Overcoming Common Pitfalls in Automated Functional Testing
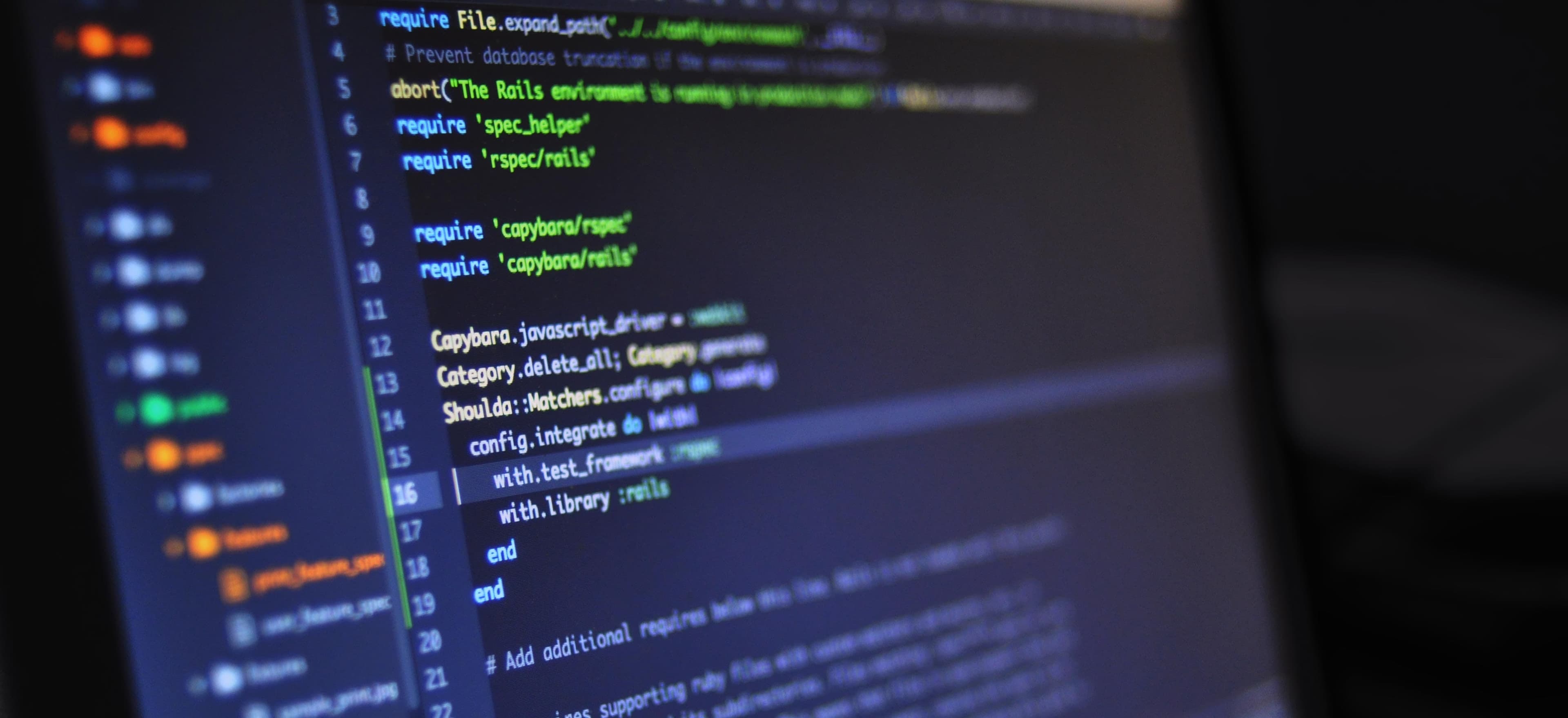
- Published on
Overcoming Common Pitfalls in Automated Functional Testing
Automated functional testing is a critical aspect of software development that enhances efficiency, reduces human error, and ensures high-quality outputs. However, many teams face common pitfalls that can hinder their testing processes. In this blog post, we'll explore these pitfalls, provide strategies to overcome them, and highlight best practices for successful automated functional testing.
What Is Automated Functional Testing?
Before diving into the pitfalls, let's define automated functional testing. This process involves using software tools to execute tests automatically on a software application to validate its functionalities against defined requirements. It helps in detecting bugs and verifying that the application behaves as expected.
Common Pitfalls and How to Avoid Them
1. Lack of Clear Objectives
Pitfall: One of the most prevalent issues in automated functional testing is not having well-defined goals. Without clarity, automated tests can become chaotic and unproductive.
Solution: Establish clear objectives for your testing efforts. Define what you want to achieve with your automated tests. Are you aiming to improve test coverage, reduce testing time, or enhance software quality? Each objective can significantly influence your test strategy.
// Example of defining clear test objectives
@Test
public void testLoginWithValidCredentials() {
// Objective: Verify that user can log in using valid credentials
String username = "user@example.com";
String password = "securePassword";
boolean result = login(username, password);
assertTrue("User should be logged in", result);
}
2. Overlooking Test Maintenance
Pitfall: Automated tests can quickly become outdated as the application evolves. Developers often overlook maintaining these tests, leading to increased fragility.
Solution: Implement a regular maintenance schedule for your automated tests. Revisit your test cases when there are changes in the application. Consider using a version control system to track updates and manage tests efficiently. This will reduce the likelihood of encountering false positives and negatives.
// Commented code outlines how to update tests when application changes
// This is a test for a feature we recently updated
@Test
public void testLoginButtonColor() {
// Ensure the color of the login button is correct after an update
String expectedColor = "blue"; // New color after UI update
String actualColor = loginButton.getColor();
assertEquals("Button color should match expected", expectedColor, actualColor);
}
3. Insufficient Test Coverage
Pitfall: Many teams fail to achieve adequate test coverage due to time constraints or lack of resources. Insufficient coverage can lead to missed bugs.
Solution: Use the current codebase to determine your test coverage, ideally aiming for 70-80% coverage of critical functionalities. Tools like JaCoCo can help you measure coverage effectively. Prioritize testing the most critical parts of your application first.
// Example to check for coverage
@Test
public void testCriticalFunction() {
// Testing critical functionality
String input = "important data";
String result = criticalFunction(input);
assertNotNull("Result should not be null", result);
}
4. Ignoring Test Data Management
Pitfall: Lack of attention to test data can lead to inconsistent results and unreliable test outcomes.
Solution: Establish a robust test data management strategy. Use mock data or data generators to create predictable test scenarios. Regularly clean your test data and ensure it aligns with the test cases.
// Example of creating mock data for tests
@Test
public void testWithMockData() {
MockData mockData = new MockData();
User testUser = mockData.createUser("test@example.com", "password123");
boolean result = login(testUser.getEmail(), testUser.getPassword());
assertTrue("User should be logged in", result);
}
5. Failure to Analyze Test Results
Pitfall: After running automated tests, teams may neglect to analyze the results thoroughly, thus failing to uncover underlying issues.
Solution: Integrate continuous feedback mechanisms into your testing process. Regularly review test results, identify patterns in failures, and address root causes. Utilize tools that provide detailed reporting and dashboard views for more insightful analysis.
// Pseudo-code to analyze test results
public void analyzeTestResults(TestResults results) {
for (TestCaseResult result : results) {
if (!result.isSuccessful()) {
logFailure(result);
notifyTeam(result);
}
}
}
6. Ignoring User Perspectives
Pitfall: Focusing solely on code functionality while ignoring user experiences can lead to a disconnect between what the software does and how users interact with it.
Solution: Incorporate user experience testing into your functional tests. Automate tests that simulate user interactions and capture a user's perspective. This will bridge the gap between technical validation and user expectations.
// Example simulating user interaction using Selenium
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/login");
driver.findElement(By.id("username")).sendKeys("testUser");
driver.findElement(By.id("password")).sendKeys("password");
driver.findElement(By.id("submit-button")).click();
String expectedWelcomeMessage = "Welcome back!";
String actualMessage = driver.findElement(By.id("welcome-msg")).getText();
assertEquals("User should see a welcome message", expectedWelcomeMessage, actualMessage);
7. Automating Everything
Pitfall: Not every test case should be automated. Attempting to automate all tests can lead to wasted resources and increased maintenance overhead.
Solution: Evaluate which tests are better suited for automation. Focus on repetitive tasks, tests that are stable, and those that require input from multiple environments. Manual testing may still be necessary for exploratory tests or those with complex user interactions.
The Closing Argument
Automated functional testing is essential for delivering high-quality software efficiently. However, avoiding common pitfalls ensures that your testing efforts yield the best results. By establishing clear objectives, maintaining your tests, ensuring adequate coverage, managing test data, analyzing results, incorporating user perspectives, and wisely choosing what to automate, you can significantly enhance your testing processes.
By implementing these strategies, you can not only avoid the pitfalls but also elevate the quality of your automated functional tests, leading to more reliable and user-friendly software. For more insights and continuous learning, consider checking out Martin Fowler's blog and Microsoft's Testing Documentation, both of which provide excellent resources for improving your testing practices.
Start your testing journey smartly today, and embrace automation with a clear, robust approach.
Checkout our other articles