Common Pitfalls for Hazelcast Beginners and How to Avoid Them
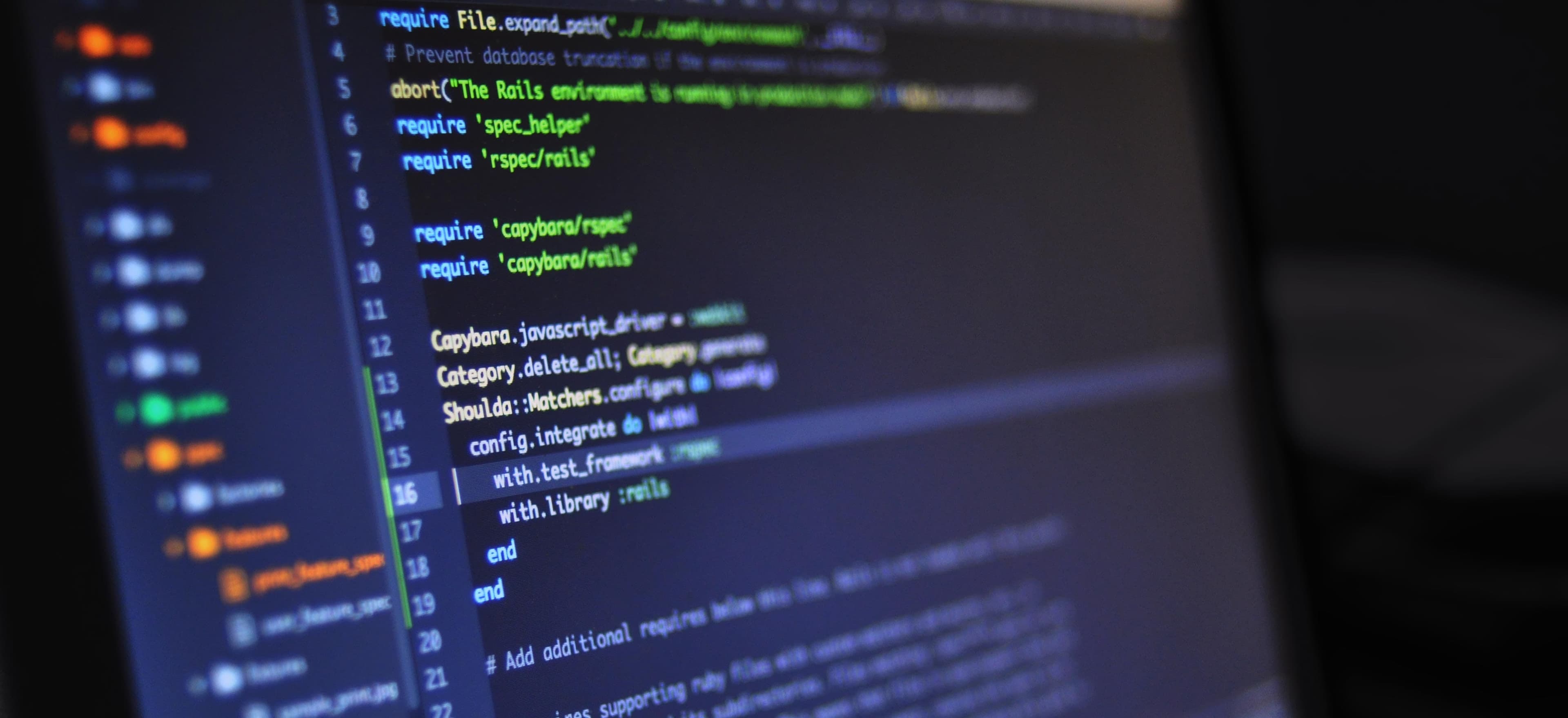
- Published on
Common Pitfalls for Hazelcast Beginners and How to Avoid Them
Hazelcast, as an in-memory data grid platform, offers a powerful solution for scaling applications and improving performance. However, like any technology, beginners may encounter pitfalls that can hinder their success. In this blog post, we will discuss common mistakes beginners make with Hazelcast and how to avoid them. By understanding these pitfalls and their solutions, you can harness the true power of Hazelcast efficiently.
Understanding Hazelcast
Before diving into the common pitfalls, it is essential to understand what Hazelcast is. Hazelcast provides distributed data structures such as maps, queues, and sets, along with several powerful features like distributed computing, stream processing, and more. It enables you to store and process large sets of data in a distributed manner across a cluster of nodes.
For more detailed information on Hazelcast, visit the official Hazelcast documentation.
Common Pitfalls
1. Ignoring Configuration Defaults
The Problem
Many beginners dive headfirst into coding without paying attention to the configuration settings. Hazelcast comes with several default configurations that might not align with your application requirements. Relying entirely on these defaults can lead to performance issues, particularly as your data grows.
The Solution
Always review the default configurations in your Hazelcast setup. This includes settings like:
- Memory Allocation: Ensure that your JVM has enough heap space for Hazelcast to operate effectively.
- Network Settings: Check the network timeout and other parameters to avoid unexpected disconnections.
<hazelcast>
<cluster>
<network>
<join>
<multicast enabled="false"/>
<tcp-ip enabled="true">
<member>192.168.1.1:5701</member>
<member>192.168.1.2:5701</member>
</tcp-ip>
</join>
</network>
</cluster>
</hazelcast>
In this XML configuration, the TCP-IP join method is enabled. Ensure that the members list includes all servers required for your cluster to function correctly.
2. Underestimating Data Serialization
The Problem
Serialization is the process of converting an object into a format that can be easily transported or stored. Newcomers often overlook the significance of data serialization in Hazelcast, leading to performance bottlenecks and increased latency.
The Solution
Make use of efficient serialization mechanisms. Hazelcast supports several serialization strategies, including:
- Java Serialization: Easy to implement but inefficient.
- IdentifiedDataSerializable: Offers better performance through field-based serialization.
- Portable Serialization: Allows cross-version compatibility, making it ideal for evolving schemas.
public class Person implements IdentifiedDataSerializable {
private String name;
private int age;
@Override
public void writeData(ObjectDataOutput out) throws IOException {
out.writeUTF(name);
out.writeInt(age);
}
@Override
public void readData(ObjectDataInput in) throws IOException {
name = in.readUTF();
age = in.readInt();
}
@Override
public int getFactoryId() {
return 1; // Factory ID for this class
}
@Override
public int getId() {
return 1; // Class ID for this class
}
}
In the above code, we implement the IdentifiedDataSerializable
interface, which allows for more efficient serialization compared to standard Java serialization. Custom logic for reading and writing fields is provided to enhance performance.
3. Neglecting Backup and Partitioning
The Problem
Hazelcast enables data persistence through partitioning and backup. Beginners may ignore these important features, which can lead to data loss when nodes fail.
The Solution
Set up proper partitioning and backup strategies in your cluster. You can specify the number of backups per data structure to ensure redundancy.
Config config = new Config();
MapConfig mapConfig = new MapConfig("myMap")
.setBackupCount(2);
config.addMapConfig(mapConfig);
In this code snippet, we configure a map named "myMap" to have two backups in case of node failure, ensuring that your data is redundantly stored across different cluster members.
4. Not Utilizing Hazelcast API Features
The Problem
Hazelcast has a rich API with numerous features, but beginners often stick to basic functionalities without exploring the more advanced methodologies, such as distributed executors or listeners.
The Solution
Take the time to explore the Hazelcast API thoroughly. Utilize features like:
- Distributed Executors: Run tasks on the cluster nodes.
- Listeners: Trigger actions based on events in data structures.
IMap<String, String> map = hazelcastInstance.getMap("myMap");
map.addEntryListener(new EntryListener<String, String>() {
@Override
public void entryAdded(EntryEvent<String, String> event) {
System.out.println("Entry added: " + event);
}
// Other listener methods (entryRemoved, etc.)
}, true);
Adding an entry listener enables you to react to changes in your map in real-time, enhancing your application's responsiveness to data changes.
5. Mismanaging Resource Cleanup
The Problem
Failing to clean up resources properly is a common mistake. Resources like connections and data structures can quickly lead to memory leaks if they are not managed effectively.
The Solution
Implement appropriate cleanup strategies in your application. Shutdown the Hazelcast instance when it is no longer needed. Use the HazelcastInstance.getLifecycleService()
method to monitor the lifecycle of your instance.
hazelcastInstance.shutdown();
Calling the shutdown method on your Hazelcast instance properly releases all resources and avoids potential memory leaks, laying the groundwork for better application performance.
Closing the Chapter
Hazelcast is an immensely powerful tool that can dramatically improve your application's scalability and performance. However, as with any technology, beginners must avoid common pitfalls that can hinder their progress. By understanding configuration defaults, optimizing serialization, utilizing proper backup and partitioning strategies, exploring API features, and responsibly managing resource cleanup, you can position yourself for success with Hazelcast.
For further learning, consider exploring the Hazelcast GitHub Repository and the community forum for discussions and answers to your queries.
Harnessing the full potential of Hazelcast will not only enhance your data management capabilities but also pave the way for building robust, scalable applications.
This article aims to provide you with actionable insights, ensuring you navigate the complexities of Hazelcast with confidence. Happy coding!