Common Pitfalls When Handling Forms in Spring 3 MVC
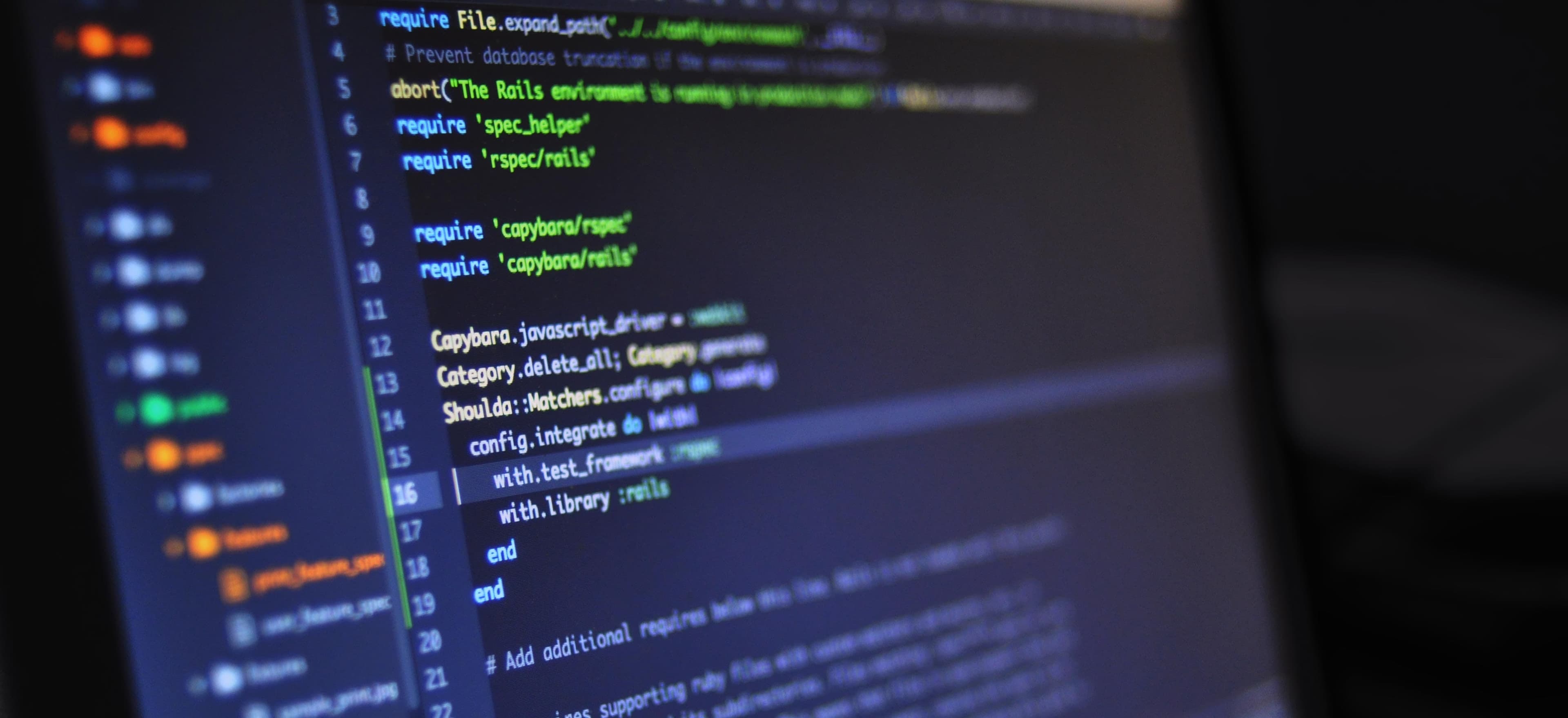
- Published on
Common Pitfalls When Handling Forms in Spring 3 MVC
Spring MVC simplifies web application development in Java, especially when managing user input through forms. However, developers frequently encounter pitfalls while handling forms in Spring 3 MVC. In this blog, we will discuss some common errors and best practices to ensure the efficient handling of forms in your web applications.
Understanding Spring 3 MVC Forms
Before diving into the pitfalls, it's essential to understand how Spring 3 MVC handles forms. The framework employs a model-view-controller (MVC) architecture which separates the application logic from the user interface.
Key Components in Spring MVC
- Models: Represent data in the application.
- Views: The user interface elements.
- Controllers: Handle user input and process it.
This setup allows developers to create responsive web applications with less coupling between components. Forms are typically handled by controllers that bind submitted data to model objects.
Common Pitfalls in Form Handling
1. Missing Data Binding
One of the most common mistakes is failing to bind form data to model objects. Spring uses data binding to map request parameters to Java objects. If not configured correctly, this can lead to incomplete or incorrect data being processed.
Solution:
Make sure your controllers correctly annotate their method parameters with @ModelAttribute
.
@Controller
public class UserController {
@RequestMapping(value = "/user", method = RequestMethod.POST)
public String submitUser(@ModelAttribute("user") User user, BindingResult result) {
if (result.hasErrors()) {
return "form";
}
// Save user to the database
...
return "redirect:/success";
}
}
In this code, we bind the incoming user data from the form to the User
model object. If any binding errors occur, they are handled via BindingResult
.
2. Ignoring Validation Errors
A common pitfall is not handling validation errors effectively. Users expect immediate feedback if they fill out forms incorrectly. Not providing this can lead to a poor user experience.
Solution:
Utilize Spring's validation framework and display errors on the form.
@InitBinder
protected void initBinder(WebDataBinder binder) {
binder.setValidator(new UserValidator());
}
@RequestMapping(value = "/user", method = RequestMethod.POST)
public String submitUser(@ModelAttribute("user") User user, BindingResult result) {
if (result.hasErrors()) {
return "form";
}
...
}
In this example, we have initialized a binder with a custom validator and are checking for errors in the submitUser
method. If errors exist, we return the user to the same form page, allowing them to correct their mistakes.
3. Not Using Redirect Attributes
Users often find themselves stuck on the same page after submitting forms, causing confusion. If you don't redirect after processing form data, users can inadvertently resubmit the form upon refreshing the page.
Solution:
Utilize RedirectAttributes
to redirect users after successful form submission.
@RequestMapping(value = "/user", method = RequestMethod.POST)
public String submitUser(@ModelAttribute("user") User user,
BindingResult result,
RedirectAttributes redirectAttributes) {
if (result.hasErrors()) {
return "form";
}
// Save user data to your database
redirectAttributes.addFlashAttribute("message", "User successfully created!");
return "redirect:/success";
}
With these changes, once the user’s data is correctly processed, we utilize redirectAttributes
to inform the user of their successful operation while redirecting them to another page.
4. Hardcoding Form Action URLs
Hardcoding URLs in your forms can lead to maintainability issues later. Changes in a single URL can cascade through multiple files, making refactoring cumbersome.
Solution:
Use Spring's URL rewriting capabilities.
<form action="${pageContext.request.contextPath}/user" method="post">
<!-- form fields -->
</form>
By using ${pageContext.request.contextPath}
, the action URL becomes dynamic. This way, changing the URL structure won't require updates across multiple files.
5. Not Securing Input Data
In a rush to implement features, developers sometimes neglect input validation and security measures. Forms are a common entry point for attacks like SQL injection and cross-site scripting (XSS).
Solution:
Implement comprehensive validation for all inputs and utilize Spring's built-in features.
@InitBinder
protected void initBinder(WebDataBinder binder) {
binder.setValidator(new UserValidator());
}
public void validate(Object target, Errors errors) {
User user = (User) target;
if (user.getUsername().length() < 5) {
errors.rejectValue("username", "error.username", "Username must be at least 5 characters long");
}
// Additional validation checks
}
Use the validator to sanitize and validate user input thoroughly. Also, ensure the application is built with XSS protection and input sanitization measures.
6. Failing to Pre-populate Form Fields
When a validation error occurs, it's common to forget to repopulate form fields with previously entered data. This leads to frustration among users.
Solution:
By returning the model object to the form without resetting it, Spring knows to populate form fields with existing values.
@RequestMapping(value = "/user", method = RequestMethod.GET)
public String showForm(Model model) {
model.addAttribute("user", new User());
return "form";
}
In case of errors, the existing user object will maintain the state, allowing the user to see their previous input.
In Conclusion, Here is What Matters
Handling forms in Spring 3 MVC can be straightforward once you are aware of the common pitfalls. With attention to detail in data binding, error handling, redirection, security, and user experience, you can create a robust web application.
Further Learning
For more in-depth understanding, check out:
- Spring MVC Reference Documentation
- Java Validation Framework
By employing the strategies discussed above, you will enhance both the functionality and user experience of your Spring MVC applications. Happy coding!
Checkout our other articles