Struggling with Rate Limiting? Master Google Guava Today!
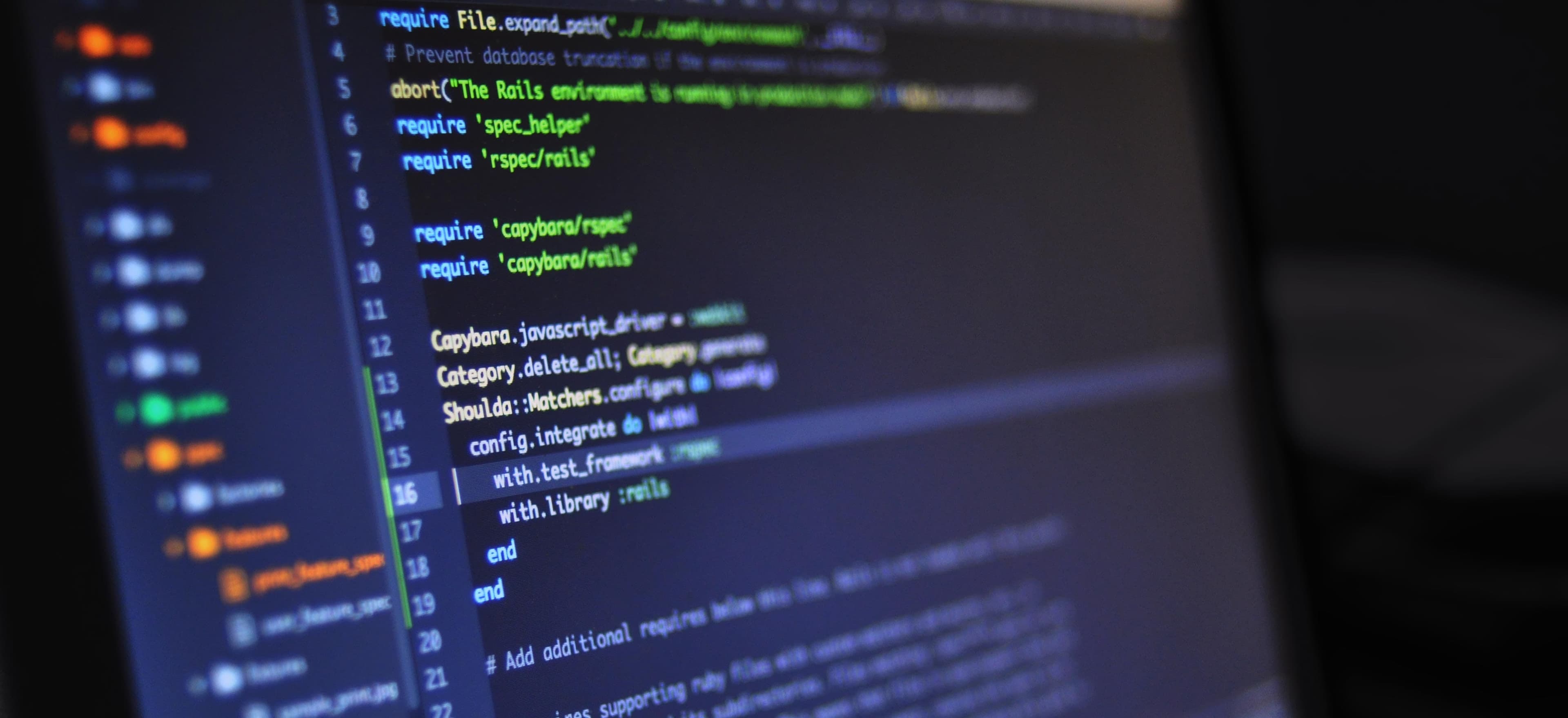
- Published on
Struggling with Rate Limiting? Master Google Guava Today!
In the realm of software development, one topic that often surfaces, especially when dealing with APIs, is rate limiting. Rate limiting is crucial for protecting your services from being overwhelmed by too many requests in a short span of time. This safeguards both server health and user experience. If you're working with Java, the Google Guava library has some excellent tools to simplify this process. In this post, we will dive deep into how to effectively manage rate limiting using Guava in a straightforward and efficient manner.
What is Rate Limiting?
Rate limiting is a technique used to control the amount of incoming or outgoing requests to or from a network. By imposing restrictions, developers can:
- Prevent Abuse: Limiting the number of requests protects against denial-of-service (DoS) attacks.
- Ensure Fair Usage: Allows for equitable distribution of resources among users.
- Maintain Performance: Helps to smooth out load spikes and maintain consistent service performance.
For an in-depth understanding of rate limiting, check out this detailed explanation on rate limiting by Cloudflare.
What is Google Guava?
Google Guava is an open-source set of core libraries for Java, developed by Google. It provides a range of utilities that simplify tasks such as:
- Collections manipulation
- Hashing
- Caching
- String processing
- Concurrency, including rate limiting
Guava's rate limiter provides a simple way to control the rate of actions, like API requests or resource usage. Let’s explore how to utilize it effectively.
Getting Started with Guava's RateLimiter
The first step to implement rate limiting with Guava is adding the Guava dependency to your project. If you're using Maven, include the following in your pom.xml
:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>32.1.0-jre</version>
</dependency>
Initializing RateLimiter
Once the dependency is included, creating a RateLimiter is straightforward. The primary method for instantiation is create(double permitsPerSecond)
, where permitsPerSecond
indicates how many actions (or requests) your application can handle in a second.
Example Code Snippet:
Here's a snippet demonstrating how to create and utilize a RateLimiter:
import com.google.common.util.concurrent.RateLimiter;
public class RateLimiterExample {
public static void main(String[] args) {
// Create a RateLimiter allowing 2 requests per second
RateLimiter rateLimiter = RateLimiter.create(2.0);
for (int i = 0; i < 10; i++) {
// Acquire a permit before proceeding
rateLimiter.acquire(); // Blocks if necessary to limit the rate
// Simulate processing a request
System.out.println("Processing request " + (i + 1));
}
}
}
Commenting on the Code:
-
RateLimiter Creation: By setting
permitsPerSecond
to2.0
, we are defining that the application can handle 2 requests per second. -
Acquiring Permits: The
acquire()
method is called before processing each request. If the limit is reached, it will wait (or block) until a permit is available, ensuring that the rate limit is respected. -
Request Simulation: The loop simulates handling requests. When you run this code, you'll notice that requests are spaced out to maintain a maximum limit of two per second.
Customizing Rate Limiting Behavior
Guava’s RateLimiter can be customized based on specific requirements. For instance, one can employ a burst strategy, allowing short bursts of requests that exceed the rate limit.
To enable burst traffic, create a RateLimiter with a maximum burst of permits:
RateLimiter rateLimiterWithBurst = RateLimiter.create(5.0, 1, TimeUnit.SECONDS);
In this example, it permits a burst of up to 5 requests and refills permits at a rate of one per second thereafter. This allows for temporary spikes in request handling.
Using RateLimiter in Asynchronous Environments
When dealing with asynchronous tasks, integrating the RateLimiter into your workflow remains essential. Here's how to apply RateLimiter with the ExecutorService:
import com.google.common.util.concurrent.RateLimiter;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class AsyncRateLimiterExample {
public static void main(String[] args) {
RateLimiter rateLimiter = RateLimiter.create(1.0); // 1 request per second
ExecutorService executor = Executors.newFixedThreadPool(5); // 5 concurrent threads
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
rateLimiter.acquire(); // Ensure a permit before processing
System.out.println("Handled request by: " + Thread.currentThread().getName());
});
}
executor.shutdown();
}
}
Commentary on Asynchronous Implementation:
-
ExecutorService: Here we create a thread pool of size 5. Multiple requests can be handled simultaneously.
-
Permit Acquisition: Before processing, each thread acquires a permit. As the rate limit is respected, this ensures none of the threads exceed the defined rate.
-
Thread Safety: The RateLimiter is thread-safe out-of-the-box, making it suitable for concurrent environments.
Monitoring and Logging
While implementing rate limiting, monitoring the behavior of your RateLimiter is crucial. Consider utilizing logging frameworks like SLF4J or Log4j to track API usage patterns. This information can help refine your rate limiting strategies and adjust them based on real-world traffic patterns.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
// Inside your main class
private static final Logger logger = LoggerFactory.getLogger(RateLimiterExample.class);
for (int i = 0; i < 10; i++) {
rateLimiter.acquire();
logger.info("Processing request {}", (i + 1));
}
In Conclusion, Here is What Matters
Implementing rate limiting is a critical step in ensuring the reliability and stability of your application. Google Guava's RateLimiter makes this task straightforward and efficient. With features supporting various configurations, you can customize how your application responds to high load situations.
In this blog post, we explored the fundamentals of rate limiting, introduced Google Guava, and illustrated how to implement a basic and an asynchronous rate limiting solution. These tools will help you maintain better control over request handling in your applications. Embrace these techniques, and rest easy knowing that your service is adequately protected against unnecessary strain.
If you're interested in diving deeper into concurrency in Java, consider looking into Java's CompletableFuture for a more advanced approach to managing asynchronous tasks.
With this foundation, you should be well-equipped to handle rate limiting effectively. Happy coding!
Checkout our other articles