Managing Task Overload: Throttling with BlockingExecutor
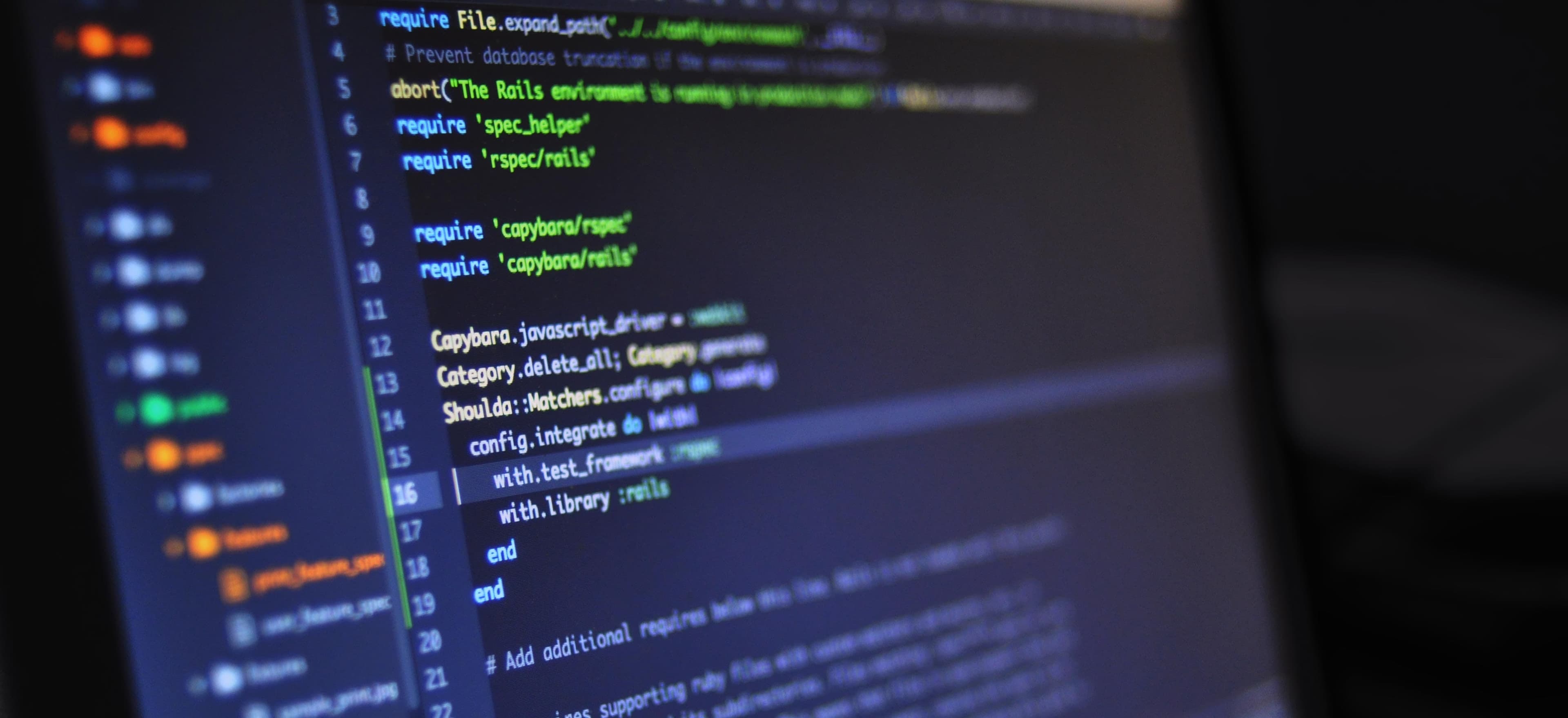
- Published on
Managing Task Overload: Throttling with BlockingExecutor
In today's fast-paced digital world, managing task overload is a challenge that every developer faces. The exponential growth of user demands often leads to situations where services become overwhelmed. To mitigate this problem, effective task management strategies are required. One such method involves using BlockingExecutor to throttle tasks. This approach can ensure that your applications remain responsive and can handle incoming load without crashing or slowing down significantly.
In this blog post, we will explore the concept of task throttling, delve into the details of the BlockingExecutor, and illustrate how you can implement throttling mechanisms using Java. Let's dive in!
Understanding Task Overload
Before we discuss the solutions for managing task overload, it's important to recognize what task overload entails. In a multi-threaded environment, task overload occurs when more tasks are submitted to a thread pool than the pool can execute concurrently. This situation can lead to several issues, such as increased latency, resource exhaustion, or even application failure.
The Importance of Throttling
Throttling is a strategic approach to limit the rate of task submissions to ensure that the system can handle the load effectively. By implementing throttling, we can:
- Maintain Stability: Keep the system stable and prevent crashes.
- Improve User Experience: Provide consistent response times to end users.
- Optimize Resource Usage: Ensure that system resources are utilized efficiently without being overwhelmed.
Introducing BlockingExecutor
In Java, the BlockingExecutor is a type of executor service that provides built-in throttling capabilities. This particular executor is designed to control the volume of tasks that can be submitted and executed concurrently, allowing you to avoid overwhelming the system.
Key Features of BlockingExecutor
- Capacity Limits: It allows you to set a maximum capacity for uncompleted tasks.
- Blocking Behavior: If the task queue is full, it can block new task submissions until there's available capacity.
- Configurability: The number of concurrent working threads can be configured, which facilitates fine-tuning for specific applications.
Now, let’s explore implementing a BlockingExecutor in Java.
Implementation Example
In this section, we will create a simple Java program to demonstrate how to use BlockingExecutor for throttling task submissions.
Step 1: Setting up the Environment
Before we begin coding, ensure you have the necessary Java environment set up. You need JDK 8 or higher installed on your machine. You can download it from the Official Oracle JDK.
Step 2: Code Snippet
Here is the full code for a simple BlockingExecutor implementation:
import java.util.concurrent.*;
public class BlockingExecutorExample {
public static void main(String[] args) {
// Configure the parameters for the BlockingExecutor
int corePoolSize = 2; // Number of concurrent threads for task execution
int maxQueueSize = 5; // Maximum number of tasks in the queue
// Create a blocking executor service
BlockingExecutor executor = new BlockingExecutor(corePoolSize, maxQueueSize);
for (int i = 0; i < 10; i++) {
final int taskId = i;
executor.submit(() -> {
try {
// Simulate task execution time
System.out.println("Executing task " + taskId);
Thread.sleep(2000);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
System.out.println("Completed task " + taskId);
});
}
executor.shutdown();
}
}
class BlockingExecutor {
private final ExecutorService executorService;
private final BlockingQueue<Runnable> blockingQueue;
public BlockingExecutor(int corePoolSize, int maxQueueSize) {
this.blockingQueue = new ArrayBlockingQueue<>(maxQueueSize);
this.executorService = new ThreadPoolExecutor(corePoolSize, corePoolSize,
0L, TimeUnit.MILLISECONDS, blockingQueue);
}
public void submit(Runnable task) {
try {
// Submit the task to the executor service
blockingQueue.put(task); // This will block if the queue is full
executorService.execute(task); // Execute the task immediately
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore the interrupted status
}
}
public void shutdown() {
executorService.shutdown();
try {
if (!executorService.awaitTermination(60, TimeUnit.SECONDS)) {
executorService.shutdownNow();
}
} catch (InterruptedException e) {
executorService.shutdownNow();
}
}
}
Step 3: Explanation of the Code
-
Executor Configuration: We define
corePoolSize
for the number of threads we want to run concurrently andmaxQueueSize
for how many tasks we can queue before blocking new submissions. -
Blocking Executor Logic: The
BlockingExecutor
class encapsulates theExecutorService
and manages task submissions with aBlockingQueue
. It uses theArrayBlockingQueue
to control the number of tasks in the queue. -
Blocking Submission: The
submit
method adds tasks to the queue usingblockingQueue.put(task)
. If the queue is full, this call blocks until space becomes available. -
Shutdown Management: The
shutdown
method will terminate the executor service gracefully.
Key Takeaways
- Utilize
BlockingExecutor
to manage task overload efficiently. - Prevent excessive task submission that can lead to system instability.
- Provide a scalable way to handle various numbers of tasks in a threaded environment.
Lessons Learned
Managing task overload is crucial for the performance and reliability of your applications. By using a BlockingExecutor, you can effectively throttle incoming tasks, ensuring that your infrastructure remains responsive under heavy load.
As systems and user demands continue to evolve, the implementation of sophisticated task management strategies will only become more critical. You can explore additional resources on Java Concurrency and consider other executor frameworks suitable for your specific use cases.
In your project, consider when to implement throttling and always aim for the right balance between system responsiveness and resource usage. Happy coding!
Checkout our other articles