Top 5 REST API Mistakes and How to Avoid Them
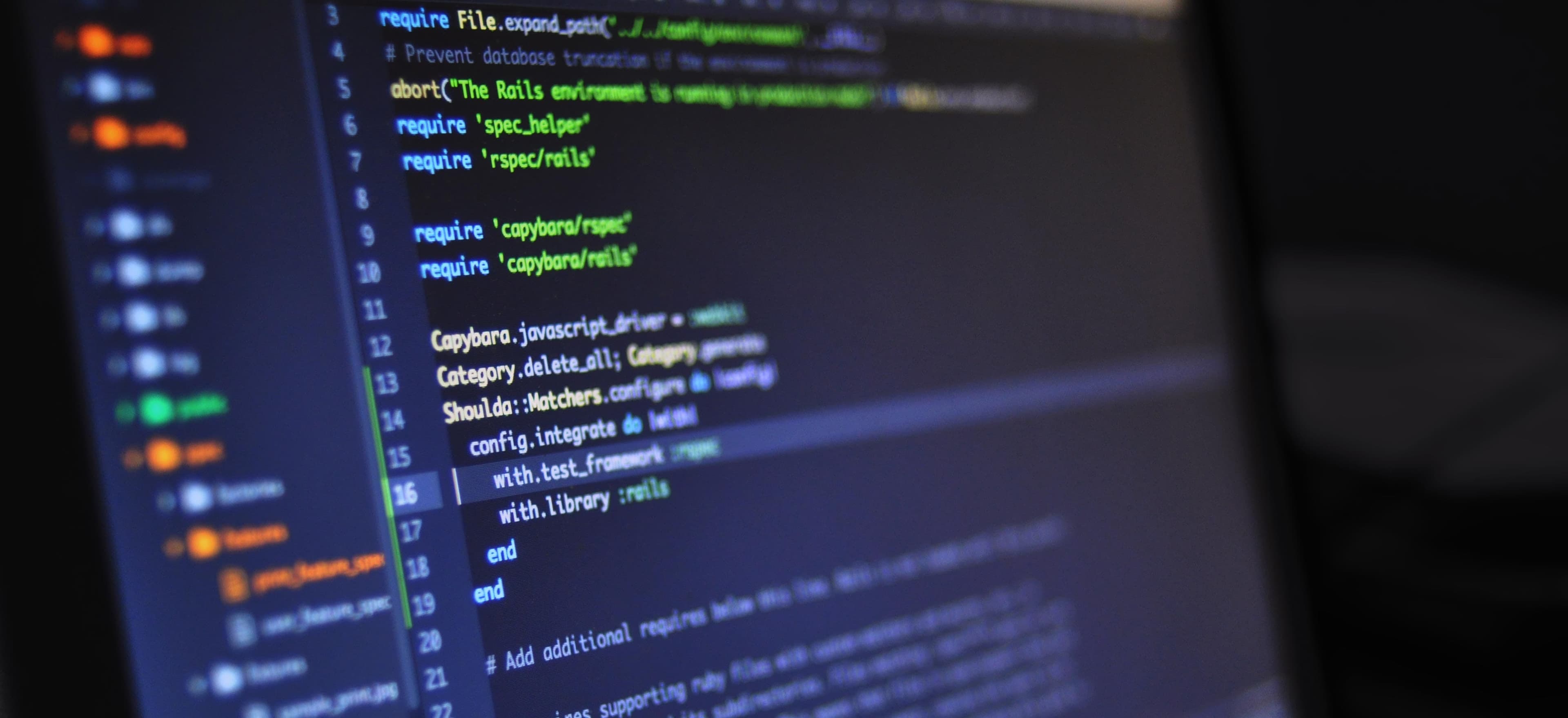
- Published on
Top 5 REST API Mistakes and How to Avoid Them
Creating a REST API can be a daunting task, especially if you are new to web development or the concept of RESTful services. There are many pitfalls that developers commonly encounter, leading to poorly designed APIs that fail to meet users' needs or make integration with other services cumbersome. In this blog post, we’ll discuss the top 5 REST API mistakes and how to avoid them, ensuring that your API is efficient, user-friendly, and scalable.
1. Inconsistent Naming Conventions
The Mistake
One of the most common mistakes when designing a REST API is inconsistent naming conventions. APIs should use clear, descriptive names for endpoints, and adhering to a consistent style is crucial. Whether you choose camelCase, snake_case, or kebab-case, the key is to stick to it across the entire API.
Why It Matters
Inconsistencies can lead to confusion and frustration for developers who are using your API. Clear and consistent naming makes it easier to remember endpoints and reduces the cognitive load.
How to Avoid
- Choose a Style: Decide on a naming convention at the beginning of your project.
- Document It: Keep a style guide that includes examples.
- Review Code: Perform regular audits of your API to ensure naming consistency.
Example Code Snippet
// Example of consistent endpoint naming
@GetMapping("/api/v1/users")
public ResponseEntity<List<User>> getUsers() {
//...
}
In this example, we are using kebab-case (getUsers
) consistently across our API versioning (/api/v1/
). This clarity aids in understanding what the function does at a glance.
2. Lack of Proper Versioning
The Mistake
Failing to properly version your API can lead to major disruptions. If you are making changes to your API and don't have a versioning strategy, users might be thrown off by unexpected changes.
Why It Matters
Versioning ensures that both developers and users can maintain compatibility with the features and data structure they rely on, thus avoiding breaking changes.
How to Avoid
- Include Version in the URL: Use a version number in the URL (e.g.,
/api/v1/
). - Semantic Versioning: Follow a versioning system that makes sense for your API (usually MAJOR.MINOR.PATCH).
Example Code Snippet
@GetMapping("/api/v1/products")
public ResponseEntity<List<Product>> getProducts() {
//...
}
In this example, /api/v1/
clearly indicates that this is version 1 of the API for products. If you need to make breaking changes in the future, you can create /api/v2/products
.
3. Not Utilizing HTTP Methods Properly
The Mistake
Many developers misuse HTTP methods when designing their REST APIs. For instance, they might use GET to create a resource or DELETE to retrieve data.
Why It Matters
Utilizing HTTP methods correctly is essential for building a RESTful architecture. Each method has a specific purpose, and using them adequately helps to maintain the stateless nature of REST.
How to Avoid
- Follow REST Principles: Use GET for retrieving data, POST for creating resources, PUT/PATCH for updating, and DELETE for removing resources.
- Resource Identification: Clearly define the unique IDs for each resource to avoid ambiguity.
Example Code Snippet
@PostMapping("/api/v1/users")
public ResponseEntity<User> createUser(@RequestBody User user) {
// Logic to create user would go here
}
This example uses the POST method to create a user, which aligns perfectly with RESTful standards.
4. Ignoring Error Handling and Status Codes
The Mistake
Another common mistake is neglecting proper error handling and status code management. When a request fails, the API should provide sufficient feedback rather than returning a generic error response.
Why It Matters
Proper error handling and specific status codes help users identify problems quickly and resolve them, leading to smoother development in client applications.
How to Avoid
- Use Standard HTTP Status Codes: Familiarize yourself with HTTP status codes such as 200 (OK), 404 (Not Found), 500 (Server Error), etc.
- Provide Descriptive Error Responses: Include details that indicate what went wrong.
Example Code Snippet
@GetMapping("/api/v1/users/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
Optional<User> user = userService.findById(id);
if (user.isPresent()) {
return ResponseEntity.ok(user.get());
} else {
return ResponseEntity.status(HttpStatus.NOT_FOUND)
.body("User not found");
}
}
In this snippet, we return a 404 status code when a user is not found, along with a meaningful message. This makes it easier for front-end developers to handle errors appropriately.
5. Poor Documentation
The Mistake
Poor or nonexistent documentation is a critical oversight that can lead to users being unable to effectively utilize your API. Good documentation helps users understand your API's capabilities and how to interact with it.
Why It Matters
Well-documented APIs enhance usability and reduce the onboarding time for new developers, thereby improving the overall user experience.
How to Avoid
- Use Tools: Consider using tools such as Swagger or Postman to automatically generate API documentation.
- Include Examples: Provide clear usage examples and explain the purpose of each endpoint.
Example Documentation Snippet
### Get User by ID
**GET** /api/v1/users/{id}
**Parameters:**
- id: Unique identifier of the user.
**Response:**
- 200 OK: Returns user data.
- 404 Not Found: User not found.
**Example:**
GET /api/v1/users/1
This example provides clear instructions on how to use the endpoint, what to expect, and how to handle different responses.
Key Takeaways
Designing a REST API involves several crucial considerations. By avoiding the common pitfalls associated with inconsistent naming, lack of versioning, improper use of HTTP methods, insufficient error handling, and poor documentation, you can create a more robust, user-friendly API.
By adhering to best practices and laying a solid foundation now, you can save yourself and your team considerable time and energy in the future. Ultimately, a well-designed REST API will not only improve the experience for developers but also enhance the user experience at large.
For further reading on RESTful API best practices, check out these resources:
- RESTful API Design: Best Practices in a Nutshell
- Understanding RESTful Services in Java
- API Documentation Best Practices
Stay tuned for more insightful posts as we explore the world of REST APIs and related web technologies!
Checkout our other articles