Mastering Multi-Level Grouping in Stream Processing
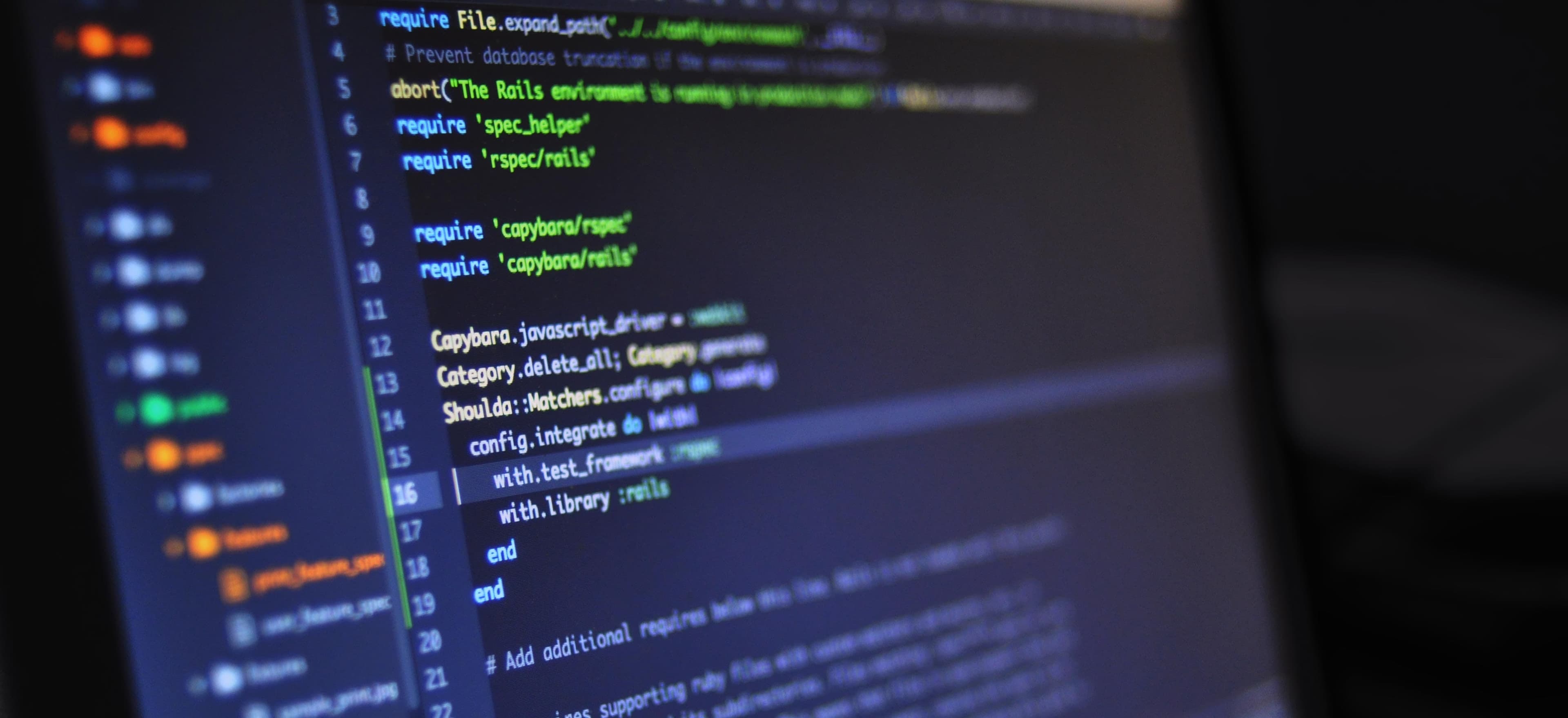
- Published on
Mastering Multi-Level Grouping in Stream Processing
In today's data-driven world, handling and processing data efficiently is crucial. Stream processing is an essential component of this landscape, enabling real-time data processing and analytics. In this post, we'll delve into the concept of multi-level grouping within stream processing, especially using Java's Stream API. We will explore how to implement this concept effectively, complete with code snippets and explanations to solidify your understanding.
Understanding Stream Processing
Stream processing allows for handling real-time data flows continuously. The Java Stream API simplifies this process by providing a powerful abstraction for processing sequences of elements.
To get started, it is crucial to familiarize yourself with some foundational concepts:
- Streams: Representing a sequence of elements supporting sequential and parallel aggregate operations.
- Operations: Typically categorized into intermediate (e.g., map, filter) and terminal (e.g., collect, forEach).
- Pipelines: A combination of various operations that can be executed together.
For more details, consider checking the Java Stream API documentation.
Multi-Level Grouping: An Overview
Multi-level grouping involves organizing data across several dimensions or levels. This approach can significantly enhance the data's visibility and enable insightful analysis. For instance, if you're processing sales data, you might want to group by region and then by product category within each region.
The Scenario
Let's consider a practical scenario where you have a list of sales transactions. Each transaction comprises a product name, category, region, and the amount sold. Our goal is to group these transactions firstly by region and then by product category to derive insights into sales performance.
Sample Data Structure
To illustrate our example, we'll create a Sale
class to represent our sales records.
public class Sale {
private String productName;
private String category;
private String region;
private double amount;
public Sale(String productName, String category, String region, double amount) {
this.productName = productName;
this.category = category;
this.region = region;
this.amount = amount;
}
// Getters
public String getProductName() { return productName; }
public String getCategory() { return category; }
public String getRegion() { return region; }
public double getAmount() { return amount; }
}
Creating Sample Data
Next, we'll create a list of sales transactions to work with.
import java.util.Arrays;
import java.util.List;
public class SalesData {
public static List<Sale> getSales() {
return Arrays.asList(
new Sale("Laptop", "Electronics", "North", 1200.00),
new Sale("Smartphone", "Electronics", "North", 800.00),
new Sale("Desktop", "Electronics", "South", 1500.00),
new Sale("Shoes", "Fashion", "North", 100.00),
new Sale("Jacket", "Fashion", "South", 200.00),
new Sale("Shirt", "Fashion", "South", 50.00)
);
}
}
Implementing Multi-Level Grouping
Using the Stream API, we can group the sales data by region and then by category. The method Collectors.groupingBy()
will serve as the primary tool for our implementation.
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class MultiLevelGroupingExample {
public static void main(String[] args) {
List<Sale> sales = SalesData.getSales();
// Multi-level grouping by region and then by category
Map<String, Map<String, List<Sale>>> groupedSales = sales.stream()
.collect(Collectors.groupingBy(Sale::getRegion,
Collectors.groupingBy(Sale::getCategory)));
// Displaying the grouped sales
groupedSales.forEach((region, categoryMap) -> {
System.out.println("Region: " + region);
categoryMap.forEach((category, salesList) -> {
System.out.println("\tCategory: " + category);
salesList.forEach(sale -> System.out.println("\t\tProduct: " + sale.getProductName() +
", Amount: " + sale.getAmount()));
});
});
}
}
Explanation of the Code
-
Stream Initiation:
sales.stream()
begins the stream processing of the sales data. -
First Grouping:
Collectors.groupingBy(Sale::getRegion)
groups the sales by theregion
property. -
Nested Grouping: The second
Collectors.groupingBy(Sale::getCategory)
groups the sales bycategory
within each region. -
Displaying Results: The nested
forEach
loops through the grouped data to display results neatly.
Output
If we run the above program, we would get an output similar to the following:
Region: North
Category: Electronics
Product: Laptop, Amount: 1200.0
Product: Smartphone, Amount: 800.0
Category: Fashion
Product: Shoes, Amount: 100.0
Region: South
Category: Electronics
Product: Desktop, Amount: 1500.0
Category: Fashion
Product: Jacket, Amount: 200.0
Product: Shirt, Amount: 50.0
Advantages of Multi-Level Grouping
- Enhanced Insights: Gaining insights at multiple levels allows businesses to identify trends and make data-driven decisions.
- Flexibility: Stream processing with Java allows for a variety of grouping strategies, enhancing adaptability.
- Simplicity: Leveraging the Java Stream API results in clean code, promoting maintainability and scalability.
The Last Word
Stream processing in Java has become increasingly indispensable, particularly when dealing with vast amounts of real-time data. By mastering multi-level grouping, developers can derive deeper insights from complex datasets, enhancing their applications' analytical capabilities.
By following the outlined steps and implementing multi-level grouping using Java's Stream API, you can handle and analyze data efficiently.
For further reading, check out this excellent resource on Java 8 Stream API to deepen your understanding of stream processing.
Now it's your turn to experiment. Consider extending the example by calculating total sales amounts per category or integrating other data sources. The possibilities are endless, and each exploration brings you closer to mastering multi-level grouping in stream processing. Happy coding!