Common Type Conversion Mistakes in Spring Framework
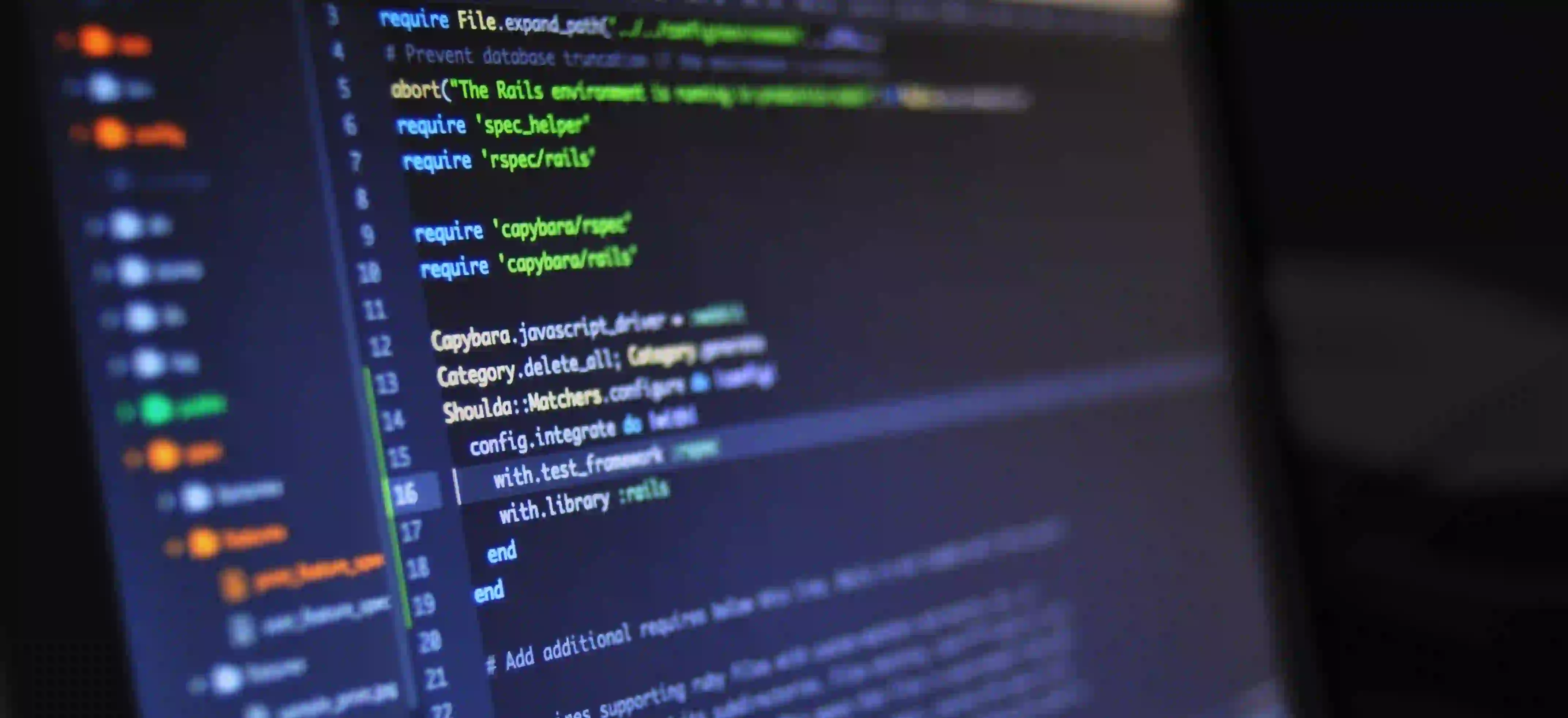
Common Type Conversion Mistakes in Spring Framework
Type conversion is a fundamental aspect of programming, especially when interacting with various data types in Java. The Spring Framework provides a robust mechanism for type conversion, which is essential for binding request parameters to Java objects. However, many developers encounter common pitfalls when dealing with type conversion in Spring. In this blog post, we will explore these pitfalls, how to avoid them, and showcase best practices.
Understanding Type Conversion in Spring
Before diving into the mistakes, let's clarify what type conversion is. In Spring, type conversion is the process of converting a value from one type to another. For instance, converting a string input from an HTTP request into an integer for processing.
Spring provides a Converter
interface and a ConversionService
that allows for flexible, reusable type conversion.
Pitfall 1: Ignoring Default Converters
One of the most common mistakes is not leveraging Spring's built-in conversion capabilities. Spring has several default converters out of the box, including those for converting strings to dates, numbers, and even custom object types.
Example:
import org.springframework.core.convert.converter.Converter;
import org.springframework.stereotype.Component;
@Component
public class StringToDateConverter implements Converter<String, Date> {
@Override
public Date convert(String source) {
try {
return new SimpleDateFormat("yyyy-MM-dd").parse(source);
} catch (ParseException e) {
throw new IllegalArgumentException("Invalid date format");
}
}
}
Why: Here we create a custom converter that can convert string representations of dates into actual Date
objects. If you ignore these defaults and write your own conversion logic, you risk duplicating effort.
Pitfall 2: Not Handling Conversion Errors Gracefully
Another common mistake is failing to address conversion errors. Without proper error handling, a small mistake in input can lead to application crashes or unexpected behavior.
Example:
@RequestMapping(value = "/submit", method = RequestMethod.POST)
public String submit(@RequestParam("age") Integer age) {
if (age < 0) {
throw new IllegalArgumentException("Age cannot be negative");
}
// Continue processing...
}
Why: Always validate input after conversion. Here we ensure that the converted age
isn't negative, thus preventing possible logical errors later in the application flow.
Pitfall 3: Using Primitive Types Instead of Wrappers
Another frequent issue arises when developers use primitive types instead of wrapper classes. This choice can lead to NullPointerExceptions
if a value is not provided in an HTTP request.
Example:
@RequestMapping(value = "/register", method = RequestMethod.POST)
public String register(@RequestParam("age") Integer age) {
// process registration
return "registrationSuccess";
}
Why: Using Integer
instead of int
allows your application to gracefully handle null
values when the 'age' parameter is absent. This way your code won't throw an exception upon returning a null value.
Pitfall 4: Underestimating the Need for Custom Converters
Developers often underestimate the situation where built-in converters are insufficient. This can lead to confusion and runtime errors.
Example:
public class StringToUserConverter implements Converter<String, User> {
@Override
public User convert(String source) {
String[] parts = source.split(",");
return new User(parts[0], Integer.parseInt(parts[1]));
}
}
Why: Here, we create a custom converter that takes a string formatted as name,age
and converts it to a User
object. Relying solely on built-in converters would not suffice here, showcasing the need for custom conversions when dealing with complex data structures.
Pitfall 5: Failing to Setup Application Properties
Type-conversion failures may also stem from incorrect or missing application.properties
configurations. Remember to define any custom property editors if you're not using Spring's default mechanism.
Example:
# application.properties
spring.mvc.data-binding.default-initiator=true
Why: Properly configuring application properties can prevent type conversion issues from propelling the application into unexpected behavior, thus enhancing reliability.
Best Practices for Type Conversion in Spring
-
Utilize Built-in Converters: Always start by leveraging Spring's built-in types to handle basic conversions.
-
Implement Custom Converters When Necessary: For complex conversions that default converters cannot handle, consider creating custom converters.
-
Validate Input Properly: After conversion, validate the input to avoid logical errors.
-
Use Wrapper Types: Always opt for wrapper types over primitives to handle nullable inputs gracefully.
-
Log Conversion Errors: Implement logging for conversion errors for easier debugging and maintainability.
For a deeper comprehension of customization in Spring, refer to Spring's Documentation on Type Conversion.
Final Considerations
Type conversion is an integral part of working with data in the Spring Framework. Avoiding common pitfalls like ignoring built-in converters, failing to handle errors, and not using wrapper types can significantly enhance your application's performance and reliability. By following the best practices outlined in this post, developers can seamlessly manage type conversions and avoid costly mistakes.
For further reading, check out the official Spring MVC Guide for insights on handling form submissions and understanding request parameter bindings.
By focusing on proper type conversion practices, you can ensure that your Spring applications remain robust, efficient, and easy to maintain. With the right tools and approaches, you can eliminate common type-conversion mistakes and enhance the functionality of your applications!