Optimizing Drools: Managing FireAllRules and Timers Efficiently
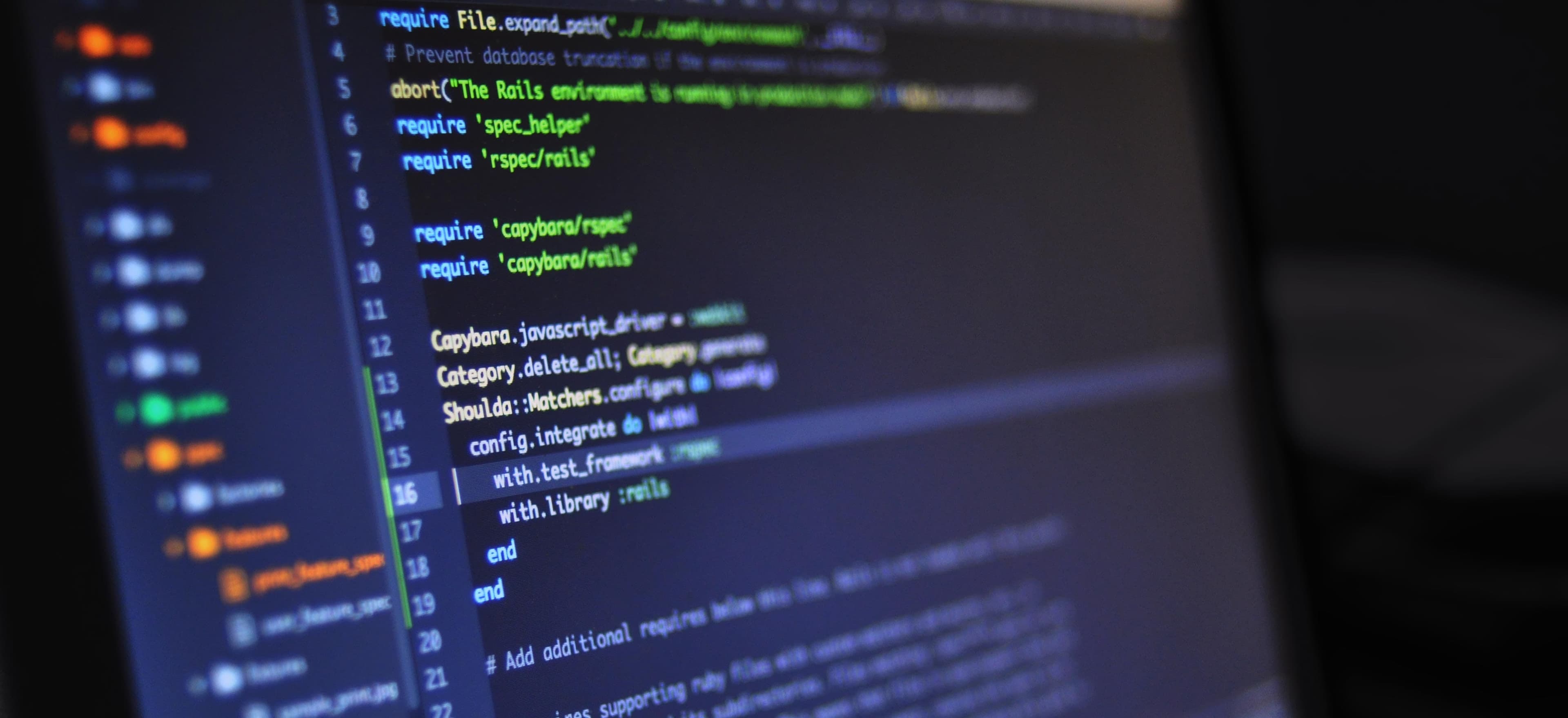
- Published on
Optimizing Drools: Managing FireAllRules and Timers Efficiently
When it comes to Business Rule Management Systems (BRMS), Drools stands out as a powerful tool for implementing complex event processing and rule-based logic. However, as systems grow in complexity, so too does the necessity for optimizing rule execution, especially with functions like fireAllRules()
and the effective use of timers. In this blog post, we'll delve into strategies for optimizing Drools, focusing on fireAllRules()
and timers, ensuring better performance and resource management.
Understanding Drools and the Role of fireAllRules()
Drools uses a rule engine to execute business logic defined in rules files. A typical operation involves calling fireAllRules()
, a method that triggers all the rules that are applicable to the current set of facts in the working memory.
Why Use fireAllRules()?
The fireAllRules()
method is essential for evaluating rules. However, calling it indiscriminately and frequently can lead to performance pitfalls. So, why is this method so crucial?
- Rule Enactment: It processes all active rules against the current data state.
- State Management: It allows the system to maintain the current state of business logic execution.
Optimizing fireAllRules() Execution
Here are a few strategies to optimize the use of fireAllRules()
:
1. Batching Facts
Instead of firing rules for a single fact, batch your facts together and fire rules once. This reduces overhead significantly.
KieSession kieSession = ...; // Presuming we have a KieSession
List<MyFact> facts = new ArrayList<>();
// Populate your facts
facts.add(new MyFact(...));
facts.add(new MyFact(...));
// Insert facts in batch
for (MyFact fact : facts) {
kieSession.insert(fact);
}
// Fire rules once for all facts
kieSession.fireAllRules();
Why Batching? Batching reduces the number of calls to fireAllRules()
, therefore lowering the overall system load and improving performance.
2. Using Agenda Groups
Drools provides a mechanism to isolate sets of rules called Agenda Groups. By using agenda groups, you can control which rules should be executed based on conditions.
kieSession.getAgenda().getAgendaGroup("group1").setFocus();
kieSession.fireAllRules();
Why Use Agenda Groups? This allows for better organization and prioritization of rule execution, minimizing unnecessary evaluations and focusing only on relevant rules.
3. Rule Prioritization
Adjust rules' priorities so that the most crucial rules execute first and irrelevant rules are minimized in order to optimize throughput.
rule "Important Rule"
salience 100 // highest priority
when
// conditions
then
// actions
Why Prioritize Rules? It ensures that critical business logic has precedence in being executed, which can significantly impact performance in high-traffic applications.
Efficient Timer Management in Drools
Timers in Drools allow for executing rules at specific intervals or after a defined delay. They are particularly useful for scenarios requiring periodic checks or delayed actions.
Using Timers Effectively
- Configuring Timers Appropriately
You can set up timers in your rules to define when they should be fired.
rule "My Timer Rule"
timer (cron: 0/10 * * * * ?) // Every 10 seconds
when
// conditions
then
// actions
end
Why Configure Timers? It allows you to manage timing-based conditions effectively without constant polling, thus saving resources.
- Minimize Timer Conflict
In complex systems, multiple timers may trigger concurrently, leading to performance issues.
rule "Conflict Rule"
timer (cron: 0/5 * * * * ?) // Every 5 seconds
when
exists (ConflictingFact())
then
// actions
end
Why Minimize Conflict? Preventing concurrent execution of conflicting rules ensures that system performance remains optimal, and outcomes are predictable.
Best Practices for Timer Usage
- Use the right timer types: Cron timers are useful but can be overkill for simple timing mechanisms. Consider alternatives like simple duration timers when applicable.
- Set timeout conditions: Implement conditions that stop timers when they are no longer necessary, further freeing resources.
rule "Cleanup Timer Rule"
timer (duration: 10000) // 10 seconds
when
// conditions
then
// actions
if (cleanUpRequired()) {
// cleanup logic
} else {
// cancel the timer
// Note: You should ideally remove the rule or replace it with a no-op.
}
end
Performance Monitoring
Once you have implemented the optimizations, it is crucial to monitor the performance continuously. Drools generates detailed logs that include session performance metrics. Analyzing these logs will provide insights into rule execution times and areas for further optimization.
Key Metrics to Monitor
- Execution Time: How long does
fireAllRules()
take? - Rule Activation Rates: Which rules are frequently activated?
- Memory Usage: How much memory is being utilized by the KieSession?
Closing Remarks
Effectively optimizing the use of fireAllRules()
and timers in Drools can lead to significant performance improvements in rule processing. By batching facts, utilizing agenda groups, setting priority on rules, and managing timers effectively, you can ensure that your Drools setup runs smoothly and efficiently.
For more about Drools and best practices, check out the Drools Documentation. And remember, continual monitoring and refinements are essential for maintaining performance as business logic grows and changes.
With these best practices at your disposal, you are well on your way to mastering Drools optimization. Happy coding!