Decoding Stack Traces: Common Mistakes to Avoid
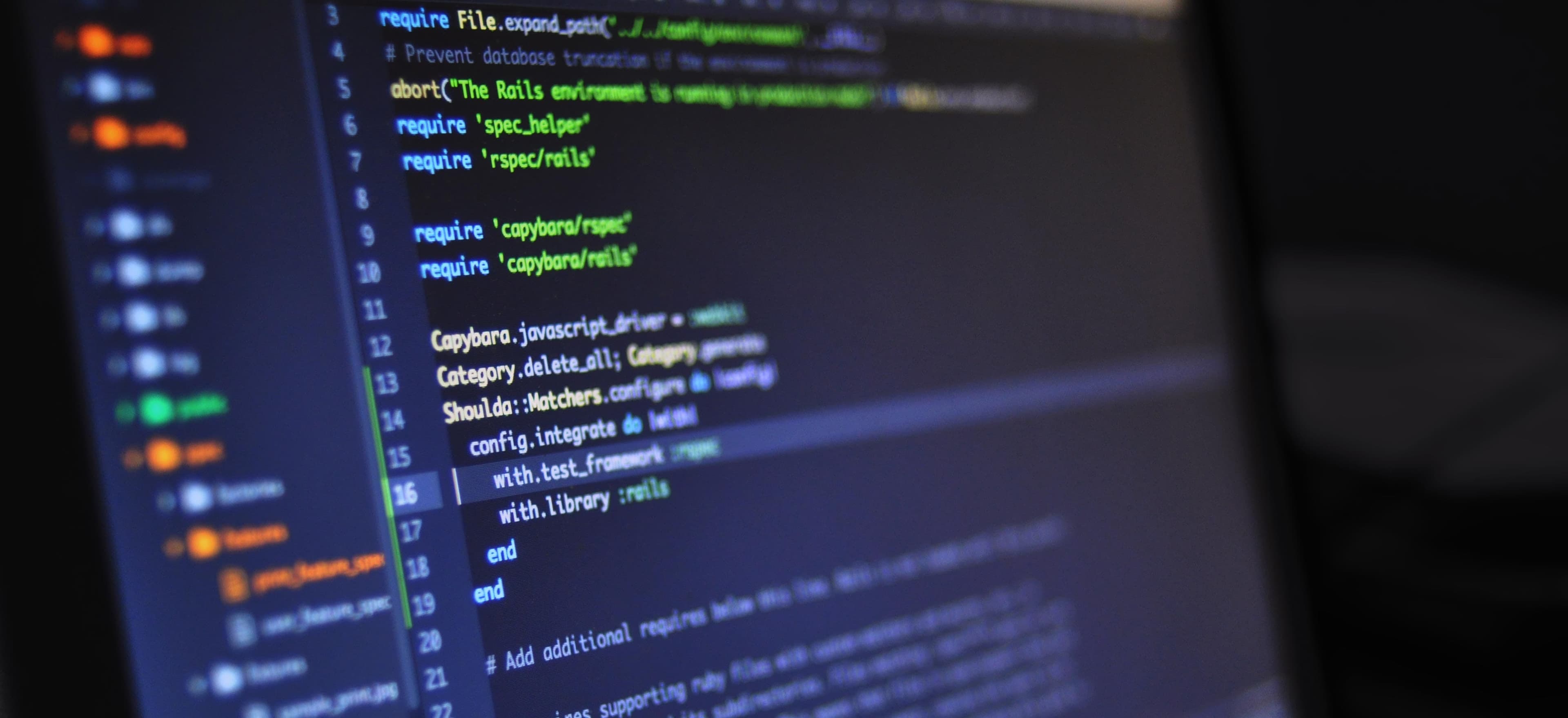
- Published on
Decoding Stack Traces: Common Mistakes to Avoid
Debugging is an essential skill in software development. Among the various tools at our disposal, stack traces stand as one of the most powerful means for diagnosing issues. A well-crafted stack trace allows developers to understand the flow of execution leading up to an error, making it easier to pinpoint where things went wrong. However, there are common pitfalls that can lead to misunderstanding or misinterpreting these trace logs. In this blog post, we will decode the intricacies of stack traces, highlight frequent mistakes, and offer best practices for effective debugging.
Understanding Stack Traces
Before we dive into common mistakes, let's start by understanding what a stack trace is. A stack trace is essentially a report containing information about the program’s state at a specific point in time, particularly when an exception occurs. It includes:
- The sequence of method calls leading up to the exception.
- The exact line numbers where the exceptions occurred.
- A description of the error itself.
While stack traces can seem intimidating at first glance, breaking them down into simpler components is key to effective debugging.
Example of a Stack Trace
Here’s a simple example of a stack trace you might encounter in a Java application:
Exception in thread "main" java.lang.NullPointerException
at com.example.myapp.MainClass.processData(MainClass.java:23)
at com.example.myapp.MainClass.main(MainClass.java:10)
In this example, the first line identifies the type of exception thrown (NullPointerException
), while the subsequent lines list the method calls leading to it. The format typically includes:
- Exception type: In this case, it's a NullPointerException.
- Call stack: Each entry indicates the class, method, and line number where the error is encountered.
This concise structure encapsulates powerful information for the developer.
Common Mistakes in Decoding Stack Traces
1. Ignoring the Root Cause
One of the primary mistakes developers make is focusing on the last line of the stack trace without considering the cause of the exception. The last method call might not represent the true source of the problem.
Why This Matters
By investigating earlier calls in the stack, you might discover that the cause of an issue stems from incorrect input, external service failures, or other conditions that led to the error.
Example Code Snippet
Consider this simple Java method that might throw an exception:
public void processData(List<String> data) {
String firstItem = data.get(0); // This might throw an IndexOutOfBoundsException
System.out.println(firstItem.length());
}
If the data
list is empty, an IndexOutOfBoundsException
will occur. The stack trace will likely show this line as the source, but neglecting to check earlier code that populates the list would be a mistake.
2. Misinterpreting Exception Types
Java exceptions are categorized into checked and unchecked exceptions. Some developers may misinterpret an unchecked RuntimeException
as something that should be expected and ignored.
Clarification on Exception Handling
Unchecked exceptions usually indicate a programming error, such as a logical flaw in the code, whereas checked exceptions often represent external conditions that require handling, like I/O interruptions.
Example Code Snippet
Here's an illustration:
public void readFile(String filename) {
try {
BufferedReader reader = new BufferedReader(new FileReader(filename));
// Potential IOExceptions here
} catch (IOException e) {
e.printStackTrace(); // Good practice to handle
}
}
The IOException is a checked exception and should be logged or handled appropriately. Ignoring it could lead to further complications in your code.
3. Overlooking Thread Issues
In multi-threaded applications, stack traces can originate from various threads and lead to confusion about the source of an issue.
Why This is Critical
A stack trace coming from a background thread may not always indicate where the error originated in the main thread. Understanding which thread is active during exceptions is crucial.
Example Code Snippet
public class ThreadDemo {
public static void main(String[] args) {
new Thread(() -> {
throw new RuntimeException("Thread error");
}).start();
}
}
Here, if the RuntimeException
occurs, the stack trace will belong to a different thread, which could mislead the debugger if unaware of the threading model.
4. Failing to Keep Context
In large applications, developers may not have a complete context of the business logic behind the code. Consequently, they might misinterpret the consequences of certain method calls within the stack trace.
Importance of Context
Being aware of the application’s architecture is necessary for tracing problems accurately. Without context, it’s easy to jump to conclusions that may not apply to the actual case.
Example Code Snippet
Imagine this scenario within a fictional banking application:
public void transferFunds(Account from, Account to, double amount) {
if (from.getBalance() < amount) {
throw new InsufficientFundsException("Not enough funds"); // Stack trace will show this
}
// Code to transfer funds...
}
The InsufficientFundsException
can indicate a simple business logic violation, and ignoring relevant context regarding Account
operations could lead to further confusion.
5. Not Utilizing Logging Frameworks
Some developers omit using structured logging, which can exacerbate stack trace readability. Structured logs can add context, making stack traces much easier to navigate.
The Benefit of Structured Logging
By implementing logging frameworks like SLF4J or Log4j, you can tag logs with identifiers that correlate with user requests or state, thus enriching the information provided alongside stack traces.
Example Code Snippet
Using SLF4J for better structured logs might look like this:
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public void process(String userId) {
logger.info("Processing request for user: {}", userId);
// Code that might throw exceptions...
}
When an exception occurs, the logs give context about who was involved and the task at hand, making the stack trace invaluable.
Best Practices for Decoding Stack Traces
To effectively decode stack traces and avoid common mistakes, follow these best practices:
- Examine the entire stack trace: Always look through all method calls leading to the exception.
- Understand exception types: Differentiate between checked and unchecked exceptions.
- Monitor thread contexts: Consider multi-threading implications when analyzing stack traces.
- Utilize logging frameworks: Take advantage of structured logging to add searchable context to your traces.
- Keep application context in mind: Follow the business logic and understand how your project interacts with its components.
Final Considerations
Decoding stack traces effectively is crucial for minimizing debugging time and maximizing productivity as a Java developer. By avoiding common mistakes and complying with best practices, you can enhance your debugging skills and streamline your development process. If you're looking to improve your understanding further, check out The Art of Unit Testing for insights into robust testing strategies that can help you prevent issues before they require a deep dive into stack traces.
Happy debugging!