalance = 1000.0; // Global variable
public static void deposit(double amount) {
accountBalance += amount; // Modifying global variable
System.out.println("Deposited: " + amount);
}
public static void withdraw(double amount) {
if (accountBalance >= amount) {
accountBalance -= amount; // Modifying global variable
System.out.println("Withdrew: " + amount);
} else {
System.out.println("Insufficient balance.");
}
}
public static void displayBalance() {
System.out.println("Current Balance: " + accountBalance);
}
}
Refactored Code
Instead of a global variable, we can manage the account balance using instance variables and method parameters. Below is the updated code.
public class Bank {
private double accountBalance; // Instance variable
public Bank(double initialBalance) {
this.accountBalance = initialBalance; // Set initial balance
}
public void deposit(double amount) {
accountBalance += amount; // Updating instance variable
System.out.println("Deposited: " + amount);
}
public void withdraw(double amount) {
if (accountBalance >= amount) {
accountBalance -= amount; // Updating instance variable
System.out.println("Withdrew: " + amount);
} else {
System.out.println("Insufficient balance.");
}
}
public void displayBalance() {
System.out.println("Current Balance: " + accountBalance);
}
}
Why This Works Better
- Encapsulation: The account balance is now tied to the instance of the
Bank
class. This limits the scope ofaccountBalance
, making the class easier to understand. - Easier Testing: You can create multiple instances of
Bank
with different account balances, making it much easier to test various scenarios. - Reduced Side Effects: Since
accountBalance
is no longer global, any method that modifies it does so in a clearly defined scope.
Example 2: Using Return Values
Another common situation where programmers might lean toward global variables is when maintaining a list or collection. Consider the following example where a global list is employed:
Initial Code Using a Global List
import java.util.ArrayList;
import java.util.List;
public class UserManagement {
private static List<String> users = new ArrayList<>(); // Global list
public static void addUser(String user) {
users.add(user); // Adding to global list
}
public static List<String> getUsers() {
return users; // Returning global list
}
}
Refactored Code
Now, let's refine this code to avoid the use of a global list.
import java.util.ArrayList;
import java.util.List;
public class UserManagement {
private List<String> users; // Instance variable
public UserManagement() {
users = new ArrayList<>(); // Initialize the list
}
public void addUser(String user) {
users.add(user); // Adding to instance variable
}
public List<String> getUsers() {
return new ArrayList<>(users); // Return a copy of the list
}
}
Why This Works Better
- Controlled Access: By returning a copy of the users list, we prevent callers from inadvertently modifying the internal state of the
UserManagement
instance. - Enhanced Clarity: Anyone working with the class can immediately understand that modifications are happening within a specific scope, thus increasing comprehension.
A Final Look: Embrace Locality and Modularity
Ditching global variables may require an initial investment of time in refactoring, but the long-term gains in code maintainability, readability, and testability far outweigh the costs. As we’ve seen, utilizing method parameters, returning values, and limiting variable scope can significantly elevate the quality of your Java code.
For further exploration of Java programming best practices, consider checking out Java Code Conventions, which emphasize the importance of writing clean and maintainable code.
If you would like to dive deeper into how encapsulation in object-oriented programming can streamline your code, the resource on Encapsulation in Java can provide additional insights.
Final Thoughts
By implementing the principles discussed, the next time you sit down to code, think twice before reaching for a global variable. The cleaner your calls and clearer your code, the more efficient your development process will be.
Checkout our other articles