Overcoming Challenges in Microservice Deployment Strategies
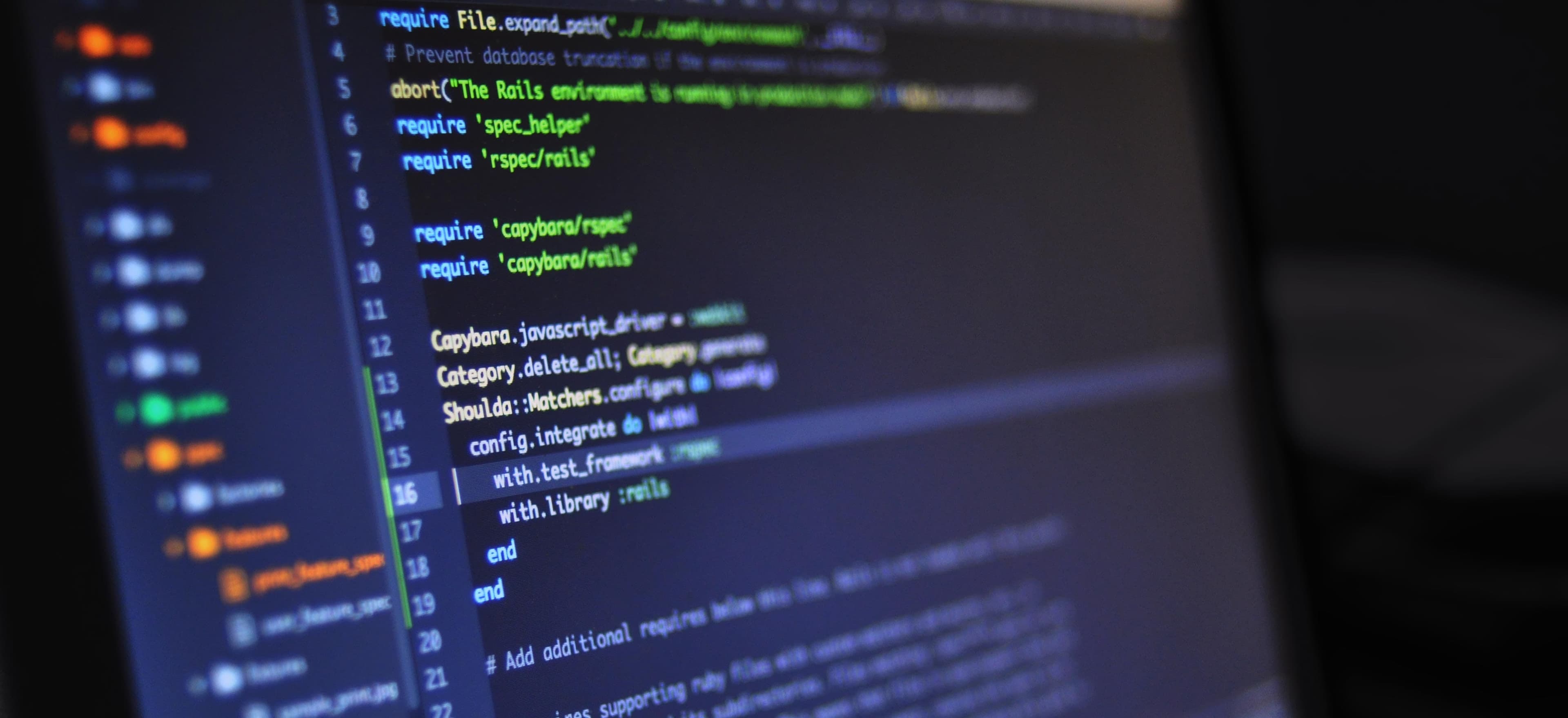
- Published on
Overcoming Challenges in Microservice Deployment Strategies
Microservices architecture has become the go-to strategy for developing applications that are scalable, flexible, and resilient. However, deploying microservices presents unique challenges. In this blog post, we will explore these challenges and offer effective deployment strategies, with examples and explanations to illustrate the concepts.
The Microservices Architecture Overview
Microservices architecture breaks down a single application into smaller, independent services, each responsible for a specific business function. This design allows for:
- Independent Deployment: Changes can be made to one service without affecting others.
- Technology Agnosticism: Different services can use different technologies suited to their needs.
- Scalability: Each service can be scaled independently based on its demands.
However, this decentralized nature introduces complexities in deployment and management.
Common Challenges in Microservice Deployment
1. Service Communication and Data Management
In microservices, services often need to communicate with one another. This brings challenges like ensuring service discovery, managing inter-service communication, and maintaining data consistency. When each service manages its own data, segregated data strategies like Event Sourcing or the Saga Pattern typically need to be implemented.
Example: Service Communication
In a Java application using Spring Boot, a REST client can be used to enable communication between services. Here is a simple example of a REST client using RestTemplate
.
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
@Service
public class UserService {
private final RestTemplate restTemplate;
public UserService(RestTemplate restTemplate) {
this.restTemplate = restTemplate;
}
public User getUserById(Long id) {
String url = "http://user-service/users/" + id;
return restTemplate.getForObject(url, User.class);
}
}
In this example, we fetch a user by calling another microservice. The RestTemplate
is a simple way to perform web service calls.
Why this is important:
Understanding how services communicate is crucial for designing resilient microservices systems that can handle failures and recover gracefully.
2. Deployment Automation and CI/CD Integration
Continuous Integration and Continuous Deployment (CI/CD) pipelines are essential for automating the build, testing, and deployment phases of microservices. This increases efficiency and reduces human error.
However, challenges arise from having numerous microservices. Configuring each service’s environment, dependency management, and ensuring proper orchestration can create hurdles.
CI/CD Pipeline Example:
Using Jenkins to automate the deployment of microservices could look like this:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Deploy') {
steps {
sh 'docker-compose up -d'
}
}
}
}
In this Jenkins example, we build our microservice using Maven and automatically deploy it using Docker Compose.
Why this is important:
A well-implemented CI/CD strategy helps you ensure that your microservices are delivered consistently without manual intervention, thus accelerating development speed.
3. Configuration Management
Managing configurations across multiple microservices can be tedious. Each service may require unique configurations based on its environment (development, testing, production).
Using tools such as Spring Cloud Config can simplify this process significantly.
Example: Using Spring Cloud Config
To centralize your configuration management, define a property file for your service:
# application.yml
spring:
application:
name: user-service
cloud:
config:
uri: http://localhost:8888
In this scenario, user-service
retrieves its configuration from a central configuration server.
Why this is important:
Configuration management ensures that your services are easily maintainable and can be modified independently without extensive redeployment.
4. Service Dependencies and Coordination
Microservices may have complex interdependencies. Managing these dependencies without tightly coupling services can be challenging. Techniques such as the Strangler Fig Pattern or Service Mesh can be used to address these issues effectively.
Example: Strangler Fig Pattern
If you are gradually migrating a monolithic application to a microservices architecture, you can use the Strangler Fig Pattern. This pattern allows you to slowly replace parts of the monolith with microservices.
Why this is important:
By adopting this pattern, you can migrate to microservices at your own pace without the risk of application downtime.
5. Logging and Monitoring
Monitoring microservices is critical due to their distributed nature. Traditional monitoring tools that work well for monolithic applications often fall short.
Implement solutions like ELK Stack (Elasticsearch, Logstash, and Kibana) or Prometheus for effective monitoring and logging.
Example: YAML Configuration for Prometheus
Below is a basic Prometheus configuration to scrape metrics from your services:
scrape_configs:
- job_name: 'spring-boot-microservice'
metrics_path: '/actuator/prometheus'
static_configs:
- targets: ['user-service:8080']
In this example, Prometheus is configured to scrape metrics from the microservice at the specified endpoint.
Why this is important:
Efficient logging and monitoring enable you to identify, trace, and fix issues quickly, minimizing downtime.
My Closing Thoughts on the Matter
While deploying microservices comes with its challenges, employing the right strategies can lead to successful implementation. Relying on robust service communication, implementing efficient CI/CD processes, managing configurations centrally, coordinating service dependencies adeptly, and ensuring comprehensive monitoring will drive your microservices deployment in the right direction.
By embracing these strategies and understanding the complexities involved, you can create resilient microservices that drive business value while keeping maintenance manageable.
For further reading on managing microservices, consider exploring these resources:
Embrace the microservices architecture and unlock the flexibility and scalability needed in modern application development!