How to Fix Thrashing in Task Submission with BlockingExecutor
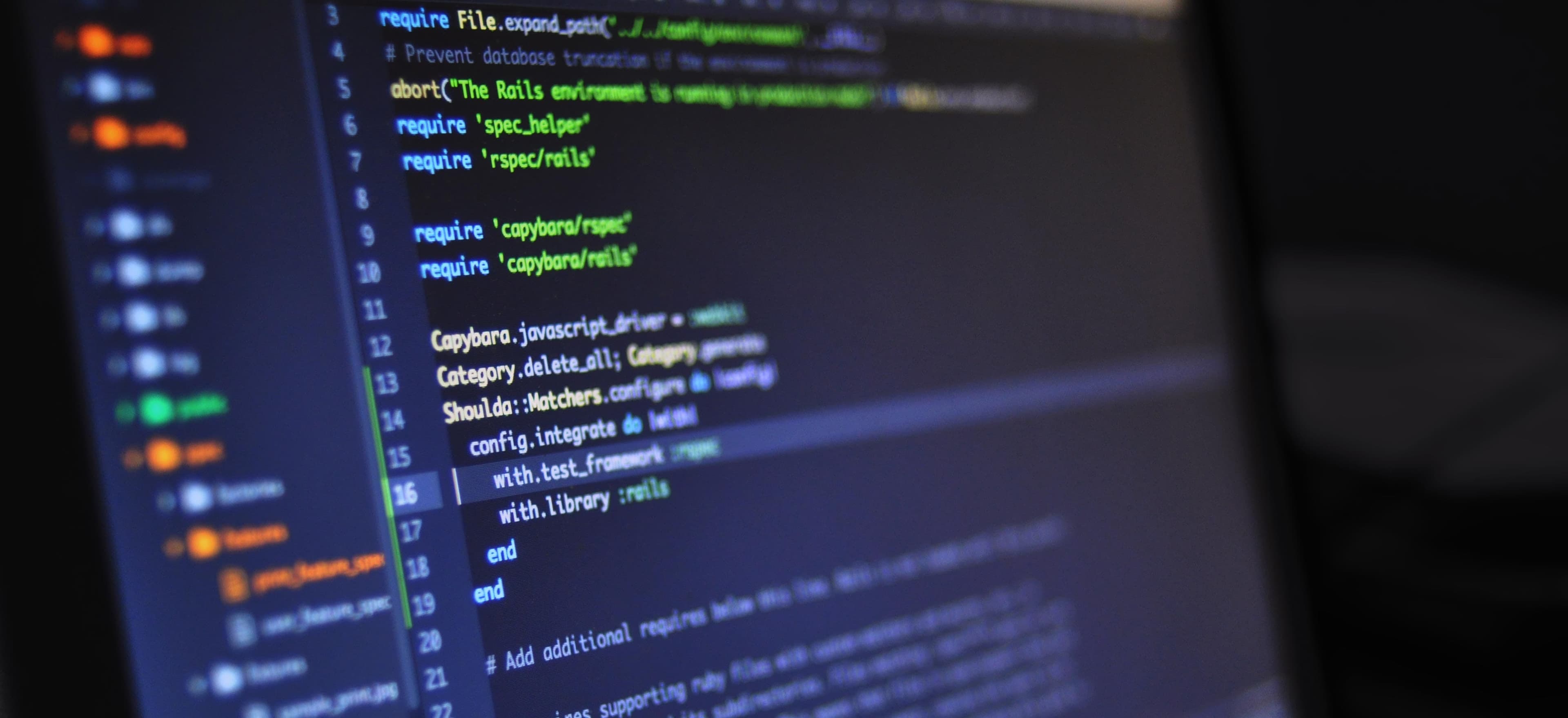
- Published on
How to Fix Thrashing in Task Submission with BlockingExecutor
In the world of concurrent programming, managing task execution effectively is vital. If you have used the java.util.concurrent
package, you may be familiar with the BlockingExecutor
. It offers an intuitive way to manage a pool of worker threads and efficiently submit tasks. However, if not configured properly, you may experience performance issues such as thrashing when tasks are submitted. This blog post will delve deep into the mechanics behind this issue and present actionable strategies to fix it.
What is Thrashing?
Thrashing occurs when a system spends more time managing tasks than executing them. This phenomenon can arise from excessive context switching between threads, leading to decreased system performance. In the context of Java's BlockingExecutor
, thrashing typically surfaces when there are too many tasks being submitted compared to the number of available worker threads.
Common Symptoms of Thrashing:
- High CPU Utilization: The CPU is busy but not effectively executing tasks.
- Increased Latency: Tasks take longer to complete than expected.
- Frequent Context Switching: A large number of threads competing for CPU time.
To address these issues effectively, we will explore how BlockingExecutor
operates, configurations, and practical code examples.
Understanding BlockingExecutor
The BlockingExecutor
is a part of the concurrent framework introduced in Java 5+. It is designed to handle the task submission in a blocking manner. Here’s a brief overview:
- Constructor: It initializes a fixed-size thread pool.
- Submit Method: It can either queue tasks until they are executed or reject them when the queue is full.
Despite its advantages, misconfiguration can lead to thrashing. Let's explore some common issues and their resolutions.
Identify Configuration Problems
When setting up your BlockingExecutor
, it's essential to identify potential configuration-related issues.
1. Insufficient Thread Pool Size
If the thread pool size is too small compared to the number of tasks you’re submitting, you'll quickly reach the limits of your executor.
Solution: Size the thread pool appropriately. To calculate an optimal thread pool size, consider factors such as CPU cores, task duration, and I/O wait.
Code Example:
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
public class OptimizedThreadPool {
private static final int THREAD_POOL_SIZE = Runtime.getRuntime().availableProcessors() * 2;
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(THREAD_POOL_SIZE);
// Submit tasks...
for (int i = 0; i < 100; i++) {
executor.submit(new Task(i));
}
executor.shutdown();
}
}
class Task implements Runnable {
private final int id;
Task(int id) {
this.id = id;
}
@Override
public void run() {
// Simulating task processing
System.out.println("Task " + id + " is running");
try {
Thread.sleep(1000); // Simulates workload
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
Why? We multiply the number of available processors by two because each core can handle multiple threads efficiently, particularly if tasks involve I/O operations or waiting.
2. Submitting Too Many Tasks
Submitting more tasks than can be processed concurrently can lead to filled queues and increased wait time, inducing thrashing.
Solution: Limit the number of submitted tasks or control the submission rate.
Code Example:
import java.util.concurrent.Executors;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
public class RateLimitedExecutor {
private static final int QUEUE_CAPACITY = 10;
private static final int THREAD_POOL_SIZE = 4;
public static void main(String[] args) {
BlockingQueue<Runnable> queue = new ArrayBlockingQueue<>(QUEUE_CAPACITY);
ExecutorService executor = Executors.newFixedThreadPool(THREAD_POOL_SIZE);
for (int i = 0; i < 100; i++) {
try {
queue.put(new Task(i)); // Blocks if the queue is full
executor.submit(queue.take()); // Take tasks from the queue
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
executor.shutdown();
}
}
Why? By using a bounded queue with a blocking mechanism, we can effectively throttle the submission of tasks and prevent overwhelming the executor.
Monitor Performance
After making adjustments to your executor configuration, it’s crucial to monitor the performance of your application.
Tools for Monitoring
- Java VisualVM: A powerful tool for monitoring Java applications with real-time data.
- JConsole: Useful for monitoring memory usage, thread count, and CPU time of each thread.
Utilize these tools to analyze CPU usage, threading behavior, and memory footprint during execution.
Analyze Thread Dumps
In case of performance bottlenecks, thread dumps can reveal the status of your threads at a specific point in time.
jstack <pid> > thread_dump.txt
Key Metrics to Observe
- Thread Count: Ensure threads are not being constantly created and destroyed.
- Queue Length: A lengthy queue could indicate an imbalanced load.
- Await Time: The time tasks spend waiting for execution.
Conclusion
Thrashing in BlockingExecutor
can severely impact application performance, but through careful configuration and monitoring, you can alleviate this issue. Remember to consider thread pool size, task submission rate, and actively monitor performance metrics. By understanding these key concepts and applying the suggested code changes, you will set your application on a course toward efficient concurrent execution.
For more detailed information on Executor frameworks, consider checking the official Java Documentation. Happy coding!
Checkout our other articles