Avoiding Common Red Flags in Java Development Projects
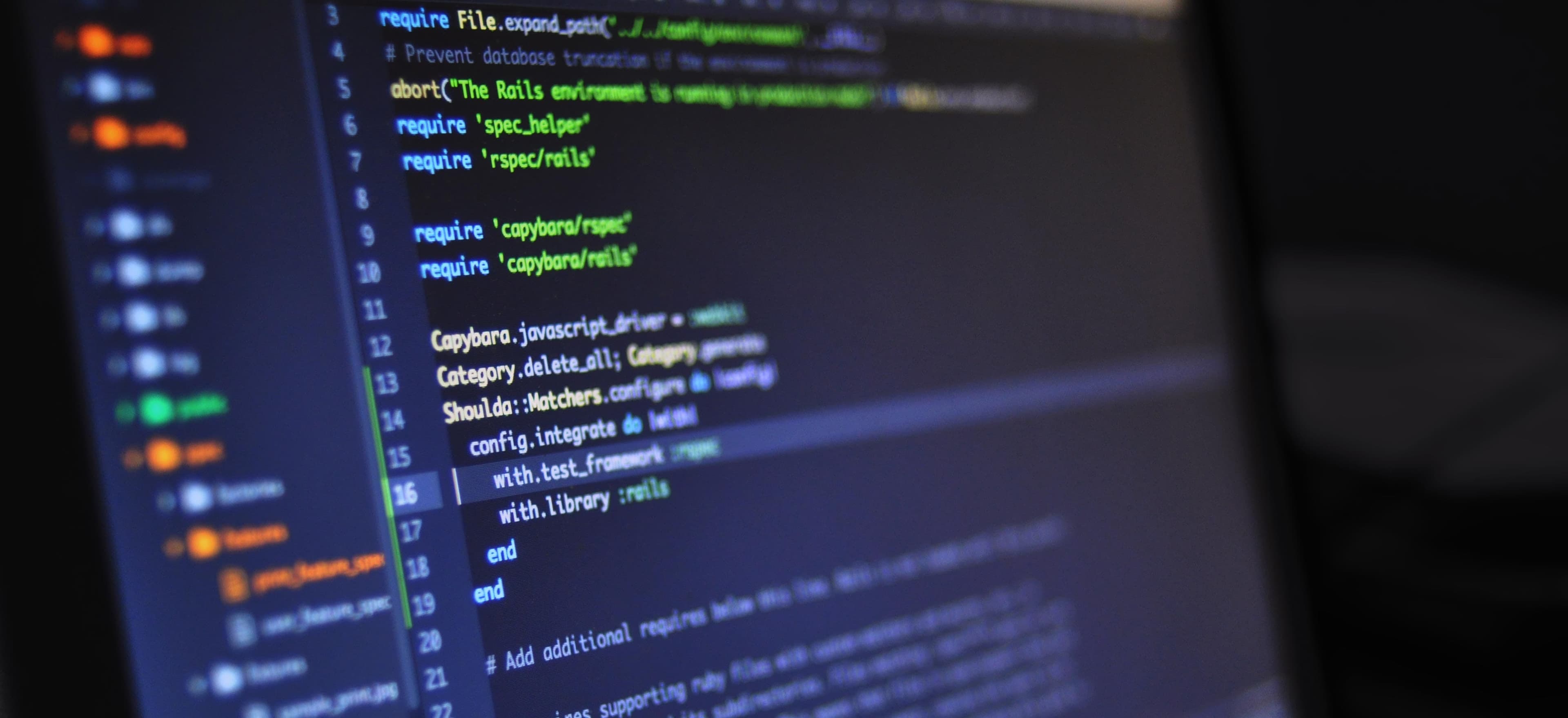
- Published on
Avoiding Common Red Flags in Java Development Projects
Java has long been a cornerstone in the realm of software development. Its versatility, object-oriented nature, and large ecosystem make it a go-to language for various applications. However, along the journey of a Java development project, developers often encounter red flags that can jeopardize the project’s success. In this blog post, we will explore some common pitfalls in Java development and provide you with strategies to sidestep these issues.
1. Lack of Clear Requirements
Before initiating any Java development project, it is paramount to have a well-defined set of requirements. Ambiguous or unclear requirements lead to misunderstandings, scope creep, and ultimately, project failure.
Why It's Important
Without clear requirements, your project may deviate from its intended goals, leading to additional costs and delays.
Solution
Conduct thorough stakeholder meetings to gather and document requirements. Agile methodologies can aid in progressing through iterations, allowing for adjustments based on ongoing feedback.
public class UserRequirements {
private String role;
private String feature;
public UserRequirements(String role, String feature) {
this.role = role;
this.feature = feature;
}
@Override
public String toString() {
return "UserRequirements{" +
"role='" + role + '\'' +
", feature='" + feature + '\'' +
'}';
}
}
In this example, capturing user roles and their required features instills specificity and clarity into your project.
2. Neglecting Code Quality
In the world of Java development, adhering to coding standards is crucial. Neglecting code quality can lead to issues like maintainability, readability, and performance degradation.
Why It's Important
Poorly structured code can hinder other developers from understanding and collaborating efficiently, which can cause delays in development.
Solution
Incorporate coding standards such as Google Java Style Guide and employ code review practices. Utilize tools such as PMD or SonarQube to identify bad practices.
public class OrderService {
public void processOrder(Order order) {
if (order == null) {
throw new IllegalArgumentException("Order cannot be null");
}
// processing logic
}
}
The use of exceptions to handle null inputs promotes cleaner and more maintainable code.
3. Ignoring Proper Exception Handling
Java’s robust exception handling mechanism is designed to manage error situations effectively. Ignoring exception handling can lead to application crashes, data loss, or unexpected behavior.
Why It's Important
Proper exception management can help maintain application stability and deliver a reliable user experience.
Solution
Always use try-catch blocks wisely and ensure to log exceptions with enough detail for debugging.
public void readFile(String filePath) {
try {
// Code to read file
} catch (IOException e) {
System.err.println("Error reading file: " + filePath + " " + e.getMessage());
}
}
The logging of exceptions not only informs you of problems but also aids in maintaining consistency, which users appreciate.
4. Overlooking Unit Testing
Unit testing is essential for maintaining code quality and ensuring that each component of your Java application works as intended. A lack of tests can lead to high defect rates.
Why It's Important
Tests help catch bugs early, saving time and resources in the long run.
Solution
Adopt Test-Driven Development (TDD) or write unit tests after developing your features. Use frameworks like JUnit or TestNG to improve your test coverage.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3));
}
}
In this code snippet, unit tests confirm that the add
method of the Calculator
class behaves as specified. TDD fosters confidence in code correctness.
5. Ignoring Performance Optimization
Performance issues can manifest as the project scales, affecting user satisfaction. Often neglected during the initial stages of development, performance optimization can be a serious red flag.
Why It's Important
Users will abandon applications that are slow or unresponsive, directly impacting business success.
Solution
Profile your application using tools like VisualVM to identify bottlenecks. Adopt best practices like using efficient data structures and algorithms.
public void optimizeDataProcessing(List<Data> dataList) {
// Using a HashMap for efficient lookups
Map<String, Data> dataMap = new HashMap<>();
for (Data data : dataList) {
dataMap.put(data.getId(), data);
}
// Further processing using dataMap
}
By utilizing a HashMap, data lookups become faster, illustrating a fundamental principle of performance optimization.
6. Bypassing Version Control Systems
Version control systems (VCS) like Git play a pivotal role in maintaining the integrity of your codebase. Bypassing these tools can lead to version conflicts and loss of important historical changes.
Why It's Important
Without version control, tracking changes and collaborating with team members becomes chaotic.
Solution
Always use a version control system from day one. Utilize branches for new features, bug fixes, and testing to keep the main codebase stable.
# Example of branching in Git
git checkout -b feature/new-user-authentication
By managing different features in separate branches, you ensure that the main branch remains stable while still allowing innovation.
7. Forgetting Documentation
Documentation may seem trivial, but it is vital for project sustainability. A lack of documentation can alienate future developers or even your future self.
Why It's Important
Without proper documentation, understanding the codebase can take significantly longer for new developers.
Solution
Utilize Javadoc for documenting public APIs, methods, and classes. Additionally, keep a README file to explain project setup and architecture.
/**
* Calculates the sum of two integers.
* @param a first integer
* @param b second integer
* @return sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
Well-structured documentation provides clarity and facilitates easier onboarding for new team members.
Wrapping Up
Navigating the complexities of Java development requires vigilance against common pitfalls. By proactively addressing these red flags—clear requirements, quality code, robust exception handling, rigorous testing, performance optimization, version control, and documentation—you set the stage for a successful project.
Investing time in these areas not only saves you headaches down the line but also positions your project for long-term success. For further reading, consider exploring comprehensive resources such as "Effective Java" by Joshua Bloch and the Java SE 17 Documentation.
In this rapidly evolving tech landscape, staying ahead of issues ensures that your Java applications remain robust, maintainable, and user-friendly.
Happy coding!