Mastering Spring AI: Overcoming Output Mapping Challenges
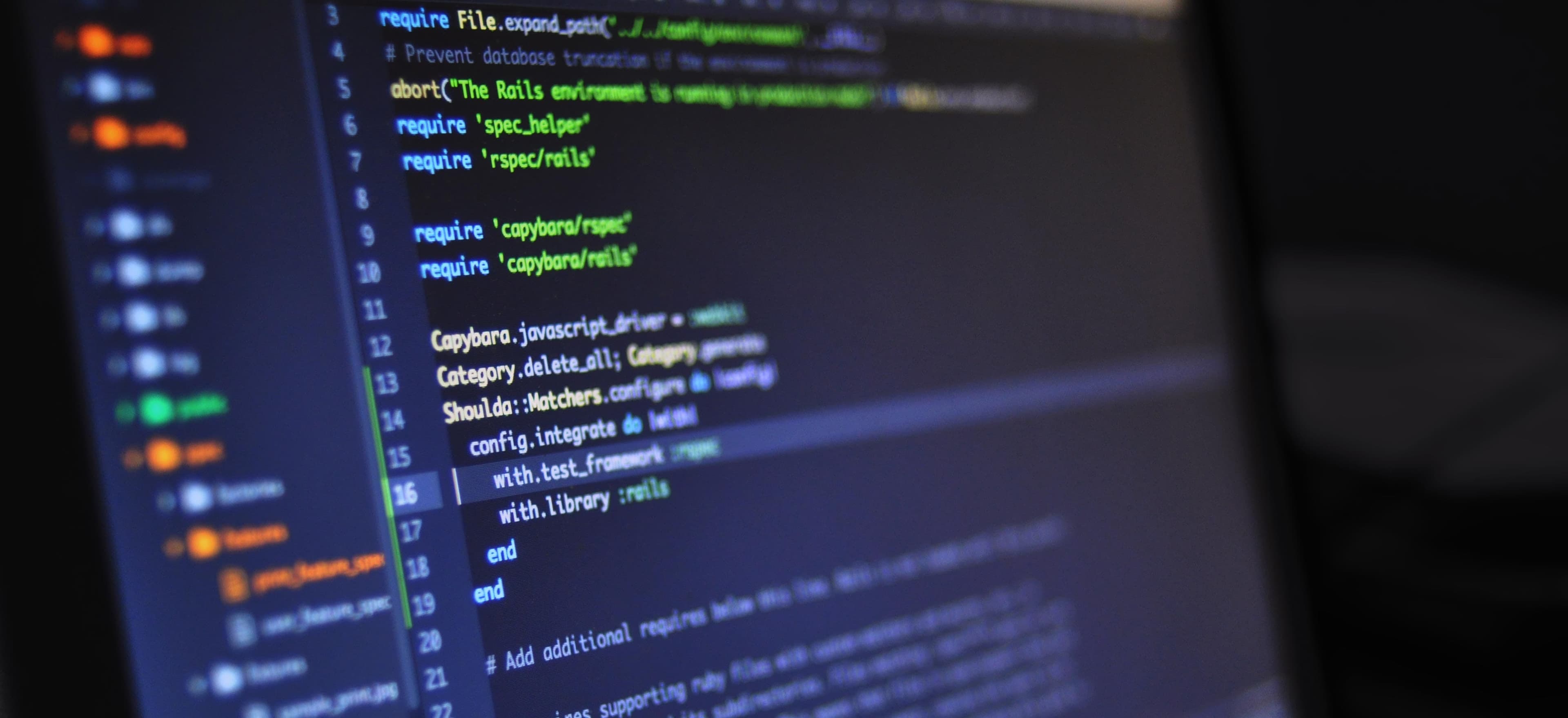
- Published on
Mastering Spring AI: Overcoming Output Mapping Challenges
In the evolving landscape of software development, the integration of artificial intelligence (AI) with frameworks like Spring is reshaping how we build applications. The Spring framework, renowned for its flexibility and robust architecture, is a perfect match for AI applications. However, as developers dive into AI, they often face challenges, particularly in output mapping. In this blog, we'll explore these challenges and provide practical solutions to master output mapping using Spring AI.
Understanding Output Mapping in Spring AI
Output mapping is the process of transforming the results produced by an AI model into a format that the application can use effectively. It involves converting raw data into a structured form, ensuring it's understandable and actionable in the context of your application.
The following points highlight the importance of effective output mapping:
- Data Usability: Ensures that the data returned by AI models can be easily consumed by clients or further processed by backend services.
- Efficiency: Maps large sets of data quickly, allowing for seamless integration with applications and user interfaces.
- Maintainability: Simplifies the changes needed if the AI model's output structure evolves over time, maintaining code clarity.
Common Output Mapping Challenges
Despite its significance, developers often grapple with several output mapping challenges when integrating AI models within a Spring application.
1. Complex Data Structures
AI models frequently return complex or nested data structures. Mapping these structures into a format suitable for user interfaces or business logic can become intricate.
2. Data Formatting Issues
Different data formats may be required for various components of your application. For example, output data for a web interface may differ from that required for storage in a database.
3. Model Updates
AI models may evolve, which means their output might change over time. Handling these updates gracefully is crucial for maintaining the integrity of your application.
4. Performance Considerations
Inefficient mapping can result in performance bottlenecks, leading to slower response times and a poor user experience.
Solutions for Effective Output Mapping
Having established the challenges of output mapping, let's explore some effective strategies to overcome these hurdles in a Spring AI application.
1. Use Data Transfer Objects (DTOs)
DTOs are a popular way to manage how data is transferred across the layers of your application. They simplify data handling and make mapping clear and straightforward.
Example: Simple DTO Implementation
public class UserDTO {
private String firstName;
private String lastName;
private String email;
// Constructors, Getters, and Setters
}
Here, the UserDTO
class provides a clear structure for transferring user information from your AI model to the frontend. By using DTOs, you abstract the complexity of your underlying model data, which can change over time.
2. Use Mapping Libraries
Libraries like MapStruct can help automate the mapping process between your models and DTOs. By defining mapping interfaces, you can delegate the heavy lifting to the library.
Example: MapStruct Mapping
@Mapper(componentModel = "spring")
public interface UserMapper {
UserDTO userToUserDTO(User user);
}
With MapStruct, you create a simple mapping interface that generates an implementation at compile-time. This approach saves time and reduces boilerplate code, ensuring your mappings are both efficient and maintainable.
3. Implement Custom Mappers as Needed
In some cases, you may need to implement custom mapping logic, especially for complex structures. Here’s a brief look at creating a custom mapping solution:
Example: Custom Mapping Logic
@Component
public class CustomModelMapper {
public UserDTO mapUserOutputToDTO(UserOutput userOutput) {
UserDTO userDTO = new UserDTO();
userDTO.setFirstName(userOutput.getName().split(" ")[0]);
userDTO.setLastName(userOutput.getName().split(" ")[1]);
userDTO.setEmail(userOutput.getContact().getEmail());
return userDTO;
}
}
In this example, the CustomModelMapper
class defines a method to map a complex UserOutput
returned by an AI model into a simpler UserDTO
. Custom logic allows for fine-tuning the mapping process where automated solutions fall short.
4. Handle Errors Gracefully
To ensure that any issues with mapping do not disrupt your application, include error handling. Consider logging mapping errors for further diagnosis.
Example: Error Handling during Mapping
public UserDTO safeMapUserOutputToDTO(UserOutput userOutput) {
try {
return mapUserOutputToDTO(userOutput);
} catch (Exception e) {
log.error("Mapping error: {}", e.getMessage());
return new UserDTO(); // return an empty DTO or handle appropriately
}
}
Using a try-catch block ensures that your application remains stable even when unexpected data formats are encountered.
5. Regularly Review and Refactor
The best practices in software development advocate for periodic review and refactor of your codebase. This applies to your mapping logic as well.
As models evolve, stay proactive by updating your mappings, ensuring they're still efficient and properly aligned with your application's requirements.
Final Thoughts
Navigating the intricacies of output mapping with Spring AI can be challenging, but by employing structured strategies, you can simplify this process. Using DTOs, mapping libraries, custom logic, and efficient error handling ensures your application can handle the complexities of AI seamlessly.
For those looking for additional resources and best practices, check out the Spring Framework Documentation and MapStruct Documentation. These platforms offer valuable insights and allow you to delve deeper into Spring AI development.
By mastering output mapping, you equip your Spring applications to harness the full potential of AI, driving performance, scalability, and an improved user experience. Embrace these techniques, and take significant steps toward creating robust AI-enabled applications.
Checkout our other articles