Mastering Sorting in Spring Data Solr: Common Pitfalls
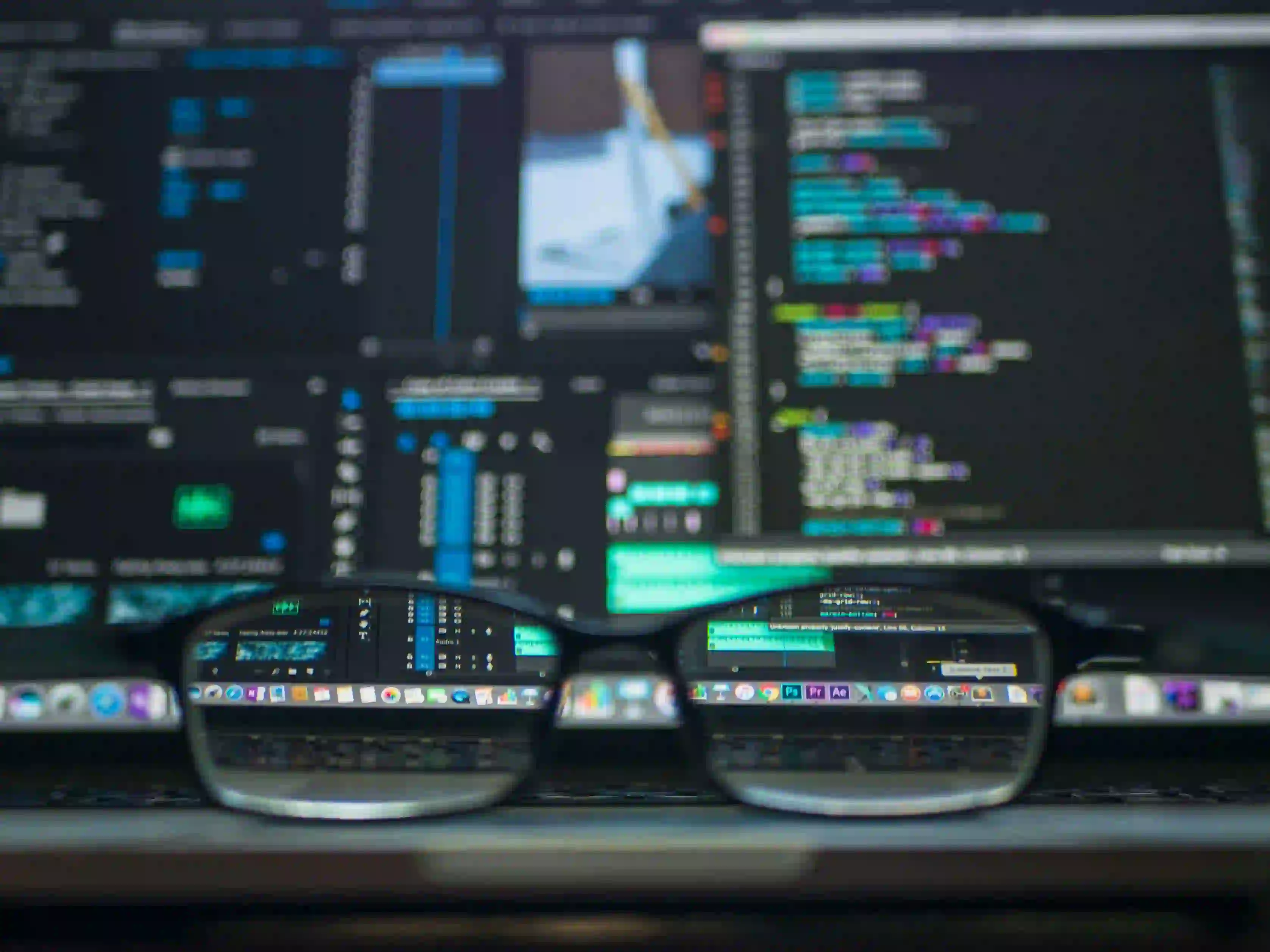
Mastering Sorting in Spring Data Solr: Common Pitfalls
Sorting is fundamental in any application that deals with collections of data. In the realm of search engines and database queries, sorting can enhance user experience dramatically by presenting data in a meaningful order. In the case of Spring Data Solr, a component of the Spring ecosystem that simplifies interaction with Apache Solr, sorting can seem straightforward but comes with its own set of challenges and pitfalls.
In this blog post, we will explore the common pitfalls encountered in sorting with Spring Data Solr, sharing best practices, and providing code examples to ensure you master sorting effectively.
Understanding Sorting in Spring Data Solr
Spring Data Solr provides a simplified way to interact with Solr's powerful search capabilities. Understanding how sorting works in this setup is essential to leverage its efficacy fully. Sorting is typically defined on the query level, and it is possible to sort by any field that is indexed in Solr.
Basic Sorting Syntax
Before diving into pitfalls, let’s start with a basic example. Suppose we have an entity called Product
that contains fields like name
, price
, rating
, and availability
. To sort products by price in ascending order, you might write:
import org.springframework.data.solr.core.query.Query;
import org.springframework.data.solr.core.query.SimpleSolrQuery;
import org.springframework.data.solr.core.query.Sort;
public List<Product> findAllSortedByPrice() {
Query query = new SimpleSolrQuery("*:*");
query.addSort(new Sort(Sort.Order.asc("price")));
return solrTemplate.queryForList("products", query, Product.class);
}
Why This Matters
The key here is that you can control how the data is presented to users, ensuring that they see the most relevant or affordable products first. However, there are common pitfalls in achieving reliable sorting.
Common Pitfalls When Sorting with Spring Data Solr
Let us examine some common pitfalls that developers encounter when implementing sorting in Spring Data Solr:
1. Not Understanding Field Types
One of the most common pitfalls is not being aware of the data types of the fields in Solr. Attempting to sort a numeric value stored as a string can lead to unexpected results.
Example and Explanation
If you have a field price
defined as a string in Solr and you try to sort numerically, the results will be incorrect. For instance:
query.addSort(new Sort(Sort.Order.asc("price"))); // price is a string
To avoid this, ensure that the price
field is defined as a float or an integer in the schema.
2. Incorrect Query Syntax
Improper query construction can also lead to failures in sorting. If the query contains unnecessary parameters or incorrect syntax, the sort may not work as expected.
Query query = new SimpleSolrQuery("name:productName"); // only querying by name
query.addSort(new Sort(Sort.Order.desc("price"))); // trying to sort by price
In the above code, if the name
does not match any documents, the resultant query will return zero documents. Always ensure your primary query encompasses the necessary data.
3. Pagination and Sorting Conflicts
When combining sorting with pagination, you might encounter challenges. Pagination can interfere when your primary query does not return a consistent dataset.
Maintaining Consistency with Sort and Pagination
Here's how you can handle this properly:
query.setOffset(0);
query.setRows(10);
query.addSort(new Sort(Sort.Order.asc("rating")));
In addition, ensure that the sort order is consistent with your pagination logic to avoid returning duplicate or missing records.
4. Performance Issues with Large Datasets
Sorting large datasets can lead to performance degradation. Solr efficiently handles queries, but there are practices to make sorting faster.
Using Cursors for Better Performance
When dealing with larger datasets, using cursors can significantly enhance performance:
SolrQuery solrQuery = new SolrQuery("*:*");
solrQuery.set("cursorMark", "*");
solrQuery.set("rows", 10);
solrQuery.addSort("price", SolrQuery.ORDER.asc);
5. Ignoring Faceting and Highlighting
When implementing sorting, it is critical to remember that faceting and highlighting can add complexity. If not properly managed, sorting can affect your ecosystem of search features.
Best Practices to Integrate Sort with Other Features
When combining these features, ensure you handle the output correctly. Use facets to provide additional context for the sorted data appropriately.
solrQuery.setFacet(true);
solrQuery.addFacetField("category");
6. Not Considering the Default Sort Order
If sorting is not explicitly defined in a query, Solr will revert to its default behavior, which may not be desirable. Always provide a definitive sort when necessary.
if (query.getSortFields().isEmpty()) {
query.addSort(new Sort(Sort.Order.asc("name"))); // fallback sort on name
}
Wrapping Up
Sorting in Spring Data Solr is an essential yet complex feature that, when mastered, can significantly enhance a search application. By understanding the pitfalls outlined above—such as field types, query syntax, pagination, performance, faceting, and default sorts—you can avoid common mistakes and ensure performance optimization.
Use sorting to provide value-driven user experiences. For further reading, delve into Spring Data Solr documentation and explore the Apache Solr documentation for more detailed use cases and advanced configurations.
Your path to mastering sorting in Spring Data Solr starts here—embrace the challenges and grow your skills!