Solving Common Issues with Mutual TLS Authentication
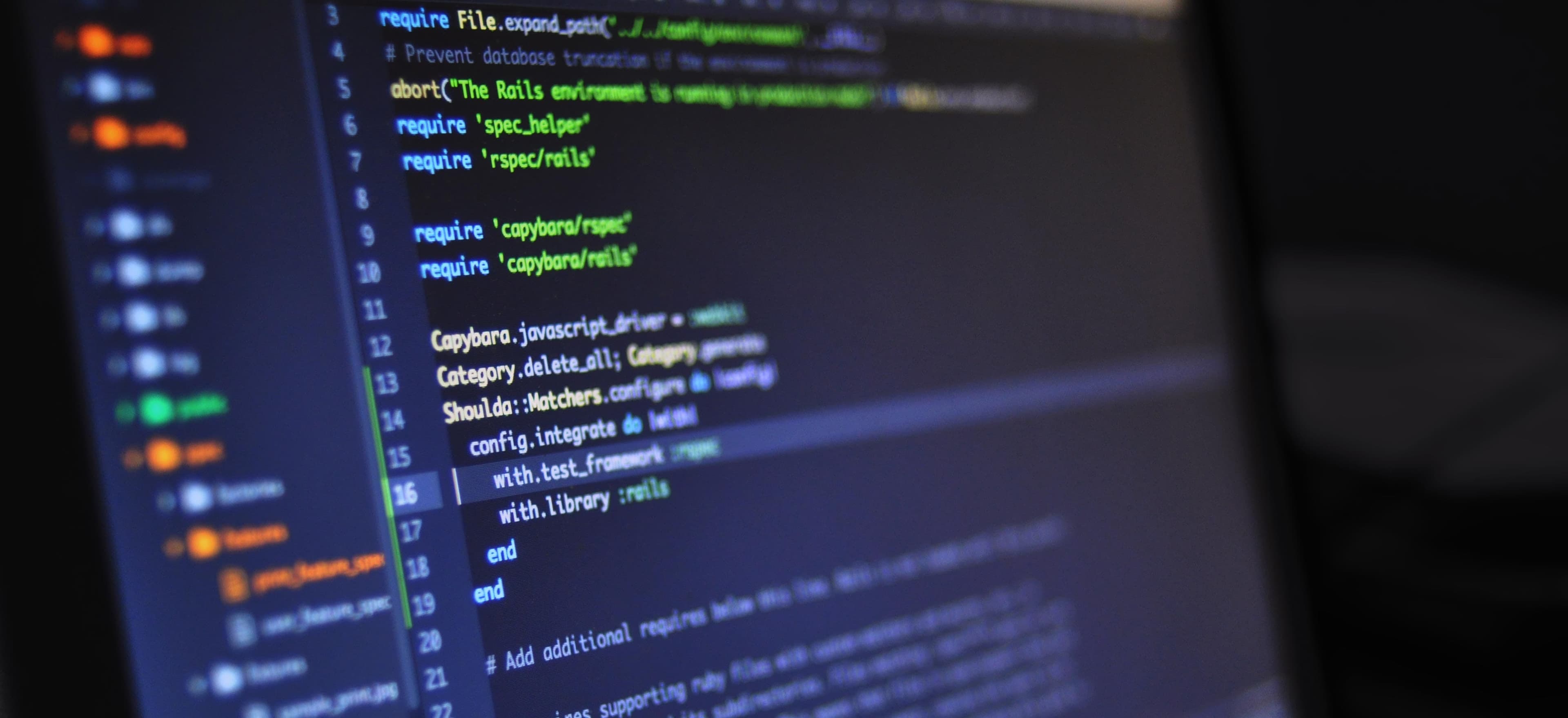
- Published on
Solving Common Issues with Mutual TLS Authentication
In today's cybersecurity landscape, ensuring secure communication between clients and servers is crucial. One method is Mutual TLS (mTLS) authentication, which enhances security by requiring both the client and server to authenticate each other's certificates. Although powerful, mTLS can introduce a set of challenges when trying to implement it. This blog post will help you navigate these issues, giving you clear solutions and code examples along the way.
Understanding Mutual TLS Authentication
Before diving into common issues, it is essential to understand what Mutual TLS is. At its core, mTLS uses Transport Layer Security (TLS) to secure communications. It works by:
- Server Authentication: The client verifies the server's identity by validating its certificate.
- Client Authentication: The server also verifies the client's identity with its certificate.
This two-way verification creates a secure channel that is less vulnerable to man-in-the-middle attacks.
For further reading on how TLS works, you can check out this detailed overview on TLS.
Common Issues With mTLS and Their Solutions
1. Certificate Validation Errors
One of the most common issues when implementing mTLS is certificate validation failures.
Why It Happens
Certificate validation errors can occur for several reasons, such as:
- Expired certificates
- Mismatched domain names
- Untrusted certificate authorities (CA)
Solution
To handle this, you need to ensure that:
- Certificates are renewed before expiration.
- Domains match the certificates being used.
- The client trusts the CA that issued the server's certificate.
Here is an example in Java, demonstrating how to set up a TrustManager to handle certificate validation:
import javax.net.ssl.X509TrustManager;
import java.security.cert.X509Certificate;
public class MyTrustManager implements X509TrustManager {
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType) {
// Implementation for client certificate validation
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType) {
// Implementation for server certificate validation
}
@Override
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0]; // Return an empty array
}
}
In the code above, you would implement the logic to validate certificates based on your specific requirements. This might include checking the issuer, expiration date, and purpose.
2. Configuration Misalignments
Misconfiguration on either the client or the server side can lead to failed connections.
Why It Happens
Common pitfalls include:
- Different cipher suites being used
- Incorrect keystore/truststore paths
- Misconfigured TLS versions
Solution
Start by ensuring that both sides use compatible configurations. Here's an example of how to set up SSLContext in Java:
import javax.net.ssl.SSLContext;
public class SSLConfig {
public static SSLContext createSSLContext() throws Exception {
SSLContext sslContext = SSLContext.getInstance("TLS");
// Set up key and trust managers
sslContext.init(null, new MyTrustManager[]{new MyTrustManager()}, new java.security.SecureRandom());
return sslContext;
}
}
In this snippet, the MyTrustManager
ensures that we are only accepting trusted certificates. Adjust the cipher suites and protocols in your SSLContext as needed.
3. Performance Overheads
Mutual TLS adds extra overhead as certificate verification requires computing and network resources.
Why It Happens
The validation of certificates and the overhead of the handshake contribute to increased latency.
Solution
You can use various techniques to mitigate performance issues:
- Session Resumption: Utilize session caching to avoid the full handshake.
- Load Testing: Ensure you are adequately load testing your application to identify bottlenecks.
Here is a simplified example of session resumption with SSLSession in Java:
import javax.net.ssl.SSLSession;
public class SessionHandler {
public void resumeSession(SSLSession session) {
if (session.isValid()) {
System.out.println("Resuming existing session: " + session.getId());
} else {
System.out.println("Session has expired. A new handshake is required.");
}
}
}
4. Revocation Checking
Certificate revocation can also be a big concern. If a certificate is revoked, clients and servers should not accept it.
Why It Happens
Not checking certificate revocation lists (CRLs) or relying solely on OCSP (Online Certificate Status Protocol) can expose vulnerabilities.
Solution
Implementing both OCSP and CRL checks can help maintain security compliance. In Java, you can add a socket factory to enforce revocation checking:
import javax.net.ssl.SSLSocket;
import javax.net.ssl.SSLSocketFactory;
public class RevocationChecker {
public SSLSocket createSocket(String host, int port) throws Exception {
SSLSocketFactory socketFactory = (SSLSocketFactory) SSLSocketFactory.getDefault();
SSLSocket socket = (SSLSocket) socketFactory.createSocket(host, port);
// Enable revocation checks (this method is illustrative; check your requirements)
socket.setEnabledCipherSuites(new String[] {"TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA256"});
return socket;
}
}
5. Debugging Transport Layer Issues
Debugging mTLS issues can be particularly challenging due to the complexities involved.
Why It Happens
When things go wrong, it can be hard to pinpoint whether the issue lies within the network, the certificates, or the application layers.
Solution
Utilize Java's built-in debugging capabilities by enabling SSL logging. Here's how:
-Djavax.net.debug=ssl:handshake:verbose
This command will output detailed logs about the TLS handshake process, allowing you to spot where failures occur.
To Wrap Things Up
Implementing Mutual TLS authentication can provide robust security for your applications, but it is not without its challenges. By being aware of common issues—including certificate validation, configuration misalignments, performance overheads, revocation checking, and debugging procedures—you can navigate these hurdles with confidence.
For additional resources, consider exploring:
- Oracle's Guide to Secure Sockets
- Mozilla's TLS Configuration Guide
- OWASP's Mutually Authenticated TLS
In your quest for enhanced security through mTLS, remember that understanding the problems and having clear solutions can significantly improve your implementation success rate. Happy coding!
Checkout our other articles