Overcoming Common Issues with REST Pagination in Spring
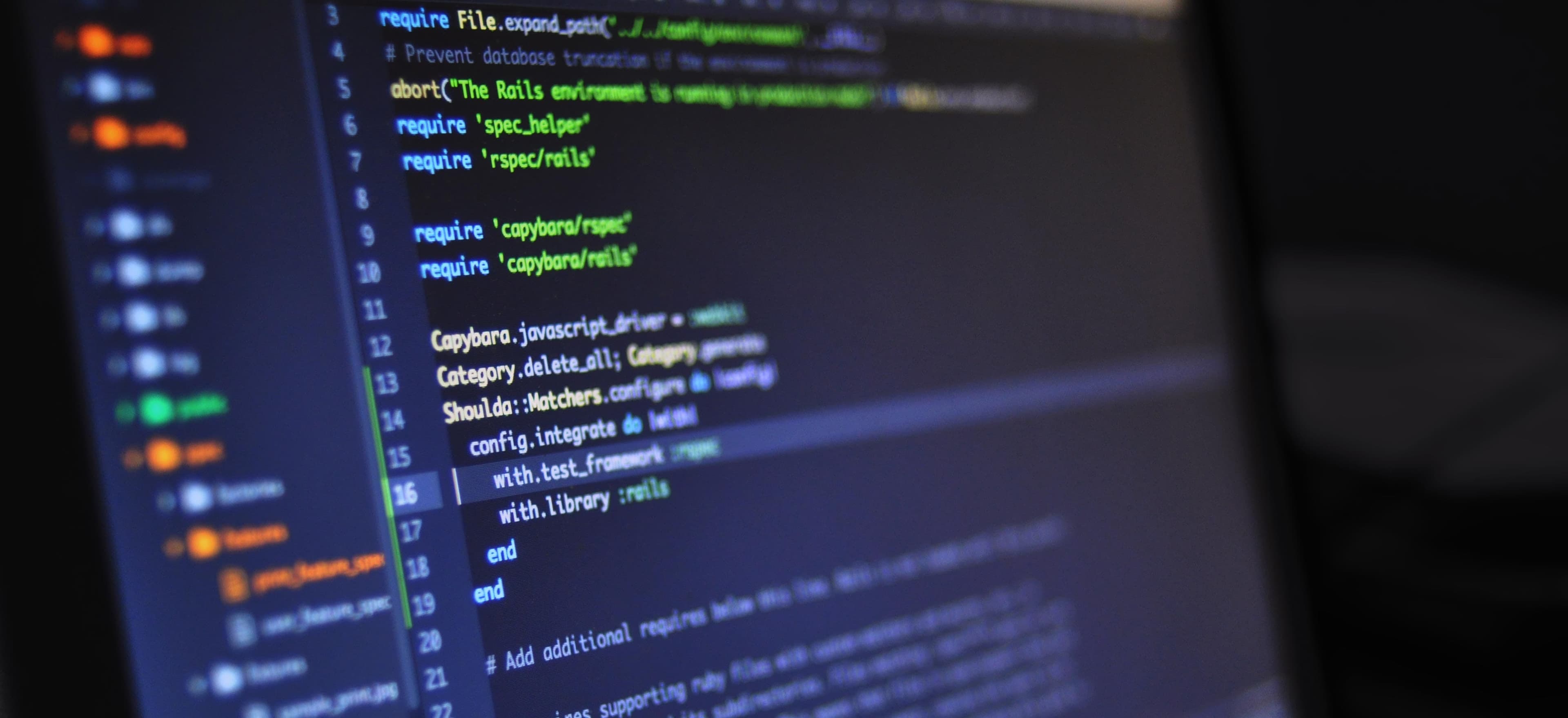
- Published on
Overcoming Common Issues with REST Pagination in Spring
RESTful APIs have become the backbone of modern web development. As the volume of data we handle grows, implementing efficient pagination in your APIs is essential for performance and usability. In a Spring application, REST pagination can sometimes present challenges. In this blog post, we'll discuss some common issues developers face with REST pagination in Spring and how to overcome them.
Understanding REST Pagination
Before delving into the problems, let's briefly review what pagination means. Pagination refers to the process of dividing a large dataset into smaller, more manageable chunks, or pages. The primary goal of pagination is to improve the performance of your API and the user experience by reducing data retrieval times.
In a RESTful API, pagination typically involves parameters such as:
page
: The number of the page to fetch.size
: The number of records per page.
Creating a Spring REST API with Pagination
To demonstrate the implementation of pagination using Spring, we first need to set up a basic Spring Boot application.
- Add Dependencies
In your pom.xml
, include the necessary dependencies for Spring Boot and Spring Data JPA:
<depency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>h2</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
- Define a Model
Let’s create a simple entity, Product
, to showcase pagination:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private double price;
// Constructors, Getters and Setters
}
- Create a Repository
Now, we'll create a repository interface that extends JpaRepository
:
import org.springframework.data.jpa.repository.JpaRepository;
public interface ProductRepository extends JpaRepository<Product, Long> {
}
- Develop the Controller
To expose our API endpoint, we need to build a controller:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageRequest;
import org.springframework.data.domain.Pageable;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class ProductController {
@Autowired
private ProductRepository productRepository;
@GetMapping("/products")
public ResponseEntity<Page<Product>> getProducts(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
Pageable pageable = PageRequest.of(page, size);
Page<Product> productPage = productRepository.findAll(pageable);
return ResponseEntity.ok(productPage);
}
}
How the Controller Works
In the code above:
- We define a GET endpoint
/products
that fetches products. - It accepts
page
andsize
as request parameters, with default values of0
and10
, respectively. - A Pageable object is created using
PageRequest.of()
, which helps manage pagination seamlessly. - Finally,
findAll(pageable)
fetches a page of products.
Common Issues with Pagination in Spring
Now that we have the basics down, let’s address some common issues developers encounter when implementing pagination in Spring.
1. Incorrect Page Size
Issue: Users might request a page size of zero or a negative number, leading to unexpected behaviors or errors.
Solution: Validate input parameters to ensure page size is a positive integer and at least 1.
@GetMapping("/products")
public ResponseEntity<Page<Product>> getProducts(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
if (page < 0) {
throw new IllegalArgumentException("Page number cannot be negative.");
}
if (size <= 0) {
throw new IllegalArgumentException("Page size must be greater than zero.");
}
Pageable pageable = PageRequest.of(page, size);
Page<Product> productPage = productRepository.findAll(pageable);
return ResponseEntity.ok(productPage);
}
2. Returning Excessive Data
Issue: Pagination can inadvertently return too much data, especially when default sizes are used during development.
Solution: Always consider implementing limits on the maximum page size to prevent performance degradation.
public ResponseEntity<Page<Product>> getProducts(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
int maxSize = 100; // Define a maximum limit for the page size
size = Math.min(size, maxSize);
// Continue with pagination logic...
}
3. Total Count of Records
Issue: Users often need to know the total number of records available, which is beyond the current page.
Solution: The Spring Data Page
object contains this information, so require it be included in the API response.
public ResponseEntity<Page<Product>> getProducts(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size) {
Pageable pageable = PageRequest.of(page, size);
Page<Product> productPage = productRepository.findAll(pageable);
// Return response with total elements and pages metadata
return ResponseEntity.ok(productPage);
}
4. Increasing Complexity with Sorting
Issue: As applications grow, the need for sorting alongside pagination emerges, complicating the queries.
Solution: You can extend the PageRequest
with sorting options. Users can pass in sort parameters like "sortBy" and "direction".
import org.springframework.data.domain.Sort;
@GetMapping("/products")
public ResponseEntity<Page<Product>> getProducts(
@RequestParam(defaultValue = "0") int page,
@RequestParam(defaultValue = "10") int size,
@RequestParam(defaultValue = "id") String sortBy,
@RequestParam(defaultValue = "asc") String direction) {
Sort sort = Sort.by(Sort.Direction.fromString(direction), sortBy);
Pageable pageable = PageRequest.of(page, size, sort);
Page<Product> productPage = productRepository.findAll(pageable);
return ResponseEntity.ok(productPage);
}
Additional Considerations
- Caching: Implementing caching can drastically reduce load times for frequently accessed data.
- Consistent API Design: Follow the REST API Design Best Practices for a user-friendly API structure.
- Query Parameters: Utilize a consistent structure for query parameters in your pagination requests for better readability.
Closing the Chapter
Implementing pagination in Spring's REST APIs is vital for ensuring smooth and efficient data access. By addressing common issues like input validation, data limits, metadata inclusion, and sort functionality, you can build a robust and user-friendly paging system.
Remember to always test for edge cases in your pagination logic to ensure reliability. Happy coding!
For further reading on RESTful APIs and data pagination, explore the Spring Framework Documentation or Spring Data JPA documentation for more in-depth resources.
Checkout our other articles