Mastering Jelly Bean Notifications: Common Issues Solved!
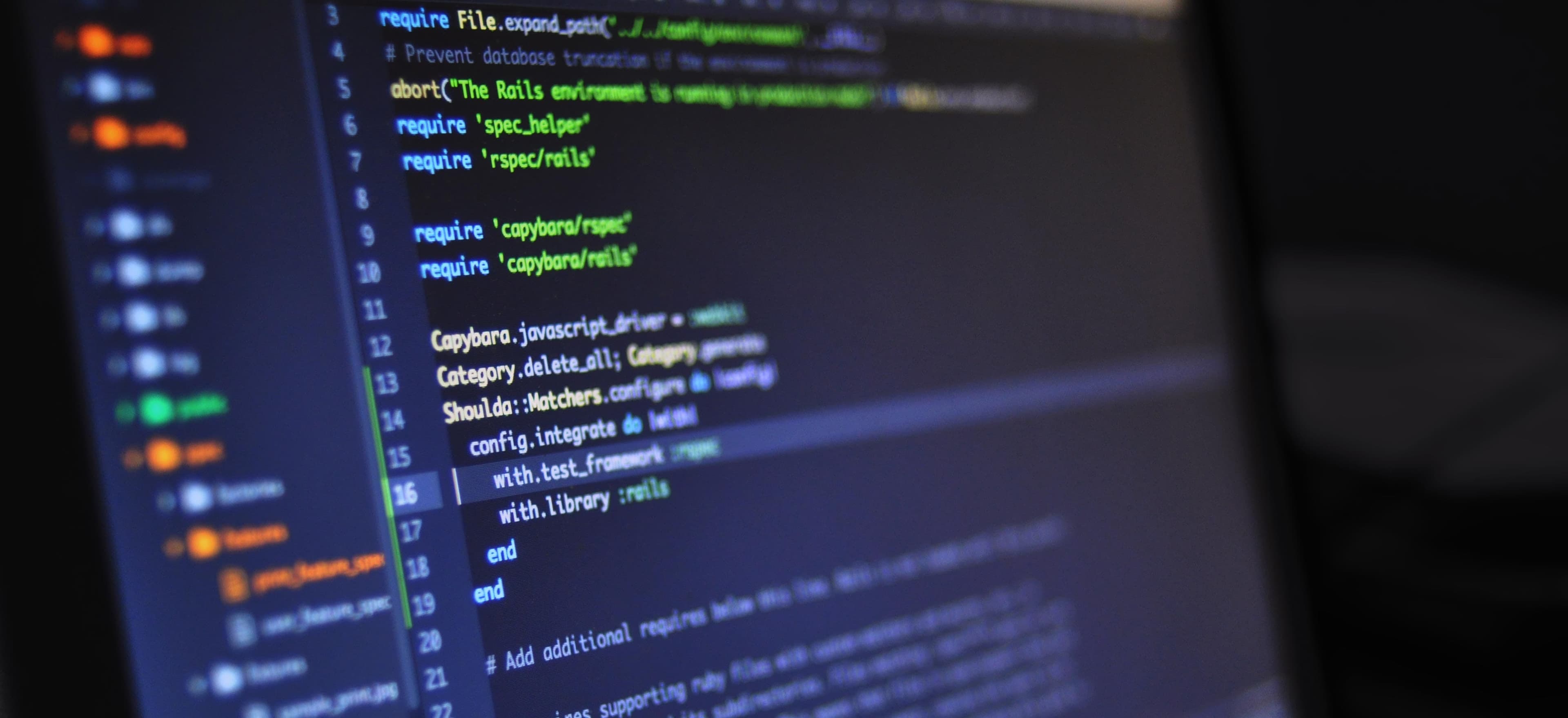
- Published on
Mastering Jelly Bean Notifications: Common Issues Solved!
The Android Jelly Bean (Android 4.1-4.3) era introduced a plethora of improvements, notably in the Notification system. However, developers often encounter specific issues while working with notifications in this version. In this blog post, we will explore some common issues related to Jelly Bean notifications and provide solutions to these dilemmas. This guide is designed for Android developers who want to enhance their applications' user experience through effective notifications.
Understanding Jelly Bean Notifications
Before diving into the problems, it’s essential to understand what makes the Jelly Bean Notification system unique. Notifications in Jelly Bean are more user-friendly and interactive. They support:
- Rich media notifications, including images and actionable buttons.
- A more streamlined design that focuses on user experience.
- Expanded notifications for more content visibility.
These enhancements allow developers to create notifications that not only inform users but also engage them.
Common Issues with Jelly Bean Notifications
Let’s delve into common issues developers face with Jelly Bean notifications, providing insights and solutions for each.
1. Notifications Not Displaying
Issue: In some instances, notifications do not appear on the device at all.
Solution: Ensure that the notification channel is correctly set up. While channels are primarily a feature of Android Oreo (8.0) and onwards, the absence or misconfiguration of notification setups might impact notifications across API levels.
// Creating a notification
NotificationCompat.Builder builder = new NotificationCompat.Builder(context, CHANNEL_ID)
.setSmallIcon(R.drawable.ic_notification)
.setContentTitle("New Message")
.setContentText("You have received a new message.")
.setPriority(NotificationCompat.PRIORITY_DEFAULT);
The CHANNEL_ID
must correlate with the correct notification channel. If you're working on older API systems or need to create a systematic default in newer versions, it's crucial to fill in even the layers that do not prompt notifications directly.
2. Notifications Are Not Clickable
Issue: Users sometimes find that clicking on notifications does not trigger the intended action.
Solution: This is frequently caused by failing to set the correct PendingIntent
. Make sure you define the intent correctly to handle clicks.
Intent intent = new Intent(context, YourActivity.class);
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK);
PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT);
// Attach it to the notification
builder.setContentIntent(pendingIntent);
By using PendingIntent.FLAG_UPDATE_CURRENT
, you ensure that any existing PendingIntent
matching the intent will be updated with the new intent’s data. This can make a significant difference in how notifications behave when clicked.
3. Notifications Overlapping
Issue: Multiple notifications can sometimes overlap, causing confusion for users.
Solution: Use unique notificationId
for each notification to manage them effectively.
int notificationId = generateUniqueId(); // A method to generate unique IDs
notificationManager.notify(notificationId, builder.build());
Implementing a method for unique IDs helps keep notifications distinct. Consider timestamp or a hash of the message content for creating unique identifiers.
4. Notifications Not Showing in the Status Bar
Issue: Users have reported that notifications sometimes don’t appear in the status bar.
Solution: Ensure your application has the proper permissions to display notifications. In particular, double-check your app’s settings in the device configuration.
<uses-permission android:name="android.permission.VIBRATE"/>
Additionally, don’t forget to check if "Do Not Disturb" mode is in effect, which can silence notifications across apps.
5. Sound & Vibration Not Working
Issue: Notifications might not be accompanied by sound or vibrations.
Solution: Confirm that you've defined sound and vibration options in your notification settings.
Uri alarmSound = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION);
builder.setSound(alarmSound)
.setVibrate(new long[] { 1000, 1000, 1000, 1000 });
Including a sound
URI and a vibration pattern allows you to customize how users receive your alerts.
6. Custom Views Not Rendering
Issue: Notifications created with custom layouts sometimes fail to render correctly.
Solution: Ensure that the custom view is inflated correctly and is not larger than the recommended dimensions for notifications.
RemoteViews customView = new RemoteViews(context.getPackageName(), R.layout.custom_notification);
builder.setContent(customView);
The notification layout should be simple and efficient while following the design guidelines set by Google. Note that complex layouts may have issues in rendering and performance.
Advanced Features to Consider
Once you've addressed the basic issues with your Jelly Bean notifications, you may want to explore more advanced topics, such as:
-
Action Buttons: Creating actionable notifications allows users to respond directly from the notification shade, improving user engagement.
-
Big Text and Big Picture Styles: Use NotificationCompat's expanded views to present additional information.
builder.setStyle(new NotificationCompat.BigTextStyle()
.bigText("Here is some more detailed text about the message."));
- Notifications with Progress: Helpful for long-running tasks, such as downloads, where users benefit from knowing the status.
builder.setProgress(100, progress, false);
Bringing It All Together
By mastering the common issues and their solutions surrounding Jelly Bean notifications, you stand a better chance of developing a user-friendly Android application. Notifications are a vital aspect of keeping users informed and engaged, and correctly managing them can significantly enhance the user experience.
For further reading, check out the following resources:
Remember that while Jelly Bean may seem dated, many users still operate on this version; thus, ensuring backward compatibility can significantly enhance your app's usability. Happy coding!