Challenges of Integration Testing with External Services
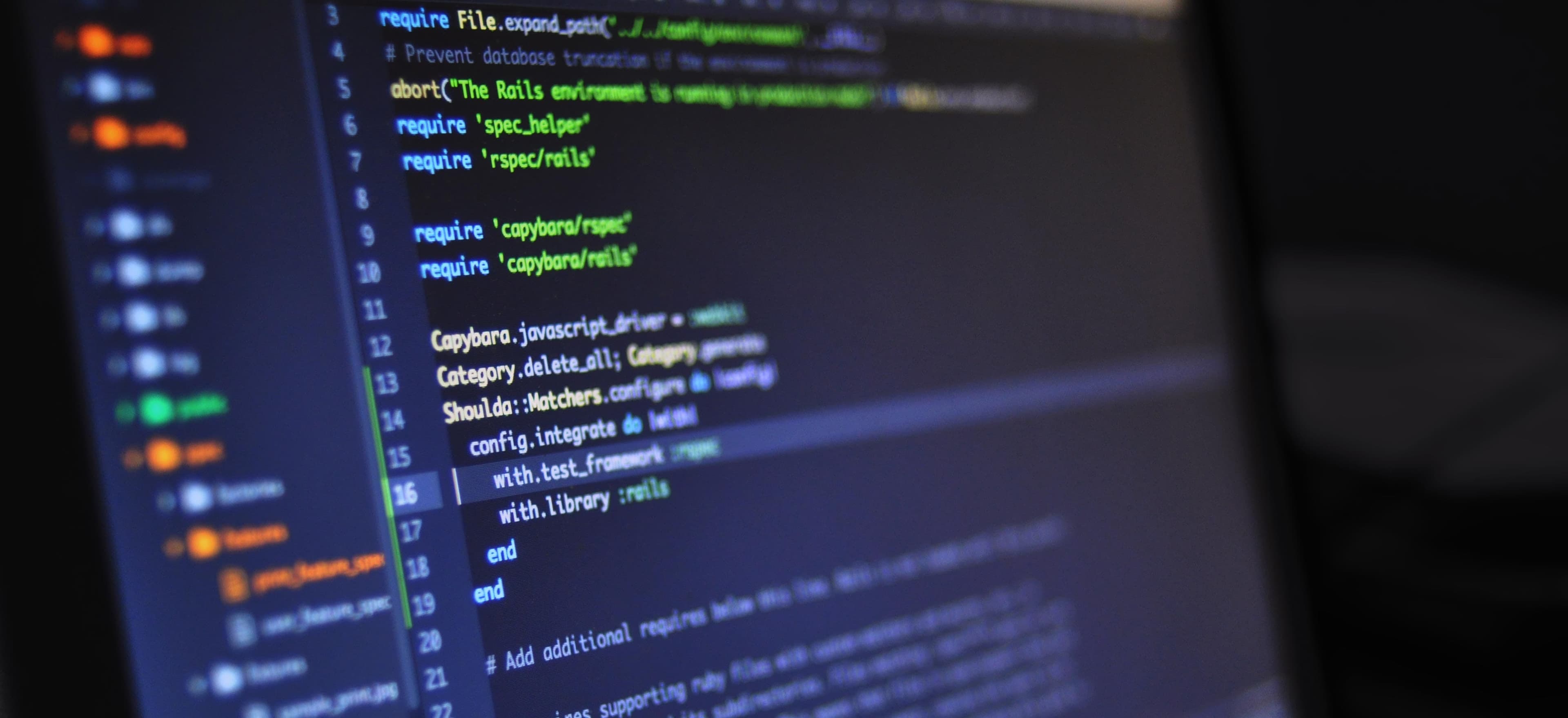
- Published on
Challenges of Integration Testing with External Services
Integration testing is an essential software development phase, playing a critical role in ensuring that various modules of a software application work together as intended. When integrating external services, the complexity of these tests can increase significantly. In this blog post, we will explore the challenges of integration testing with external services, discussing the implications, best practices, and providing sample code snippets to illustrate key points.
Understanding Integration Testing
Before diving into the challenges, let's clarify what integration testing entails. Integration testing focuses on the interaction between different components of a system. This includes:
- Testing interactions between modules: Confirming that different parts of an application work together.
- Validating data flow: Ensuring that data is passed correctly between components.
- Confirming external systems integration: Ensuring external services or APIs function as expected when interacting with your application.
The Role of External Services
In modern software development, external services such as API integrations, payment gateways, and third-party libraries are commonplace. While they add valuable functionalities, they also introduce several challenges in integration testing.
Challenges of Integration Testing with External Services
1. Unpredictability of External Services
External systems may be subjected to varying loads, data structures, or even downtime. This unpredictability can lead to flakiness in your tests. Here’s a brief example to illustrate this point:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class ExternalServiceTest {
private ExternalServiceClient externalServiceClient = new ExternalServiceClient();
@Test
public void testServiceResponse() {
// As an external service may not be available, this test
// could fail solely due to service downtime.
Response response = externalServiceClient.getData();
assertEquals(200, response.getStatusCode());
}
}
In the above code, the test retrieves data from an external service. If that service is down or changes its response format, it can lead to inconsistent test results.
2. Mocking Third-Party APIs
Mocking external dependencies can help alleviate some unpredictability; however, creating a realistic mock is challenging. Poorly designed mocks lead to tests that pass but do not accurately reflect potential failures in production.
To create a useful mock, consider using libraries such as Mockito or WireMock in Java. Here’s an example using Mockito:
import static org.mockito.Mockito.*;
public class PaymentServiceTest {
@Test
public void testProcessPayment() {
// Creating a mock of ExternalPaymentGateway
ExternalPaymentGateway paymentGateway = mock(ExternalPaymentGateway.class);
when(paymentGateway.process(any(Payment.class))).thenReturn(new PaymentResponse(200));
PaymentService paymentService = new PaymentService(paymentGateway);
PaymentResponse response = paymentService.processPayment(new Payment());
assertEquals(200, response.getStatusCode());
}
}
In this example, the ExternalPaymentGateway
is mocked to ensure that tests do not rely on the actual external service. However, it is crucial to simulate real-world scenarios accurately to gain meaningful insights.
3. Rate Limiting and Quotas
Once your test interacts with external services, you may encounter rate limiting. Most APIs impose constraints on how many requests can be made over a specific time frame. Hitting these limits can result in test failures due to throttled responses.
To mitigate this, aggregate tests so that they run at optimal times when outside of peak request periods. Furthermore, consider using asynchronous calls to manage execution time better.
4. Dependency on Network Conditions
Network availability affects integration tests, especially in environments where stable internet connections are not guaranteed. Your tests might fail due to timeout exceptions or communication errors that are entirely out of your control.
public class NetworkTest {
@Test
public void testNetworkTimeout() {
NetworkClient networkClient = new NetworkClient();
assertThrows(TimeoutException.class, () -> {
networkClient.makeRequest("https://api.example.com/data");
});
}
}
In the code snippet above, a test simulates a network timeout, demonstrating the importance of handling various network scenarios gracefully.
5. Security and Data Privacy Concerns
When integrating with external services, you must manage sensitive data carefully. Issues related to authentication, encryption, and overall data privacy must be addressed.
For example, when testing API requests that require authentication, always use environment variables or secure vaults to store sensitive information:
import org.springframework.beans.factory.annotation.Value;
public class SecureService {
@Value("${api.key}")
private String apiKey;
public ApiResponse fetchData() {
// Use the API key to authenticate, ensuring sensitive data is protected.
}
}
This method ensures that sensitive information is kept out of your codebase, reducing the risk of exposure.
Best Practices for Integration Testing with External Services
While challenges abound, adopting best practices can help overcome these hurdles:
- Use Mocks and Stubs: Always mock services when possible, ensuring realistic behavior aligned with potential production conditions.
- Implement Retry Logic: Employing retry mechanisms can be vital in reducing flakiness due to intermittent failures caused by external services.
- Continuous Monitoring: Keep your integration tests under continuous monitoring to identify changes in response from external services.
- Handle Errors Gracefully: Your application should anticipate and manage errors from external services, using structured logging and error messaging.
- Invest in Test Environments: Create isolated and stable environments for running integration tests, limiting disruptions due to network conditions.
Final Considerations
Integration testing with external services is filled with challenges, yet it remains a cornerstone of developing robust applications. Understanding the unpredictability of external services, managing mocks properly, and adopting best practices will enhance your testing process and lead to better software quality.
For further reading on effectively managing external API dependencies, check out How to Mock REST APIs and Testing in the Cloud: Strategies and Tools. Embrace these principles, and your integration testing process will yield more reliable results, ensuring that you deliver a resilient product to your users.
Checkout our other articles