Why You Should Migrate from Nashorn to Modern JavaScript Engines
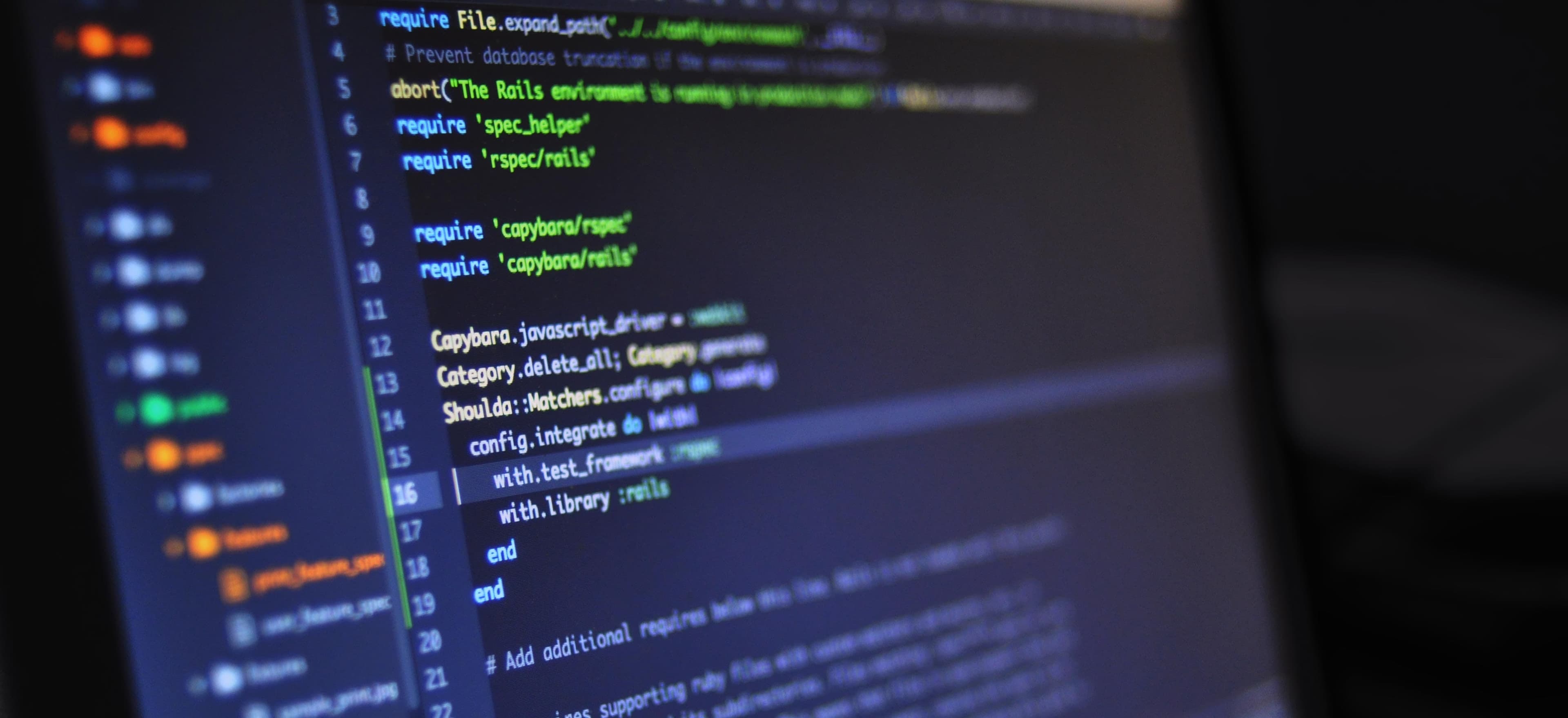
- Published on
Why You Should Migrate from Nashorn to Modern JavaScript Engines
Java developers often turn to JavaScript for various reasons, including integrating web applications or executing scripts. Historically, Nashorn was the go-to JavaScript engine in the Java ecosystem, embedded directly within the Java Virtual Machine (JVM). However, with technological advancements and the deprecation of Nashorn, it is crucial for developers to consider migrating to modern JavaScript engines.
In this blog post, we will explore the limitations of Nashorn, the benefits of modern JavaScript engines, and provide guidance on how to make this transition smoothly.
Understanding Nashorn
Nashorn was introduced in Java 8 as a lightweight JavaScript engine that allowed for easy integration of Java and JavaScript. However, it's important to note that Nashorn is deprecated since JDK 11 and removed in JDK 15. Its limitations include:
-
Performance Issues: Nashorn does not leverage the latest JavaScript engine optimizations. As performance is crucial in web applications, relying on an outdated engine may adversely impact an application's speed.
-
Limited JavaScript Features: Nashorn mainly supports older JavaScript standards. It does not support many modern ES6+ features like Promises, async/await, and destructuring, which are commonplace in current development.
-
Maintenance and Updates: Since Nashorn is no longer being actively maintained, using it can create risks, like security vulnerabilities and lack of official support.
Benefits of Migrating to Modern JavaScript Engines
With Nashorn becoming obsolete, migrating to a modern JavaScript engine offers several compelling benefits:
1. Performance Improvements
Modern engines like V8 (used in Chrome) and GraalVM provide substantial performance improvements. They implement Just-in-Time (JIT) compilation, optimizing code execution at runtime.
Here's a simple representation of how V8 compiles functions:
function factorial(n) {
if (n === 0) {
return 1;
}
return n * factorial(n - 1);
}
In this case, V8 dynamically compiles and optimizes the factorial
function at runtime, leading to faster execution times compared to Nashorn.
2. Full ES6+ Feature Support
Modern JavaScript engines support the ES6 standard and beyond. Features like arrow functions, template literals, and modules significantly enhance coding efficiency and readability.
Consider this example that shows the use of template literals:
const name = "Java Developer";
const greeting = `Hello, ${name}!`;
Modern engines fully support this syntax, while Nashorn may struggle or ignore it.
3. Active Community and Support
With engines like V8 and GraalVM receiving active and ongoing support, developers benefit from access to community forums, documentation, and frequent updates to address bugs and introduce new features.
If you're transitioning to GraalVM, their official documentation provides a thorough understanding of how to work with JavaScript in a modern context.
4. Knitr Integration
Tools like GraalVM offer the ability to run multiple languages, including Python, Ruby, R, and Java. This polyglot capability enables seamless integration of different programming paradigms, which was not feasible with Nashorn.
Suppose you want to execute JavaScript from within a Java application using GraalVM. An example snippet illustrates this:
import org.graalvm.polyglot.*;
public class JavaScriptExample {
public static void main(String[] args) {
try (Context context = Context.create()) {
context.eval("js", "var x = 10; var y = 20; x + y;");
System.out.println(context.eval("js", "x + y").asInt()); // Outputs: 30
}
}
}
This code demonstrates how to execute JavaScript code within a Java program easily.
5. Better Tooling and Ecosystem
Modern engines come with better debugging tools, libraries, and frameworks. When integrating JavaScript into your Java applications, popular libraries like React, Angular, or Vue.js can greatly enhance development.
Integrating a front-end framework into a Java ecosystem often leads to improved application maintainability and user experience.
Steps to Migrate from Nashorn to Modern JavaScript Engines
1. Assess Current Usage of Nashorn
Identify areas in your application where you are using Nashorn. Focus on the JavaScript features that might need to be rewritten or adjusted to conform to a modern engine.
2. Choose a New Engine
Pick a new JavaScript engine based on your project requirements. GraalVM integrated with your Java can be a great choice due to its compatibility and polyglot capabilities.
3. Rewrite JavaScript Code
As modern engines support newer JavaScript standards, you will likely need to rewrite the JavaScript code. Utilize the newly available features for cleaner and more efficient code.
4. Test Thoroughly
Once the migration is complete, conduct extensive testing. Ensure all functionalities work as expected. Pay close attention to performance metrics and watch for any discrepancies in application behavior.
5. Monitor and Optimize
After migration, continuously monitor the application. Leverage the profiling tools available within modern engines to optimize and enhance performance further.
Closing the Chapter
Migrating from Nashorn to modern JavaScript engines is not just a pressing need but an opportunity. Embracing newer technologies improves performance, enhances maintainability, and enables your applications to leverage contemporary programming practices.
If you're ready to make the switch, consider exploring GraalVM or V8. The resources and community support available surrounding these modern engines facilitate a smoother transition.
By migrating, you are not only future-proofing your applications but also equipping your development team with better tools and capabilities to tackle contemporary web development challenges.
For further insights on Java and JavaScript integration, consider checking out the Oracle Java Tutorials or Mozilla Developer Network.