Streamlining Fluent Object Creation: Common Pitfalls to Avoid
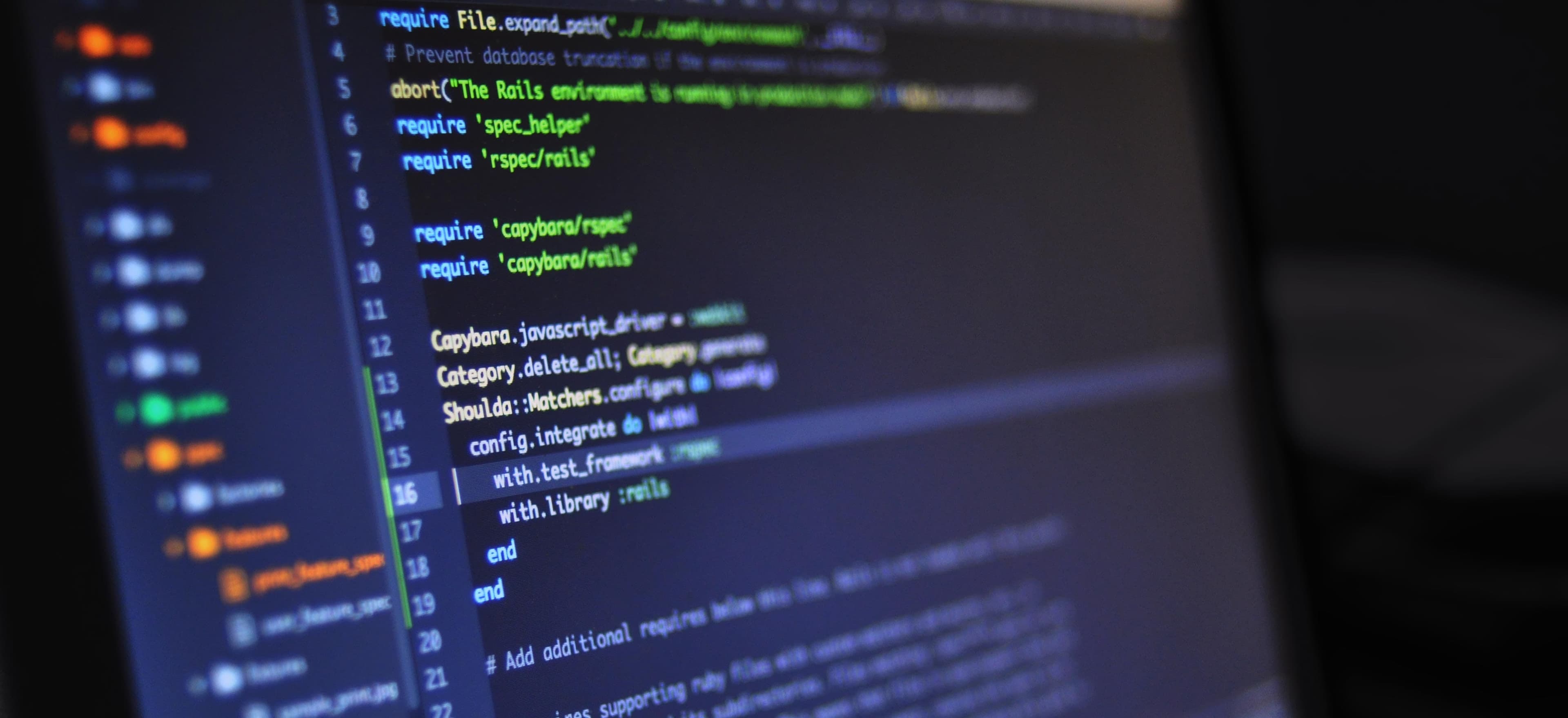
- Published on
Streamlining Fluent Object Creation: Common Pitfalls to Avoid
Fluent interfaces are a powerful design pattern in software development, particularly in Java. They allow for the creation of objects in a more readable and expressive manner, making your code cleaner and easier to maintain. However, using fluent interfaces effectively requires a detailed understanding of common pitfalls that can lead to confusing or buggy code. In this blog post, we will explore these pitfalls, share insightful examples, and ultimately help you create more robust and expressive code using fluent interfaces.
What is a Fluent Interface?
A fluent interface is an approach to configuring Java objects where the method calls can be chained together in a manner that is often more human-readable. This is achieved by returning the current object in each method, allowing multiple method calls to be linked in a single line.
Key Benefits of Fluent Interfaces
- Readability: Code becomes more descriptive and easier to follow.
- Intent: In some cases, fluent methods resemble natural language.
- Reduction of Boilerplate: Helps in reducing repetitive setter calls.
Basic Example of Fluent Object Creation
Before diving into common pitfalls, let's look at a basic example.
public class Person {
private String name;
private int age;
public Person setName(String name) {
this.name = name;
return this;
}
public Person setAge(int age) {
this.age = age;
return this;
}
@Override
public String toString() {
return "Person{name='" + name + "', age=" + age + "}";
}
}
// Usage
public class Main {
public static void main(String[] args) {
Person person = new Person()
.setName("John Doe")
.setAge(30);
System.out.println(person);
}
}
In this code, the Person
class chain-calls setName
and setAge
in a readable manner.
Common Pitfalls
While fluent interfaces can enhance your code, they come with their own challenges. Let's explore these pitfalls in detail.
1. Returning "This" Incorrectly
One of the most critical aspects of implementing a fluent interface is ensuring that methods return the correct instance. Incorrectly returning a different object can lead to unexpected behavior.
public class Builder {
private String field;
public Builder setField(String field) {
this.field = field;
return this; // Correctly return the current object.
}
public Builder build() {
// Don't return a new Builder here; return the constructed object.
return new Builder();
}
}
In the above example, the build()
method incorrectly instantiates a new Builder
instead of returning the constructed instance. This can confuse users expecting to continue chaining methods after build()
.
2. Long Method Chains
While chaining methods can lead to succinct code, excessively long method chains can reduce readability. It can become difficult to follow the logic when the chain stretches over multiple lines.
new Person()
.setName("John Doe")
.setAge(30)
.setAddress("123 Street")
.setPhone("1234567890")
.setEmail("john.doe@example.com");
To combat this, consider breaking down complex configurations into smaller methods or using a Builder
pattern to encapsulate the logic.
3. Solving Mutability Issues
Fluent interfaces often assume that the objects are mutable. However, this can introduce issues, especially in multi-threaded environments.
public class ImmutablePerson {
private final String name; // Must be final for immutability
private final int age;
public ImmutablePerson(Builder builder) {
this.name = builder.name;
this.age = builder.age;
}
public static class Builder {
private String name;
private int age;
public Builder setName(String name) {
this.name = name;
return this;
}
public Builder setAge(int age) {
this.age = age;
return this;
}
public ImmutablePerson build() {
return new ImmutablePerson(this);
}
}
}
In this case, the ImmutablePerson
class ensures that once an object is created, it cannot be altered, preventing potential side-effects in concurrent settings.
4. Navigating the Builder Pattern
While creating fluent interfaces, you might consider the Builder pattern. However, using the pattern incorrectly can make your code unnecessarily complex.
public class Car {
private String model;
private int year;
public static class Builder {
private String model;
private int year;
public Builder setModel(String model) {
this.model = model;
return this;
}
// Logic to ensure year is set, and perhaps other validations
public Builder setYear(int year) {
if (year < 1886) { // First automobile
throw new IllegalArgumentException("Year is not valid");
}
this.year = year;
return this;
}
public Car build() {
return new Car(model, year);
}
}
private Car(String model, int year) {
this.model = model;
this.year = year;
}
}
The above Builder
takes care of ensuring that the year
is a valid value. However, if your Builder
becomes too complex, re-evaluate whether you truly need a Builder or if you can simplify your fluent interface.
5. Chaining Non-Chainable Methods
Mixing chainable methods with methods that do not return this
can complicate the fluent interface experience. You should always design methods that return this
within the context of a fluent interface.
public class Rectangle {
private int length;
private int width;
public Rectangle setLength(int length) {
this.length = length;
return this;
}
public Rectangle setWidth(int width) {
this.width = width;
return this;
}
// Not Fluent
public void draw() {
// Draw the rectangle without returning this -- molecules fluent.
}
}
When designing your methods, ensure they maintain consistency in their return types.
Conclusion
Fluent interfaces are a potent addition to any Java developer's toolkit. However, misuse can lead to confusion and complexity. By being aware of common pitfalls, such as incorrect use of method chaining, mutability issues, and maintaining readability, you can streamline your object creation process.
Resources for Further Reading
- Effective Java by Joshua Bloch
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma et al.
- Java Language Documentation for deeper insights into language features.
Embrace fluent interfaces, but do so with caution. Create a balance between readability and maintainability to produce the best Java code. Happy coding!
Checkout our other articles