Securing jBPM Workflow with Keycloak: Challenges Unveiled
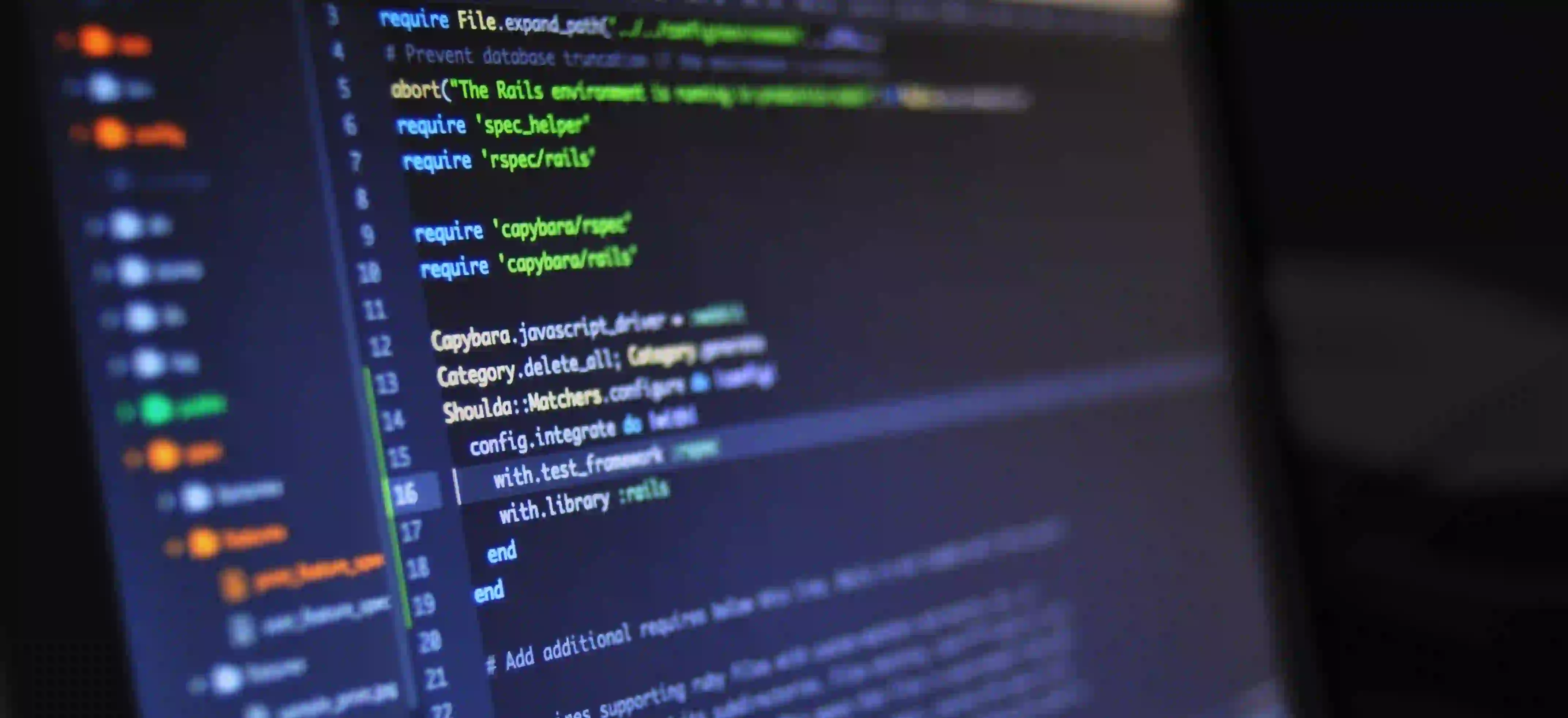
Securing jBPM Workflow with Keycloak: Challenges Unveiled
In the realm of business process management, jBPM (Java Business Process Model) stands out as a flexible and powerful workflow engine. However, as organizations increasingly rely on digital workflows, securing these processes becomes paramount. By integrating jBPM with Keycloak, an open-source Identity and Access Management (IAM) solution, businesses can strengthen their security posture. Yet, this integration does not come without its challenges. In this blog post, we will explore the complexities of securing jBPM workflows with Keycloak, offering insights, best practices, and code snippets to illuminate the path forward.
Why Choose jBPM and Keycloak?
Understanding jBPM
jBPM allows you to model, execute, and monitor business processes. It offers capabilities to integrate seamlessly with other systems, allowing for rich process execution. The underlying architecture is based on Java, which fosters a robust development environment.
What is Keycloak?
Keycloak simplifies authentication and authorization in a standard manner. As organizations transition to microservices and distributed architectures, Keycloak's ability to manage users, roles, and access control becomes crucial.
Integration Challenges
While integrating jBPM with Keycloak can enhance security, several challenges may arise:
-
Complex Configuration: Setting up Keycloak can be cumbersome. Proper configuration is necessary for effective integration.
-
Role Mapping: Defining and mapping roles correctly can become complex, especially in larger organizations.
-
Token Management: Handling security tokens efficiently is critical; improper management can expose workflows to vulnerabilities.
-
Performance Concerns: Overhead from security checks can impact workflow performance.
-
Error Handling: Properly managing authentication failures within workflows is crucial to ensure user experience.
Setting Up Keycloak
To begin, let's set up Keycloak and configure a realm for securing your jBPM workflows. Follow these steps:
Step 1: Installing Keycloak
You can use Docker for a quick setup. Here's a command to pull the Keycloak image and run it:
docker run -p 8080:8080 --name keycloak -e KEYCLOAK_USER=admin -e KEYCLOAK_PASSWORD=admin quay.io/keycloak/keycloak:latest start-dev
Step 2: Create a Realm
- Navigate to
http://localhost:8080/auth
and log in with the admin credentials. - Create a new realm for your jBPM applications.
Step 3: Create a Client for jBPM
- In the created realm, go to Clients > Create.
- Set the client ID (e.g.,
jbpm-client
) and selectopenid-connect
as the protocol.
Step 4: Configure Client Settings
- Ensure that Access Type is set to
confidential
. - Note down the Client Secret, which will be used by jBPM to authenticate with Keycloak.
Securing jBPM Workflows
With Keycloak set up, the next step is to secure your jBPM workflows. Below are some best practices for integration.
Configure jBPM to Use Keycloak
To authenticate with Keycloak, you will need to enable Security within your jBPM configuration. One approach is to implement a custom security manager.
public class KeycloakSecurityManager extends AbstractSecurityManager {
private Keycloak keycloak;
public KeycloakSecurityManager(Keycloak keycloak) {
this.keycloak = keycloak;
}
@Override
public boolean checkPermission(String username, String role) {
// Validate token with Keycloak
AccessToken token = keycloak.token(username);
return token.getRealmAccess().getRoles().contains(role);
}
}
Commentary on the Code Snippet
- The
KeycloakSecurityManager
extends an abstract class to provide a framework for handling security. AccessToken
is fetched through Keycloak, containing user role information, which is crucial for validating permissions against defined roles in your workflows.
Token Management
Implement a simple method for handling tokens between your jBPM engine and Keycloak:
public class TokenManager {
// Store the access token
private String accessToken;
// Method to refresh the token
public String refreshAccessToken() {
// Call Keycloak to refresh the token
accessToken = fetchNewToken();
return accessToken;
}
}
Why Manage Tokens This Way?
- By centralizing token management, you can help mitigate token expiration issues during workflow execution.
- Ensures that a valid token is consistently used throughout the jBPM process.
Role-Based Access Control (RBAC)
Establishing proper roles will streamline access control in your workflows. Define roles in Keycloak and match them with jBPM's workflow participants.
public class WorkflowRoleMapper {
public static String mapRoles(String keycloakRole) {
switch (keycloakRole) {
case "process-admin":
return "Admin";
case "process-user":
return "User";
default:
throw new IllegalArgumentException("Role not recognized");
}
}
}
Code Insight
- This mapping allows for a cleaner architecture as roles in jBPM can be abstracted from Keycloak's role definitions.
- This separation simplifies future changes and extensions to your role management logic.
Testing and Validation
Testing Integration
Perform integration tests by initiating workflows and observing security checks. Ensure that the following checkpoints are validated:
- Users with no roles cannot initiate workflows.
- Users with the appropriate role can complete the tasks.
- Verify session management by testing token expiration.
Error Handling
Always anticipate potential failures in authentication. You can handle errors gracefully to improve user experience:
public class WorkflowExecutor {
public void executeWorkflow(String workflowId, String username) {
try {
// Perform workflow execution logic
} catch (AuthorizationException e) {
// Log and handle authorization failure
System.out.println("Authorization failed for user: " + username);
}
}
}
In Conclusion, Here is What Matters
Integrating jBPM workflows with Keycloak can significantly bolster your security model. However, it's paramount to understand and navigate the associated challenges, including configuration, role mapping, token management, and performance.
By following best practices, leveraging code snippets for seamless integration, and performing adequate testing, you can secure your jBPM workflows effectively. For further reading on this topic, you can check the official jBPM documentation and the Keycloak documentation for deeper insights.
Remember, security is an ongoing process. Revisit your integration as new features are added and as your organization evolves. Embrace the complexities, and you will reap the benefits of a secure, efficient business process management solution.
If you found this post helpful or have any questions, don't hesitate to leave a comment below. Happy coding!