Struggling to Install Local JAR with Maven? Here's How!
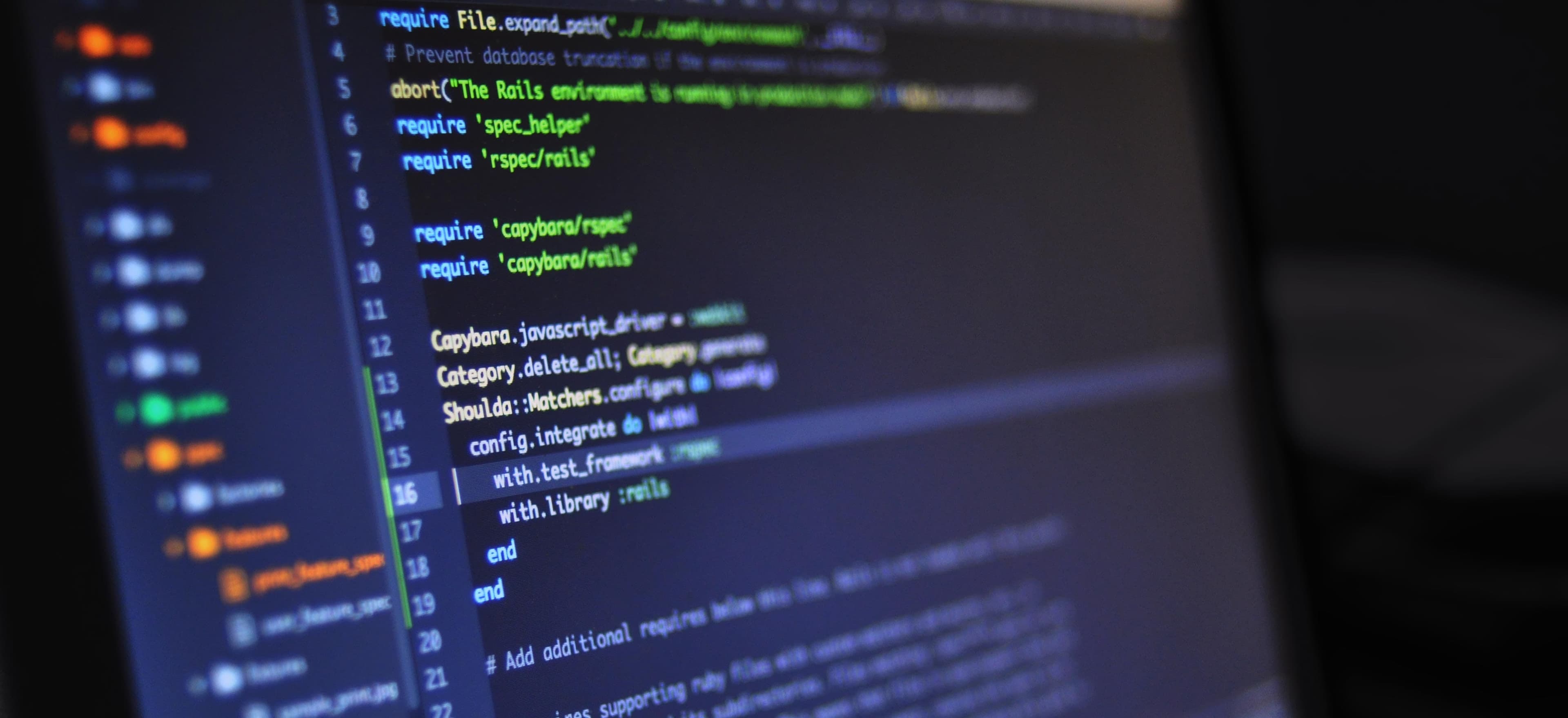
- Published on
Struggling to Install Local JAR with Maven? Here's How!
When it comes to Java development, Maven is one of the most indispensable tools in a developer's arsenal. However, when you don't find the JAR file you need in a Maven repository, installing a local JAR file can often become a stumbling block. This comprehensive guide will walk you through the steps to install a local JAR file using Maven, explaining the why’s and how’s along the way.
What is Maven?
Maven is a build automation tool used primarily for Java projects. It simplifies project management, builds, dependencies, and documentation. Central to Maven is its ability to manage dependencies via repositories—repositories are locations where libraries (or JAR files) are stored and can be retrieved.
Why Install a Local JAR in Maven?
There are instances when you may have a JAR file that is not available in any public Maven repository. This could be a proprietary library, a jar file from a third-party vendor, or even one that you have compiled yourself. Installing these local JARs allows you to incorporate their functionalities into your project easily.
Step-by-Step Guide to Install a Local JAR File
Step 1: Create a Maven Project
Before we dive into installing the local JAR, let's ensure you have a Maven project set up. You can create a new Maven project using the following command in your command line:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-project -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command generates a simple Maven project with the specified groupId
and artifactId
.
Step 2: Identify the Local JAR File
Next, make sure you have your local JAR file ready. Let's say you have a JAR file named my-library.jar
.
Step 3: Install the Local JAR with the Maven Install Plugin
To install a local JAR in Maven, you will use the maven-install-plugin
. The command to do this looks like:
mvn install:install-file -Dfile=/path/to/my-library.jar -DgroupId=com.example -DartifactId=my-library -Dversion=1.0 -Dpackaging=jar
Breaking Down the Command
-Dfile
: This specifies the absolute path to the JAR file you want to install.-DgroupId
: This is the unique identifier for your project, usually following the reverse domain name convention.-DartifactId
: This is the name of the library.-Dversion
: The version of the JAR file you are installing.-Dpackaging
: The type of package, which in this case isjar
.
Step 4: Update Your Maven Project's pom.xml
After installing the JAR file, you will need to update your project's pom.xml
file to include the newly installed JAR as a dependency.
Here’s how to do that:
<dependency>
<groupId>com.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0</version>
</dependency>
Why Update the pom.xml?
Updating the pom.xml
ensures that Maven is aware of the dependency and can include it during builds, tests, and packaging stages. Not updating the pom.xml
means you may encounter ClassNotFoundException
during runtime when you try to use the classes within the library.
Step 5: Verify the Installation
Once you've added the dependency to your pom.xml
, you can verify that the JAR is now included in your project by running:
mvn clean install
If everything is set up correctly, Maven should compile your project without issues and recognize the local JAR file.
Common Issues and Troubleshooting
-
JAR Not Found: If Maven cannot find the JAR during the installation command, double-check the path you provided. It should be the absolute path to the JAR file.
-
Version Conflicts: If you have an existing dependency with the same
groupId
,artifactId
, and a differentversion
, Maven may default to the older version, causing conflicts. Always ensure that your versions are consistent. -
Permissions: If you’re using a machine with restricted permissions, ensure that your user account has the necessary access to read the JAR file.
-
Repository Settings: In some instances, your Maven settings might have specific repository configurations that affect local installations. Check your
settings.xml
file located in the.m2
directory for any unusual configurations.
Additional Resources
For further reading about Maven, its lifecycle, and how to build better Java projects, check out the official Apache Maven documentation. This resource is invaluable for developers at all stages of learning Maven.
A Final Look
Installing a local JAR file in a Maven project can be straightforward once you know the correct process. By following these outlined steps and understanding the purpose behind each command, you’ll be better equipped to handle local JAR installations efficiently. Maven is an incredibly powerful tool, and knowing how to leverage it for local dependencies can significantly enhance your Java development workflow.
Now that you have the knowledge, give it a try! With your Maven project set up and your local JAR installed, you can take full advantage of the functionalities offered by libraries even when they are not available in public repositories. Happy coding!