Finding the Best Deployment Strategy for Your Project
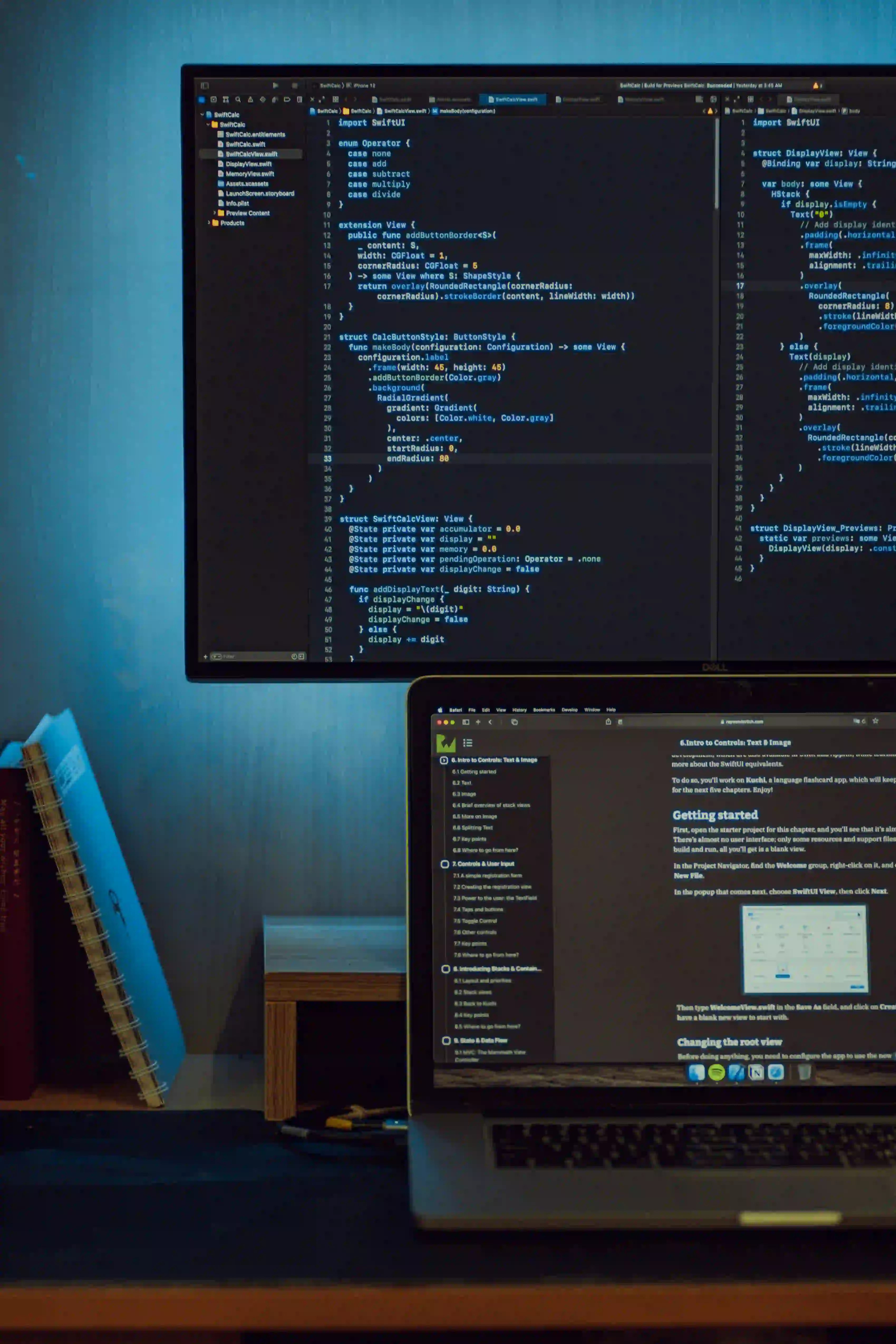
Finding the Best Deployment Strategy for Your Project
Deployment is a crucial phase in software development. It's the process through which your application moves from development to production, ultimately delivered to users. Selecting the right deployment strategy can significantly influence your project's success in terms of performance, reliability, and user experience.
In this extensive guide, we'll explore various deployment strategies, examine their benefits and drawbacks, and provide you with code snippets and real-life examples to help you make an informed decision for your project.
Understanding Deployment Strategies
Before diving into specific strategies, let's clarify what deployment strategies entail. A deployment strategy is a systematic approach that outlines how software updates are rolled out to production environments. Your chosen strategy affects how users perceive updates, the overall application performance, and the risk factor surrounding potential bugs.
Common Deployment Strategies
- Blue-Green Deployment
- Canary Deployment
- Rolling Deployment
- Recreate Deployment
- Shadow Deployment
1. Blue-Green Deployment
With Blue-Green deployment, two identical environments exist: one ("Blue") serving the live production traffic, and the other ("Green") where the new version of the application is deployed and tested. Once the new version is verified, traffic is switched from Blue to Green.
Advantages:
- Reduced Downtime: Users experience minimal disruption during deployments.
- Instant Rollback: If issues arise, quickly switch back to the Blue environment.
Disadvantages:
- Resource Intensive: Maintaining two identical environments might increase costs.
Example Code:
# Simulating Blue-Green deployment using AWS CLI
aws elasticbeanstalk swap-environments --source-environment-name Blue-env --destination-environment-name Green-env
The above command efficiently swaps the two environments in AWS Elastic Beanstalk, ensuring zero downtime.
2. Canary Deployment
A canary deployment entails rolling out the new version of an application to a small subset of users before a full-scale release. This method allows teams to monitor the new version’s performance and gather feedback.
Advantages:
- Early Detection: Identifies any potential issues with minimal user impact.
- Gradual Exposure: Reduces risk by gradually increasing the user base.
Disadvantages:
- User Variability: The feedback mechanism might be skewed if the canary group is not representative.
Example Code:
# Kubernetes example of Canary Deployment
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp
image: myapp:v2
The above Kubernetes manifest showcases how to deploy the new version while keeping the original running. Adjust replicas
as needed to create an effective canary strategy.
3. Rolling Deployment
This strategy entails updating a few instances at a time. For example, if your application has ten instances, a rolling deployment will update two or three, ensuring the application remains available during the process.
Advantages:
- Continuous Availability: At least a portion of the service remains online.
- Controlled Rollout: Provides opportunities to monitor system performance and functionality during deployment.
Disadvantages:
- Complexity: Managing a progressive rollout can complicate monitoring and testing.
Example Code:
# A simpler approach to a rolling deployment in Kubernetes
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 10
strategy:
type: RollingUpdate
rollingUpdate:
maxUnavailable: 1
maxSurge: 1
selector:
matchLabels:
app: myapp
template:
metadata:
labels:
app: myapp
spec:
containers:
- name: myapp
image: myapp:latest
This configuration allows for the rolling update process, guaranteeing that only one pod is updated at a time.
4. Recreate Deployment
In a recreate deployment, the current version of the application is completely stopped before the new version starts. This offers a straightforward approach but can lead to significant downtime.
Advantages:
- Simple Implementation: Easy to understand and execute.
Disadvantages:
- Downtime: Users will experience a break in service during updates.
Example Code:
# Using Docker Compose for Recreate Deployment
docker-compose down
docker-compose up --build -d
Stopping the existing containers first ensures that no old versions run while the new one is being deployed.
5. Shadow Deployment
Shadow deployments involve running a new version of the application alongside the current live version but without impacting end-users. Requests sent to the live version are also sent to the new version, allowing for testing under real production conditions.
Advantages:
- Real-World Testing: Provides valuable insights into the new version without user impact.
- Immediate Feedback: If sufficient data is gathered, the new version can be modified before launch.
Disadvantages:
- Resource Usage: Additional resources are used since both versions run simultaneously.
Example Code:
# A simplified shadow deployment snippet using Flask
from flask import Flask, request
app = Flask(__name__)
@app.route('/api/data', methods=['GET'])
def get_data():
# Old version logic
pass
@app.route('/api/data', methods=['POST'])
def post_data():
# New version logic running behind the scenes
response = new_version_post_logic(request.json) # Not impacting users yet
return response
In this example, the existing functionality remains intact while you simultaneously test the new logic.
Bringing It All Together: Choosing the Right Strategy
When selecting a deployment strategy for your project, consider the following factors:
- Project Size & Complexity: Larger applications might benefit from advanced strategies like Blue-Green or Canary deployments.
- Team Expertise: Ensure your team possesses the necessary skills to implement and maintain the chosen strategy.
- Budget Constraints: Resource-intensive methodologies may not fit every project's financial plan.
- User Experience: Ultimately, maintaining a seamless experience for end-users should always be a priority.
By weighing these factors alongside the descriptions and examples provided here, you are better positioned to choose a deployment strategy that fits your project's unique needs.
For further reading, check out this resources on Modern Deployment Strategies and Kubernetes Best Practices. These links can deepen your understanding of deployment methodologies in varying contexts.
Happy deploying!