Troubleshooting RxNetty Access Issues with Meetup's API
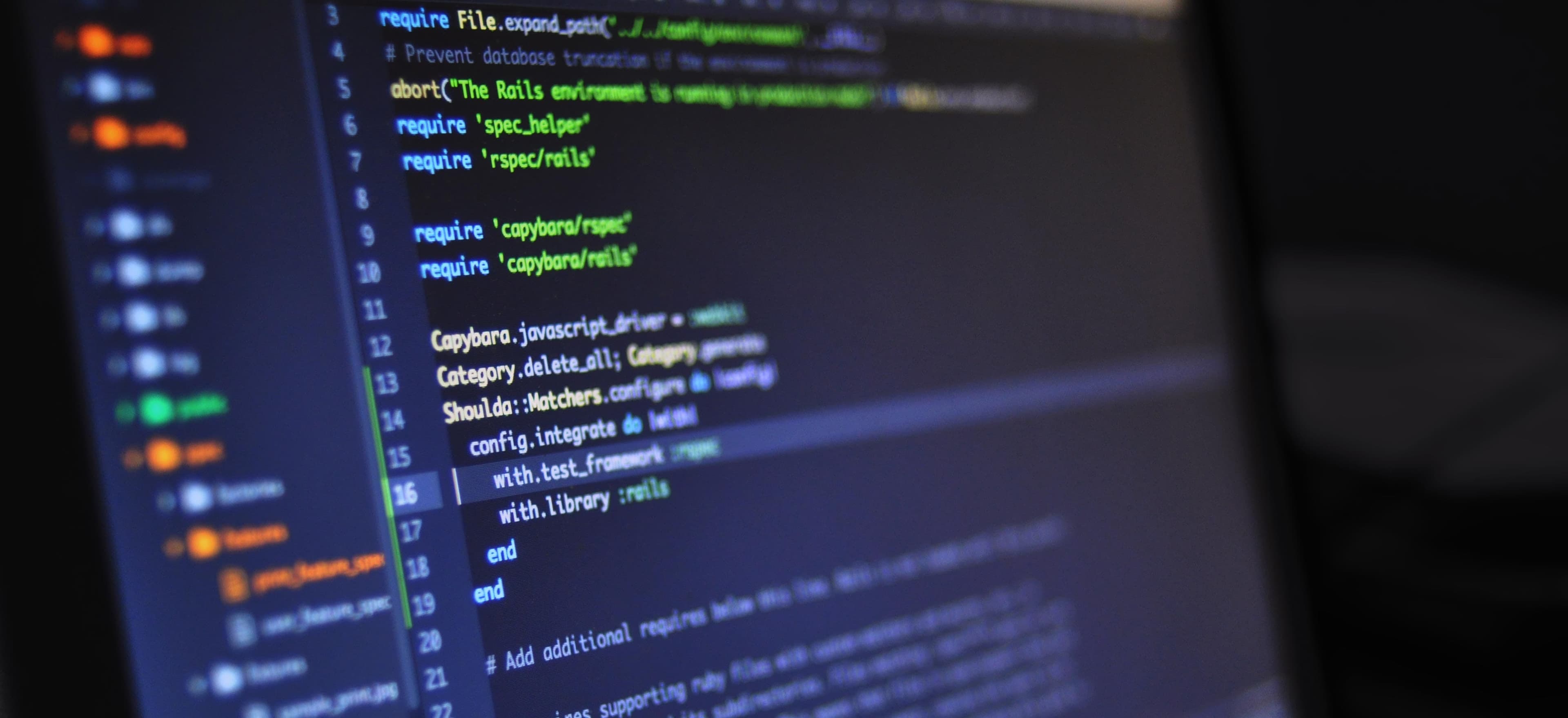
- Published on
Troubleshooting RxNetty Access Issues with Meetup's API
In modern application development, relying on robust APIs is key for enhancing functionality and user experience. Meetup's API allows developers to interact with its platform, retrieving event data, member information, and more. However, utilizing this API effectively often comes with its own set of challenges, especially when using frameworks like RxNetty.
In this blog post, we will walk through the common access issues encountered when integrating with the Meetup API using RxNetty. We will also provide code snippets to illustrate the solutions. By the end of this guide, you will have a deeper understanding of troubleshooting techniques that can help you overcome these challenges efficiently.
What is RxNetty?
Before we dive into troubleshooting, let's briefly explain what RxNetty is. RxNetty is an asynchronous networking framework based on the principles of reactive programming. It extends the capabilities of Netty, a popular networking library in Java, by adding reactive streams support. This makes RxNetty ideal for handling I/O operations with non-blocking behavior, which is essential when dealing with APIs that may have variable response times.
Common Access Issues with Meetup's API
When working with the Meetup API through RxNetty, several access issues may arise:
- Authentication Failures
- Rate Limiting
- Network Errors
- Response Parsing Issues
Let’s explore each of these issues in detail and provide solutions along the way.
1. Authentication Failures
The first hurdle many developers face is authentication. The Meetup API requires an OAuth2 token for most requests. If you are not passing this token correctly, you will likely receive a 401 Unauthorized error.
Solution
Ensure that you have obtained the OAuth2 token and are passing it in your headers. Here's a sample code snippet showing how to set up headers for an authorized request:
import io.reactivex.netty.protocol.http.client.HttpClient;
import io.reactivex.netty.protocol.http.client.HttpClientRequest;
import io.reactivex.netty.protocol.http.client.HttpClientResponse;
import rx.Observable;
public void makeAuthenticatedRequest(String token) {
HttpClient<ByteBuf, ByteBuf> client = HttpClient.newClient("api.meetup.com", 80);
HttpClientRequest<ByteBuf> request = HttpClientRequest.createGet("/path/to/endpoint")
.withHeader("Authorization", "Bearer " + token);
client.submit(request)
.flatMap(HttpClientResponse::getContent)
.subscribe(
responseContent -> System.out.println("Response: " + responseContent.toString()),
throwable -> System.err.println("Error during request: " + throwable.getMessage())
);
}
Commentary
In the above snippet:
- We create a new HTTP client for Meetup's API.
- Make a GET request and include the OAuth2 token in the Authorization header.
- The response is printed to the console, allowing you to confirm that your request was successful or to catch any errors.
2. Rate Limiting
Meetup enforces rate limiting to prevent abuse of their resources. If you exceed the allowed number of requests, you will receive a 429 Too Many Requests response.
Solution
Implement an exponential backoff strategy when retries are necessary. This will allow your application to gracefully handle the situation rather than resulting in a flood of requests.
import rx.Observable;
import rx.functions.Func1;
public Observable<Void> handleRateLimit(URL url, int attempts) {
return Observable.error(new RuntimeException("Rate limit exceeded"))
.flatMap(new Func1<Throwable, Observable<Void>>() {
@Override
public Observable<Void> call(Throwable throwable) {
if (attempts < 5) {
try {
Thread.sleep((long) Math.pow(2, attempts) * 1000); // Exponential backoff
} catch (InterruptedException e) {
return Observable.error(e);
}
return makeRequest(url, attempts + 1);
}
return Observable.error(new RuntimeException("Max attempts reached"));
}
});
}
Commentary
This code outlines how to implement exponential backoff:
- If the rate limit is hit, the function waits for a specified time before attempting again.
- The wait time increases exponentially with each retry, preventing further strain on the API.
3. Network Errors
Network connectivity issues can prevent your application from accessing the Meetup API. This can be due to various reasons, such as loss of internet connection or server downtime.
Solution
Proper error handling can help you manage such scenarios. Here’s a straightforward example:
client.submit(request)
.flatMap(HttpClientResponse::getContent)
.subscribe(
responseContent -> System.out.println("Response: " + responseContent.toString()),
throwable -> {
if (throwable instanceof IOException) {
System.err.println("Network error occurred: " + throwable.getMessage());
// Handle recovery logic here
} else {
System.err.println("Error during request: " + throwable.getMessage());
}
}
);
Commentary
In this code, we check if the error is a network-related issue:
- By specifically catching IOExceptions, we can handle this issue differently than other errors.
- It creates a clean separation of error types, allowing for precise handling of problems.
4. Response Parsing Issues
Another common issue developers face is parsing the API responses correctly. If the API's response structure changes and your parsing logic doesn't account for this, you may run into runtime exceptions.
Solution
Always validate the structure of the response before trying to parse it. Using JSON libraries like Jackson or Gson can make this easier, but here is a generic way of checking:
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public void processApiResponse(String jsonResponse) {
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode rootNode = objectMapper.readTree(jsonResponse);
if (rootNode.has("desiredField")) {
System.out.println("Field value: " + rootNode.get("desiredField").asText());
} else {
System.err.println("Expected field is missing in the response.");
}
} catch (IOException e) {
System.err.println("Failed to parse the JSON response: " + e.getMessage());
}
}
Commentary
In the above code:
- We parse the JSON response safely using Jackson.
- A check for the presence of a field helps avoid
NullPointerExceptions
. - This lays the foundation for robust error handling around response contents.
My Closing Thoughts on the Matter
Troubleshooting access issues with Meetup's API using RxNetty can initially seem like a daunting task. However, understanding common pitfalls—such as authentication, rate limiting, network errors, and response parsing—can significantly reduce downtime.
By implementing the solutions outlined in this article, you can create a much smoother integration experience. Remember to continuously monitor your application's health and usage patterns, optimizing your requests based on real-world feedback.
For more details on using Meetup’s API, feel free to explore the Meetup API Documentation. Happy coding!
Checkout our other articles