Why NoSQL is Essential for Java EE Development Today
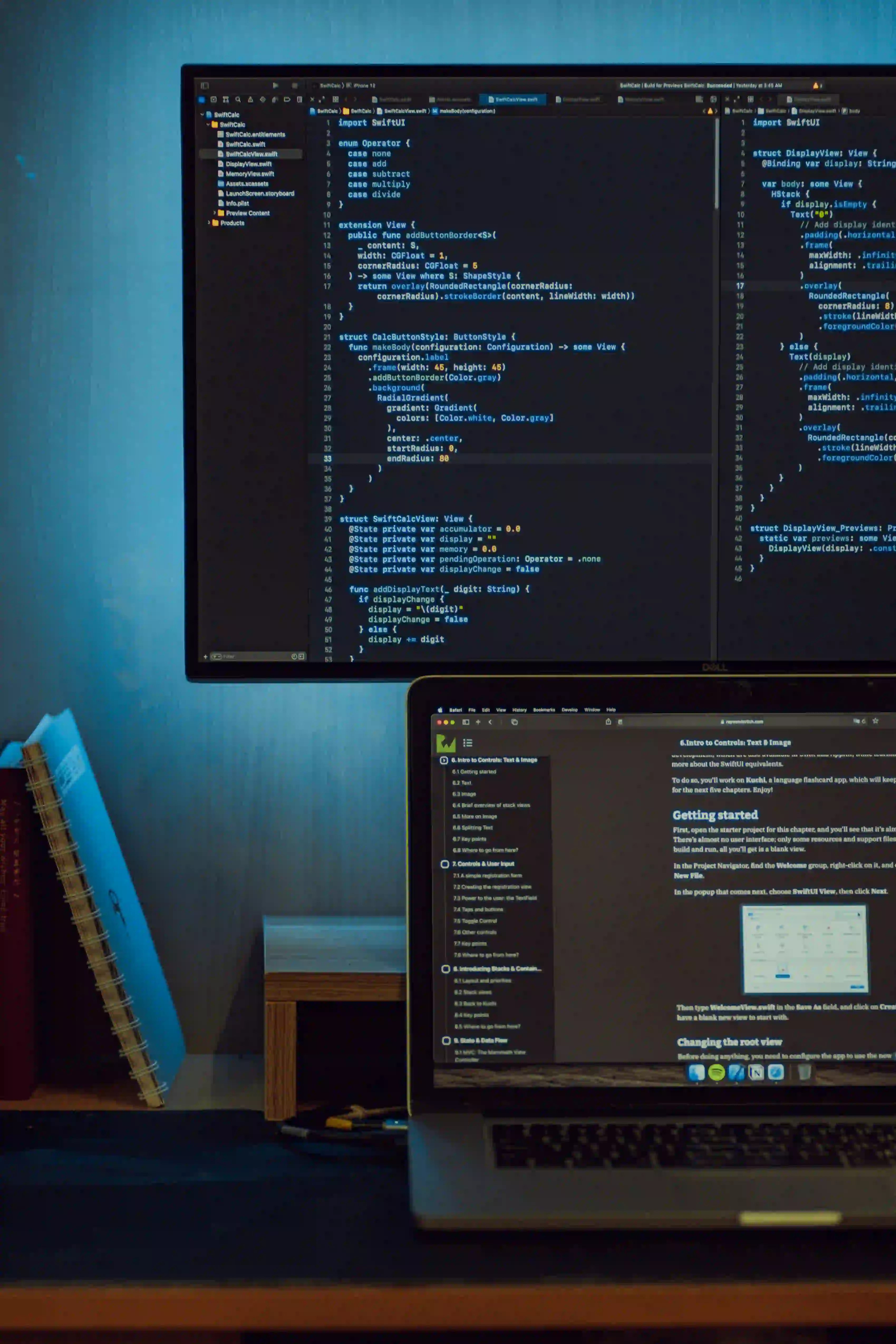
Why NoSQL is Essential for Java EE Development Today
In recent years, the dynamic nature of the web and the increasing volume of data have led to a significant shift in how applications are developed. As businesses strive to manage and analyze vast amounts of data, NoSQL databases are becoming integral to Java EE development. In this blog post, we will explore why NoSQL is essential for Java EE development today, its various advantages, and how it streamlines data management in modern applications.
Understanding NoSQL
NoSQL, or "Not Only SQL", represents a broad category of database management systems that do not rely solely on relational databases. Traditionally, database systems utilized structured query language (SQL) to define, query, and manipulate data. However, as the limitations of such systems became apparent, NoSQL emerged to offer more flexibility, scalability, and performance.
Types of NoSQL Databases
- Document Stores: These databases store data in JSON-like documents. Examples include MongoDB and CouchDB. They are particularly useful for applications with varying data structures.
- Key-Value Stores: These databases store data as a collection of key-value pairs. Redis and Amazon DynamoDB are common examples. They excel in speed and are perfect for caching.
- Column-Family Stores: Designed to handle large volumes of data, these databases store data in columns rather than rows. Apache Cassandra is a well-known column-family store.
- Graph Databases: These databases focus on relationships between data points. Neo4j is a popular choice for applications requiring complex querying of relationships.
Advantages of NoSQL for Java EE Development
1. Flexibility in Data Models
One of the core strengths of NoSQL databases is their flexibility in data modeling. In contrast to relational databases, which require a predefined schema, NoSQL allows developers to create dynamic schemas.
Example:
When building a content management system (CMS), the properties of different content types (e.g., articles, videos, images) can vastly differ. Using NoSQL, you can store each content type in the same collection without rigidly defining it in advance.
// Example of a document in MongoDB
Document article = new Document("title", "NoSQL in Java EE")
.append("author", "John Doe")
.append("tags", Arrays.asList("NoSQL", "Java EE", "Database"))
.append("publishedDate", new Date());
mongoCollection.insertOne(article);
In this example, the document structure can easily adapt to include additional fields like length
for a video or imageResolution
for images without necessitating any schema alterations.
2. Scalability
Scalability is crucial as applications grow. NoSQL databases are inherently designed for horizontal scaling, which makes it easy to distribute the dataset across multiple servers.
// In Apache Cassandra, you can scale by simply adding more nodes
Cluster cluster = Cluster.builder().addContactPoint("127.0.0.1").build();
Session session = cluster.connect("my_keyspace");
// Nodes can be added without downtime
As your user base expands, you can add more nodes seamlessly to handle the increased load.
3. High Performance
NoSQL databases are optimized for high read/write performance. They handle a variety of data formats and provide a low-latency response. This is particularly important for web applications that require real-time data processing.
For instance, when using Redis as a caching layer for session management:
// Example of caching user session data
Jedis jedis = new Jedis("localhost");
jedis.set("session:12345", "user_data_here");
Here, Redis provides rapid access to user session data, significantly reducing the average response time of requests.
4. Schema Evolution
With NoSQL databases, schema evolution becomes a breeze. As requirements change, you can modify your data structure without downtime, allowing businesses to adapt quickly.
5. Built for Big Data
As the volume of data grows exponentially, NoSQL databases have emerged as vital tools for big data solutions. They can handle massive amounts of structured and unstructured data seamlessly.
For example, Apache Cassandra is well-suited for storing time-series data generated by IoT devices or social media applications. With its ability to write high volumes of data quickly, it becomes an essential asset in data analytics.
6. Cost-Effectiveness
Many NoSQL solutions offer open-source options. This not only lowers the cost of entry but also encourages a large community-driven ecosystem. In cases where your application scales, the flexibility of cloud-based NoSQL solutions can also lead to significant cost savings on database management.
Implementing NoSQL in Java EE Applications
Integrating NoSQL into Java EE applications can be relatively straightforward, thanks to various libraries and frameworks.
1. Using Spring Data
Spring Data provides support for NoSQL databases making it easy to integrate NoSQL operations into your Java EE applications. Here's a brief example of using Spring Data MongoDB:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.mongodb.core.MongoTemplate;
import org.springframework.stereotype.Service;
@Service
public class ArticleService {
@Autowired
private MongoTemplate mongoTemplate;
public void saveArticle(Article article) {
mongoTemplate.save(article);
}
}
Using Spring Data simplifies your interaction with MongoDB, automatically managing the persistence logic.
2. Jakarta Persistence API (JPA) for NoSQL
The JPA specification has now extended to support NoSQL databases. Examples include EclipseLink for MongoDB or Hibernate OGM for various NoSQL stores. Using JPA, developers can interact with NoSQL databases in a familiar way.
@Entity
public class User {
@Id
private String id;
private String name;
// Getters and setters...
}
Through abstraction, you gain the ability to work with NoSQL databases using an ORM paradigm alongside relational databases.
To Wrap Things Up
The increasing complexity and volume of data in today's applications necessitate a departure from traditional relational databases. NoSQL provides the flexibility, scalability, and performance necessary to address modern data challenges. Java EE developers benefit significantly from these features, enabling them to create more versatile and robust applications.
As businesses continue to evolve, embracing NoSQL technology will be essential in meeting user demands and maintaining a competitive edge. To learn more about NoSQL databases, check out these resources from MongoDB and Apache Cassandra.
Additional Resources:
By integrating NoSQL into Java EE development today, you're not just future-proofing your applications but also embracing an innovative data paradigm that enables endless possibilities.