Struggling with Value Annotations? Master Placeholders in Spring!
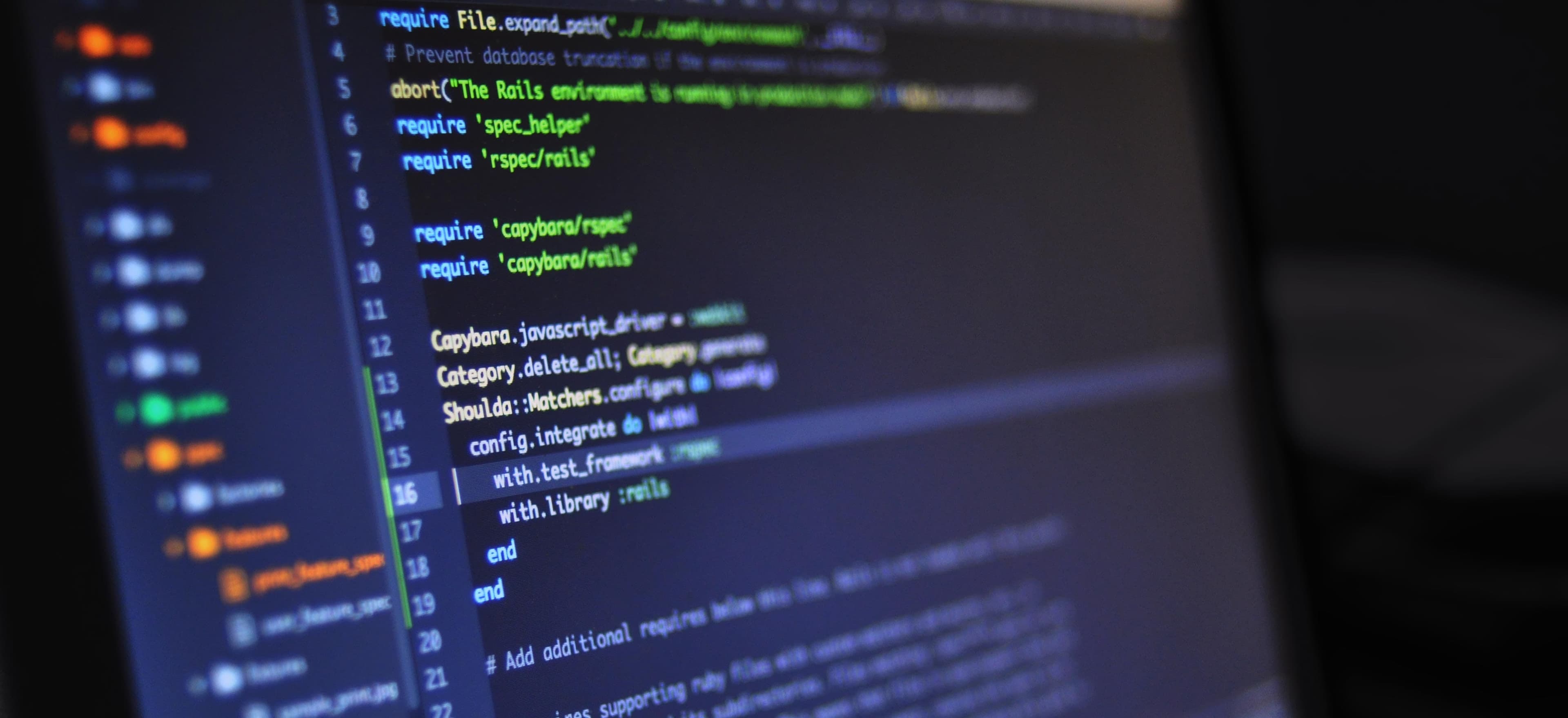
- Published on
Struggling with Value Annotations? Master Placeholders in Spring!
Spring Framework is a powerful tool in the world of Java development, facilitating the creation of robust applications. However, many developers encounter challenges when dealing with configuration values. One such way to handle configurations in Spring is through the use of @Value
annotations and placeholders. This article will demystify these concepts, making it easy for you to implement them in your own projects.
Understanding @Value
Annotations
In Spring, the @Value
annotation acts as a way to inject property values into your beans. This means that instead of hard-coding configuration settings directly into your application, you can externalize them into a properties file, making your application more flexible and easier to manage.
Why Use @Value
?
- Separation of Concerns: Keeping your configuration outside of your code promotes a clean design.
- Flexibility: Changing a configuration value doesn’t require redeploying or recompiling your application.
- Environment-Specific Configurations: You can have different properties files for different environments (e.g., development, testing, production).
Basic Example of Using @Value
To illustrate how to leverage the @Value
annotation, let's consider the following example.
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class MessagePrinter {
@Value("${app.message:Default Message}")
private String message;
public void printMessage() {
System.out.println(message);
}
}
In this code snippet, we inject the value of app.message
from a properties file. If the property is not set, it defaults to "Default Message". This is achieved using the syntax ${propertyKey:defaultValue}
.
Configuring Property Files
For the @Value
annotations to work seamlessly, you need a properties file.
- Create a file named
application.properties
in thesrc/main/resources
directory.
app.message=Hello, Spring!
- Spring will automatically load this file during the application startup, making the property available for injection.
Running the Application
You can now run your Spring Boot application. Upon invoking the printMessage()
method, the console will display:
Hello, Spring!
This emphasizes the strength of using @Value
— easy to manage and adaptable.
Placeholders Made Simple
Now, let's take a deeper dive into placeholders. Placeholders allow you to define and inject more complex values dynamically. This is particularly useful when dealing with multiple configurations.
Example of Using Placeholders
Suppose you want to define a host and a port for connecting to a database. Here’s how you can structure your properties:
db.host=localhost
db.port=5432
db.name=mydatabase
And then, your Spring component might look like this:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class DatabaseConfig {
@Value("${db.host}")
private String host;
@Value("${db.port}")
private int port;
@Value("${db.name}")
private String dbName;
public void printDatabaseConfig() {
System.out.println("Connecting to database:");
System.out.println("Host: " + host);
System.out.println("Port: " + port);
System.out.println("Database: " + dbName);
}
}
Why Are Placeholders Important?
Using placeholders:
- Improves Readability: Configuration values are easy to read and manage.
- Promotes Reusability: You can reuse these placeholders across multiple beans or services.
Profiles for Environment-Specific Configuration
Spring also supports different profiles. This means you can create specific properties files for different environments (e.g., Dev, Test, Prod).
Creating Profile-Specific Properties
- Create an application-dev.properties file for your development environment:
db.host=localhost
db.port=5432
db.name=mydatabase_dev
- Create an application-prod.properties file for your production environment:
db.host=prod-db-server
db.port=5432
db.name=mydatabase_prod
- Activate a profile by specifying it as a command-line argument or in your application’s properties.
To run the application with a specific profile:
java -jar yourapp.jar --spring.profiles.active=dev
This allows seamless management of different configurations without altering your code.
Best Practices when Using @Value
-
Default Values: Always provide a default value when possible, to avoid null values.
@Value("${app.timeout:3000}") private int timeout; // Default timeout is 3000 milliseconds
-
Type Safety: Ensure the type of the properties matches the field’s type. It prevents
ClassCastException
at runtime. -
Avoid Overuse: Too many
@Value
annotations can clutter your code. Consider using a@ConfigurationProperties
class if you have numerous related properties.
Further Reading on Spring Configuration
To deepen your understanding, you might want to explore Spring Boot Documentation on Externalized Configuration and Spring Profiles Documentation.
A Final Look
Mastering @Value
annotations and placeholders significantly enhances your ability to manage configurations in Spring applications. By externalizing configuration, you increase your application's maintainability, flexibility, and scalability.
Implement these best practices in your own projects, and you'll find that managing application settings becomes a breeze. Don't shy away from experimenting with properties and profiles, as they can be invaluable in creating clean and efficient Java applications.
Happy coding!
Checkout our other articles