Securing Your JWT Tokens: The Importance of Secret Rotation
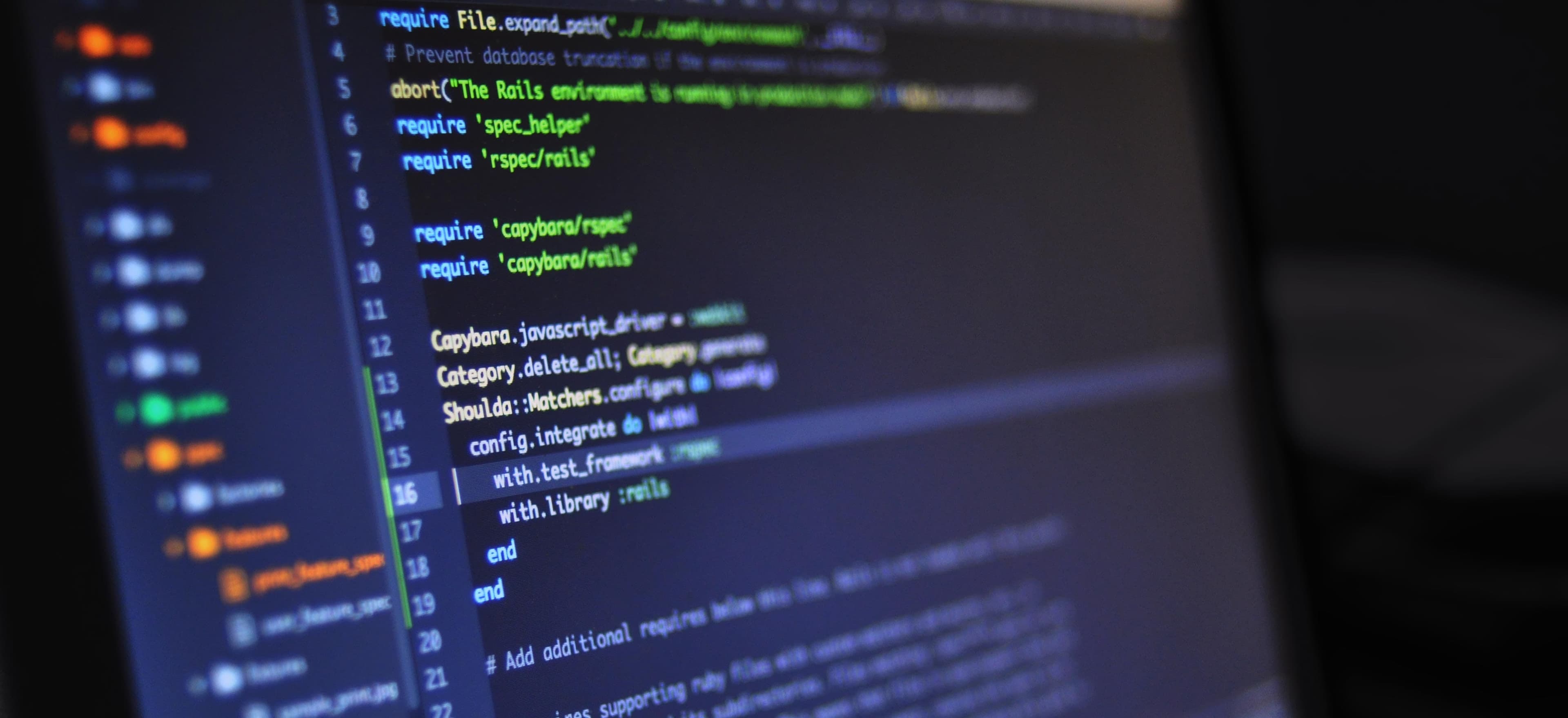
- Published on
Securing Your JWT Tokens: The Importance of Secret Rotation
In the modern web development landscape, JSON Web Tokens (JWT) play a fundamental role in securing applications. They are widely used for authentication and information exchange. However, with their growing adoption comes significant responsibility, particularly regarding security best practices. One of the most critical practices is secret rotation. In this post, we'll dive deep into the importance of secret rotation, how to implement it within your Java applications, and why it matters.
Understanding JSON Web Tokens
Before diving into secret rotation, it's essential to understand JWTs. A JWT is a compact, URL-safe means of representing claims to be transferred between two parties. The token consists of three parts: header, payload, and signature.
JWT Structure
- Header: Typically consists of two parts: the type of token (JWT) and the signing algorithm being used, such as HMAC SHA256.
- Payload: Contains the claims. Claims are statements about an entity (typically, the user) and additional data.
- Signature: The signature is calculated using the encoded header, the encoded payload, a secret, and the algorithm specified in the header.
Here's an example of a JWT structure:
eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiaWF0IjoxNTE2MjM5MDIyfQ.SflKxwRJSMeKKF2QT4fwpMeJf36POk6yJV_adQssw5c
The Role of Secrets in JWTs
JWTs rely on secrets for their integrity. When a JWT is signed using a secret key, any tampering will result in an invalid token, preventing unauthorized access. However, if that secret is compromised, the security of your application is at risk.
Why Rotate Your Secrets?
- Mitigate Impact of Compromise: If a secret is compromised, rotating it ensures that the attacker cannot use it indefinitely.
- Regulatory Compliance: Many industries mandate certain security protocols, including regular secret changes.
- Enhanced Trust: Regularly rotating your secrets demonstrates a commitment to security, which can enhance trust with your users.
Implementing Secret Rotation in Java
Let's now explore how to implement secret rotation in a Java application. We will leverage Spring Boot to create a secure JWT-based authentication system with secret rotation.
Step 1: Setting Up Your Spring Boot Application
First, you need a Spring Boot project. You can initialize it using Spring Initializr and include dependencies for Spring Web
, Spring Security
, and jjwt
(Java JWT).
Step 2: Create a Properties File
In your application.properties file, define your JWT secret. Instead of hardcoding it, you can manage it through environment variables or a secrets manager.
jwt.secret=YourInitialSecretKey
jwt.expiration=3600000
It’s crucial to load the secret dynamically, allowing for an easy update during rotation.
Step 3: Generate and Validate JWT
Here's a simple utility for generating and validating JWT tokens.
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
public class JwtUtil {
private String secret = "YourInitialSecretKey";
private long expiration = 3600000; // 1 hour
public String generateToken(String username) {
Map<String, Object> claims = new HashMap<>();
return createToken(claims, username);
}
private String createToken(Map<String, Object> claims, String subject) {
return Jwts.builder().setClaims(claims).setSubject(subject)
.setIssuedAt(new Date(System.currentTimeMillis()))
.setExpiration(new Date(System.currentTimeMillis() + expiration))
.signWith(SignatureAlgorithm.HS256, secret).compact();
}
public Boolean validateToken(String token, String username) {
final String extractedUsername = extractUsername(token);
return (extractedUsername.equals(username) && !isTokenExpired(token));
}
private String extractUsername(String token) {
return extractAllClaims(token).getSubject();
}
private Claims extractAllClaims(String token) {
return Jwts.parser().setSigningKey(secret).parseClaimsJws(token).getBody();
}
private Boolean isTokenExpired(String token) {
return extractAllClaims(token).getExpiration().before(new Date());
}
}
Commentary on the Code
- Secret Management: The secret key is currently hardcoded; it’s recommended to manage it safely using environment variables or a configuration service.
- Claims: We are using claims to store user-related information. You can customize them as per your business needs.
- Expiration: The expiration time helps invalidate old tokens automatically.
Step 4: Rotating Secrets
To rotate secrets, follow these steps:
- Generate a new secret.
- Update the JWT secret in your properties or configuration management service.
- Implement a dual secret validation mechanism to support existing tokens until they expire.
Here's how you can modify the validateToken
method:
private Boolean validateToken(String token, String username) {
// First try with the current secret
try {
final String extractedUsername = extractUsername(token);
return (extractedUsername.equals(username) && !isTokenExpired(token));
} catch (Exception e) {
// If it fails, validate with the previous secret (assuming you have stored it)
try {
final String extractedUsername = extractUsernameWithOldSecret(token);
return (extractedUsername.equals(username) && !isTokenExpired(token));
} catch (Exception ignored) {
}
}
return false;
}
Best Practices for Secret Rotation
- Automate the Process: Whenever possible, automate secret rotation using CI/CD pipelines or cron jobs.
- Log Rotation Events: Maintain logs of when and how secrets are rotated for audit purposes.
- Secure Storage: Use secure storage solutions like HashiCorp Vault or AWS Secrets Manager.
Final Considerations
Secret rotation for JWT tokens is not just a recommendation; it is a necessity. Understanding the importance of securely managing and periodically changing your secrets can significantly reduce the risk of attacks on your application. Implementing a well-designed and robust secret rotation strategy within your Spring Boot application allows you to safeguard your JWT tokens effectively.
For further reading on the importance of securing JWTs, check out the OWASP JWT Cheat Sheet for comprehensive guidelines. Additionally, you might want to explore how to secure storage solutions via AWS Secrets Manager.
Incorporating these practices will enhance the security posture of your applications, enabling you to provide a safer environment for your users.
Feel free to experiment with the provided code snippets and tailor the structure to suit your development needs. Security in application development is an ongoing journey, and staying updated is vital. Happy coding!