Why Your Jenkins Pipeline Might Fail: Common Pitfalls
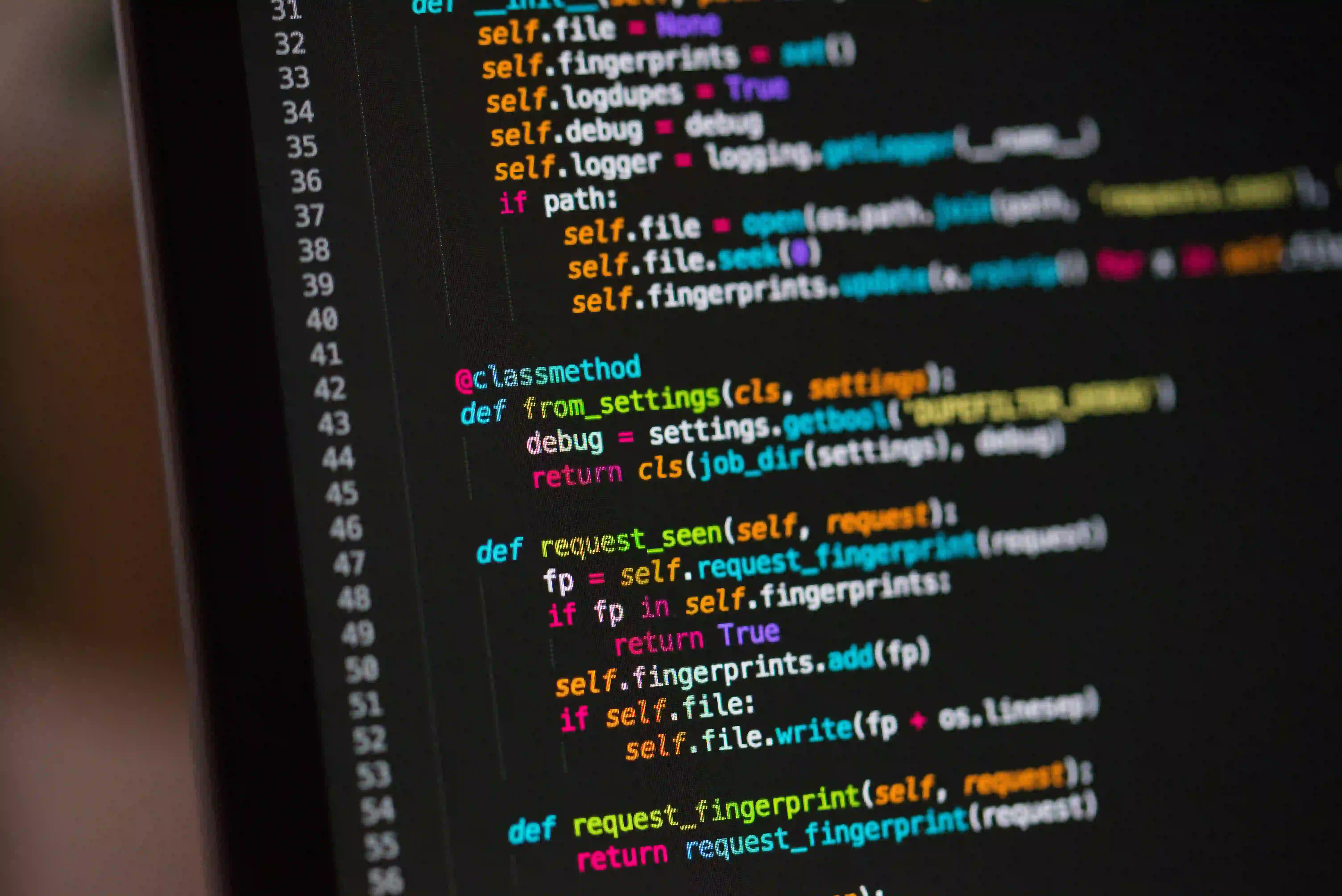
Why Your Jenkins Pipeline Might Fail: Common Pitfalls
Jenkins has become an essential tool in the software development lifecycle, particularly for Continuous Integration/Continuous Deployment (CI/CD). However, while Jenkins pipelines are powerful, they are not immune to failure. Understanding the common pitfalls can equip you to troubleshoot and optimize your pipelines effectively.
In this blog post, we will explore the reasons your Jenkins pipeline might fail, provide solid recommendations, and illustrate concepts with code snippets for better understanding.
Understanding Jenkins Pipelines
Before diving into potential failures, let’s briefly understand what Jenkins Pipelines are. A Jenkins Pipeline is an automated process that defines the steps needed to build, test, and deploy your application. It offers tremendous flexibility and can be defined using Declarative or Scripted syntax.
Example of a Declarative Pipeline
pipeline {
agent any
stages {
stage('Build') {
steps {
echo 'Building..'
sh 'make'
}
}
stage('Test') {
steps {
echo 'Testing..'
sh 'make test'
}
}
stage('Deploy') {
steps {
echo 'Deploying..'
sh 'make deploy'
}
}
}
}
In this example, you can clearly see the structure of a Declarative Pipeline. A defined agent allows Jenkins to allocate resources, while the stages define what needs to be done. Simple, right? Let’s now move on to the common pitfalls that can lead to pipeline failures.
Common Pitfalls in Jenkins Pipelines
1. Environment Issues
Problem:
One of the most frequent causes of Jenkins pipeline failures is mismatched environment configurations. This can be due to missing environment variables, inconsistent Node configurations, or lack of necessary permissions.
Solution:
Always ensure your environment is properly configured.
environment {
NODE_ENV = 'production'
DATABASE_URL = credentials('db-url')
}
In the above snippet, we’re defining environment variables for use within the pipeline. Properly setting these ensures smooth execution of various scripts that depend on them.
2. Faulty Build Scripts
Regardless of how meticulously you configure your Jenkins pipeline, a faulty build script can bring everything crashing down. This could be as simple as typo or syntax issues in your build tool (e.g., Maven, Gradle).
Solution:
Implement thorough code reviews and automated testing for your scripts.
3. Insufficient Resources
Problem:
Overloading your Jenkins instance can lead to insufficient resources for builds. This commonly happens in larger organizations where multiple projects run concurrently and consume resources.
Solution:
Consider scaling your Jenkins infrastructure or employing Jenkins Agents to distribute workloads effectively.
Code Example for Scaling
You can configure a Jenkinsfile to use different agents effectively:
pipeline {
agent { label 'linux' }
stages {
stage('Build') {
agent { label 'maven' }
steps {
echo 'Building with Maven..'
sh 'mvn clean install'
}
}
}
}
In the above example, the pipeline specifies that the build stage should specifically run on a Maven agent. Using dedicated agents can drastically minimize resource-related problems.
4. Hardcoding Values
Build scripts with hardcoded values can quickly lead to issues, especially when changes are made in your environment or configurations.
Solution:
Use parameters to avoid hardcoding and enable flexibility.
parameters {
string(name: 'BRANCH_NAME', defaultValue: 'main', description: 'Git branch to build')
}
pipeline {
agent any
stages {
stage('Checkout') {
steps {
checkout([$class: 'GitSCM', branches: [[name: "${params.BRANCH_NAME}"]]])
}
}
}
}
In this code snippet, the BRANCH_NAME
parameter allows the user to specify which branch of the code to checkout at runtime—this prevents the need for manual updates to the pipeline when code branches change.
5. Poor Error Handling
Often, Jenkins fails because it lacks a clear strategy for handling errors. A missed error can cause a complete halt, leaving developers puzzled.
Solution:
Incorporate structured error handling into your pipeline.
pipeline {
agent any
stages {
stage('Run Tests') {
steps {
script {
try {
sh 'make test'
} catch (Exception e) {
echo "Error occurred: ${e.message}"
currentBuild.result = 'FAILURE'
}
}
}
}
}
}
In this snippet, we utilize a try-catch
block to gracefully handle errors that occur during the test phase. This strategy ensures that you'll be informed of any issues while still maintaining the integrity of your pipeline execution flow.
6. Unmanaged Dependencies
Sometimes Jenkins pipelines will fail due to unmanaged dependencies lacking proper version control. This situation frequently arises in Node.js and Java projects.
Solution:
Strictly define your dependencies using a package manager or build tool.
// package.json example for a Node.js application
{
"dependencies": {
"express": "^4.17.1"
}
}
Using versioning in your project’s configuration ensures your pipeline can access the correct libraries and packages required for building successfully.
Final Thoughts
While Jenkins pipelines are incredibly versatile and powerful tools for CI/CD processes, they come with their own set of challenges. By understanding the common pitfalls—environment issues, faulty scripts, insufficient resources, hardcoding values, poor error handling, and unmanaged dependencies—you can implement best practices designed to mitigate failures.
Regularly reviewing your pipeline configurations, implementing strategies for error handling, and ensuring your resources are adequately scaled are vital steps to enhancing reliability.
By paying attention to these details, you set the groundwork for smoother builds, effective integrations, and robust deployments—providing a better experience for your development team and end-users alike.
For more in-depth guidance on Jenkins or CI/CD practices, explore Jenkins Official Documentation or consider diving deeper into DevOps practices. Happy coding!