Mastering Batch Deletion in Hibernate: Common Pitfalls Explained
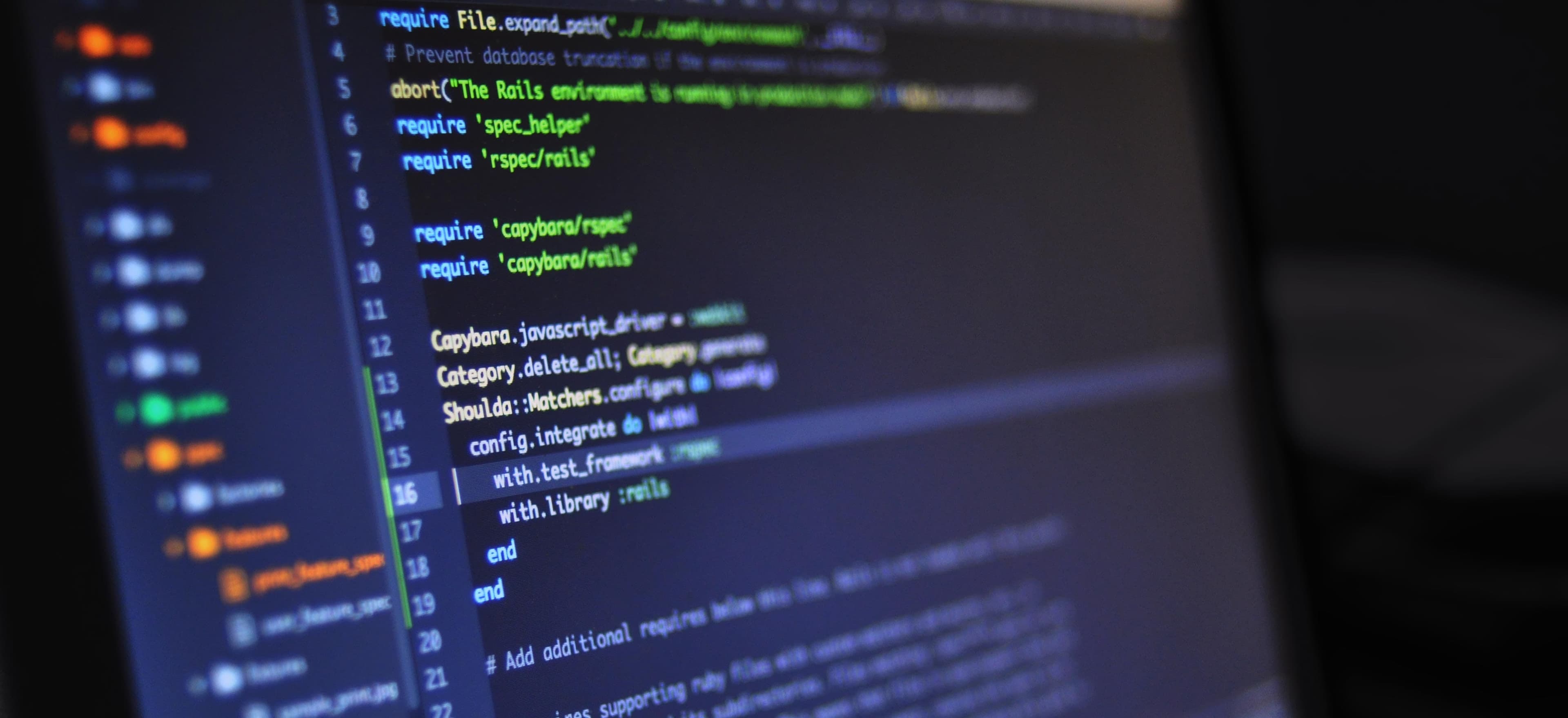
- Published on
Mastering Batch Deletion in Hibernate: Common Pitfalls Explained
Batch deletion is a critical feature within Hibernate that can significantly optimize your database operations. When you need to delete a large number of records, executing one query at a time can be inefficient, leading to costly performance issues. In this blog post, we will explore the nuances of batch deletion in Hibernate, common pitfalls to avoid, and practical coding examples. By the end of this post, you'll be able to confidently implement batch deletion while steering clear of common mistakes.
Understanding Hibernate's Batch Processing
Hibernate offers batch processing capabilities to optimize database operations, including inserts, updates, and deletions. By processing multiple records in a single transaction, you reduce the overhead of communication between your application and the database.
Why Choose Batch Deletion?
- Performance: Executing multiple delete statements in one go can greatly reduce database round trips.
- Resource Management: It conserves memory and resources, especially when dealing with large datasets.
- Transaction Control: It provides better transaction management as you can commit changes after a specified number of operations.
Enabling Batch Processing in Hibernate
Before diving into batch deletion, you must enable batch processing in your Hibernate configuration:
<property name="hibernate.jdbc.batch_size">50</property>
<property name="hibernate.order_inserts">true</property>
<property name="hibernate.order_updates">true</property>
These settings tell Hibernate to group insertions and updates into batches, improving performance. The hibernate.jdbc.batch_size
property specifies the number of operations in each batch.
The Basics of Batch Deletion in Hibernate
Batch deletion can be performed by either HQL (Hibernate Query Language) or Criteria API. Below is an example of batch deletion using HQL:
Session session = sessionFactory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
// HQL for batch deletion
String hql = "DELETE FROM EntityName WHERE criteria = :value";
Query query = session.createQuery(hql);
query.setParameter("value", someValue);
int result = query.executeUpdate();
System.out.println(result + " entities deleted.");
tx.commit();
} catch (Exception e) {
if (tx != null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
Commentary on the Code Snippet
In this example, we open a new session and start a transaction. We then construct an HQL DELETE statement, which deletes all entities matching specific criteria. After setting a parameter, we execute the query, which returns the number of deleted records.
Pitfalls to Avoid
While leveraging batch deletion, there are several common pitfalls that developers might encounter:
- Session Management: Not managing the session properly can lead to memory leaks. Always ensure sessions are closed, even in case of exceptions.
- Flush Mode: If the default flush mode is set to AUTO, Hibernate will flush the session before executing the batch deletion, which can degrade performance.
To mitigate the flush mode issue, you can explicitly set the flush mode like this:
session.setFlushMode(FlushMode.MANUAL);
// Your batch deletion logic
session.flush();
- Large Batches: While batching can improve performance, overly large batches might lead to memory issues, especially in environments with limited resources. A general rule of thumb is to batch around 50-100 operations.
Implementing Batch Deletion with Criteria API
You can also use the Criteria API to implement batch deletion. Here's how:
Session session = sessionFactory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
CriteriaBuilder builder = session.getCriteriaBuilder();
CriteriaDelete<EntityName> criteriaDelete = builder.createCriteriaDelete(EntityName.class);
Root<EntityName> root = criteriaDelete.from(EntityName.class);
criteriaDelete.where(builder.equal(root.get("criteriaField"), someValue));
int deletedCount = session.createQuery(criteriaDelete).executeUpdate();
System.out.println(deletedCount + " entities deleted.");
tx.commit();
} catch (Exception e) {
if (tx != null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
Commentary on the Criteria API Approach
In this snippet, we use the Criteria API to construct a delete operation. This approach enhances type safety and provides a programmatic way to build queries, which can help avoid errors due to typos.
Best Practices for Batch Deletion
- Transactional Control: Always wrap your batch deletion logic in a transaction.
- Regularly Flush: If you are performing multiple delete operations in a loop, flush after a certain number of deletes to keep memory usage in check.
- Testing: Test your batch deletion logic thoroughly to confirm that the desired records are deleted without side effects.
- Use Logging: Implement logging to monitor the number of records processed in each batch, which can help in diagnosing performance issues.
My Closing Thoughts on the Matter
Batch deletion is a powerful feature in Hibernate that can greatly enhance the efficiency of your data operations. By understanding the mechanics behind Hibernate's batch processing and avoiding the common pitfalls discussed above, you can master batch deletions and improve the performance of your applications significantly.
For more in-depth reading, consider referring to Hibernate Documentation and Spring Data JPA - Batch Processing.
As you continue to work with Hibernate, remember to apply these principles to ensure optimal performance, maintainability, and reliability in your applications. Happy coding!