Resolving NoSuchMethodError in Hadoop HDFS Integration
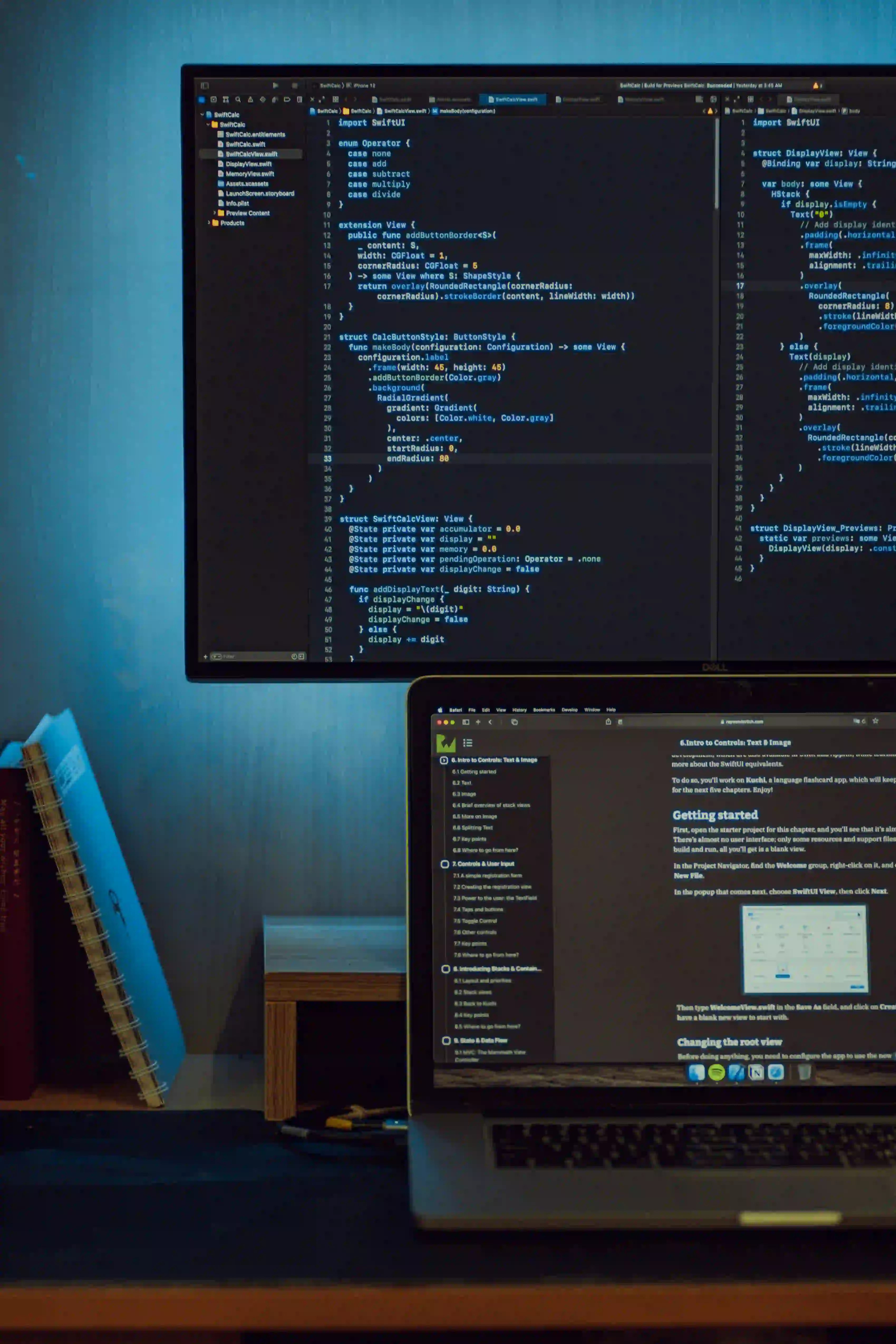
Resolving NoSuchMethodError in Hadoop HDFS Integration
Integrating Hadoop's HDFS (Hadoop Distributed File System) with Java applications can be a robust and scalable solution for managing large datasets. However, developers may encounter various exceptions during integration. One such frequent issue is NoSuchMethodError
. This blog post will provide a comprehensive guide on resolving this error within the context of Hadoop HDFS integration.
Understanding NoSuchMethodError
Before delving into solutions, it is crucial to understand what a NoSuchMethodError
is. This error occurs when the Java Virtual Machine (JVM) tries to call a method that doesn't exist. This can happen for several reasons:
- The method in the class has been renamed or removed.
- The class version loaded at runtime differs from the one during compile time.
- Incompatible libraries are present on the classpath.
In the context of Hadoop, incorrect library versions or missing dependencies can be common culprits.
Why It Matters
When integrating HDFS with Java applications, running into a NoSuchMethodError
can lead to application crashes or unpredictable behavior. Therefore, resolving this issue is crucial for the stability and reliability of your data processing tasks.
Common Causes of NoSuchMethodError in Hadoop HDFS Integration
-
Library Version Mismatch: Perhaps the most common issue, where the version of Hadoop libraries used during development is different from those available at runtime.
-
Compiling against Incorrect Dependencies: If you compile your application against a local version of a library that differs from the one used at runtime in the Hadoop environment, it can lead to
NoSuchMethodError
. -
Missing Dependencies: Sometimes required dependencies are not included in the classpath, leading to method calls failing.
Letβs look at how you can troubleshoot these causes effectively.
Step-by-Step Resolution Guide
Step 1: Check Your Hadoop Dependencies
The first step in resolving NoSuchMethodError
is to inspect your project's dependencies. If you're using Apache Maven or Gradle, it's easy to verify which versions of Hadoop libraries are being used.
Maven Example:
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-common</artifactId>
<version>3.3.0</version> <!-- Version number should match your Hadoop installation -->
</dependency>
Make sure to update your pom.xml to match the Hadoop version in your cluster.
Step 2: Importing Compatibility Libraries
If you're using libraries like hadoop-client
, ensure you import compatible versions. Use the following dependencies:
Maven Example:
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-client</artifactId>
<version>3.3.0</version>
</dependency>
Using an incorrect version can lead to a NoSuchMethodError
. Always cross-reference compatibility here.
Step 3: Inspect Your Classpath
Run your application with a verbose option to check the classpath. Look for duplicates or stray versions of the libraries that could lead to conflicts. Use the command:
java -cp your-application-*.jar:$(hadoop classpath) com.example.YourMainClass
Step 4: Confirm Version Listings
Inspect the library versions loaded in your Hadoop environment with:
hadoop version
The output will confirm the Hadoop version running. If there's a discrepancy with your local development version, correct the dependency versions in your build file.
Step 5: Clean and Rebuild Your Project
After making any dependency changes, perform a clean build of your project. For Maven:
mvn clean install
For Gradle:
./gradlew clean build
Step 6: Reset Your Environment
- Ensure that your IDE does not cache any old library versions.
- Clear and reset your environment settings.
- Make sure that the Git repository configurations do not point to old versions.
Example Code Snippet
Here is an example to demonstrate reading from HDFS, which may trigger the NoSuchMethodError
if dependencies are not properly resolved. Ensure that you include the correct versions as discussed.
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class HdfsReader {
public static void main(String[] args) throws Exception {
// Set up the configuration
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
// Path for the HDFS file you want to read
Path path = new Path("/user/hadoop/input/sample.txt");
// Read the file
try (BufferedReader br = new BufferedReader(new InputStreamReader(fs.open(path)))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
}
}
}
Commentary on Code
- Imports: Ensure that all classes from Hadoop are compatible versions.
- Configuration: Always set up your Hadoop configuration properly. If you miss a property, methods intended for your class may not be present.
- FileSystem: Using
FileSystem.get(conf)
is standard for accessing files in HDFS. Ensure that your configuration is consistent with the version of Hadoop you are using.
Additional Resources
For more in-depth information, the Hadoop Documentation is an excellent place to start. The guidelines are usually version-specific, so make sure to look for the relevant information based on the version you are using.
Final Considerations
Resolving a NoSuchMethodError
in Hadoop HDFS integration demands a systematic approach. By carefully inspecting dependencies, ensuring compatibility, and maintaining consistent versions across your development and runtime environments, you can minimize such errors. Following these steps will not only help address the immediate concern but will also lay down a strong foundation for future Hadoop development.
Remember, the key to avoiding integration issues lies in maintaining updated documentation and a vigilant eye on dependencies. Happy coding!