Choosing Between Abstract Factory and Factory Method Patterns
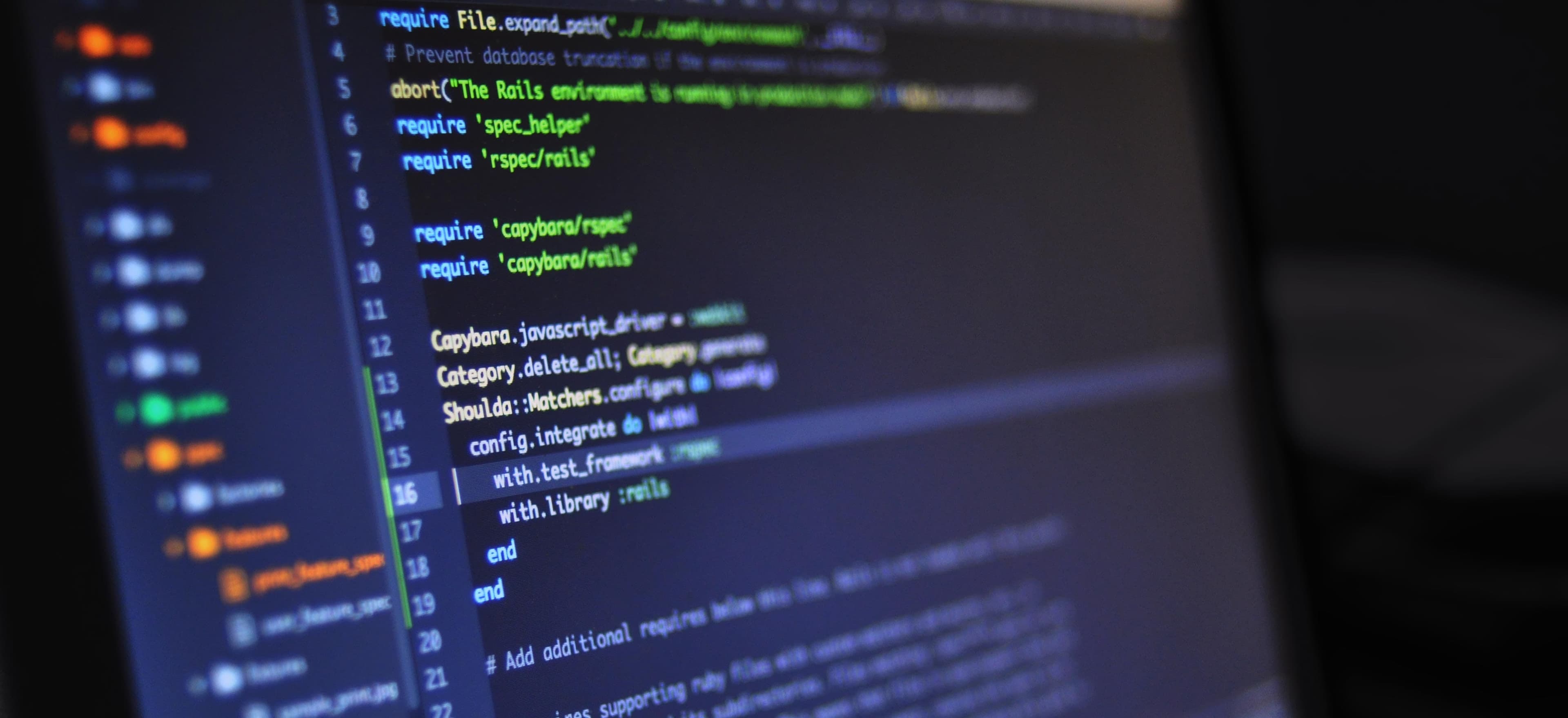
- Published on
Choosing Between Abstract Factory and Factory Method Patterns
In the world of software design, understanding different design patterns is crucial for creating efficient, maintainable, and scalable applications. Two of the most commonly used creational design patterns are the Factory Method and the Abstract Factory patterns. This article delves into the distinctions between these patterns, when to use them, and how they can improve your Java applications.
Understanding the Concepts
Factory Method Pattern
The Factory Method pattern defines an interface for creating an object, but allows subclasses to alter the type of objects that will be created. The method encapsulates the instantiation of objects and gives control to the subclasses to create the needed objects.
Key Characteristics:
- Involves a single method in a factory interface.
- Subclasses implement this method to create products.
- Often used when a class cannot anticipate the class of objects it must create.
Abstract Factory Pattern
On the other hand, the Abstract Factory pattern is a bit more complex. It provides an interface for creating families of related or dependent objects without specifying their concrete classes. Essentially, it allows you to create a suite of products that belong to a particular family.
Key Characteristics:
- Involves multiple factory methods.
- Creates a set of related products, providing flexibility and encapsulation.
- Often used in systems that need to be independent of how their products are created.
With these definitions in mind, let’s discuss when to use each of these patterns.
When to Use Each Pattern
Use Factory Method When:
- You have a single product family and are looking to extend the product types.
- You want to delegate the instantiation process to subclasses to promote loose coupling.
- You are working within a class hierarchy that makes it easier to create variations of products.
Use Abstract Factory When:
- You have multiple product families and want to ensure their products can be created without specifying the concrete classes.
- The product families are related and you need to enforce consistency among them.
- The system needs to be decoupled from the concrete classes of the products it uses.
Example Scenario in Java
To clarify these points further, let’s take a look at some Java code that showcases both patterns.
Factory Method Pattern Example
Let’s consider a scenario where you have a simple interface for shapes. The Shape
interface will have an implementation for Circle
and Square
.
// Shape.java
public interface Shape {
void draw();
}
// Circle.java
public class Circle implements Shape {
public void draw() {
System.out.println("Drawing a Circle");
}
}
// Square.java
public class Square implements Shape {
public void draw() {
System.out.println("Drawing a Square");
}
}
// ShapeFactory.java
public abstract class ShapeFactory {
public abstract Shape createShape();
}
// CircleFactory.java
public class CircleFactory extends ShapeFactory {
public Shape createShape() {
return new Circle();
}
}
// SquareFactory.java
public class SquareFactory extends ShapeFactory {
public Shape createShape() {
return new Square();
}
}
// Client.java
public class Client {
public static void main(String[] args) {
ShapeFactory factory = new CircleFactory(); // Change to SquareFactory to create a square
Shape shape = factory.createShape();
shape.draw(); // Output: Drawing a Circle
}
}
Commentary
In this example, the ShapeFactory
is our Factory Method. The concrete factories (CircleFactory
and SquareFactory
) decide the type of shape to create. This helps us keep the creation logic separate from the product logic.
Abstract Factory Pattern Example
Now, let's look at an example of the Abstract Factory pattern where we create a GUIFactory
to help create GUI components.
// Button.java
public interface Button {
void paint();
}
// WindowsButton.java
public class WindowsButton implements Button {
public void paint() {
System.out.println("Rendering a button in Windows style.");
}
}
// MacOSButton.java
public class MacOSButton implements Button {
public void paint() {
System.out.println("Rendering a button in MacOS style.");
}
}
// GUIFactory.java
public interface GUIFactory {
Button createButton();
}
// WindowsFactory.java
public class WindowsFactory implements GUIFactory {
public Button createButton() {
return new WindowsButton();
}
}
// MacOSFactory.java
public class MacOSFactory implements GUIFactory {
public Button createButton() {
return new MacOSButton();
}
}
// Client.java
public class Client {
private Button button;
public Client(GUIFactory factory) {
button = factory.createButton();
}
public void render() {
button.paint();
}
public static void main(String[] args) {
GUIFactory factory = new WindowsFactory(); // Change to MacOSFactory for macOS
Client client = new Client(factory);
client.render(); // Output: Rendering a button in Windows style.
}
}
Commentary
In this code, the GUIFactory
represents our Abstract Factory. It has a method createButton()
that subclasses (like WindowsFactory
and MacOSFactory
) implement to create specific button styles. This allows the Client
to remain agnostic to the concrete components that it uses, fostering flexibility.
Summary
In conclusion, both the Factory Method and Abstract Factory patterns serve essential roles in object creation, but they cater to different scenarios. The Factory Method is simpler and effectively encapsulates object creation for a single family of products. In contrast, the Abstract Factory shines when there’s a need to manage multiple related products.
Here’s a quick overview:
| Feature | Factory Method | Abstract Factory | |--------------------------|----------------|-------------------| | Number of products | Single | Multiple | | Complexity of structure | Simple | Complex | | Flexibility of design | Moderate | High |
It's important to choose the right pattern for your situation. Misapplying a pattern can lead to convoluted code that is hard to maintain. For more information on design patterns, check out Refactoring Guru or Java Design Patterns.
In summary, mastering these patterns will empower you to structure your Java applications more effectively. As always, understanding the context and requirements of your software will guide you toward the right choice.