Common CI/CD Testing Pitfalls and How to Avoid Them
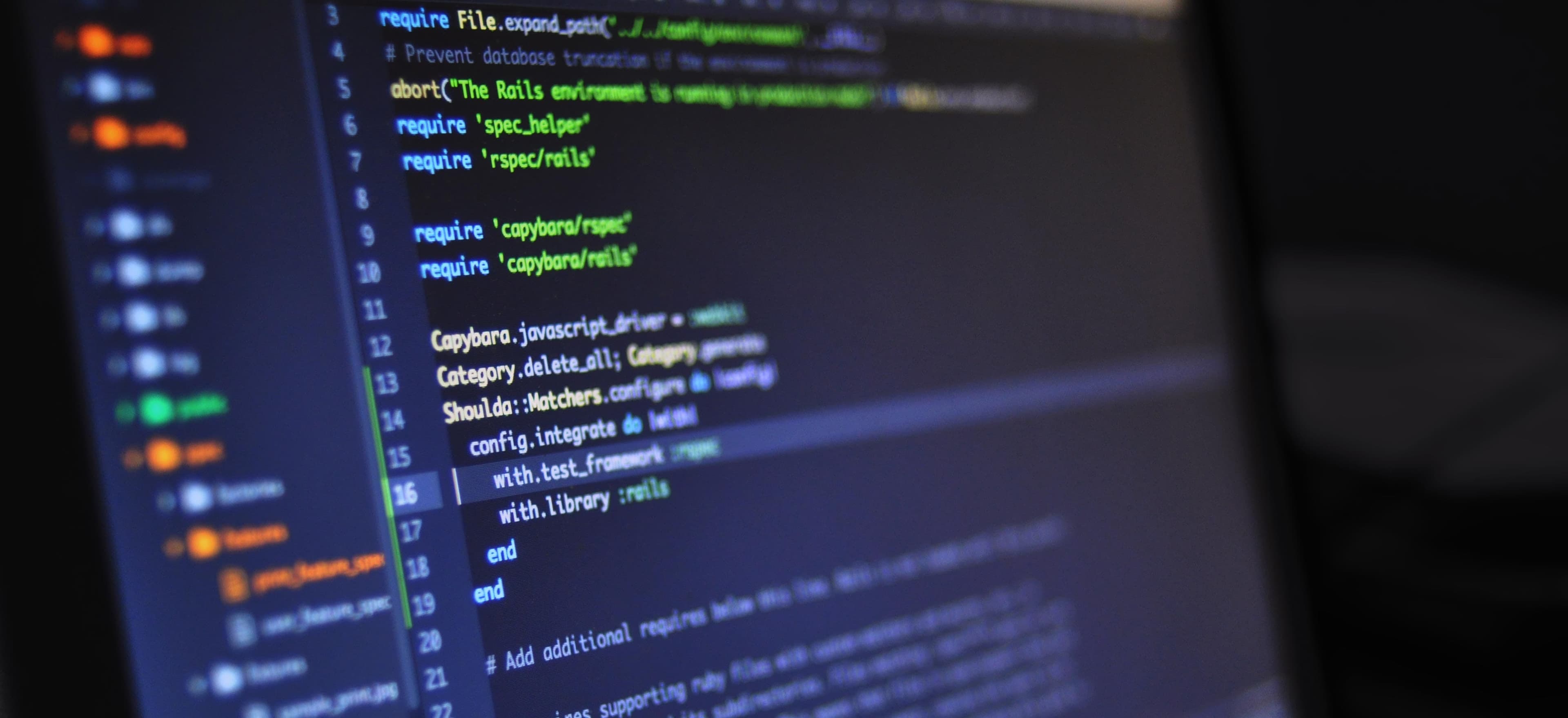
- Published on
Common CI/CD Testing Pitfalls and How to Avoid Them
Continuous Integration and Continuous Deployment (CI/CD) have transformed how software is developed and delivered. Integrating automated testing into your CI/CD pipeline is indispensable for maintaining quality. However, it often poses certain pitfalls. In this blog post, we'll explore these common CI/CD testing pitfalls and offer actionable solutions to avoid them.
Understanding CI/CD
Before diving into pitfalls, let's briefly revisit what CI/CD entails:
- Continuous Integration (CI): This practice involves automatically testing and merging code changes into a shared repository, enabling developers to detect issues early.
- Continuous Deployment (CD): This takes automation further by automatically deploying code changes to production after passing tests.
Together, CI/CD fosters a collaborative environment, improving code quality while accelerating deployment cycles.
Pitfall 1: Ignoring Testing in the Pipeline
The Issue
One common pitfall is overlooking the importance of automated tests in the CI/CD pipeline. Developers may skip tests, assuming that their local testing is sufficient. This leads to stubborn bugs making their way into production.
Solution
- Implement a Comprehensive Testing Strategy: Ensure that every code commit is accompanied by automated tests. This includes unit tests, integration tests, and end-to-end tests.
- Example Code Snippet:
@Test
public void testFunctionality() {
String expectedOutput = "Output expected";
String actualOutput = myClass.myFunction();
assertEquals(expectedOutput, actualOutput);
}
Why This is Important: Writing tests ensures that every part of your code is verified. The goal is to catch errors at the earliest stage possible.
Resources
Pitfall 2: Flaky Tests
The Issue
Flaky tests yield inconsistent results during repeated executions, causing confusion. They can mask genuine failures or lead to unnecessary reruns.
Solution
- Identify and Resolve Flaky Tests: Analyze your test suite to identify flaky tests and understand their root causes.
- Use Retry Logic Wisely: Implement retry logic for unreliable tests judiciously to prevent masking deeper issues.
@Test
public void flakyTestExample() {
assertDoesNotThrow(() -> myClass.mightFlake());
}
Why This is Important: Identifying flaky tests contributes to the credibility of your testing suite. If tests aren’t reliable, they can compromise your development process.
Resources
- Flaky Tests Documentation
Pitfall 3: Setting Up an Inconsistent Test Environment
The Issue
Inconsistent testing environments can lead to unanticipated bugs. Code may work on a developer's machine but fail in integration or staging environments.
Solution
- Use Containerization: Leverage Docker or Kubernetes to create consistent test environments. Containerization ensures that all dependencies are managed uniformly.
FROM openjdk:11
COPY target/my-app.jar my-app.jar
ENTRYPOINT ["java", "-jar", "my-app.jar"]
Why This is Important: Ensuring uniformity across environments reduces the risk of "it works on my machine" scenarios. It enhances reliability.
Resources
Pitfall 4: Not Testing Backward Compatibility
The Issue
Failing to test backward compatibility, especially in microservices architecture, can lead to significant issues post-deployment. New code may inadvertently break older parts of the system.
Solution
- Conduct Backward Compatibility Tests: Ensure that any new code can interact correctly with previous versions of the application.
- Example Code Snippet:
@Test
public void testBackwardCompatibility() {
Object oldBehavior = olderService.methodCall();
Object newBehavior = newService.methodCall();
assertEquals(oldBehavior, newBehavior);
}
Why This is Important: Ensuring backward compatibility adds robustness to your system. It prevents new changes from inadvertently wrecking existing functionality.
Resources
- Backward Compatibility Testing
Pitfall 5: Overlooking Load and Performance Testing
The Issue
Another pitfall is neglecting load testing until just before deployment. This can create bottlenecks and performance issues in production environments.
Solution
- Integrate Load Testing Early: Include performance and load testing in your CI/CD pipeline. Use tools like JMeter or Gatling to simulate expected usage patterns.
- Example Code Snippet (Gatling):
import io.gatling.core.Predef._
import io.gatling.http.Predef._
import scala.concurrent.duration._
val httpProtocol = http.baseUrl("http://myapp.com")
val scn = scenario("BasicLoadTest")
.exec(http("request_1").get("/api/resource"))
setUp(
scn.inject(atOnceUsers(10)).protocols(httpProtocol)
)
Why This is Important: Frequent load testing allows you to identify performance bottlenecks early, ensuring a smooth user experience upon release.
Resources
Pitfall 6: Lacking Clear Communication Among Team Members
The Issue
Poor communication in cross-functional teams can lead to misunderstandings about testing requirements, resulting in subpar QA practices.
Solution
- Establish Clear Communication Channels: Utilize tools like Slack, Microsoft Teams, or project management software to ensure clear and continuous communication among team members.
- Conduct Regular Stand-Ups: Short daily meetings can promote alignment and accountability in testing efforts.
Why This is Important: Effective communication fosters collaboration and enhances the overall quality of testing practices.
Resources
Pitfall 7: Not Analyzing Test Results
The Issue
Simply running tests and moving forward without analyzing results is a missed opportunity for improvement. Not all tests shine light on the underlying code health.
Solution
- Create Dashboards for Metrics: Use tools like Jenkins, CircleCI, or GitLab to visualize test results and code quality metrics.
- Regular Code Reviews: Foster a culture of regular code reviews to ensure that the team learns from failures and successes.
Why This is Important: Analysis allows teams to refine their processes over time and improve code quality and team productivity.
Resources
To Wrap Things Up
Incorporating automated testing into your CI/CD pipeline is crucial but rife with potential pitfalls. By recognizing common issues such as test neglect, flakiness, and the importance of performance testing, you can bolster your software quality assurance practices.
By adopting best practices suggested in this post, you'll foster a more reliable CI/CD process, enhance code quality, and ultimately deliver a better product. Remember, in CI/CD, quality is just as important as speed. Happy coding!
Further Reading
If you are interested in exploring more on CI/CD strategies or best practices for creating a robust testing culture, feel free to check out the following resources:
By adhering to these guidelines, you can sidestep these common pitfalls, ensuring a smoother and more efficient CI/CD experience.