Common Pitfalls in Java Bloom Filter Implementation
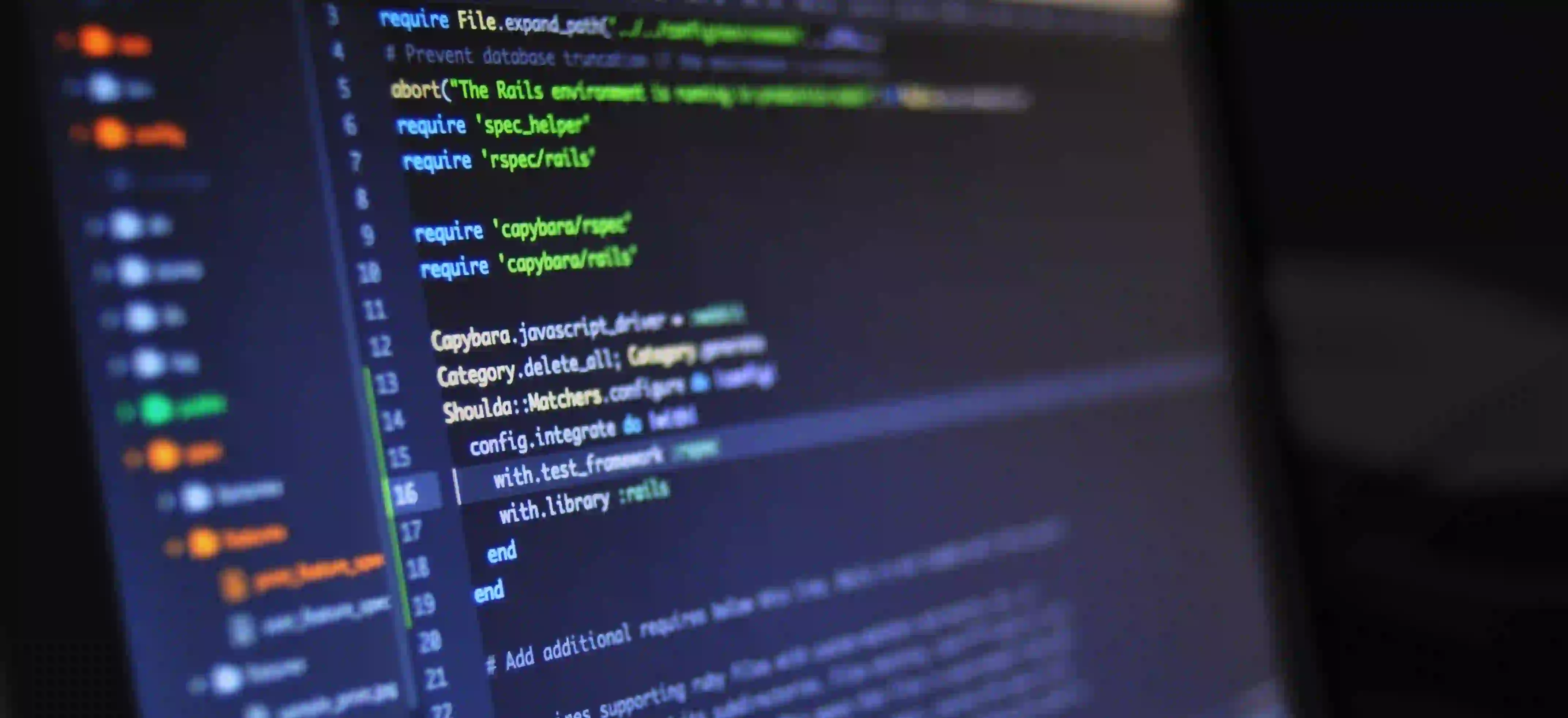
Common Pitfalls in Java Bloom Filter Implementation
Bloom filters are a space-efficient probabilistic data structure designed to test whether an element is a member of a set. They offer a significant memory advantage but come with some caveats, especially in their implementation. Whether you're implementing a Bloom filter for caching, database access, or network applications, understanding common pitfalls will help you create a robust solution.
In this blog post, we will explore the fundamental concepts of Bloom filters, best practices during implementation, and common pitfalls you should avoid. This guide will help you to not only implement Bloom filters effectively but also understand the rationale behind the design choices.
What is a Bloom Filter?
Before diving into the common pitfalls, let’s briefly review what a Bloom filter is. A Bloom filter uses multiple hash functions to manage a fixed-size bit vector.
Characteristics of a Bloom Filter
- Probabilistic Membership Test: It can tell you if an element is definitely not in a set or may be in it.
- False Positives: It can yield false positives, meaning it may indicate an element is present when it is not.
- Efficiency: It saves memory as it does not store actual elements.
Basic Implementation of a Bloom Filter
Here is a simple Java implementation of a Bloom filter:
import java.util.BitSet;
import java.util.List;
import java.util.ArrayList;
import java.util.Random;
public class BloomFilter {
private BitSet bitSet;
private int size; // Size of the bit array
private int numHashFunctions; // Number of hash functions
public BloomFilter(int size, int numHashFunctions) {
this.size = size;
this.numHashFunctions = numHashFunctions;
this.bitSet = new BitSet(size);
}
// Hash the data using different methods
private int hash(String value, int seed) {
Random hashFunction = new Random(value.hashCode() + seed);
return Math.abs(hashFunction.nextInt(size));
}
// Add elements to the Bloom filter
public void add(String value) {
for (int i = 0; i < numHashFunctions; i++) {
int hash = hash(value, i);
bitSet.set(hash);
}
}
// Check if an element might be present
public boolean mightContain(String value) {
for (int i = 0; i < numHashFunctions; i++) {
int hash = hash(value, i);
if (!bitSet.get(hash)) {
return false; // Definitely not present
}
}
return true; // Might be present
}
}
Explanation of the Code
- BitSet: We use Java's
BitSet
to manage our bits efficiently. - Hashing: The
hash
method generates hash values using a combination ofvalue.hashCode()
and a seed determined by the current index. - Adding Elements: In the
add
method, we calculate multiple hashes for the same value and set those bits totrue
. - Membership Testing: In
mightContain
, all relevant bits are checked to determine membership.
Now that we have an overview of how to implement a Bloom filter, let's discuss common pitfalls.
Common Pitfalls
1. Inappropriate Bit Array Size
When implementing a Bloom filter, selecting the right size of the bit array is crucial. If the array is too small, it will lead to many collisions, increasing the rate of false positives.
Solution: Use the formula for determining bit array size:
m = -(n * ln(p)) / (ln(2)^2)
Where:
m
is the bit array sizen
is the number of elements expected to be insertedp
is the desired false probability
2. Incorrect Number of Hash Functions
The choice of the number of hash functions (k) is equally important. Too few hash functions can lead to high false positive rates, while too many can introduce unnecessary complexity.
Formula:
k = (m / n) * ln(2)
This will help you calculate an optimal number of hash functions based on the expected size of your set.
3. Hash Function Quality
Using weak or poorly distributed hash functions can lead to clustering of bits. This increases the likelihood of false positives because multiple hash functions collide at the same bit.
Best Practice: Use cryptographic hash functions (like SHA-256) or well-distributed hash functions from libraries like Google Guava.
4. Ignoring Resizing
When the number of elements exceeds your expectations, it may be tempting to ignore the need for resizing. Once the Bloom filter hits its capacity, the false positive rate will increase exponentially.
Solution: Implement a resizing strategy. For example, you can create a new Bloom filter of optimal size and rehash the existing items when the current filter fills to a certain threshold.
5. Not Validating Input
Failing to validate the input being added to the Bloom filter can lead to unexpected behaviors or even exceptions. For instance, inputting null
could cause a NullPointerException
.
Recommendation: Always validate inputs before processing. Here’s how you might implement rudimentary input validation:
public void add(String value) {
if (value == null) {
throw new IllegalArgumentException("Cannot add null value");
}
// Rest of the method...
}
6. Lack of Concurrency Support
If your application is multi-threaded, a typical Bloom filter isn't thread-safe. Concurrent modifications can lead to inconsistent states or corrupted data.
Solution: Use synchronization mechanisms such as synchronized
blocks or implement ConcurrentHashMap
for thread-safe access. You can also consider leveraging Java's ConcurrentSkipListSet
.
7. Underestimating Memory Usage
While Bloom filters are space-efficient, they still consume memory. Failing to account for this can lead to performance degradation if your application starts paging to disk.
Best Practice: Monitor the memory footprint and ensure the Java heap size is appropriately configured using the -Xmx
and -Xms
flags.
In Conclusion, Here is What Matters
Creating a robust Bloom filter in Java requires careful consideration of various factors, from hash function quality to the sizing of the bit array. By avoiding the common pitfalls highlighted in this article, you can build an efficient Bloom filter suitable for your project’s needs.
Further Reading
By understanding these principles, you'll be well on your way to implementing an efficient and effective Bloom filter in your Java applications. Happy coding!