Mastering JavaFX: Icon Definitions in CSS Made Easy
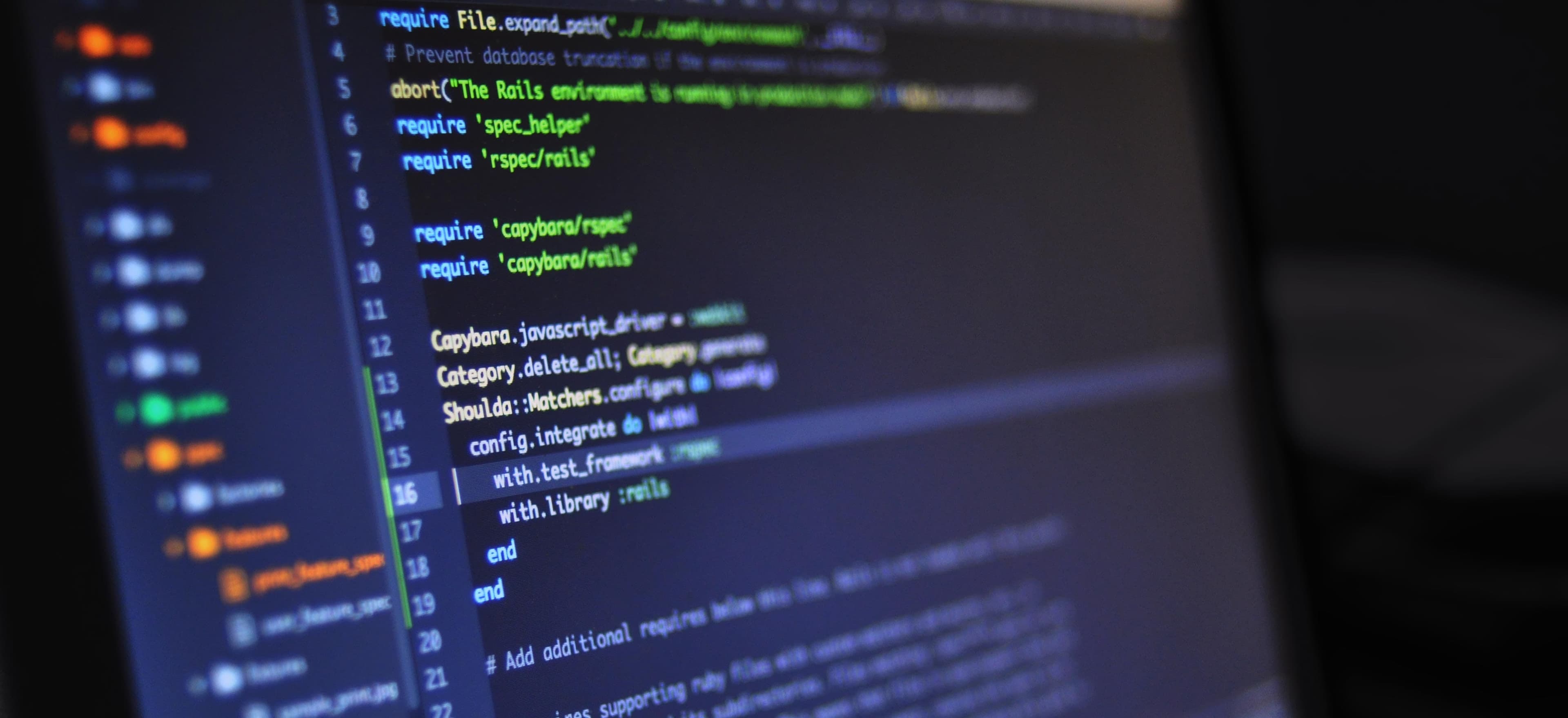
- Published on
Mastering JavaFX: Icon Definitions in CSS Made Easy
JavaFX is a powerful framework that allows developers to create rich desktop applications with ease. One of the standout features of JavaFX is its support for CSS styling, which provides a significant amount of flexibility in designing applications. In this blog post, we'll dive deep into how to define and use icons in JavaFX using CSS, making your applications visually appealing and user-friendly.
Table of Contents
- Introduction to JavaFX and CSS
- Image Resources: The Foundation of Icon Use
- Defining Icons in JavaFX CSS
- Applying CSS Styles
- Best Practices for Icon Usage
- Conclusion
1. Introduction to JavaFX and CSS
JavaFX applications are built using a combination of Java code and FXML (an XML-based language for defining UI components). One of the big advantages of JavaFX is the ability to use CSS for styling, allowing developers to maintain a separation of concerns.
CSS in JavaFX can do everything from changing colors and fonts to setting images as icons. It makes your application not only look better but also easier to maintain.
2. Image Resources: The Foundation of Icon Use
Before diving into CSS, let’s first understand how to manage image resources in JavaFX. To effectively use icons in your application, ensure your images are organized logically within your project structure. A typical setup would look like this:
src/
└── main/
└── resources/
└── images/
├── icon-home.png
├── icon-settings.png
└── icon-user.png
This organization helps simplify path management. You should load your images in your Java code effectively as follows:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.stage.Stage;
public class IconExample extends Application {
@Override
public void start(Stage primaryStage) {
Image image = new Image(getClass().getResourceAsStream("/images/icon-home.png"));
ImageView imageView = new ImageView(image);
StackPane root = new StackPane(imageView);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("Icon Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary:
- getResourceAsStream: This method is used to load the image file as a stream. Make sure the resource path starts with a "/".
- ImageView: This JavaFX node is used to display images within your layout structure.
3. Defining Icons in JavaFX CSS
Once your images are organized and accessible, the next step is defining them in your CSS. Create a CSS file in your resources directory, e.g., style.css
. This file will include your icon definitions.
Example CSS
.icon-home {
-fx-background-image: url("/images/icon-home.png");
-fx-background-size: contain;
-fx-background-repeat: no-repeat;
-fx-pref-width: 32px;
-fx-pref-height: 32px;
}
Commentary:
- -fx-background-image: This CSS property applies the image as a background.
- url(): Ensure that you provide the correct path to the image.
- -fx-background-size: Setting it to
contain
ensures that the image fits within the allocated space proportionally. - -fx-pref-width & -fx-pref-height: Allows you to define the preferred size of your icon.
4. Applying CSS Styles
Now that we have the CSS class defined, we can apply it to any JavaFX control. A common approach is to utilize a Button node for an icon button.
Example Java Code
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class IconButtonExample extends Application {
@Override
public void start(Stage primaryStage) {
Button homeButton = new Button();
homeButton.getStyleClass().add("icon-home"); // Apply the CSS class
StackPane root = new StackPane(homeButton);
Scene scene = new Scene(root, 300, 250);
// Load CSS
scene.getStylesheets().add(getClass().getResource("/style.css").toExternalForm());
primaryStage.setTitle("Icon Button Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Commentary:
- homeButton.getStyleClass().add("icon-home"): This line applies the CSS class we've defined in the
style.css
file to the button. - scene.getStylesheets().add(...): This loads the CSS file into our scene, enabling the styles defined.
5. Best Practices for Icon Usage
While incorporating icons into your JavaFX application is straightforward, adhering to certain best practices can improve both the maintainability and usability of your application.
Consistency:
Ensure consistent sizing and positioning of all icons throughout your application.
Accessibility:
Use appropriate alternative texts or tooltips for users relying on assistive technologies.
Optimization:
Keep image sizes optimized for quick loading. PNG and SVG formats are preferable.
Version Control:
Maintain version control of your icon designs, ensuring easy updates and rollbacks.
External Libraries:
Consider using libraries like Font Awesome for icon management, which can provide scalable vector icons with CSS support.
6. Conclusion
Managing icons in JavaFX with CSS offers a neat and efficient way to enhance the visual appeal of your applications. By understanding the fundamentals of image management, defining icons in CSS, and applying them correctly, you can create user-friendly interfaces that engage users effectively.
For more insights on mastering JavaFX, consider exploring the official JavaFX documentation, or check out additional tutorials on JavaFX CSS Basics.
By following the practices outlined in this post, you can seamlessly integrate icons into your JavaFX applications. Happy coding!