The Limitations of Java 8 Default Methods Explained
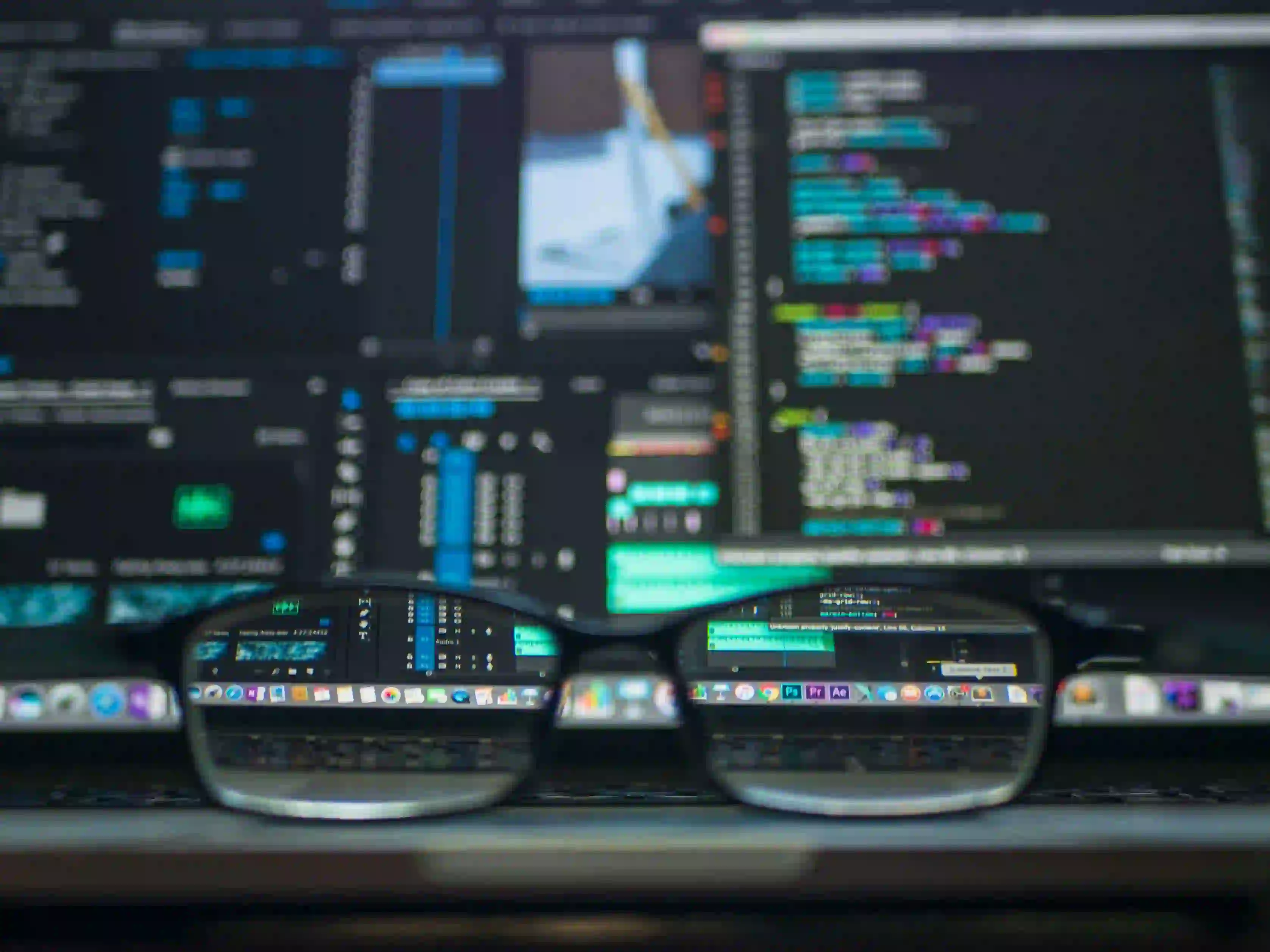
The Limitations of Java 8 Default Methods Explained
Java 8 introduced several key features that revolutionized the way we write and structure Java code. Among these, default methods in interfaces stand out for providing a way to add new functionality to interfaces without breaking backward compatibility. However, while they offer many advantages, default methods do come with their share of limitations.
In this post, we will explore the concept of default methods, their benefits, limitations, and some practical code snippets to illustrate their use. Let's dive right in!
Understanding Default Methods
Before tackling their limitations, it’s crucial to grasp what default methods are. A default method is a method defined in an interface that includes a body. By providing a default implementation, Java allows classes that implement the interface to inherit this method, reducing boilerplate code.
Example of a Default Method
Here’s a simple example that demonstrates the concept of default methods:
public interface Vehicle {
void start();
default void stop() {
System.out.println("Vehicle is stopping");
}
}
public class Car implements Vehicle {
@Override
public void start() {
System.out.println("Car is starting");
}
}
In this example, the Vehicle
interface defines a default method stop()
. The Car
class implements the Vehicle
interface but does not override the default stop()
method. Thus, if we create an instance of Car
and call stop()
, it will use the default implementation.
public class Main {
public static void main(String[] args) {
Vehicle myCar = new Car();
myCar.start(); // Car is starting
myCar.stop(); // Vehicle is stopping
}
}
Advantages of Default Methods
Before delving into the limitations, it’s worthwhile to summarize the advantages:
- Backward Compatibility: Add new functionalities to interfaces without breaking existing implementations.
- Code Reusability: Use default methods to avoid duplicate implementations across multiple classes.
- Enhanced Abstraction: Provide a way to specify common behavior while allowing flexibility in individual implementations.
Limitations of Default Methods
Despite these advantages, default methods come with several notable limitations:
1. Inability to Override Static Methods
In Java, static methods belong to the class or interface and cannot be overridden in the same way as instance methods. This means that if you have a static method in an interface, it cannot have a default implementation in subclasses.
public interface Vehicle {
static void maintain() {
System.out.println("Maintenance is required.");
}
}
public class Car implements Vehicle {
// Error: cannot override static method
}
Static methods in interfaces can be called as shown below, but they don't participate in polymorphism as instance methods do.
public class Main {
public static void main(String[] args) {
Vehicle.maintain(); // Maintenance is required.
}
}
2. Diamond Problem
The diamond problem arises when two interfaces inherit from the same superclass and provide different default implementations for the same method. In such cases, the implementing class must explicitly choose which implementation to use, leading to potential confusion.
interface A {
default void display() {
System.out.println("Display from A");
}
}
interface B extends A {
default void display() {
System.out.println("Display from B");
}
}
interface C extends A {
default void display() {
System.out.println("Display from C");
}
}
class D implements B, C {
@Override
public void display() {
B.super.display(); // Choose the implementation from B
}
}
In this example, class D
must choose which display
method to implement. Not handling this properly can lead to bugs or inconsistent behavior.
3. No Constructor in Interfaces
Interfaces cannot have constructors. Consequently, you cannot create an instance of a default method in the way you might with regular classes. This limits the flexibility of interfaces in specific contexts.
4. Method Scope
Default methods cannot access instance variables of an implementing class. They can, however, access static variables from the interface. This limitation can impact how interfaces interact with the implementation.
5. Interface Inheritance Complexity
As interfaces evolve, they can become complex. If multiple interfaces define default methods, keeping track of which method comes from where can lead to confusion. This complexity makes interfaces harder to maintain over time, especially in large codebases.
Practical Implications
Understanding these limitations is essential for any developer who employs default methods in their design. Let’s take a look at how to handle default methods while keeping limitations in mind.
Best Practices
- Use Default Methods Sparingly: Only when you need backward compatibility or common behavior across implementations.
- Be Explicit: In the case of the diamond problem, always clearly define your overriding behavior.
- Document Your Interfaces: Make sure other developers understand the purpose and usage of your default methods.
An Example of Careful Implementation
Let's consider an example where we effectively manage default methods and address potential pitfalls.
interface Drawable {
default void draw() {
System.out.println("Drawing a shape");
}
}
interface Colorable {
default void color() {
System.out.println("Coloring the shape");
}
}
class Circle implements Drawable, Colorable {
// Override the default draw method to provide specific functionality
@Override
public void draw() {
System.out.println("Drawing a Circle");
}
public void color() {
System.out.println("Coloring the Circle");
}
}
In this case, Circle
demonstrates the use of default methods effectively. It overrides draw()
while opting to use the color()
default method uniquely defined in Colorable
.
Final Thoughts
Default methods in Java 8’s interfaces bring a powerful toolset to the Java developer but come with limitations that need to be managed carefully. By understanding their constraints, including the inability to override static methods, diamond problems, and the lack of constructors, developers can more effectively utilize default methods without introducing unnecessary complexity.
For further reading on Java interfaces and default methods, consider visiting Oracle's official Java documentation or Baeldung's in-depth articles on Java.
By embracing both the benefits and the limitations of default methods, you can enhance your software's design while maintaining clarity and maintainability. Happy coding!