Common Pitfalls in Oracle Java SE 7 Certification Exams
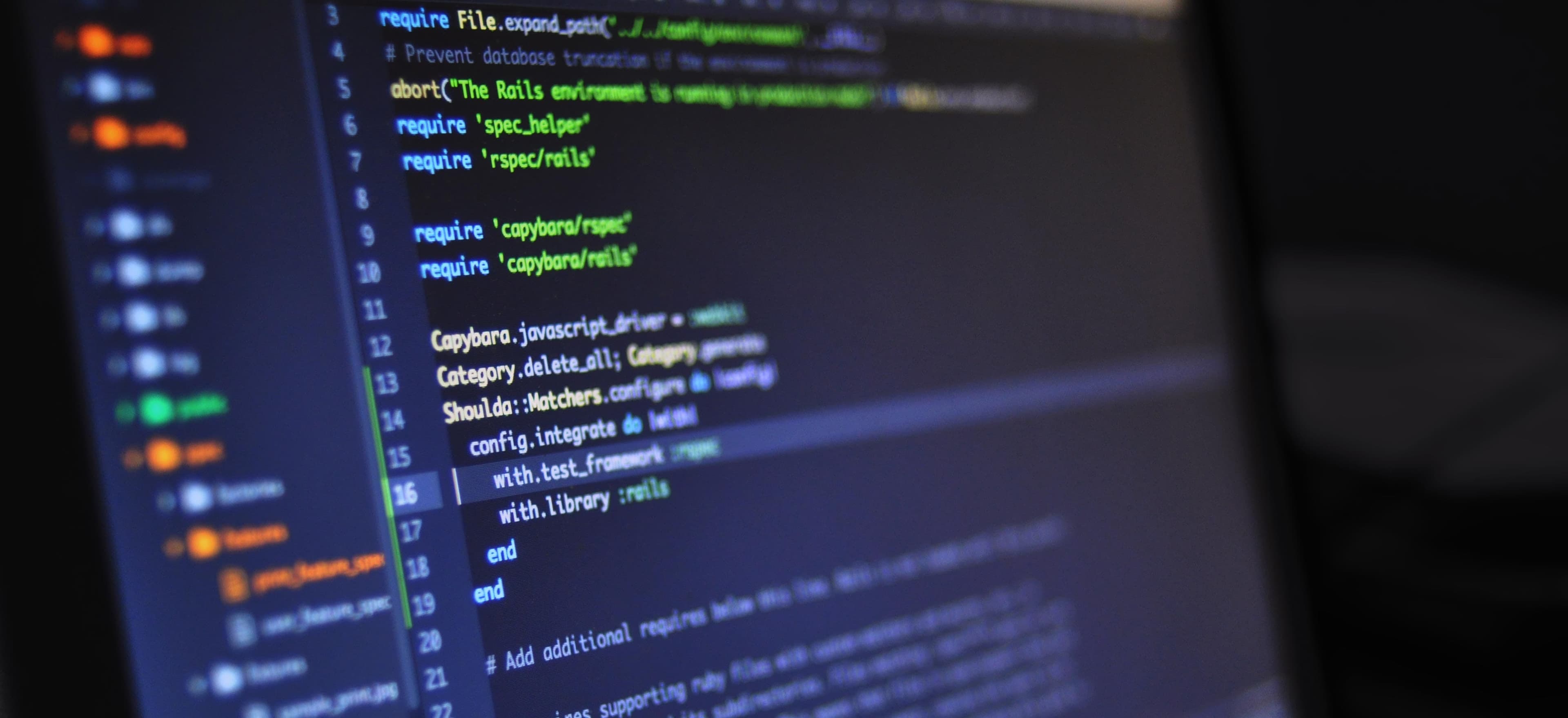
- Published on
Common Pitfalls in Oracle Java SE 7 Certification Exams
Preparing for the Oracle Java SE 7 Certification exams can be both exciting and overwhelming. With the right knowledge and preparation, many candidates can successfully pass the exam. However, numerous pitfalls can make this journey difficult. In this blog post, we will explore some common pitfalls candidates face and provide insights on how to navigate them effectively.
Understanding the Exam Structure
Before diving into common pitfalls, it's essential to understand the structure of the Oracle Java SE 7 Certification exam (1Z0-804). The exam primarily consists of multiple-choice questions, covering a wide range of topics related to the Java programming language.
Topics Covered
- Java fundamentals
- Object-oriented programming concepts
- Exception handling
- Collections framework
- Java I/O (Input/Output)
- Concurrency
- Assertions and enums
Understanding these topics will help you focus your study materials effectively.
Common Pitfalls and How to Avoid Them
1. Ignoring Core Java Concepts
One of the first pitfalls is neglecting core Java concepts. Many candidates underestimate the depth of knowledge required. It's crucial to have a solid grasp of the fundamentals.
What to Do: Try to break down each core concept into simpler chunks. For instance, if you're studying Object-Oriented Programming, understand each principle—encapsulation, inheritance, polymorphism, etc.
Example Code Snippet:
class Animal {
void sound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
void sound() {
System.out.println("Dog barks");
}
}
Why this matters: This demonstrates polymorphism. While the reference type is Animal, the actual method that gets executed is resolved at runtime, based on the object type (Dog).
2. Overlooking the Java API Documentation
Candidates often overlook the importance of the official Java API documentation. Familiarity with the documentation can save you time and help you troubleshoot effectively.
What to Do: Regularly refer to the Java SE 7 API Documentation during your study sessions.
Example Code Snippet:
import java.util.ArrayList;
public class Example {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
list.add("Java");
System.out.println(list.get(0));
}
}
Why this matters: The ArrayList
class allows dynamic arrays that can grow as needed. Understanding such classes deeply will enhance your performance in the exam.
3. Neglecting to Practice Coding
The exam doesn't just test theoretical knowledge – it requires practical understanding. Many candidates fail to spend enough time coding.
What to Do: Implement what you learn through regular coding exercises on platforms like LeetCode or HackerRank.
Example Code Snippet:
public class Fibonacci {
public static void main(String[] args) {
int n = 10, t1 = 0, t2 = 1;
System.out.print("Fibonacci Series: " + t1 + ", " + t2);
for (int i = 2; i < n; ++i) {
int nextTerm = t1 + t2;
System.out.print(", " + nextTerm);
t1 = t2;
t2 = nextTerm;
}
}
}
Why this matters: Practicing coding helps in understanding algorithms and enhances your problem-solving skills.
4. Ignoring the Importance of Exception Handling
Many candidates fail to grasp the significance of exception handling, resulting in poor performance.
What to Do: Focus on how exceptions work in Java, including checked versus unchecked exceptions.
Example Code Snippet:
import java.util.Scanner;
public class ExceptionExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
try {
int num = scanner.nextInt();
System.out.println("You entered: " + num);
} catch (java.util.InputMismatchException e) {
System.out.println("That's not a valid number!");
}
}
}
Why this matters: Proper exception handling leads to more robust applications, demonstrating essential skills during the exam.
5. Misunderstanding Concurrency Concepts
Concurrency can be tricky; many candidates hesitate when tackling questions involving threads and synchronization.
What to Do:
Study the java.lang.Thread
class and the java.util.concurrent
package in detail.
Example Code Snippet:
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
public class ThreadExample {
public static void main(String[] args) throws InterruptedException {
Counter counter = new Counter();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) counter.increment();
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) counter.increment();
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println("Count: " + counter.getCount());
}
}
Why this matters: Proper synchronization is crucial for reliable multi-threaded applications, which may appear in exam scenarios.
6. Underestimating Importance of Mock Exams
Candidates often underestimate mock exams, thinking they can pass by reviewing materials alone.
What to Do: Take multiple mock exams under timed conditions to get a real feel for the test environment.
Where to Find Mock Exams: Websites such as Whizlabs and Udemy offer excellent mock test materials.
7. Poor Time Management During the Exam
Time management is critical during exams. A common mistake is spending too much time on complex questions.
What to Do: Practice pacing yourself during mock exams. If a question takes too long, consider moving on and returning to it later.
Final Thoughts
Navigating the Oracle Java SE 7 Certification exam requires a delicate balance of study, practice, and application. By understanding common pitfalls, you can enhance your chances of success. Remember to study the core concepts thoroughly, leverage the Java API documentation, practice coding consistently, and never underestimate the value of mock exams.
As you pursue this certification, keep your focus sharp, and best of luck with your preparations!
For further reading on Java, consider checking out Oracle's official Java documentation. Dive deep into the resources available, and equip yourself for success.