Struggling with Architecture Metrics? Here’s How to Improve!
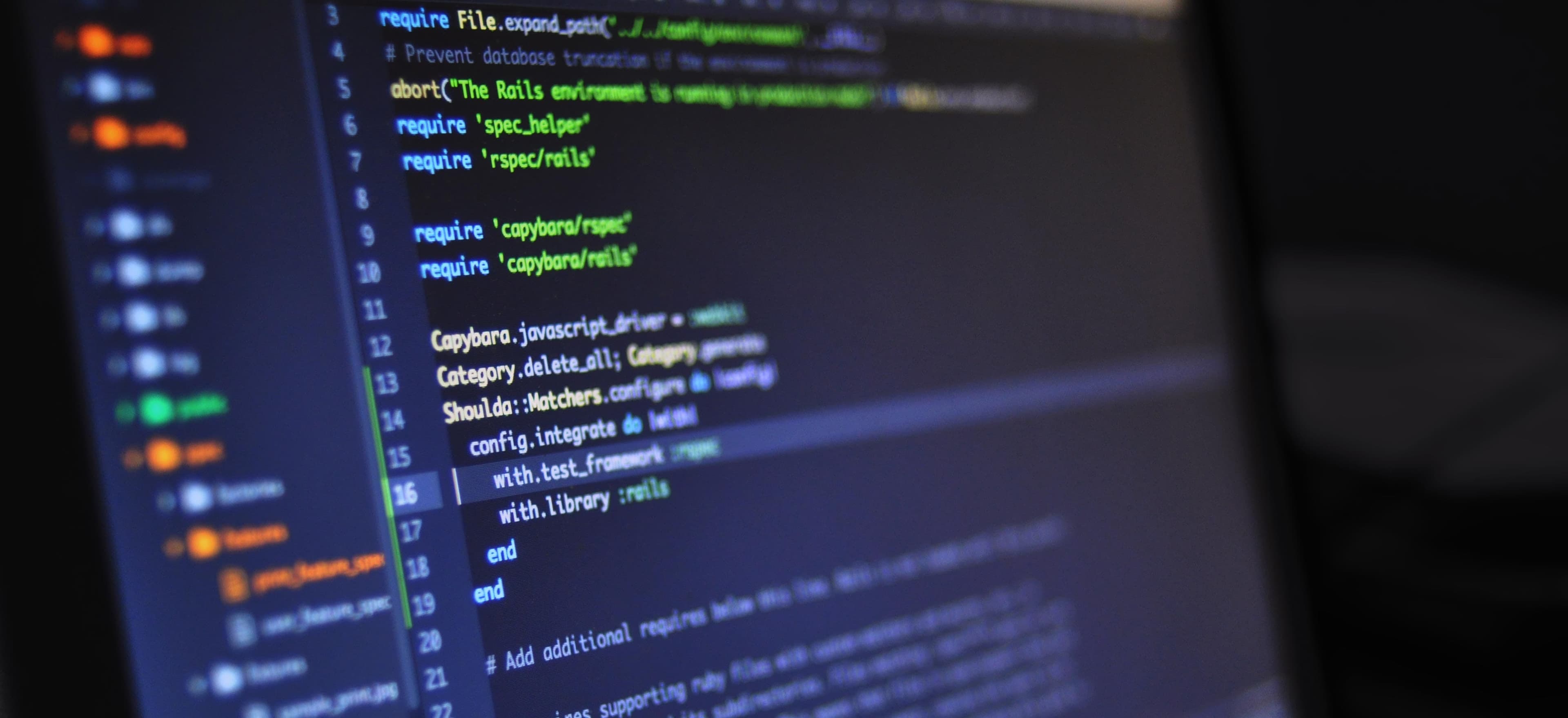
- Published on
Struggling with Architecture Metrics? Here’s How to Improve!
In today's fast-paced technology landscape, software architecture plays a vital role in determining the success of a project. While architecture defines the structure and design of your software, metrics are the compass guiding your architectural decisions. This blog post will delve into architecture metrics, common pitfalls, and strategies to enhance your measurement process effectively.
Understanding Architecture Metrics
Architecture metrics provide quantitative measures that help assess the quality and performance of software architecture. These metrics aid in making informed decisions on design, implementation, and maintenance throughout the software development life cycle (SDLC).
Key Metrics to Consider
-
Modularity: This measures how well your architecture separates concerns. A modular architecture allows for easier updates and modifications.
-
Coupling and Cohesion:
- Coupling measures how interdependent different modules are. Lower coupling is better, as it indicates that modules can be developed independently.
- Cohesion, on the other hand, evaluates how closely related the functions within a module are. Higher cohesion is preferred to ensure that a module handles a single responsibility effectively.
-
Code Complexity: This includes various measures, such as Cyclomatic Complexity. A lower complexity generally indicates code that is easier to test and maintain.
-
Performance Metrics: Metrics like response time, throughput, and resource utilization give insights into how well a system performs under different loads.
-
Technical Debt: This metric helps you understand how much work is required to return to a clean code state from the current state. High technical debt indicates that paying down that debt should be a priority.
When Metrics Go Wrong
The very existence of metrics can sometimes lead to misguided decisions if not used correctly.
-
Focusing on the Wrong Metrics: Organizations often emphasize metrics that do not align with their business objectives. For example, concentrating solely on code coverage can negate the importance of code quality and usability.
-
Over-Reliance on Quantitative Measures: While metrics provide valuable information, relying too much on numbers can overshadow qualitative factors important for architecture.
-
Ignoring Context: Different projects have different requirements. A metric that may be beneficial in one context might be detrimental in another.
Strategies to Improve Architecture Metrics
Here are several ways to bolster your architectural metrics processes and ensure they are effectively contributing to your development goals:
1. Align Metrics with Business Goals
Determine what your organization is trying to achieve, and align architecture metrics with those outcomes. For instance, if user experience is a priority, focus on performance metrics like response time.
2. Adopt Holistic Metrics
Instead of obsessing over singular metrics, consider a composite approach where multiple aspects are measured together. This can provide a clearer picture of architectural health.
public class Metrics {
private int responseTime; // in milliseconds
private int throughput; // requests per second
public Metrics(int responseTime, int throughput) {
this.responseTime = responseTime;
this.throughput = throughput;
}
public boolean isPerformanceAcceptable() {
return responseTime < 200 && throughput > 100;
}
}
In the above code, we create a simple Metrics
class that encapsulates performance metrics. The isPerformanceAcceptable
method checks whether the performance meets set thresholds.
3. Implement Automated Testing
Automation is crucial for maintaining consistent measurement—use integration tests, unit tests, and end-to-end tests to gather metrics throughout the SDLC. The code below demonstrates a simple JUnit test for a service class.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
class ServiceTest {
@Test
void testServicePerformance() {
Service service = new Service();
long duration = service.executeTask();
assertEquals(/*expected performance threshold*/, duration);
}
}
This code snippet showcases how to set performance expectations within a test. By enforcing these checks, you can collect data on performance regularly.
4. Track Technical Debt
Tools like SonarQube can be invaluable for measuring technical debt. Understanding the implications of your architecture can help prioritize what to address first. The sooner you tackle your debt, the less it'll affect the project in the long run.
5. Continuous Monitoring
Incorporate tools for performance and error tracking in production. Real-time monitoring can help catch issues before they become significant problems. Solutions like New Relic and Grafana can be integrated for this purpose.
6. Foster a Culture of Feedback
Encourage your development team to contribute insights and perspective on the metrics being monitored. Continuous feedback can lead to better metrics and decision-making. Regular retrospective meetings can help identify what’s working and what isn’t.
Best Tools for Architecture Metrics
Choosing the right tools can significantly enhance your ability to collect and analyze architecture metrics. Here are a few popular tools:
- SonarQube: This platform inspects code quality and provides insights into potential vulnerabilities and technical debt.
- New Relic: Emphasizes real-time monitoring of application performance and user experience.
- JProfiler: Offers advanced profiling capabilities to diagnose performance issues in Java applications.
Closing the Chapter
Improving architecture metrics is an essential endeavor for teams aiming to deliver high-quality software swiftly and effectively. By aligning metrics with business goals, adopting a holistic approach, implementing automated testing, and fostering a culture of continuous improvement, developers can create a robust system for evaluating their architecture.
Metrics are not just numbers; they tell a story about your software's health and capabilities. Utilize them wisely, and you'll be able to steer your projects towards success.
For further reading on architecture metrics, visit these resources:
Don't let architectural woes hold you back; start monitoring effectively today!