Navigating Service-to-Service Calls Across Anthos Clusters
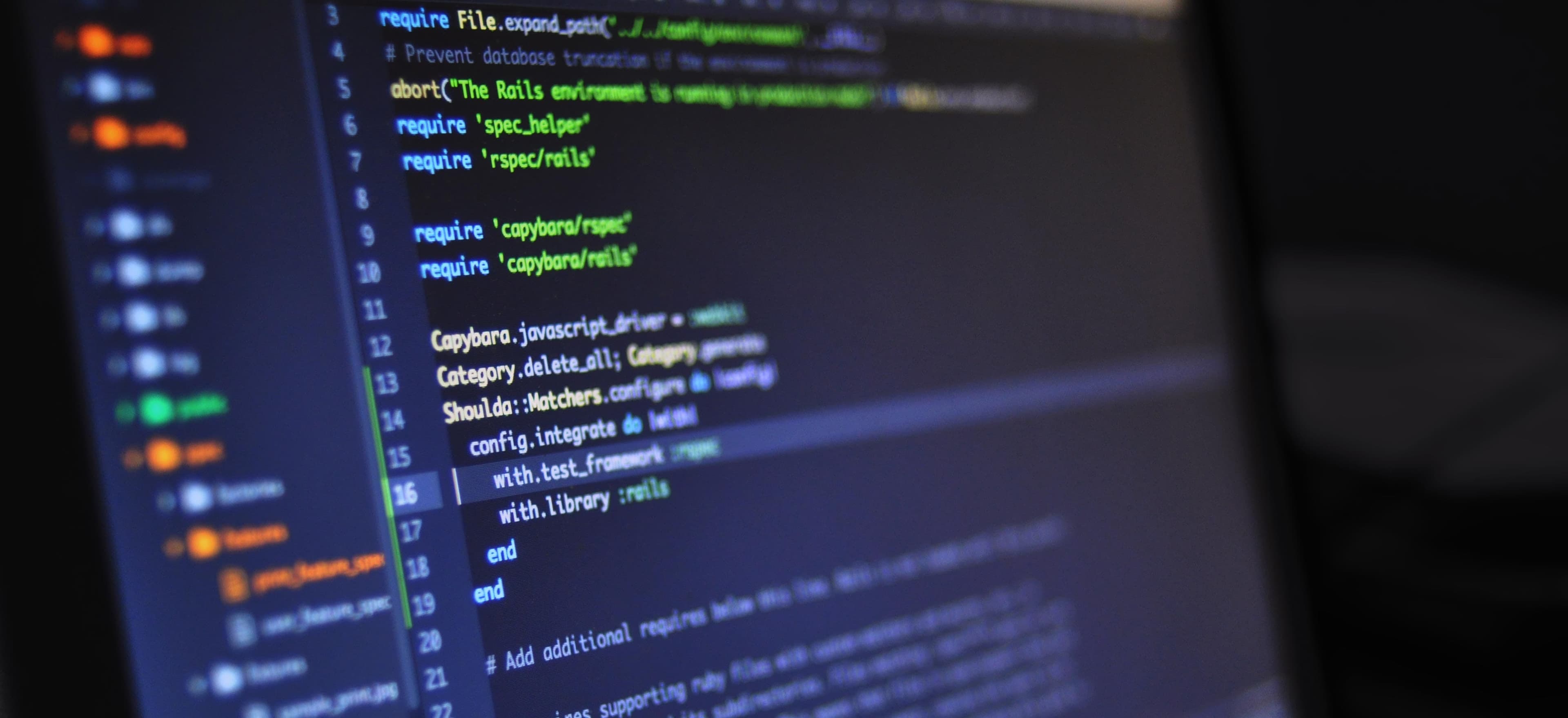
- Published on
Navigating Service-to-Service Calls Across Anthos Clusters
As enterprises increasingly adopt multi-cloud strategies, the need for efficient service-to-service communication across different environments becomes crucial. Google Cloud's Anthos is a powerful platform that allows users to manage applications on Kubernetes both on-premises and in the cloud. This blog post will explore how to navigate service-to-service calls across Anthos clusters, providing insights into best practices, configurations, and code snippets to facilitate this process.
Understanding Anthos and Its Architecture
Before diving into service calls, it is essential to grasp what Anthos is and how it operates. Anthos provides a unified management experience for applications running across various clusters, regardless of whether they reside on Google Cloud, on-premises, or across different cloud providers.
Key Features of Anthos
- Multi-cloud Capabilities: Run Kubernetes clusters anywhere, whether in Google Cloud, AWS, Azure, or on-premises.
- Service Management: Unified management of services across clusters through Anthos Service Mesh.
- Policy Enforcement: Implement policies consistently across clusters.
- Observability: Gain insights into application performance and health using integrated monitoring tools.
With these capabilities in mind, let's dive into the details of making service-to-service calls across Anthos clusters.
Setting Up Anthos Clusters
Requirements
To get started with service-to-service communication across Anthos clusters, ensure you have:
- At least two Anthos clusters set up (online and on-premises).
- Kubernetes and the required permissions to deploy and configure services.
Step-by-step Setup
-
Create Anthos Clusters: Follow the Anthos documentation to create your clusters. Ensure both are connected to your Google Cloud project.
-
Set Up Service Mesh: Anthos Service Mesh (ASM) facilitates traffic management between services. Deploy ASM with the following command:
gcloud anthos service-mesh install --project=YOUR_PROJECT_ID \ --cluster=YOUR_CLUSTER_NAME \ --cluster-location=YOUR_CLUSTER_LOCATION
This command installs Istio, which is critical for managing service-to-service communication.
Establishing Service-to-Service Communication
Once your clusters and service mesh are set up, you can begin establishing communication between services. The following sections will guide you through the components you need to configure.
Defining Services in Kubernetes
First, we need to create a few sample microservices in our clusters. Below is an example that defines two services: service-a
and service-b
.
Service A Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: service-a
labels:
app: service-a
spec:
replicas: 2
selector:
matchLabels:
app: service-a
template:
metadata:
labels:
app: service-a
spec:
containers:
- name: service-a
image: gcr.io/YOUR_PROJECT_ID/service-a:latest
ports:
- containerPort: 8080
Service A Service
apiVersion: v1
kind: Service
metadata:
name: service-a
spec:
ports:
- port: 8080
targetPort: 8080
selector:
app: service-a
Invoking Service B from Service A
Now, let's see how service-a
can communicate with service-b
. Here’s a streamlined implementation of the client-side function that sends a request to service-b
.
Service B Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: service-b
labels:
app: service-b
spec:
replicas: 2
selector:
matchLabels:
app: service-b
template:
metadata:
labels:
app: service-b
spec:
containers:
- name: service-b
image: gcr.io/YOUR_PROJECT_ID/service-b:latest
ports:
- containerPort: 8080
Invoking the Service
Here's how service-a
could call service-b
using Java:
import java.net.HttpURLConnection;
import java.net.URL;
public class ServiceAClient {
public void callServiceB() {
String serviceBURL = "http://service-b:8080/api"; // Service B's URL
try {
URL url = new URL(serviceBURL);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// Logic for processing response
System.out.println("Successfully called Service B!");
} else {
System.out.println("Failed to call Service B: " + responseCode);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why Service URLs Matter
In this code snippet, notice how the URL is defined. The format http://service-b:8080/api
uses the service name defined in Kubernetes. This is known as DNS resolution in Kubernetes, allowing services to communicate using these logical names instead of IP addresses.
Handling Cross-Cluster Communication
When services in different clusters need to interact, a few additional configurations come into play. Anthos enables cross-cluster service discovery, allowing services in one cluster to discover services in another.
Setting Up Cross-Cluster Communication
- Service Export and Import: Utilize service exports in one cluster and imports in another.
For instance, to export service-b
, you might use:
apiVersion: networking.istio.io/v1alpha3
kind: ServiceEntry
metadata:
name: service-b
spec:
hosts:
- service-b.global.svc.cluster.local
ports:
- number: 8080
name: http
protocol: HTTP
endpoints:
- address: <service-b's external IP>
- Importing the Service: In
service-a
, you would import that service using a similar configuration.
Security Considerations
Ensure that proper security measures are in place while making cross-cluster calls. Utilize Network Policies and Service Mesh features that come with Anthos to enforce restrictions and ensure secure communications.
Observability and Monitoring
Monitoring your service-to-service communication is crucial for maintaining the health of your applications. Anthos integrates with tools such as Cloud Monitoring and Cloud Trace to provide visibility into service meshes.
Enabling Monitoring
After setting up the service mesh, make sure to enable observability features in the Anthos dashboard. This will provide you with insights into request latency, errors, and service dependency mappings.
In Conclusion, Here is What Matters
Navigating service-to-service calls across Anthos clusters can be challenging, but with the proper approach and tools, it becomes manageable. Remember to set up your clusters correctly, leverage the service mesh for connectivity, and maintain best practices for security and observability.
For further details on Anthos and service mesh configurations, refer to the official Anthos documentation.
By adopting the strategies discussed in this blog, organizations can ensure robust and secure communication between services, paving the way for more scalable and efficient applications in a multi-cloud world.
Happy coding!