How Mocks Can Sabotage Your Need-Driven Development
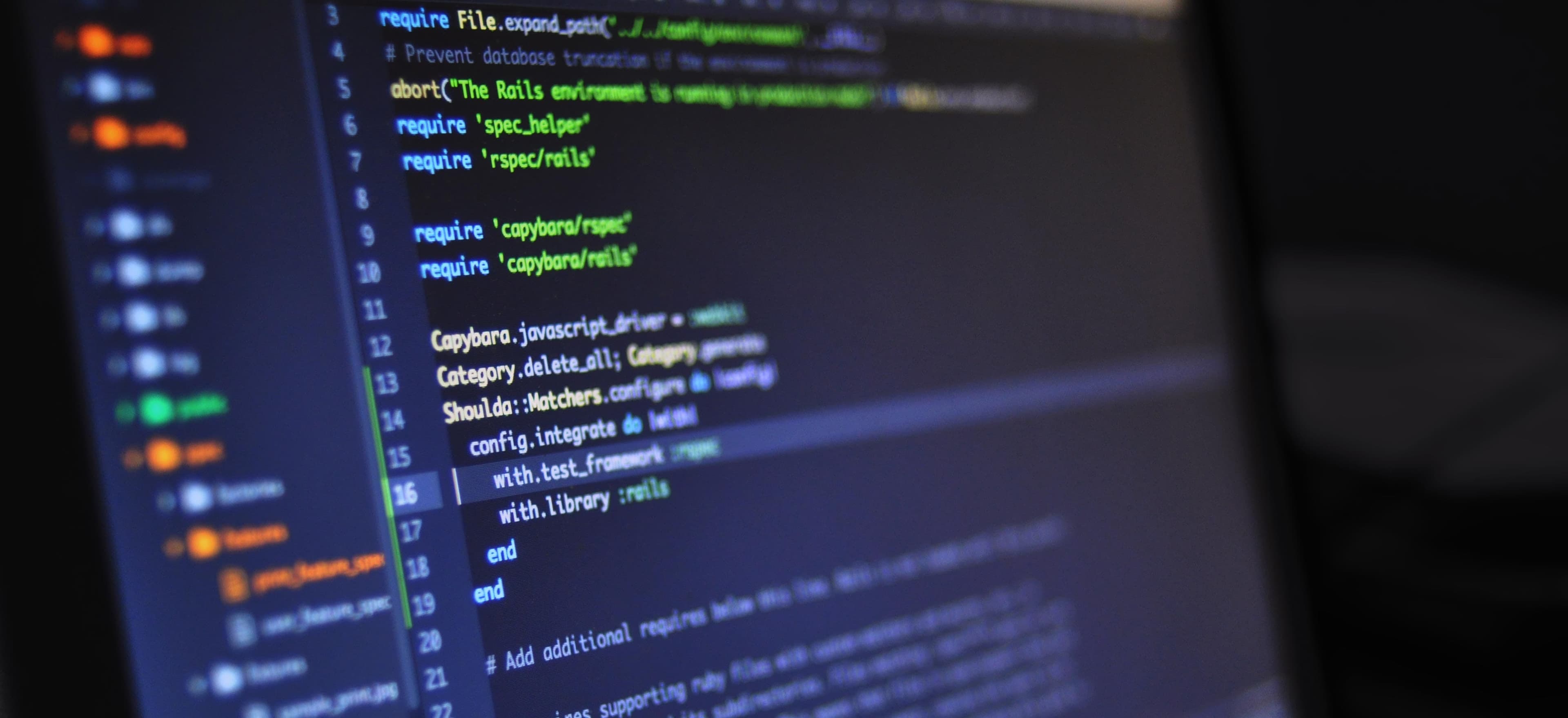
- Published on
How Mocks Can Sabotage Your Need-Driven Development
In the world of software development, we continually strive for more efficient, maintainable, and effective code. One prominent methodology that has emerged is Need-Driven Development (NDD). At its core, NDD focuses on delivering just what is needed—no more, no less. However, with the rise of mocking frameworks in the Java ecosystem, developers often find themselves trapped in a web of complications that can undermine this methodology. In this blog post, we will dive deeply into how mocks can inadvertently sabotage your need-driven approach, along with some practical insights into using them correctly.
Understanding Need-Driven Development
Before we delve into the implications of using mocks, let's clarify what Need-Driven Development entails. NDD emphasizes understanding the requirements before implementing a feature. It is about knowing what the end user truly needs, allowing you to design and develop software that is both relevant and efficient.
Benefits of NDD:
- Reduces Waste: You build only what you need.
- Enhances Collaboration: Consistent communication across stakeholders aligns expectations.
- Improves Quality: Development is focused on real user needs, reducing excess code which may introduce defects.
The Role of Mocks
Mocks, in the context of unit testing, are simulated objects that mimic the behavior of real objects in a controlled way. Mocks are often used when interacting with external systems, databases, or any dependencies that may introduce complexity if not controlled.
Example of a Mock in Java
Here’s a simple example using a popular mocking framework like Mockito:
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
class UserServiceTest {
@Test
void testGetUser() {
UserRepository mockRepo = mock(UserRepository.class);
when(mockRepo.findUserById(1)).thenReturn(new User(1, "Alice"));
UserService userService = new UserService(mockRepo);
User user = userService.getUser(1);
assertEquals("Alice", user.getName());
}
}
In the above code snippet:
- We define a mock of
UserRepository
to create a controlled test forUserService
. - The expectation is set using
when(...).thenReturn(...)
, so that whenfindUserById(1)
is called on the mock, it returns a predefined user.
The Dark Side of Mocks
While mocking can help isolate tests and improve efficiency, improper use can lead to several pitfalls that contradict the philosophy of NDD. Let's explore these caveats.
1. Over-Mocking
One of the most common mistakes is over-mocking, where developers create overly complex mock setups. This can lead to:
- Fragile Tests: If one small change occurs in the actual implementation, a plethora of mocks may break.
- Lack of Reality: Mocks only simulate behavior and often do not encapsulate the complexities of the real world.
For example, suppose you are testing a service with multiple dependencies that interact with one another. Mocking all these dependencies can create complex setups that merely cover positive scenarios
.
Best Practice: Limit the number of mocks to only those that are absolutely necessary. Embrace integration testing where feasible, as integration tests will involve real components working together.
2. Misleading Assumptions
Mocks may give you a false sense of confidence. It's easy to fall into the trap of believing that if your mocks pass the tests, your code is validated.
- Tests Don't Equate to Validations: A passing test does not guarantee that the real-world interaction will yield the same results. Risks become more evident when application complexity increases.
Take a look at this mock-based test that might pass:
@Test
void testExternalService() {
ExternalService mockExternal = mock(ExternalService.class);
when(mockExternal.getData()).thenReturn("Mock Data");
// Assuming APIClient uses this service
APIClient client = new APIClient(mockExternal);
assertEquals("Mock Data", client.fetchData());
}
In this situation:
- The test passes, but if the real
ExternalService
had issues querying a database or network hiccups, the test fails in the real world.
Best Practice: Utilize real service calls in scenarios the mock can't accurately simulate, and always complement unit tests with integration tests where feasible.
3. Focus on Testing Implementation Over Behavior
Mocks can oftentimes lead to focusing on the “how” rather than the “what”. For instance, developers may mock in an effort to validate implementation details instead of assessing the behavior expected from the application.
- Behavior-Driven Development (BDD) promotes defining the expected behavior of an application rather than how to achieve it. If you focus too heavily on mocks, you might distract yourself from fundamental checks.
Best Practice: Design your tests around user stories or features. Utilize tools like Cucumber or JBehave to align testing closely with expected behaviors.
The Last Word
While mocks in Java can be a powerful tool for unit testing, their improper application can undermine the principles of Need-Driven Development. Overuse can create a false sense of security and lead to brittle tests, while an overemphasis on implementation detail can distract from real user requirements.
In sum, remember to:
- Keep your mocking minimal and relevant.
- Validate assumptions with real interactions.
- Blend unit tests with integration tests.
- Focus on the behavior your software delivers, not just how it achieves that behavior.
By harnessing mocks judiciously, you can maintain your focus on user needs while ensuring your software remains robust and adaptable. For further reading on effective mocking strategies in Java, you may want to check Mockito’s official documentation or explore Behavior-Driven Development. Happy coding!