Exploring the Reasons Behind Raw String Literals' Removal
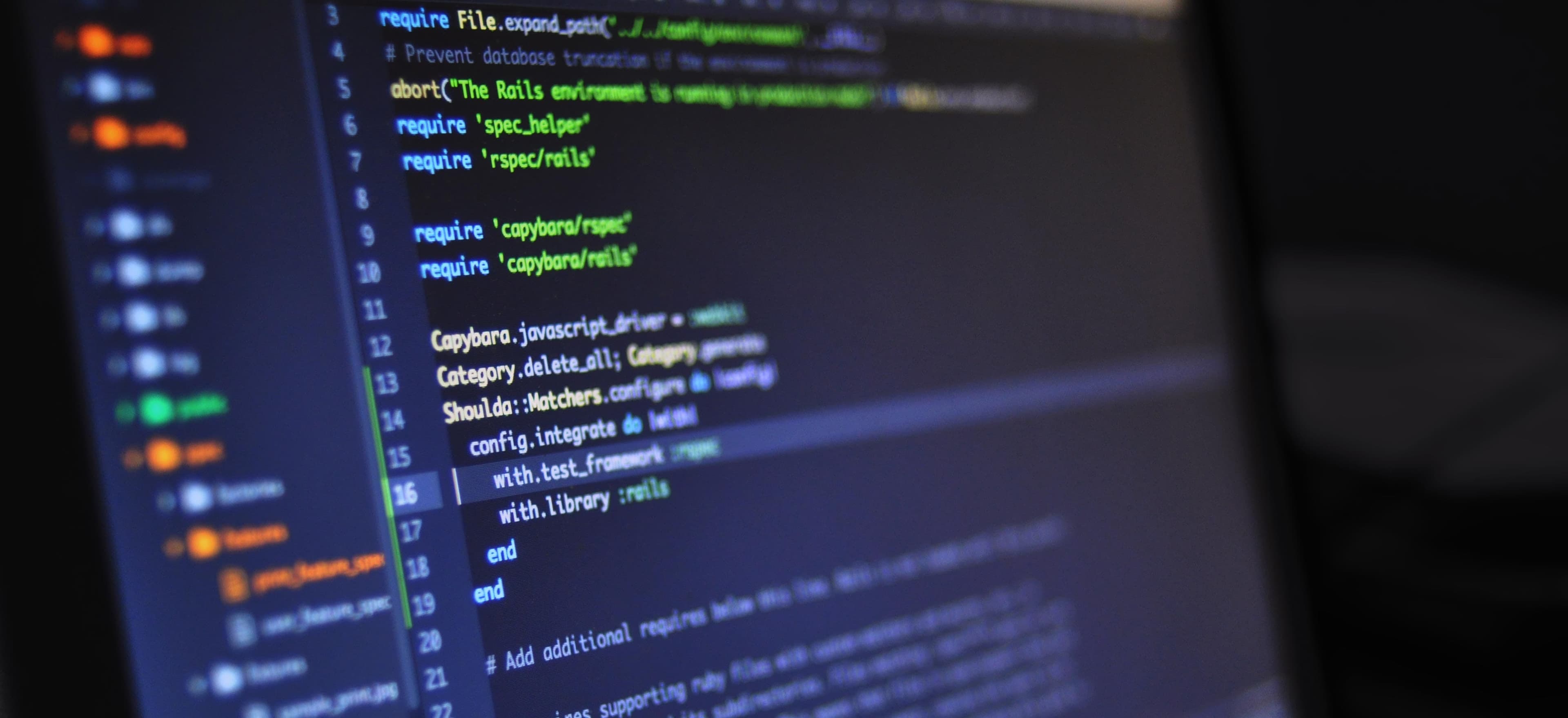
- Published on
Exploring the Reasons Behind Raw String Literals' Removal in Java
In the evolving realm of programming languages, the preservation and introduction of features are often vital topics of discussion. One particular feature that has seen significant debate within the Java community is the raw string literal. Initially proposed to simplify string handling, raw string literals were ultimately removed from the language before their official release in Java. This blog post will delve into the reasons behind the removal of raw string literals in Java, discuss their implications for Java developers, and propose alternative approaches to tackle common string manipulation challenges.
What Are Raw String Literals?
Raw string literals are a feature intended to allow developers to define strings without the need for escaping characters. For example, a raw string would permit the use of double quotes or backslashes without the need for escape sequences, making it easier to work with multiline strings or complex regular expressions.
The following pseudo-example illustrates a raw string literal:
String rawString = """
This is a raw string: "quotes" and \ backslashes
go unescaped.
""";
Advantages of Raw String Literals
- Simplicity: Writing complex strings becomes less cumbersome without the need for excessive escape characters.
- Readability: Code that contains multilines or nested quotations can be significantly more readable.
- Convenience: Helpful for documentation strings or SQL queries directly embedded within code, improving maintainability.
The Removal: The Reasons Behind It
Despite their advantages, raw string literals were ultimately removed from Java. Several key reasons contributed to this decision:
1. Complexity in Implementation
The implementation of raw string literals poses challenges. Java's type system and syntax parsing need to accommodate strings with various edge cases—like line breaks and different encoding formats. This significantly complicates the compiler's design, leading to potential performance issues and increased development time.
2. Impact on Existing APIs
Java has a well-established ecosystem where existing libraries and frameworks rely heavily on strings in their current form. The introduction of raw string literals could result in compatibility issues, especially concerning libraries that handle strings with specific expectations.
3. Alternative Solutions Exist
In most cases, the need to utilize raw string literals can be addressed through alternative approaches. Java developers can utilize string manipulation methods, regular expressions, or libraries that help with templated strings.
Here is an example of a Java code snippet that highlights how to handle complex strings using a combination of standard string literals and methods:
String queryTemplate = "SELECT * FROM users WHERE username = '%s' AND password = '%s'";
String username = "admin";
String password = "12345";
// Utilizing String.format to build a formatted query
String finalQuery = String.format(queryTemplate, username, password);
// This method is effective and avoids the need for raw string literals
System.out.println(finalQuery);
Why this approach works: The String.format()
method provides a way to construct complex strings without directly embedding dynamic values into the string, promoting better organization and security against SQL injection.
4. Lack of Demand
Although there was interest in the community for raw string literals, the demand has not reached a level compelling enough for the language stewards to prioritize it. The focus has generally been directed at enhancing other aspects of the language that affect a broader range of use cases.
5. Maintenance Issues
Adding a new feature demands continuous maintenance and documentation. The Java team decided that the potential trade-offs outweighed the benefits of raw string literals, especially in a language already boasting a rich set of features.
Navigating Alternative Approaches
Even though raw string literals have been removed, there are alternative strategies available to Java developers for handling complex strings.
1. Using StringBuilder
For dynamic string construction, Java's StringBuilder
class is highly effective:
StringBuilder sb = new StringBuilder();
sb.append("This is a string that spans ")
.append("multiple lines. ")
.append("No escaping needed here.")
.append("\n")
.append("It’s efficient for ")
.append("concatenation!");
String finalString = sb.toString();
System.out.println(finalString);
Why choose StringBuilder?: This approach minimizes the creation of intermediate string objects, which can enhance performance when dealing with large strings or frequent modifications.
2. Using External Libraries
Several libraries can aid in constructing complex strings instead of relying explicitly on Java's core string manipulation methods. The Apache Commons Lang library provides a utility class, StringUtils
, which offers many string manipulation functions that can replace the need for raw string literals.
Here is an example using StringUtils
for joining strings:
import org.apache.commons.lang3.StringUtils;
String[] parts = {"This is a string", "joined via", "StringUtils."};
String joinedString = StringUtils.join(parts, " "); // joins with a space
System.out.println(joinedString); // Output: This is a string joined via StringUtils.
3. Leveraging Regular Expressions
Java includes robust support for regular expressions through the java.util.regex
package. This feature is especially handy for manipulating strings that require complex replacements:
import java.util.regex.*;
String input = "This is a test. Testing, one two three.";
String output = input.replaceAll("test", "example"); // replaces matches with "example"
System.out.println(output); // Output: This is a example. Exampleing, one two three.
Why use regular expressions?: Regex enables powerful pattern matching and manipulation, often allowing developers to achieve results more succinctly than with basic string functions.
My Closing Thoughts on the Matter
While raw string literals presented an appealing feature of Java by simplifying string manipulation, their removal was driven by complex implementation challenges, potential maintenance issues, and the existence of effective alternatives. Java developers are still equipped with versatile tools—like StringBuilder
, third-party libraries like Apache Commons Lang, and regular expressions—to handle intricate string manipulations seamlessly.
For further insights, you can explore these resources: The Java Tutorials: Strings and Effective Java by Joshua Bloch.
In a continually evolving programming landscape, it's clear that the focus should remain on leveraging the tools available to enhance developer productivity while maintaining the integrity of the language. With innovation and research extending far beyond raw string literals, Java continues to be a powerful tool for developers around the world.
Checkout our other articles