Struggling with Custom JVM Metrics? Here's the Fix!
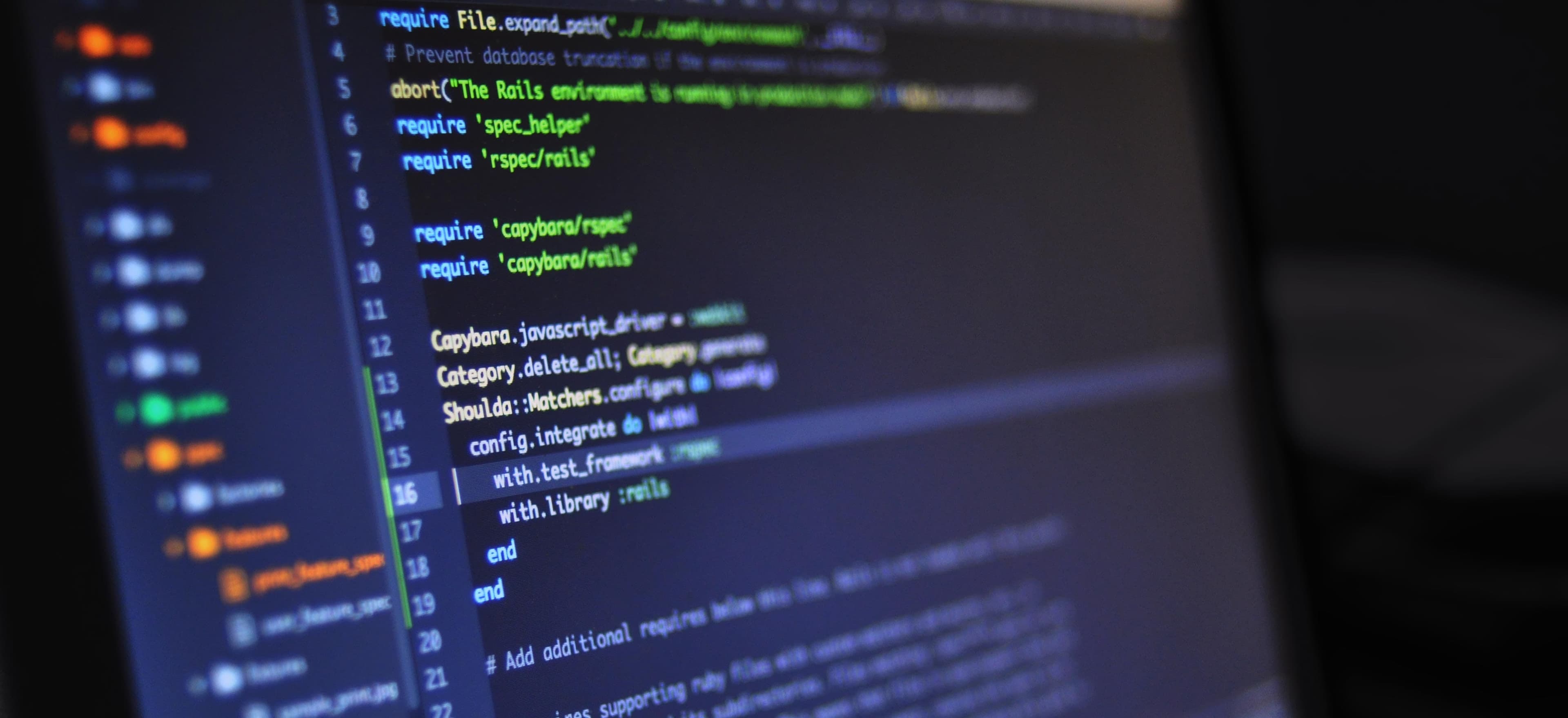
- Published on
Struggling with Custom JVM Metrics? Here's the Fix!
Java is a powerful language that powers a multitude of applications across various industries. One of its essential components is the Java Virtual Machine (JVM), which manages resources, executes bytecode, and provides several built-in metrics for monitoring application performance. However, there are times when the default JVM metrics do not provide enough insights. This is where custom JVM metrics come into play.
In this blog post, we will explore how you can create custom JVM metrics effectively, using tools like Micrometer and Prometheus. You'll see examples of code snippets that illustrate why certain practices are essential, tips on best practices, and how to visualize these metrics. Let's dive in!
Why Custom JVM Metrics Matter
Custom JVM metrics allow you to track specific application behaviors and resource usage patterns that are relevant to your business logic. Default metrics may be too generic and not reflect the application's unique needs. By adding custom metrics, you can:
- Improve Performance Monitoring: Track metrics that directly impact application performance.
- Enhance Troubleshooting: Quickly identify performance bottlenecks and bugs.
- Understand Usage Patterns: Gain insights into the application's behavior in production.
- Optimize Resource Usage: Manage memory and CPU usage effectively.
Setting Up Micrometer with Spring Boot
First, we need to set up a Spring Boot application with Micrometer. Micrometer is a metrics facade that supports various monitoring systems, including Prometheus, Graphite, and more.
Step 1: Add Dependencies
To begin, include the necessary dependencies in your pom.xml
file if you are using Maven.
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-core</artifactId>
</dependency>
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
These dependencies will ensure that you have access to Micrometer's core functionalities as well as the Prometheus registry integration.
Step 2: Create Custom Metrics
Now, let's create a simple service where we will add a custom metric.
import io.micrometer.core.instrument.Counter;
import io.micrometer.core.instrument.MeterRegistry;
import org.springframework.stereotype.Service;
@Service
public class UserService {
private final Counter userRegistrationCounter;
public UserService(MeterRegistry meterRegistry) {
// Creating a custom counter for user registrations
this.userRegistrationCounter = Counter.builder("user.registration.count")
.description("Counts the number of user registrations")
.register(meterRegistry);
}
public void registerUser(String username) {
// Logic to register user goes here
System.out.println("Registering user: " + username);
// Increment the counter every time a user registers
userRegistrationCounter.increment();
}
}
Code Explanation
- Creating a Counter: We employed the
Counter.builder
method to create a custom counter metric to track user registration counts. - Object Instantiation: The
Counter
is registered in theMeterRegistry
, allowing it to be reported to Prometheus or other monitoring solutions.
This setup allows you to capture metrics specific to your user registration logic.
Exposing Metrics for Prometheus
Since you are likely to use Prometheus for monitoring, ensure that your application exposes metrics at a specific endpoint. Update your application.properties
:
management.endpoints.web.exposure.include=health,info,prometheus
management.endpoint.prometheus.enabled=true
These configurations allow Prometheus to scrape your metrics from the /actuator/prometheus
endpoint.
How to Visualize Custom Metrics in Prometheus
After configuring the metrics in your Spring Boot application, the next step is to visualize the data in Prometheus. Here’s how you set it up:
Step 1: Set Up Prometheus
Create a prometheus.yml
configuration file:
global:
scrape_interval: 15s
scrape_configs:
- job_name: 'spring-boot-app'
metrics_path: '/actuator/prometheus'
static_configs:
- targets: ['localhost:8080']
Step 2: Start Prometheus
Run Prometheus with the following command:
prometheus --config.file=prometheus.yml
You can access the Prometheus dashboard at http://localhost:9090
.
Step 3: Querying Metrics
To view your custom metric, use the following query in the Prometheus dashboard:
user_registration_count
This query will return the total number of user registrations tracked by your application. You can create graphs and alerts based on these custom metrics to monitor application health effectively.
Best Practices for Custom JVM Metrics
Creating custom JVM metrics is not just about implementation; it's also about doing it right. Here are some best practices:
- Keep it Simple: Don't overcrowd metrics. Focus on the most impactful metrics that align with your business objectives.
- Immutable Counters: Use counters for metrics that only increment over time. Avoid any operations that can decrement them, which can lead to confusion.
- Use Tags Wisely: Tags can help you differentiate metrics. However, guard against creating too many unique combinations of tags, as this can lead to high cardinality and performance issues.
- Leverage Histograms for Performance: When measuring response times, consider using a histogram to capture distribution rather than a single average. This gives a more accurate performance picture.
The Bottom Line
Custom JVM metrics can significantly enhance your monitoring capabilities, providing essential insights into your application's behavior. With tools like Micrometer and Prometheus, you can easily create and expose these metrics. Remember, the key to effective monitoring lies in simplicity, relevance, and clarity.
By following the steps and best practices outlined in this post, you will be better equipped to handle any performance issues and build more resilient Java applications.
For more reading on this topic, consider checking out the following resources:
Feel free to leave your thoughts or questions in the comments below. Happy coding!