Handling Swallowed Exceptions in Java: A Crucial Guide
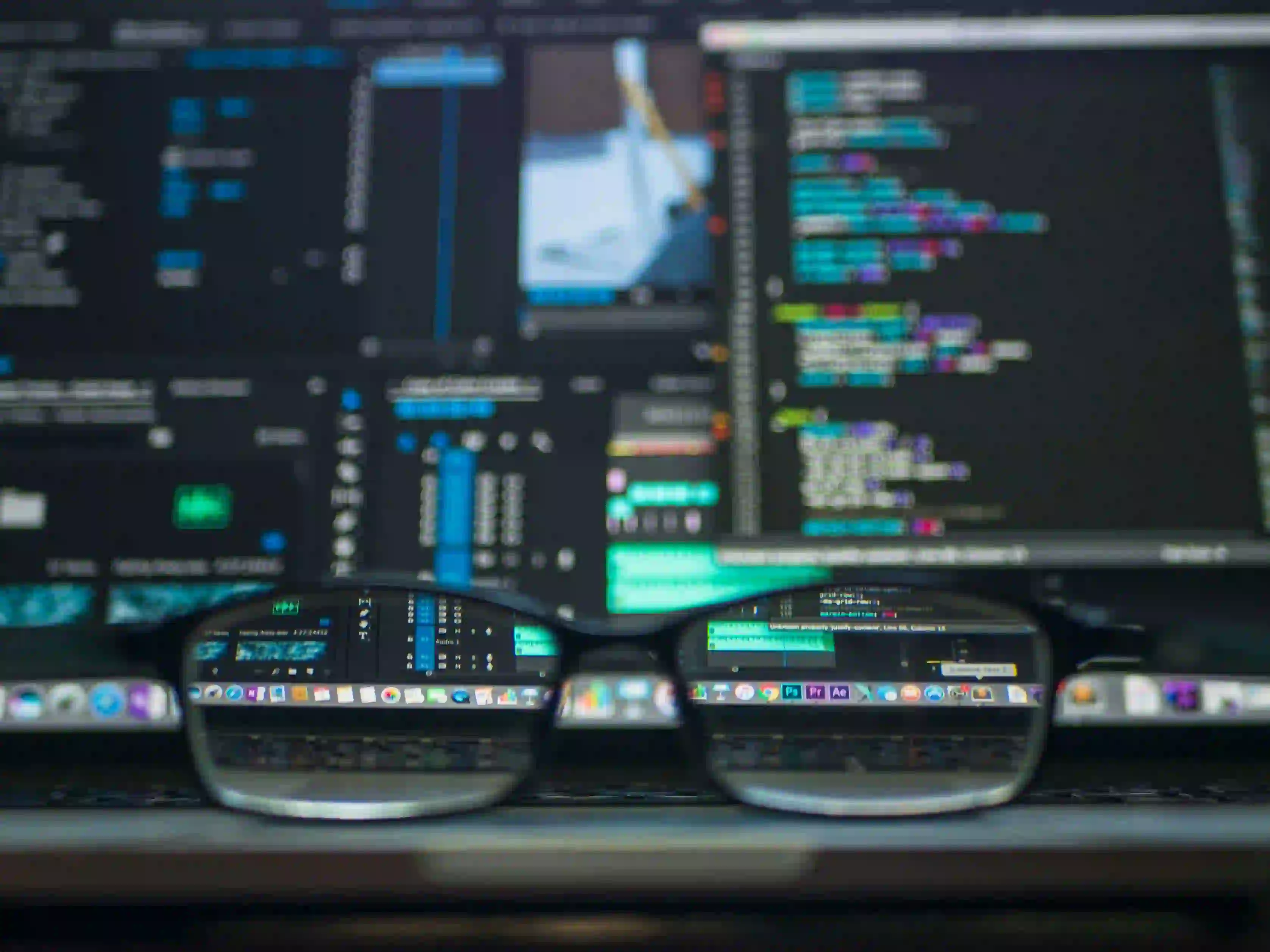
Handling Swallowed Exceptions in Java: A Crucial Guide
In the world of Java programming, exception handling is a critical component that determines the robustness and reliability of your applications. One particular issue that often goes unnoticed is “swallowed exceptions.” This term refers to situations where exceptions occur but are silently ignored or not properly handled, leading to unexpected program behavior or silent failures. In this guide, we will discuss the significance of managing swallowed exceptions, best practices, and how to ensure your code is resilient and maintainable.
What Are Swallowed Exceptions?
Swallowed exceptions occur when a developer catches an exception but does not take any further action, such as logging or re-throwing it. This can lead to difficult debugging sessions where the root cause of a problem is elusive due to the absence of relevant error logs. A swallowed exception might look like this:
try {
// Some code that may throw an exception
riskyMethod();
} catch (Exception e) {
// Exception is caught but not logged or handled
}
In this example, if riskyMethod()
throws an exception, it is caught, but without any logging or clear action taken. This can result in the loss of valuable information, making it harder to diagnose issues later on.
Why Is It Important to Handle Exceptions Properly?
Proper exception handling is vital for several reasons:
- Debugging: If exceptions are logged properly, they can provide guidance on what went wrong and where to fix it.
- User Experience: If an application fails silently, the user may encounter unexpected behavior without any notification, which can lead to frustration.
- Maintenance: Well-handled exceptions provide a clear understanding of the application flow, making it easier for future developers to comprehend the logic.
- Security: Swallowed exceptions can lead to security vulnerabilities as attackers may exploit the unhandled scenarios.
Best Practices for Exception Handling
Here are some best practices for handling exceptions properly in Java:
1. Always Log Exceptions
When you catch an exception, always log it. This can be done using popular logging frameworks like Log4j, SLF4J, or Java's built-in logging utilities. Logging provides visibility into what went wrong.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ExceptionHandlingExample {
private static final Logger logger = LoggerFactory.getLogger(ExceptionHandlingExample.class);
public void execute() {
try {
riskyMethod();
} catch (Exception e) {
logger.error("An error occurred: {}", e.getMessage());
}
}
}
In this snippet, we utilize SLF4J
to log the error message when riskyMethod()
fails, ensuring that important context is not lost.
2. Use Specific Exception Types
Instead of catching a generic Exception
, catch specific exceptions. This improves clarity and allows for tailored handling.
public void execute() {
try {
riskyMethod();
} catch (IOException e) {
logger.error("IO Exception occurred: {}", e.getMessage());
// Handle IO exception specifically
} catch (SQLException e) {
logger.error("SQL Exception occurred: {}", e.getMessage());
// Handle SQL exception specifically
} catch (Exception e) {
logger.error("An unexpected error occurred: {}", e.getMessage());
}
}
Here, different exceptions get their own handling logic, thereby making the code cleaner and easier to maintain.
3. Consider Rethrowing Exceptions
In some scenarios, it might be appropriate to rethrow the exception after logging it. This allows higher-level methods to address the issue if they have more context.
public void execute() throws CustomException {
try {
riskyMethod();
} catch (IOException e) {
logger.error("IO Exception occurred: {}", e.getMessage());
throw new CustomException("IO error in execute method", e);
}
}
In this example, we create a custom exception that wraps the original exception, retaining the stack trace and error context for debugging.
4. Utilize Finally Blocks
When managing resources such as file streams or database connections, leverage finally
blocks to ensure cleanup code always executes, even when exceptions occur.
public void readFile(String filePath) {
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader(filePath));
// Process the file
} catch (IOException e) {
logger.error("Error reading file: {}", e.getMessage());
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e) {
logger.error("Error closing reader: {}", e.getMessage());
}
}
}
}
In this method, regardless of whether an exception occurs, we ensure the reader
object is closed.
5. Avoid Empty Catch Blocks
It is a common mistake to leave catch blocks empty, as seen in our initial example. Instead, every exception caught should have appropriate handling.
try {
riskyMethod();
} catch (Exception e) {
// Do not omit handling!
logger.warn("Swallowed an exception: {}", e.getMessage());
}
This warning indicates that an exception was caught and, while it may not be critical, it still warrants attention.
The Optional
Class as an Alternative
As an alternative to traditional exception handling, Java 8 introduced the Optional
class. It may not completely replace exceptions, but it can be beneficial in certain scenarios, such as when dealing with operations that may return a null value.
Optional<String> findValue(String key) {
// Imagine fetching value, which may not exist
String value = someMethodThatMayReturnNull(key);
return Optional.ofNullable(value);
}
This pattern helps avoid NullPointerExceptions
and explicitly indicates that a return value may not be present.
To Wrap Things Up
Handling exceptions properly is a critical skill for any Java developer. By avoiding swallowed exceptions and ensuring that all exceptions are logged and adequately handled, you can significantly improve the reliability and maintainability of your applications. The practices discussed above are essential for preventing silent failures and making the debugging process a lot smoother.
For more in-depth information on exception handling in Java, consider exploring the Oracle Java Documentation or the in-depth discussion on Best Practices for Java Exception Handling.
By adhering to these guidelines, you will not only write cleaner code but also create applications that behave predictably and remain resilient in the face of errors. Happy coding!