Common Java Servlet Mistakes and How to Avoid Them
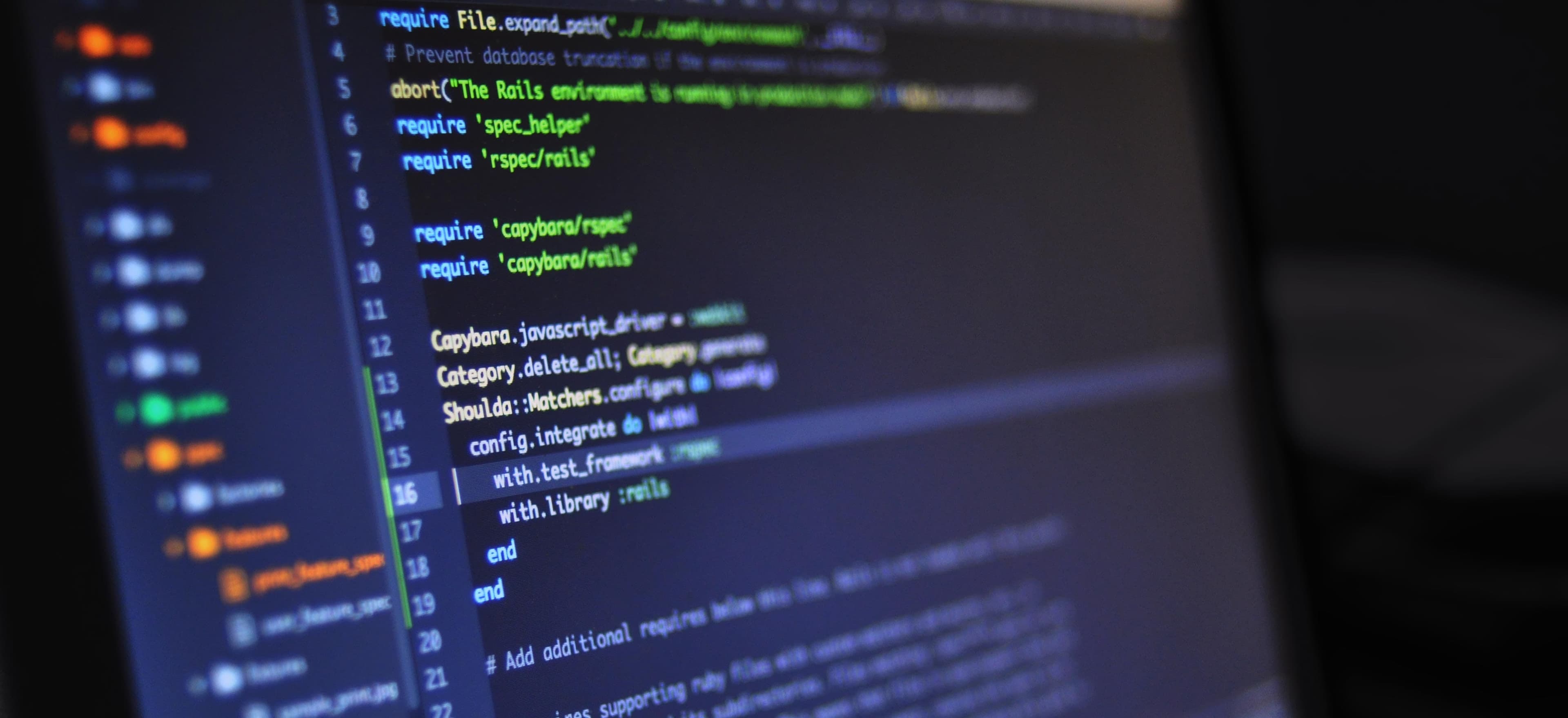
- Published on
Common Java Servlet Mistakes and How to Avoid Them
Java Servlets are powerful components of Java EE (Enterprise Edition) that enable developers to create dynamic web applications. While servlets offer great benefits, many pitfalls could trip developers up—especially those new to the servlet container. This post explores common mistakes in Java Servlet development and provides practical tips for avoiding them.
Table of Contents
- Understanding Java Servlets
- Typical Mistakes in Servlet Development
- Not Properly Managing the Lifecycle
- Ignoring Thread Safety
- Overusing Session Management
- Failure to Handle Exceptions
- Not Following the MVC Pattern
- Best Practices to Avoid Mistakes
- Conclusion
Understanding Java Servlets
Before we dive into common mistakes, let’s define what a servlet is. A Java Servlet is a Java program that runs on a server and extends the capabilities of a web server by allowing it to respond to requests from clients, usually through HTTP.
Servlets form the backbone of many web applications, allowing developers to handle complex business logic, manage user sessions, and serve dynamic content.
Typical Mistakes in Servlet Development
1. Not Properly Managing the Lifecycle
Java Servlets have a lifecycle defined by the servlet container, primarily consisting of three main methods: init()
, service()
, and destroy()
. A common mistake is neglecting to implement these methods correctly.
For example, failing to override the init()
method might lead to resource initialization problems.
public class MyServlet extends HttpServlet {
@Override
public void init() throws ServletException {
// Initialize resources here
super.init();
}
}
Why this matters: Proper lifecycle management ensures your servlet behaves correctly during its operational period, avoiding potential resource leaks or application crashes.
2. Ignoring Thread Safety
Servlets are shared among multiple users and utilize a multi-threaded environment. Not making your servlets thread-safe can lead to unexpected behavior and data corruption.
Consider the following code snippet:
public class CounterServlet extends HttpServlet {
private int counter = 0;
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
counter++;
response.getWriter().write("Counter: " + counter);
}
}
This code is not thread-safe. Multiple threads could modify the counter
value simultaneously.
Solution: Use synchronization.
@Override
protected synchronized void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
counter++;
response.getWriter().write("Counter: " + counter);
}
Why this matters: Using synchronized
ensures that only one thread can access the doGet
method at a time, maintaining data integrity.
3. Overusing Session Management
While session management can be beneficial for tracking user interactions over time, excessive reliance on it can lead to performance degradation and unnecessary memory usage.
Consider storing too much data in the session:
HttpSession session = request.getSession();
session.setAttribute("userDatabase", largeUserData);
Why this is problematic: If the session is kept alive for many users and has large data, it consumes significant memory, potentially leading to performance issues or out-of-memory errors.
Best Practice: Store only essential information in the session.
4. Failure to Handle Exceptions
Error handling is crucial in web applications. One common mistake is not properly managing exceptions, leading to ambiguous error messages or application failures.
For example:
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) {
try {
// Some processing
} catch (Exception e) {
// No logging or error handling
}
}
Why this matters: If an exception occurs without being caught, it can crash your servlet. Always log the errors.
private static final Logger logger = Logger.getLogger(MyServlet.class.getName());
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) {
try {
// Some processing
} catch (Exception e) {
logger.severe("Error occurred: " + e.getMessage());
response.sendError(HttpServletResponse.SC_INTERNAL_SERVER_ERROR, "Internal Server Error");
}
}
Solution: Combining logging with user-friendly error messages enriches the debugging process while working with users.
5. Not Following the MVC Pattern
The Model-View-Controller (MVC) design pattern is integral to most Java web applications, yet some developers neglect to implement it, resulting in tightly coupled code.
For instance, a servlet that both processes business logic and generates HTML:
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Business Logic
String result = performBusinessLogic();
// Directly generating HTML
response.getWriter().write("<html><body>" + result + "</body></html>");
}
Why this is a mistake: This approach makes testing difficult and reduces code reusability.
Solution: Split the responsibilities.
- Have a separate model for business logic.
- Use JSP or other templating systems for the view layer.
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String result = performBusinessLogic();
request.setAttribute("result", result);
RequestDispatcher dispatcher = request.getRequestDispatcher("result.jsp");
dispatcher.forward(request, response);
}
Best Practices to Avoid Mistakes
To ensure high-quality servlet development, consider these best practices:
-
Resource Management: Always release resources like database connections properly. Implement connection pooling where applicable.
-
Proper Configuration: Have a clear web.xml configuration, defining welcome files, error pages, and servlet mappings correctly.
-
Security Measures: Implement security checks (e.g., input validation, CSRF protection). This guide is a good starting point: OWASP Web Security Testing Guide.
-
Logging: Use logging libraries like SLF4J or Log4J. Consistent and informative logging can significantly ease troubleshooting.
-
Annotations over XML Configuration: Use servlet annotations (like
@WebServlet
) for cleaner code. -
Testing: Employ unit and integration testing frameworks like JUnit and Mockito to ensure your application's reliability.
Lessons Learned
Understanding and avoiding common Java Servlet mistakes is crucial for building robust, maintainable web applications. By managing the servlet lifecycle properly, ensuring thread safety, judiciously using session management, effectively handling exceptions, and adhering to MVC design principles, developers can create scalable and efficient applications.
Be mindful of these practices as you embark on your servlet development journey. With continuous learning and the integration of established best practices, you can enhance both your coding skills and the reliability of your applications.
For more information on Java Servlets, you can refer to the official documentation by Oracle.
By recognizing potential pitfalls and employing best practices, you're well on your way to becoming a proficient Java Servlet developer. Happy coding!
Checkout our other articles